Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / FontTypeConverter.cs / 2 / FontTypeConverter.cs
/*++ Copyright (C) 2004 - 2005 Microsoft Corporation All rights reserved. Module Name: FontTypeConverter.cs Abstract: This file implements the FontTypeConverter used by the Xps Serialization APIs for serializing fonts to a Xps package. Author: [....] ([....]) 1-December-2004 Revision History: --*/ using System; using System.Collections.Generic; using System.ComponentModel; using System.IO; using System.Windows.Media; using System.Windows.Xps.Packaging; namespace System.Windows.Xps.Serialization { ////// This class implements a type converter for converting /// fonts to Uris. It handles the writing of the font /// to a Xps package and returns a package URI to the /// caller. It also handles reading a font from a /// Xps package given a Uri. /// public class FontTypeConverter : ExpandableObjectConverter { #region Public overrides for ExpandableObjectConverted ////// Returns whether this converter can convert an object /// of the given type to the type of this converter. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// A Type that represents the type you want to convert from. /// ////// true if this converter can perform the conversion; /// otherwise, false. /// public override bool CanConvertFrom( ITypeDescriptorContext context, Type sourceType ) { return IsSupportedType(sourceType); } ////// Returns whether this converter can convert the object /// to the specified type. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// A Type that represents the type you want to convert to. /// ////// true if this converter can perform the conversion; /// otherwise, false. /// public override bool CanConvertTo( ITypeDescriptorContext context, Type destinationType ) { return IsSupportedType(destinationType); } ////// Converts the given value to the type of this converter. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// The CultureInfo to use as the current culture. /// /// /// The Object to convert. /// ////// An Object that represents the converted value. /// public override object ConvertFrom( ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value ) { if( value == null ) { throw new ArgumentNullException("value"); } if (!IsSupportedType(value.GetType())) { throw new NotSupportedException(ReachSR.Get(ReachSRID.Converter_ConvertFromNotSupported)); } throw new NotImplementedException(); } ////// Converts the given value object to the specified type, /// using the arguments. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// A CultureInfo object. If null is passed, the current /// culture is assumed. /// /// /// The Object to convert. /// /// /// The Type to convert the value parameter to. /// ////// The Type to convert the value parameter to. /// public override object ConvertTo( ITypeDescriptorContext context, System.Globalization.CultureInfo culture, object value, Type destinationType ) { if( context == null ) { throw new ArgumentNullException("context"); } Toolbox.StartEvent(MS.Utility.EventTraceGuidId.DRXCONVERTFONTGUID); if (!IsSupportedType(destinationType)) { throw new NotSupportedException(ReachSR.Get(ReachSRID.Converter_ConvertToNotSupported)); } PackageSerializationManager manager = (PackageSerializationManager)context.GetService(typeof(XpsSerializationManager)); // // Ensure that we have a valid GlyphRun instance // GlyphRun fontGlyphRun = (GlyphRun)value; if (fontGlyphRun == null) { throw new ArgumentException(ReachSR.Get(ReachSRID.MustBeOfType, "value", "GlyphRun")); } // // Obtain the font serialization service from the serlialization manager. // IServiceProvider resourceServiceProvider = manager.ResourcePolicy; XpsFontSerializationService fontService = (XpsFontSerializationService)resourceServiceProvider.GetService(typeof(XpsFontSerializationService)); if (fontService == null) { throw new XpsSerializationException(ReachSR.Get(ReachSRID.ReachSerialization_NoFontService)); } // // Retrieve the current font subsetter // XpsFontSubsetter fontSubsetter = fontService.FontSubsetter; // // Add the font subset to the font subsetter and retrieve a Uri // to the font within the Xps package. // Uri resourceUri = fontSubsetter.ComputeFontSubset(fontGlyphRun); Toolbox.EndEvent(MS.Utility.EventTraceGuidId.DRXCONVERTFONTGUID); return resourceUri; } ////// Gets a collection of properties for the type of object /// specified by the value parameter. /// /// /// An ITypeDescriptorContext that provides a format context. /// /// /// An Object that specifies the type of object to get the /// properties for. /// /// /// An array of type Attribute that will be used as a filter. /// ////// A PropertyDescriptorCollection with the properties that are /// exposed for the component, or null if there are no properties. /// public override PropertyDescriptorCollection GetProperties( ITypeDescriptorContext context, object value, Attribute[] attributes ) { throw new NotImplementedException(); } #endregion Public overrides for ExpandableObjectConverted #region Private static helper methods ////// Looks up the type in a table to determine /// whether this type is supported by this /// class. /// /// /// Type to lookup in table. /// ////// True is supported; otherwise false. /// private static bool IsSupportedType( Type type ) { bool bSupported = false; foreach (Type t in SupportedTargetTypes) { if (t.Equals(type)) { bSupported = true; break; } } return bSupported; } #endregion Private static helper methods #region Private static data ////// A table of supported types for this type converter /// private static Type[] SupportedTargetTypes = { typeof(Uri) }; #endregion Private static data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
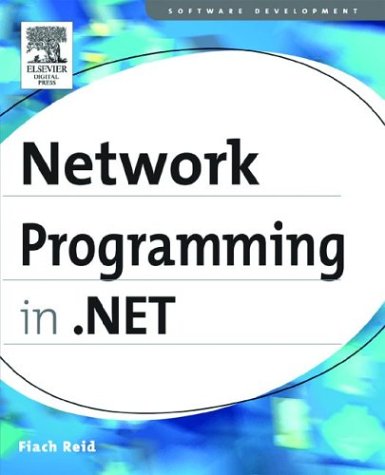
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontNamesConverter.cs
- ImageAttributes.cs
- VBCodeProvider.cs
- StylusShape.cs
- SaveWorkflowCommand.cs
- TextEditorTyping.cs
- VerificationException.cs
- HtmlInputCheckBox.cs
- XmlSchemaAttributeGroupRef.cs
- DirectoryObjectSecurity.cs
- JpegBitmapDecoder.cs
- FirewallWrapper.cs
- MaskInputRejectedEventArgs.cs
- DropShadowEffect.cs
- XmlElementAttributes.cs
- TypeDependencyAttribute.cs
- FormsAuthenticationConfiguration.cs
- WindowsScrollBarBits.cs
- RefType.cs
- GridViewDesigner.cs
- DataViewSetting.cs
- InvalidOperationException.cs
- odbcmetadatafactory.cs
- ListViewInsertEventArgs.cs
- NetNamedPipeSecurityMode.cs
- DefaultBinder.cs
- ImageList.cs
- CellParaClient.cs
- BitFlagsGenerator.cs
- RemoteX509Token.cs
- StructuredType.cs
- FontSizeConverter.cs
- HttpCapabilitiesBase.cs
- QueueProcessor.cs
- GridViewUpdatedEventArgs.cs
- RestHandler.cs
- LicenseContext.cs
- ExecutionContext.cs
- ModifierKeysValueSerializer.cs
- TypePresenter.xaml.cs
- ControlAdapter.cs
- EndpointAddress10.cs
- ResXResourceWriter.cs
- HotSpot.cs
- FramingFormat.cs
- SponsorHelper.cs
- DefaultBindingPropertyAttribute.cs
- URLIdentityPermission.cs
- FunctionQuery.cs
- PageCatalogPart.cs
- EntityDataSourceSelectedEventArgs.cs
- XmlSchemaAppInfo.cs
- IndexExpression.cs
- BitmapImage.cs
- TableProviderWrapper.cs
- ItemCheckedEvent.cs
- ListViewGroupConverter.cs
- ConfigurationErrorsException.cs
- ScriptingProfileServiceSection.cs
- ByteFacetDescriptionElement.cs
- FontCollection.cs
- MulticastNotSupportedException.cs
- CommandID.cs
- BinaryParser.cs
- NamespaceDisplay.xaml.cs
- SimpleExpression.cs
- SafeNativeMethods.cs
- InfoCardArgumentException.cs
- SeekStoryboard.cs
- MailMessage.cs
- PathFigure.cs
- FileRegion.cs
- AmbientLight.cs
- ReferenceSchema.cs
- ToolStripMenuItem.cs
- WindowsContainer.cs
- WebSysDefaultValueAttribute.cs
- TextPattern.cs
- XNodeValidator.cs
- BitmapEffectGroup.cs
- CodeDirectoryCompiler.cs
- SchemaEntity.cs
- DataSourceGeneratorException.cs
- HostedImpersonationContext.cs
- UnsafeCollabNativeMethods.cs
- DataObjectCopyingEventArgs.cs
- TimersDescriptionAttribute.cs
- ReversePositionQuery.cs
- DataSet.cs
- Formatter.cs
- Aggregates.cs
- OutputCacheSection.cs
- ByteAnimationUsingKeyFrames.cs
- FrameworkPropertyMetadata.cs
- Int32CAMarshaler.cs
- StylusPoint.cs
- ConfigDefinitionUpdates.cs
- ConnectionInterfaceCollection.cs
- SoapHeaders.cs
- ToolStripButton.cs