Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / TypeSchema.cs / 1 / TypeSchema.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.Collections.Generic; using System.Data; using System.Diagnostics; using System.Reflection; ////// Represents a schema based on an arbitrary object. /// /// DataSets and DataTables are special cased and processed for their strongly-typed properties. /// Types that implement the generic IEnumerable<T> will use the bound type T as their row type. /// Arbitrary enumerables are processed using their indexer's type. /// All other objects are directly reflected on and their public properties are exposed as fields. /// /// DataSet and DataTable schema is retrieved by creating instances of the /// objects, whereas all other schema is retrived using Reflection. /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] public sealed class TypeSchema : IDataSourceSchema { private Type _type; private IDataSourceViewSchema[] _schema; public TypeSchema(Type type) { if (type == null) { throw new ArgumentNullException("type"); } _type = type; if (typeof(DataTable).IsAssignableFrom(_type)) { _schema = GetDataTableSchema(_type); } else { if (typeof(DataSet).IsAssignableFrom(_type)) { _schema = GetDataSetSchema(_type); } else { if (IsBoundGenericEnumerable(_type)) { _schema = GetGenericEnumerableSchema(_type); } else { if (typeof(IEnumerable).IsAssignableFrom(_type)) { _schema = GetEnumerableSchema(_type); } else { _schema = GetTypeSchema(_type); } } } } } public IDataSourceViewSchema[] GetViews() { return _schema; } ////// Gets schema for a strongly typed DataSet. /// private static IDataSourceViewSchema[] GetDataSetSchema(Type t) { try { DataSet dataSet = Activator.CreateInstance(t) as DataSet; System.Collections.Generic.Listviews = new System.Collections.Generic.List (); foreach (DataTable table in dataSet.Tables) { views.Add(new DataSetViewSchema(table)); } return views.ToArray(); } catch { return null; } } /// /// Gets schema for a strongly typed DataTable. /// private static IDataSourceViewSchema[] GetDataTableSchema(Type t) { try { DataTable table = Activator.CreateInstance(t) as DataTable; DataSetViewSchema tableSchema = new DataSetViewSchema(table); return new IDataSourceViewSchema[1] { tableSchema }; } catch { return null; } } ////// Gets schema for a strongly typed enumerable. /// private static IDataSourceViewSchema[] GetEnumerableSchema(Type t) { TypeEnumerableViewSchema enumerableSchema = new TypeEnumerableViewSchema(String.Empty, t); return new IDataSourceViewSchema[1] { enumerableSchema }; } ////// Gets schema for a generic IEnumerable. /// private static IDataSourceViewSchema[] GetGenericEnumerableSchema(Type t) { TypeGenericEnumerableViewSchema enumerableSchema = new TypeGenericEnumerableViewSchema(String.Empty, t); return new IDataSourceViewSchema[1] { enumerableSchema }; } ////// Gets schema for an arbitrary type. /// private static IDataSourceViewSchema[] GetTypeSchema(Type t) { TypeViewSchema typeSchema = new TypeViewSchema(String.Empty, t); return new IDataSourceViewSchema[1] { typeSchema }; } ////// Returns true if the type implements IEnumerable<T> with a bound /// value for T. In other words, this type: /// public class Foo<T> : IEnumerable<T> { ... } /// Does not bind the value of T. However this type: /// public class Foo2 : IEnumerable<string> { ... } /// Does have T bound to the type System.String. /// /// Although it would seem that this check: /// typeof(IEnumerable<>).IsAssignableFrom(t) /// Might be sufficient, it actually doesn't work, possibly due /// to covariance/contravariance limitations, etc. /// internal static bool IsBoundGenericEnumerable(Type t) { Type[] interfaces = null; if (t.IsInterface && t.IsGenericType && t.GetGenericTypeDefinition() == typeof(IEnumerable<>)) { // If the type already is IEnumerable, that's the interface we want to inspect interfaces = new Type[] { t }; } else { // Otherwise we get a list of all the interafaces the type implements interfaces = t.GetInterfaces(); } foreach (Type i in interfaces) { if (i.IsGenericType && i.GetGenericTypeDefinition() == typeof(IEnumerable<>)) { Type[] genericArguments = i.GetGenericArguments(); Debug.Assert(genericArguments != null && genericArguments.Length == 1, "Expected IEnumerable to have a generic argument"); // If a type has IsGenericParameter=true that means it is not bound. return !genericArguments[0].IsGenericParameter; } } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
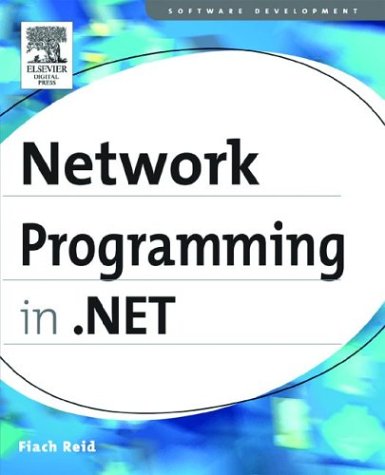
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CallbackValidator.cs
- CalendarKeyboardHelper.cs
- WindowsListBox.cs
- DataQuery.cs
- WebPartConnectionsConnectVerb.cs
- WSSecurityOneDotZeroReceiveSecurityHeader.cs
- AttachmentCollection.cs
- ZipIOExtraFieldPaddingElement.cs
- ServicePointManagerElement.cs
- NetworkStream.cs
- Win32KeyboardDevice.cs
- RpcAsyncResult.cs
- ImmComposition.cs
- Rss20FeedFormatter.cs
- Attributes.cs
- ReadOnlyDataSourceView.cs
- AndCondition.cs
- IDReferencePropertyAttribute.cs
- InteropExecutor.cs
- EmptyEnumerable.cs
- FolderBrowserDialogDesigner.cs
- XsltQilFactory.cs
- DynamicScriptObject.cs
- xmlsaver.cs
- DragDrop.cs
- HttpApplicationFactory.cs
- FileEnumerator.cs
- BinaryWriter.cs
- WindowPattern.cs
- FontFamilyConverter.cs
- LogExtent.cs
- ReferenceSchema.cs
- TreeViewImageGenerator.cs
- GetCardDetailsRequest.cs
- XmlNodeList.cs
- securitycriticaldataClass.cs
- BuildProviderAppliesToAttribute.cs
- URLMembershipCondition.cs
- MarginCollapsingState.cs
- InstanceContextManager.cs
- SizeConverter.cs
- ClientData.cs
- EntityWrapper.cs
- EndpointInfo.cs
- SafeNativeMethodsOther.cs
- CodeIdentifier.cs
- GridSplitterAutomationPeer.cs
- RequiredAttributeAttribute.cs
- ButtonChrome.cs
- IDReferencePropertyAttribute.cs
- LinqToSqlWrapper.cs
- HandlerFactoryWrapper.cs
- SqlUtil.cs
- DefaultDialogButtons.cs
- XmlSignificantWhitespace.cs
- ZipIOLocalFileHeader.cs
- TemplatePropertyEntry.cs
- RuleRef.cs
- SqlCacheDependencyDatabaseCollection.cs
- RemotingSurrogateSelector.cs
- GridViewSelectEventArgs.cs
- CodeCatchClauseCollection.cs
- PointAnimationClockResource.cs
- PresentationSource.cs
- RelativeSource.cs
- ConfigurationValue.cs
- SmtpReplyReaderFactory.cs
- TabItemWrapperAutomationPeer.cs
- LinkButton.cs
- BehaviorService.cs
- DelegateHelpers.Generated.cs
- AssemblyResourceLoader.cs
- StorageEntityTypeMapping.cs
- WSSecurityOneDotZeroReceiveSecurityHeader.cs
- AuthenticationServiceManager.cs
- MemoryPressure.cs
- BatchServiceHost.cs
- XamlClipboardData.cs
- ConfigurationConverterBase.cs
- HMACMD5.cs
- RotateTransform.cs
- SafeNativeMethods.cs
- XhtmlBasicPageAdapter.cs
- XmlDomTextWriter.cs
- TabletDevice.cs
- TimeoutValidationAttribute.cs
- VirtualPathProvider.cs
- SearchForVirtualItemEventArgs.cs
- Control.cs
- CodeDirectoryCompiler.cs
- UnionCqlBlock.cs
- PerformanceCountersElement.cs
- DataColumnMappingCollection.cs
- BrowserInteropHelper.cs
- ChildTable.cs
- FormViewPageEventArgs.cs
- PrivilegedConfigurationManager.cs
- SafeHandles.cs
- PropertyChangedEventManager.cs
- DataGridViewImageCell.cs