Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / CriticalFileToken.cs / 1 / CriticalFileToken.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //// The CriticalFileToken class ensures file represented is the one the // user has authorized us to manipulate. // // // History: // 08/28/2005: [....]: Initial implementation. //----------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using MS.Internal.PresentationUI; namespace MS.Internal.Documents.Application { ////// The CriticalFileToken class ensures file represented is the one the /// user has authorized us to manipulate. /// ////// Responsibility: /// Allow XpsViewer to safely pass around information on which file the user /// has authorized us to manipulate on thier behalf. Ensure that the creator /// of the object has the privledge to manipulate the file represented. /// /// Design Comments: /// Many classes need to perform privledged operations files on behalf of the /// user. However only DocObjHost and FilePresentation can assert it is user /// sourced data. /// /// As such we need them to create this 'token' which will will use as the only /// source of authoritative information for which files we are manipulating. /// /// As any SecurityCritical code can create SecurityCriticalData, we add the /// demand for FileIOPermission that represents the rights that will be asserted /// for in conjuction with this data. /// [FriendAccessAllowed] internal sealed class CriticalFileToken { #region Constructors //------------------------------------------------------------------------- // Constructors //------------------------------------------------------------------------- ////// Critical: /// - as this sets the _location which is Critical. /// TreatAsSafe: /// - the caller already has the value /// - the caller must satisfy the demand which this token grants /// [SecurityCritical, SecurityTreatAsSafe] internal CriticalFileToken(Uri location) { string path = location.LocalPath; new FileIOPermission( FileIOPermissionAccess.Read | FileIOPermissionAccess.Write, path) .Demand(); _location = location; } #endregion Constructors #region Object Members //-------------------------------------------------------------------------- // Object Members //------------------------------------------------------------------------- ////// Compares the values. /// ///True if they are equal. ////// Critical: /// - accesses _location /// TreatAsSafe: /// - does not leak information, simple compares to values the caller /// already had /// [SecurityCritical, SecurityTreatAsSafe] public static bool operator ==(CriticalFileToken a, CriticalFileToken b) { bool result = false; if (((object)a) == null) { if (((object)b) == null) { result = true; } } else { if (((object)b) != null) { result = a._location.ToString().Equals( b._location.ToString(), StringComparison.OrdinalIgnoreCase); } } return result; } ////// Compares the values. /// public static bool operator !=(CriticalFileToken a, CriticalFileToken b) { return !(a==b); } ////// Compares the values. /// public override bool Equals(object obj) { return (this == (obj as CriticalFileToken)); } ////// See Object.GetHashCode(); /// public override int GetHashCode() { return base.GetHashCode(); } #endregion Object Members #region Internal Properties //-------------------------------------------------------------------------- // Internal Properties //-------------------------------------------------------------------------- ////// The location for which the creator satisfied ReadWrite access. /// ////// Critical: /// - the location is sensitive data; it could leak information /// about the system; file structure, OS and user. /// internal Uri Location { [SecurityCritical] get { return _location; } } #endregion Internal Properties #region Private Fields //------------------------------------------------------------------------- // Private Fields //-------------------------------------------------------------------------- ////// Critical - by definition as this is a wrapper for Critical data. /// [SecurityCritical] private Uri _location; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
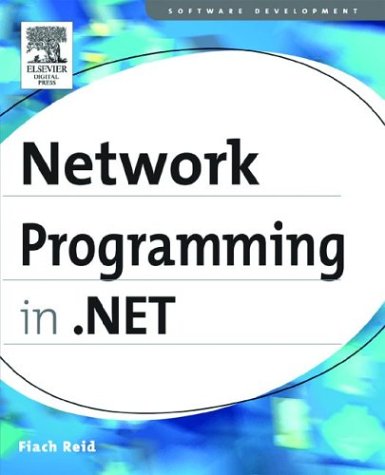
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Rule.cs
- XmlSerializationGeneratedCode.cs
- ConsoleCancelEventArgs.cs
- NotFiniteNumberException.cs
- PrePostDescendentsWalker.cs
- SqlTransaction.cs
- RtfControlWordInfo.cs
- TreeNodeMouseHoverEvent.cs
- CqlQuery.cs
- ItemsPresenter.cs
- ImportContext.cs
- ResourceSet.cs
- ConfigUtil.cs
- TargetException.cs
- ResourceAssociationType.cs
- TextEditorSpelling.cs
- TreeNodeConverter.cs
- DesignerDataSourceView.cs
- DataFieldEditor.cs
- TextWriterEngine.cs
- NativeMethods.cs
- JavaScriptObjectDeserializer.cs
- StrongNameIdentityPermission.cs
- Property.cs
- SecurityPolicySection.cs
- DocumentSchemaValidator.cs
- SecurityProtocol.cs
- FlowDecisionLabelFeature.cs
- FileDialog.cs
- FileUpload.cs
- ImportCatalogPart.cs
- LinkArea.cs
- CustomCredentialPolicy.cs
- ValueTypeFixupInfo.cs
- KeyedHashAlgorithm.cs
- login.cs
- SessionSymmetricMessageSecurityProtocolFactory.cs
- FontClient.cs
- SqlConnectionStringBuilder.cs
- Visual3D.cs
- HtmlFormParameterReader.cs
- Error.cs
- KeyProperty.cs
- TaskFormBase.cs
- RemoteWebConfigurationHost.cs
- ContentOnlyMessage.cs
- CachedPathData.cs
- SkipQueryOptionExpression.cs
- BamlCollectionHolder.cs
- EventSourceCreationData.cs
- DependencyPropertyKey.cs
- PassportAuthenticationModule.cs
- ExtenderControl.cs
- PropertyManager.cs
- ComAwareEventInfo.cs
- SolidColorBrush.cs
- ControllableStoryboardAction.cs
- UnmanagedMemoryStream.cs
- METAHEADER.cs
- WorkflowValidationFailedException.cs
- SoapInteropTypes.cs
- CommandEventArgs.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- ReadOnlyDictionary.cs
- SimpleApplicationHost.cs
- MailBnfHelper.cs
- SamlAuthorityBinding.cs
- BrushProxy.cs
- TextBox.cs
- TextViewSelectionProcessor.cs
- CuspData.cs
- XmlName.cs
- TransformCryptoHandle.cs
- Char.cs
- SafeProcessHandle.cs
- FieldBuilder.cs
- DataControlButton.cs
- ToolTipService.cs
- SslStreamSecurityBindingElement.cs
- FilterQuery.cs
- CancelEventArgs.cs
- OrderedDictionary.cs
- SubMenuStyle.cs
- OracleParameter.cs
- RangeBase.cs
- UIAgentMonitor.cs
- ObjectPersistData.cs
- GcHandle.cs
- ReadWriteSpinLock.cs
- sqlpipe.cs
- SHA1.cs
- GridItem.cs
- FileDetails.cs
- PopupRoot.cs
- PeerNameRecordCollection.cs
- TextDataBindingHandler.cs
- UniqueIdentifierService.cs
- TrackingWorkflowEventArgs.cs
- RunClient.cs
- HttpModuleAction.cs