Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / ReadWriteControlDesigner.cs / 1 / ReadWriteControlDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using Microsoft.Win32; using System; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Globalization; using System.Web.UI.WebControls; ////// /// [System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] [Obsolete("The recommended alternative is ContainerControlDesigner because it uses an EditableDesignerRegion for editing the content. Designer regions allow for better control of the content being edited. http://go.microsoft.com/fwlink/?linkid=14202")] public class ReadWriteControlDesigner : ControlDesigner { ///Provides a base designer class for all read-write server controls. ////// /// public ReadWriteControlDesigner() { ReadOnlyInternal = false; } ////// Initializes an instance of the ///class. /// /// public override string GetDesignTimeHtml() { return CreatePlaceHolderDesignTimeHtml(); } /// /// /// public override void OnComponentChanged(object sender, ComponentChangedEventArgs ce) { // Delegate to the base class implementation first! base.OnComponentChanged(sender, ce); if (IsIgnoringComponentChanges) { return; } if (!IsWebControl || (DesignTimeElementInternal == null)) { return; } MemberDescriptor member = ce.Member; object newValue = ce.NewValue; // HACK: you guys need to figure out a less hacky way then looking // for internal types... Type t = Type.GetType("System.ComponentModel.ReflectPropertyDescriptor, " + AssemblyRef.System); if (member != null && member.GetType() == t) { PropertyDescriptor propDesc = (PropertyDescriptor)member; if (member.Name.Equals("Font")) { WebControl control = (WebControl)Component; newValue = control.Font.Name; MapPropertyToStyle("Font.Name", newValue); newValue = control.Font.Size; MapPropertyToStyle("Font.Size", newValue); newValue = control.Font.Bold; MapPropertyToStyle("Font.Bold", newValue); newValue = control.Font.Italic; MapPropertyToStyle("Font.Italic", newValue); newValue = control.Font.Underline; MapPropertyToStyle("Font.Underline", newValue); newValue = control.Font.Strikeout; MapPropertyToStyle("Font.Strikeout", newValue); newValue = control.Font.Overline; MapPropertyToStyle("Font.Overline", newValue); } else if (newValue != null) { if (propDesc.PropertyType == typeof(Color)) { newValue = System.Drawing.ColorTranslator.ToHtml((System.Drawing.Color)newValue); } MapPropertyToStyle(propDesc.Name, newValue); } } } ////// Delegate to handle component changed event. /// ///protected virtual void MapPropertyToStyle(string propName, object varPropValue) { if (BehaviorInternal == null) return; Debug.Assert(propName != null && propName.Length != 0, "Invalid property name passed in!"); Debug.Assert(varPropValue != null, "Invalid property value passed in!"); if (propName == null || varPropValue == null) { return; } try { if (propName.Equals("BackColor")) { BehaviorInternal.SetStyleAttribute("backgroundColor", true /* designTimeOnly */, varPropValue, true /* ignoreCase */); } else if (propName.Equals("ForeColor")) { BehaviorInternal.SetStyleAttribute("color", true, varPropValue, true); } else if (propName.Equals("BorderWidth")) { string strPropValue = Convert.ToString(varPropValue, CultureInfo.InvariantCulture); BehaviorInternal.SetStyleAttribute("borderWidth", true, strPropValue, true); } else if (propName.Equals("BorderStyle")) { string strPropValue; if ((BorderStyle)varPropValue == BorderStyle.NotSet) { strPropValue = String.Empty; } else { strPropValue = Enum.Format(typeof(BorderStyle), (BorderStyle)varPropValue, "G"); } BehaviorInternal.SetStyleAttribute("borderStyle", true, strPropValue, true); } else if (propName.Equals("BorderColor")) { BehaviorInternal.SetStyleAttribute("borderColor", true, Convert.ToString(varPropValue, CultureInfo.InvariantCulture), true); } else if (propName.Equals("Height")) { BehaviorInternal.SetStyleAttribute("height", true, Convert.ToString(varPropValue, CultureInfo.InvariantCulture), true); } else if (propName.Equals("Width")) { BehaviorInternal.SetStyleAttribute("width", true, Convert.ToString(varPropValue, CultureInfo.InvariantCulture), true); } else if (propName.Equals("Font.Name")) { BehaviorInternal.SetStyleAttribute("fontFamily", true, Convert.ToString(varPropValue, CultureInfo.InvariantCulture), true); } else if (propName.Equals("Font.Size")) { BehaviorInternal.SetStyleAttribute("fontSize", true, Convert.ToString(varPropValue, CultureInfo.InvariantCulture), true); } else if (propName.Equals("Font.Bold")) { string styleValue; if ((bool)varPropValue) { styleValue = "bold"; } else { styleValue = "normal"; } BehaviorInternal.SetStyleAttribute("fontWeight", true, styleValue, true); } else if (propName.Equals("Font.Italic")) { string styleValue; if ((bool)varPropValue) { styleValue = "italic"; } else { styleValue = "normal"; } BehaviorInternal.SetStyleAttribute("fontStyle", true, styleValue, true); } else if (propName.Equals("Font.Underline")) { string styleValue = (string)BehaviorInternal.GetStyleAttribute("textDecoration", true, true); if ((bool)varPropValue) { if (styleValue == null) { styleValue = "underline"; } else if (styleValue.ToLower(CultureInfo.InvariantCulture).IndexOf("underline", StringComparison.Ordinal) < 0) { styleValue += " underline"; } BehaviorInternal.SetStyleAttribute("textDecoration", true, styleValue, true); } else if (styleValue != null) { int index = styleValue.ToLower(CultureInfo.InvariantCulture).IndexOf("underline", StringComparison.Ordinal); if (index >= 0) { string newStyleValue = styleValue.Substring(0, index); if (index + 9 < styleValue.Length) { newStyleValue = " " + styleValue.Substring(index + 9); } BehaviorInternal.SetStyleAttribute("textDecoration", true, newStyleValue, true); } } } else if (propName.Equals("Font.Strikeout")) { string styleValue = (string)BehaviorInternal.GetStyleAttribute("textDecoration", true, true); if ((bool)varPropValue) { if (styleValue == null) { styleValue = "line-through"; } else if (styleValue.ToLower(CultureInfo.InvariantCulture).IndexOf("line-through", StringComparison.Ordinal) < 0) { styleValue += " line-through"; } BehaviorInternal.SetStyleAttribute("textDecoration", true, styleValue, true); } else if (styleValue != null) { int index = styleValue.ToLower(CultureInfo.InvariantCulture).IndexOf("line-through", StringComparison.Ordinal); if (index >= 0) { string newStyleValue = styleValue.Substring(0, index); if (index + 12 < styleValue.Length) { newStyleValue = " " + styleValue.Substring(index + 12); } BehaviorInternal.SetStyleAttribute("textDecoration", true, newStyleValue, true); } } } else if (propName.Equals("Font.Overline")) { string styleValue = (string)BehaviorInternal.GetStyleAttribute("textDecoration", true, true); if ((bool)varPropValue) { if (styleValue == null) { styleValue = "overline"; } else if (styleValue.ToLower(CultureInfo.InvariantCulture).IndexOf("overline", StringComparison.Ordinal) < 0) { styleValue += " overline"; } BehaviorInternal.SetStyleAttribute("textDecoration", true, styleValue, true); } else if (styleValue != null) { int index = styleValue.ToLower(CultureInfo.InvariantCulture).IndexOf("overline", StringComparison.Ordinal); if (index >= 0) { string newStyleValue = styleValue.Substring(0, index); if (index + 8 < styleValue.Length) { newStyleValue = " " + styleValue.Substring(index + 8); } BehaviorInternal.SetStyleAttribute("textDecoration", true, newStyleValue, true); } } } } catch (Exception ex) { Debug.Fail(ex.ToString()); } } /// /// /// Notification that is fired upon the designer being attached to the behavior. /// [Obsolete("The recommended alternative is ControlDesigner.Tag. http://go.microsoft.com/fwlink/?linkid=14202")] protected override void OnBehaviorAttached() { base.OnBehaviorAttached(); if (!IsWebControl) { return; } WebControl control = (WebControl)Component; string colorValue; colorValue = System.Drawing.ColorTranslator.ToHtml((System.Drawing.Color)control.BackColor); if (colorValue.Length > 0) { MapPropertyToStyle("BackColor", colorValue); } colorValue = System.Drawing.ColorTranslator.ToHtml((System.Drawing.Color)control.ForeColor); if (colorValue.Length > 0) { MapPropertyToStyle("ForeColor", colorValue); } colorValue = System.Drawing.ColorTranslator.ToHtml((System.Drawing.Color)control.BorderColor); if (colorValue.Length > 0) { MapPropertyToStyle("BorderColor", colorValue); } BorderStyle borderStyle = control.BorderStyle; if (borderStyle != BorderStyle.NotSet) { MapPropertyToStyle("BorderStyle", borderStyle); } Unit borderWidth = control.BorderWidth; if (borderWidth.IsEmpty == false && borderWidth.Value != 0) { MapPropertyToStyle("BorderWidth", borderWidth.ToString(CultureInfo.InvariantCulture)); } Unit width = control.Width; if (!width.IsEmpty && width.Value != 0) { MapPropertyToStyle("Width", width.ToString(CultureInfo.InvariantCulture)); } Unit height = control.Height; if (!height.IsEmpty && height.Value != 0) { MapPropertyToStyle("Height", height.ToString(CultureInfo.InvariantCulture)); } string fontName = control.Font.Name; if (fontName.Length != 0) { MapPropertyToStyle("Font.Name", fontName); } FontUnit fontSize = control.Font.Size; if (fontSize != FontUnit.Empty) { MapPropertyToStyle("Font.Size", fontSize.ToString(CultureInfo.InvariantCulture)); } bool boolValue = control.Font.Bold; if (boolValue) { MapPropertyToStyle("Font.Bold", boolValue); } boolValue = control.Font.Italic; if (boolValue) { MapPropertyToStyle("Font.Italic", boolValue); } boolValue = control.Font.Underline; if (boolValue) { MapPropertyToStyle("Font.Underline", boolValue); } boolValue = control.Font.Strikeout; if (boolValue) { MapPropertyToStyle("Font.Strikeout", boolValue); } boolValue = control.Font.Overline; if (boolValue) { MapPropertyToStyle("Font.Overline", boolValue); } } ////// public override void UpdateDesignTimeHtml() { // do nothing } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
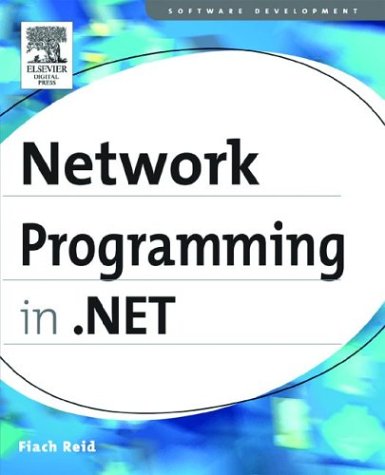
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BidPrivateBase.cs
- CommandField.cs
- ArrayElementGridEntry.cs
- ProcessModule.cs
- DirectoryInfo.cs
- DynamicExpression.cs
- ParseChildrenAsPropertiesAttribute.cs
- XPathArrayIterator.cs
- FontTypeConverter.cs
- ServiceOperation.cs
- oledbconnectionstring.cs
- BooleanStorage.cs
- TreeViewAutomationPeer.cs
- SizeLimitedCache.cs
- ValidationHelper.cs
- TextReader.cs
- BulletedListEventArgs.cs
- SourceFileBuildProvider.cs
- ListViewItem.cs
- NonParentingControl.cs
- InputScopeConverter.cs
- XmlNodeWriter.cs
- TransformerInfoCollection.cs
- CodeGenerator.cs
- ModelVisual3D.cs
- OrthographicCamera.cs
- SqlSelectStatement.cs
- KeyPullup.cs
- EntityDataSourceState.cs
- UrlMappingsModule.cs
- AssemblyNameEqualityComparer.cs
- Rectangle.cs
- DesignColumn.cs
- OracleCommandSet.cs
- SelectionBorderGlyph.cs
- ContextProperty.cs
- MappingException.cs
- SqlServer2KCompatibilityAnnotation.cs
- Rect3D.cs
- WmlFormAdapter.cs
- DropAnimation.xaml.cs
- SequentialOutput.cs
- FontSourceCollection.cs
- FontStretch.cs
- QueryTask.cs
- Identifier.cs
- TreeNodeEventArgs.cs
- SQLMembershipProvider.cs
- TextServicesHost.cs
- FilteredAttributeCollection.cs
- ProfileService.cs
- WebBrowsableAttribute.cs
- BamlRecordHelper.cs
- XhtmlBasicImageAdapter.cs
- SecurityContextTokenCache.cs
- PropertyBuilder.cs
- PrimitiveType.cs
- ValueProviderWrapper.cs
- Cloud.cs
- LocalizableAttribute.cs
- DynamicScriptObject.cs
- UpdatePanelTriggerCollection.cs
- BuiltInExpr.cs
- ChangePassword.cs
- DebugInfoGenerator.cs
- MappingSource.cs
- PreparingEnlistment.cs
- Argument.cs
- HttpListenerContext.cs
- TextEditorTyping.cs
- AutomationElementCollection.cs
- ClusterRegistryConfigurationProvider.cs
- CultureInfoConverter.cs
- ClientRuntimeConfig.cs
- LinkedResource.cs
- AppDomainFactory.cs
- UDPClient.cs
- EdmToObjectNamespaceMap.cs
- BackEase.cs
- CollectionsUtil.cs
- WorkerRequest.cs
- GenericQueueSurrogate.cs
- DBSqlParserColumn.cs
- DesignerTransaction.cs
- BrowserDefinitionCollection.cs
- DateTimeStorage.cs
- AsymmetricKeyExchangeFormatter.cs
- ListCollectionView.cs
- SafeFileMappingHandle.cs
- MaterialCollection.cs
- PerspectiveCamera.cs
- DropShadowEffect.cs
- PropertyGridEditorPart.cs
- SqlUtil.cs
- SchemaDeclBase.cs
- SqlUtil.cs
- ContextActivityUtils.cs
- ExpressionBinding.cs
- OperatorExpressions.cs
- UIElementPropertyUndoUnit.cs