Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ButtonBaseDesigner.cs / 1 / ButtonBaseDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.Design { using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Drawing; using System.Runtime.InteropServices; using System.Windows.Forms.Design.Behavior; using System.Windows.Forms; using System.Diagnostics; ////// /// internal class ButtonBaseDesigner : ControlDesigner { // private DesignerActionListCollection _actionlists; public ButtonBaseDesigner() { AutoResizeHandles = true; } public override void InitializeNewComponent(IDictionary defaultValues) { base.InitializeNewComponent(defaultValues); PropertyDescriptor prop = TypeDescriptor.GetProperties(Component)["UseVisualStyleBackColor"]; if (prop != null && prop.PropertyType == typeof(bool) && !prop.IsReadOnly && prop.IsBrowsable) { prop.SetValue(Component, true); } } ////// Provides a designer that can design components /// that extend ButtonBase. ////// /// Adds a baseline SnapLine to the list of SnapLines related /// to this control. /// public override IList SnapLines { get { ArrayList snapLines = base.SnapLines as ArrayList; FlatStyle flatStyle = FlatStyle.Standard; ContentAlignment alignment = ContentAlignment.MiddleCenter; PropertyDescriptor prop; PropertyDescriptorCollection props = TypeDescriptor.GetProperties(Component); if ((prop = props["TextAlign"]) != null) { alignment = (ContentAlignment)prop.GetValue(Component); } if ((prop = props["FlatStyle"]) != null) { flatStyle = (FlatStyle)prop.GetValue(Component); } int baseline = DesignerUtils.GetTextBaseline(Control, alignment); //based on the type of control and it's style, we need to add certain deltas to make //the snapline appear in the right place. Rather than adding a class for each control //we special case it here - for perf reasons. if ((Control is CheckBox) || (Control is RadioButton)) { Appearance appearance = Appearance.Normal; if ((prop = props["Appearance"]) != null) { appearance = (Appearance)prop.GetValue(Component); } if (appearance == Appearance.Normal) { if (Control is CheckBox) { baseline += CheckboxBaselineOffset(alignment, flatStyle); } else { baseline += RadiobuttonBaselineOffset(alignment, flatStyle); } } else { baseline += DefaultBaselineOffset(alignment, flatStyle); } } else { //default case baseline += DefaultBaselineOffset(alignment, flatStyle); } snapLines.Add(new SnapLine(SnapLineType.Baseline, baseline, SnapLinePriority.Medium)); return snapLines; } } private int CheckboxBaselineOffset(ContentAlignment alignment, FlatStyle flatStyle) { if ((alignment & DesignerUtils.anyMiddleAlignment) != 0) { if ((flatStyle == FlatStyle.Standard) || (flatStyle == FlatStyle.System)) { return -1; } else { return 0; //FlatStyle.Flat || FlatStyle.Popup || Unknown FlatStyle } } else if ((alignment & DesignerUtils.anyTopAlignment) != 0) { if (flatStyle == FlatStyle.Standard) { return 1; } else if (flatStyle == FlatStyle.System) { return 0; } else if ((flatStyle == FlatStyle.Flat) || (flatStyle == FlatStyle.Popup)) { return 2; } else { Debug.Fail("Unknown FlatStyle"); return 0; //Unknown FlatStyle } } else {//bottom alignment if (flatStyle == FlatStyle.Standard) { return -3; } else if (flatStyle == FlatStyle.System) { return 0; } else if ((flatStyle == FlatStyle.Flat) || (flatStyle == FlatStyle.Popup)) { return -2; } else { Debug.Fail("Unknown FlatStyle"); return 0; //Unknown FlatStyle } } } private int RadiobuttonBaselineOffset(ContentAlignment alignment, FlatStyle flatStyle) { if ((alignment & DesignerUtils.anyMiddleAlignment) != 0) { if (flatStyle == FlatStyle.System) { return -1; } else { return 0; //FlatStyle.Standard || FlatStyle.Flat || FlatStyle.Popup || Unknown FlatStyle } } else {// Top or bottom alignment if ((flatStyle == FlatStyle.Standard) || (flatStyle == FlatStyle.Flat) || (flatStyle == FlatStyle.Popup)) { return ((alignment & DesignerUtils.anyTopAlignment) != 0) ? 2 : -2; } else if (flatStyle == FlatStyle.System) { return 0; } else { Debug.Fail("Unknown FlatStyle"); return 0; //Unknown FlatStyle } } } private int DefaultBaselineOffset(ContentAlignment alignment, FlatStyle flatStyle) { if ((alignment & DesignerUtils.anyMiddleAlignment) != 0) { return 0; } else { // Top or bottom alignment if ((flatStyle == FlatStyle.Standard) || (flatStyle == FlatStyle.Popup)) { return ((alignment & DesignerUtils.anyTopAlignment) != 0) ? 4 : -4; } else if (flatStyle == FlatStyle.System) { return ((alignment & DesignerUtils.anyTopAlignment) != 0) ? 3 : -3; } else if (flatStyle == FlatStyle.Flat) { return ((alignment & DesignerUtils.anyTopAlignment) != 0) ? 5 : -5; } else { Debug.Fail("Unknown FlatStyle"); return 0; //Unknown FlatStyle } } } /* public override DesignerActionListCollection ActionLists { get { if(_actionlists == null) { _actionlists = new DesignerActionListCollection(); _actionlists.Add(new ButtonBaseActionList()); } return _actionlists; } } */ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
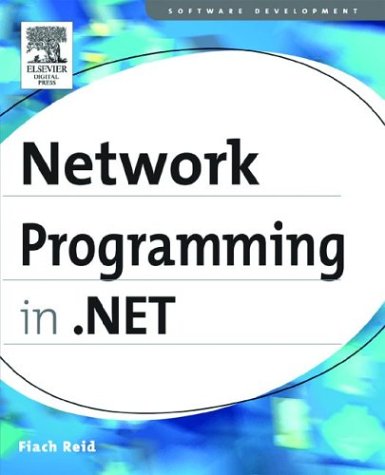
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UICuesEvent.cs
- GuidelineCollection.cs
- WindowsProgressbar.cs
- DynamicObjectAccessor.cs
- TabletCollection.cs
- NotifyCollectionChangedEventArgs.cs
- XmlSortKey.cs
- BindingCompleteEventArgs.cs
- SafeTimerHandle.cs
- PiiTraceSource.cs
- DataGridViewColumnStateChangedEventArgs.cs
- DataGridSortCommandEventArgs.cs
- DocumentApplication.cs
- Splitter.cs
- MergeEnumerator.cs
- XmlSchemaValidationException.cs
- AnnotationAuthorChangedEventArgs.cs
- MemberMemberBinding.cs
- HtmlTitle.cs
- SiteMapProvider.cs
- XMLSyntaxException.cs
- BamlLocalizabilityResolver.cs
- HttpDictionary.cs
- Authorization.cs
- WorkflowDebuggerSteppingAttribute.cs
- EndPoint.cs
- HttpChannelHelper.cs
- DispatcherProcessingDisabled.cs
- ModulesEntry.cs
- SplashScreen.cs
- EntitySetDataBindingList.cs
- ProtocolsConfigurationHandler.cs
- EncryptedData.cs
- CommonDialog.cs
- SmiRecordBuffer.cs
- MsmqIntegrationValidationBehavior.cs
- IPEndPointCollection.cs
- ButtonBaseAutomationPeer.cs
- Schedule.cs
- DataGridViewColumnCollection.cs
- ManipulationStartingEventArgs.cs
- Message.cs
- ResourceDisplayNameAttribute.cs
- EntityClassGenerator.cs
- RoleManagerEventArgs.cs
- ErrorStyle.cs
- BasicKeyConstraint.cs
- LockCookie.cs
- MonikerHelper.cs
- CompareValidator.cs
- XamlVector3DCollectionSerializer.cs
- MultipleViewPattern.cs
- RewritingPass.cs
- AttributeCollection.cs
- DES.cs
- Trustee.cs
- _SslSessionsCache.cs
- ForAllOperator.cs
- TextUtf8RawTextWriter.cs
- MembershipUser.cs
- WebPartConnection.cs
- Oid.cs
- Number.cs
- Publisher.cs
- SqlClientMetaDataCollectionNames.cs
- AccessedThroughPropertyAttribute.cs
- SqlCommand.cs
- DecoderFallback.cs
- SourceChangedEventArgs.cs
- Stackframe.cs
- PerformanceCountersElement.cs
- ThreadPool.cs
- UpnEndpointIdentity.cs
- DebugView.cs
- ServiceOperationParameter.cs
- SynchronizingStream.cs
- Subordinate.cs
- EntityDesignerDataSourceView.cs
- StatusStrip.cs
- ElementFactory.cs
- Viewport2DVisual3D.cs
- CategoriesDocumentFormatter.cs
- ExceptionHelpers.cs
- RecordsAffectedEventArgs.cs
- EventMappingSettings.cs
- Update.cs
- ActivityExecutorOperation.cs
- XmlQueryStaticData.cs
- AlphabeticalEnumConverter.cs
- PointAnimationBase.cs
- GlyphManager.cs
- AttributeCollection.cs
- ReadOnlyPropertyMetadata.cs
- EditBehavior.cs
- AbsoluteQuery.cs
- FrugalMap.cs
- RenderDataDrawingContext.cs
- ToolboxBitmapAttribute.cs
- TimerEventSubscription.cs
- CommandPlan.cs