Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Serialization / System / Runtime / Serialization / BitFlagsGenerator.cs / 1305376 / BitFlagsGenerator.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Runtime.Serialization { using System; using System.Reflection; using System.Reflection.Emit; using System.Security; [Fx.Tag.SecurityNote(Miscellaneous = "RequiresReview (Critical) - works on CodeGenerator objects, which require Critical access.")] class BitFlagsGenerator { int bitCount; CodeGenerator ilg; LocalBuilder[] locals; public BitFlagsGenerator(int bitCount, CodeGenerator ilg, string localName) { this.ilg = ilg; this.bitCount = bitCount; int localCount = (bitCount + 7) / 8; locals = new LocalBuilder[localCount]; for (int i = 0; i < locals.Length; i++) { locals[i] = ilg.DeclareLocal(typeof(byte), localName + i, (byte) 0); } } public static bool IsBitSet(byte[] bytes, int bitIndex) { int byteIndex = GetByteIndex(bitIndex); byte bitValue = GetBitValue(bitIndex); return (bytes[byteIndex] & bitValue) == bitValue; } public static void SetBit(byte[] bytes, int bitIndex) { int byteIndex = GetByteIndex(bitIndex); byte bitValue = GetBitValue(bitIndex); bytes[byteIndex] |= bitValue; } public int GetBitCount() { return bitCount; } public LocalBuilder GetLocal(int i) { return locals[i]; } public int GetLocalCount() { return locals.Length; } public void Load(int bitIndex) { LocalBuilder local = locals[GetByteIndex(bitIndex)]; byte bitValue = GetBitValue(bitIndex); ilg.Load(local); ilg.Load(bitValue); ilg.And(); ilg.Load(bitValue); ilg.Ceq(); } public void LoadArray() { LocalBuilder localArray = ilg.DeclareLocal(Globals.TypeOfByteArray, "localArray"); ilg.NewArray(typeof(byte), locals.Length); ilg.Store(localArray); for (int i = 0; i < locals.Length; i++) { ilg.StoreArrayElement(localArray, i, locals[i]); } ilg.Load(localArray); } public void Store(int bitIndex, bool value) { LocalBuilder local = locals[GetByteIndex(bitIndex)]; byte bitValue = GetBitValue(bitIndex); if (value) { ilg.Load(local); ilg.Load(bitValue); ilg.Or(); ilg.Stloc(local); } else { ilg.Load(local); ilg.Load(bitValue); ilg.Not(); ilg.And(); ilg.Stloc(local); } } static byte GetBitValue(int bitIndex) { return (byte)(1 << (bitIndex & 7)); } static int GetByteIndex(int bitIndex) { return bitIndex >> 3; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Runtime.Serialization { using System; using System.Reflection; using System.Reflection.Emit; using System.Security; [Fx.Tag.SecurityNote(Miscellaneous = "RequiresReview (Critical) - works on CodeGenerator objects, which require Critical access.")] class BitFlagsGenerator { int bitCount; CodeGenerator ilg; LocalBuilder[] locals; public BitFlagsGenerator(int bitCount, CodeGenerator ilg, string localName) { this.ilg = ilg; this.bitCount = bitCount; int localCount = (bitCount + 7) / 8; locals = new LocalBuilder[localCount]; for (int i = 0; i < locals.Length; i++) { locals[i] = ilg.DeclareLocal(typeof(byte), localName + i, (byte) 0); } } public static bool IsBitSet(byte[] bytes, int bitIndex) { int byteIndex = GetByteIndex(bitIndex); byte bitValue = GetBitValue(bitIndex); return (bytes[byteIndex] & bitValue) == bitValue; } public static void SetBit(byte[] bytes, int bitIndex) { int byteIndex = GetByteIndex(bitIndex); byte bitValue = GetBitValue(bitIndex); bytes[byteIndex] |= bitValue; } public int GetBitCount() { return bitCount; } public LocalBuilder GetLocal(int i) { return locals[i]; } public int GetLocalCount() { return locals.Length; } public void Load(int bitIndex) { LocalBuilder local = locals[GetByteIndex(bitIndex)]; byte bitValue = GetBitValue(bitIndex); ilg.Load(local); ilg.Load(bitValue); ilg.And(); ilg.Load(bitValue); ilg.Ceq(); } public void LoadArray() { LocalBuilder localArray = ilg.DeclareLocal(Globals.TypeOfByteArray, "localArray"); ilg.NewArray(typeof(byte), locals.Length); ilg.Store(localArray); for (int i = 0; i < locals.Length; i++) { ilg.StoreArrayElement(localArray, i, locals[i]); } ilg.Load(localArray); } public void Store(int bitIndex, bool value) { LocalBuilder local = locals[GetByteIndex(bitIndex)]; byte bitValue = GetBitValue(bitIndex); if (value) { ilg.Load(local); ilg.Load(bitValue); ilg.Or(); ilg.Stloc(local); } else { ilg.Load(local); ilg.Load(bitValue); ilg.Not(); ilg.And(); ilg.Stloc(local); } } static byte GetBitValue(int bitIndex) { return (byte)(1 << (bitIndex & 7)); } static int GetByteIndex(int bitIndex) { return bitIndex >> 3; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
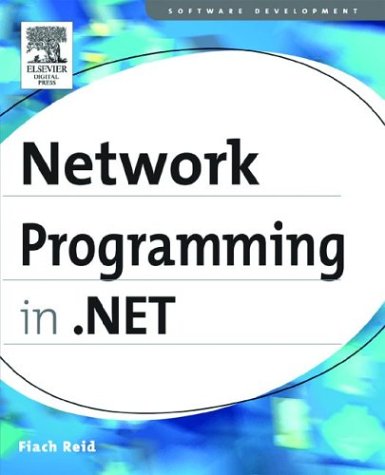
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- VariableQuery.cs
- InternalDuplexChannelListener.cs
- FontStretches.cs
- TextServicesContext.cs
- CacheChildrenQuery.cs
- ComplusEndpointConfigContainer.cs
- TraceContextRecord.cs
- QilValidationVisitor.cs
- WindowVisualStateTracker.cs
- ActivationArguments.cs
- CultureTable.cs
- Array.cs
- SettingsSavedEventArgs.cs
- QueryStringParameter.cs
- OleDbDataAdapter.cs
- DynamicActivityTypeDescriptor.cs
- ButtonBaseDesigner.cs
- NonVisualControlAttribute.cs
- PathSegmentCollection.cs
- SafeRegistryHandle.cs
- HotSpotCollection.cs
- Viewport3DAutomationPeer.cs
- AppLevelCompilationSectionCache.cs
- Executor.cs
- LinqDataSourceInsertEventArgs.cs
- FieldToken.cs
- ExtractorMetadata.cs
- SortableBindingList.cs
- NativeMethods.cs
- RecognizedWordUnit.cs
- ConfigXmlWhitespace.cs
- UInt32Converter.cs
- xmlglyphRunInfo.cs
- XmlSerializerOperationGenerator.cs
- SuppressMessageAttribute.cs
- Point3DValueSerializer.cs
- ValidatorUtils.cs
- _ConnectOverlappedAsyncResult.cs
- TextViewDesigner.cs
- XamlGridLengthSerializer.cs
- AssociationSet.cs
- PrintDialogException.cs
- DesignerVerbToolStripMenuItem.cs
- HttpHeaderCollection.cs
- ResourcePermissionBase.cs
- SQLMoneyStorage.cs
- ShutDownListener.cs
- DoubleAnimationUsingKeyFrames.cs
- BaseResourcesBuildProvider.cs
- CacheSection.cs
- PinnedBufferMemoryStream.cs
- AmbiguousMatchException.cs
- DecimalConverter.cs
- FullTextBreakpoint.cs
- NestedContainer.cs
- MemoryStream.cs
- OleStrCAMarshaler.cs
- SynchronizedDispatch.cs
- ServiceBuildProvider.cs
- BufferManager.cs
- PositiveTimeSpanValidator.cs
- TextPointerBase.cs
- CancellationScope.cs
- ToolboxItemCollection.cs
- SpanIndex.cs
- SQLRoleProvider.cs
- ListViewCancelEventArgs.cs
- ValidatorCollection.cs
- DbDataAdapter.cs
- RuleAction.cs
- CodeTypeConstructor.cs
- ValidatedControlConverter.cs
- ProfileSection.cs
- DrawingAttributes.cs
- BreakSafeBase.cs
- RemotingAttributes.cs
- ReadContentAsBinaryHelper.cs
- WebEventTraceProvider.cs
- GlyphElement.cs
- WebSysDisplayNameAttribute.cs
- QueryableDataSource.cs
- ItemCollection.cs
- Rotation3DAnimationUsingKeyFrames.cs
- PathGradientBrush.cs
- dataprotectionpermission.cs
- DataGridViewAdvancedBorderStyle.cs
- GridViewRowPresenter.cs
- ComIntegrationManifestGenerator.cs
- PrivateFontCollection.cs
- ContractAdapter.cs
- IOException.cs
- Processor.cs
- PositiveTimeSpanValidator.cs
- EntityWithChangeTrackerStrategy.cs
- InkCanvasInnerCanvas.cs
- XmlSchemaElement.cs
- RelationshipDetailsCollection.cs
- HintTextConverter.cs
- EntityTypeEmitter.cs
- LinkedResource.cs