Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / UI / AttributeCollection.cs / 1305376 / AttributeCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * AttributeCollection.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI { using System.IO; using System.Collections; using System.Reflection; using System.Web.UI; using System.Globalization; using System.Security.Permissions; using System.Web.Util; /* * The AttributeCollection represents Attributes on an Html control. */ ////// public sealed class AttributeCollection { private StateBag _bag; private CssStyleCollection _styleColl; /* * Constructs an AttributeCollection given a StateBag. */ ////// The ///class provides object-model access /// to all attributes declared on an HTML server control element. /// /// public AttributeCollection(StateBag bag) { _bag = bag; } /* * Automatically adds new keys. */ ////// public string this[string key] { get { if (_styleColl != null && StringUtil.EqualsIgnoreCase(key, "style")) return _styleColl.Value; else return _bag[key] as string; } set { Add(key, value); } } /* * Returns a collection of keys. */ ////// Gets or sets a specified attribute value. /// ////// public ICollection Keys { get { return _bag.Keys; } } ////// Gets a collection of keys to all the attributes in the /// ///. /// /// public int Count { get { return _bag.Count; } } ////// Gets the number of items in the ///. /// /// public CssStyleCollection CssStyle { get { if (_styleColl == null) { _styleColl = new CssStyleCollection(_bag); } return _styleColl; } } ////// public void Add(string key, string value) { if (_styleColl != null && StringUtil.EqualsIgnoreCase(key, "style")) _styleColl.Value = value; else _bag[key] = value; } public override bool Equals(object o) { // This implementation of Equals relies on mutable properties and is therefore broken, // but we shipped it this way in V1 so it will be a breaking change to fix it. AttributeCollection attrs = o as AttributeCollection; if (attrs != null) { if (attrs.Count != _bag.Count) { return false; } foreach (DictionaryEntry attr in _bag) { if (this[(string)attr.Key] != attrs[(string)attr.Key]) { return false; } } return true; } return false; } public override int GetHashCode() { // This implementation of GetHashCode uses mutable properties but matches the V1 implementation // of Equals. HashCodeCombiner hashCodeCombiner = new HashCodeCombiner(); foreach (DictionaryEntry attr in _bag) { hashCodeCombiner.AddObject(attr.Key); hashCodeCombiner.AddObject(attr.Value); } return hashCodeCombiner.CombinedHash32; } ////// Adds an item to the ///. /// /// public void Remove(string key) { if (_styleColl != null && StringUtil.EqualsIgnoreCase(key, "style")) _styleColl.Clear(); else _bag.Remove(key); } ////// Removes an attribute from the ///. /// /// public void Clear() { _bag.Clear(); if (_styleColl != null) _styleColl.Clear(); } ////// Removes all attributes from the ///. /// /// public void Render(HtmlTextWriter writer) { if (_bag.Count > 0) { IDictionaryEnumerator e = _bag.GetEnumerator(); while (e.MoveNext()) { StateItem item = e.Value as StateItem; if (item != null) { string value = item.Value as string; string key = e.Key as string; if (key != null && value != null) { writer.WriteAttribute(key, value, true /*fEncode*/); } } } } } ///[To be supplied.] ////// public void AddAttributes(HtmlTextWriter writer) { if (_bag.Count > 0) { IDictionaryEnumerator e = _bag.GetEnumerator(); while (e.MoveNext()) { StateItem item = e.Value as StateItem; if (item != null) { string value = item.Value as string; string key = e.Key as string; if (key != null && value != null) { writer.AddAttribute(key, value, true /*fEncode*/); } } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
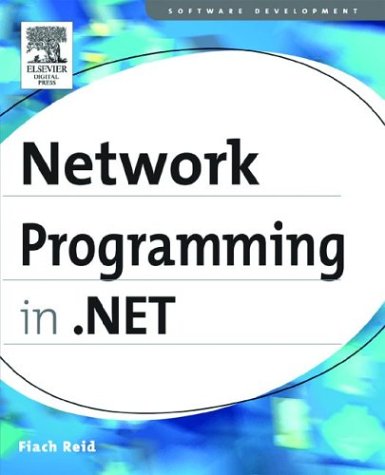
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebScriptMetadataInstanceContextProvider.cs
- Asn1IntegerConverter.cs
- Image.cs
- Wildcard.cs
- CellIdBoolean.cs
- DesignerSerializerAttribute.cs
- IsolatedStorage.cs
- TransformerTypeCollection.cs
- ListViewHitTestInfo.cs
- OAVariantLib.cs
- SignatureToken.cs
- EntitySqlException.cs
- X509Chain.cs
- KeyedCollection.cs
- DbExpressionVisitor_TResultType.cs
- DecoderNLS.cs
- ToolStripItemGlyph.cs
- FilteredAttributeCollection.cs
- SqlCacheDependencySection.cs
- Matrix.cs
- filewebresponse.cs
- BindingBase.cs
- EntityDataSourceMemberPath.cs
- DataTrigger.cs
- AppendHelper.cs
- BasicExpressionVisitor.cs
- SerialReceived.cs
- FaultCode.cs
- ColorTransform.cs
- TypeGeneratedEventArgs.cs
- arabicshape.cs
- ConfigurationSectionGroup.cs
- DataControlFieldCell.cs
- FormCollection.cs
- CalendarDay.cs
- AstTree.cs
- LicenseContext.cs
- DynamicMethod.cs
- SourceChangedEventArgs.cs
- MatchingStyle.cs
- MsmqChannelListenerBase.cs
- SafeEventLogWriteHandle.cs
- IDReferencePropertyAttribute.cs
- Privilege.cs
- FlagsAttribute.cs
- QueryOutputWriter.cs
- CaseStatementProjectedSlot.cs
- SessionStateModule.cs
- GroupItemAutomationPeer.cs
- X500Name.cs
- CounterSet.cs
- SqlFunctionAttribute.cs
- SystemIPInterfaceProperties.cs
- MultiBindingExpression.cs
- CommandHelpers.cs
- XPathScanner.cs
- MessagingDescriptionAttribute.cs
- IdentityNotMappedException.cs
- ConstructorNeedsTagAttribute.cs
- SecurityTokenException.cs
- util.cs
- RegistryExceptionHelper.cs
- GridViewHeaderRowPresenter.cs
- IssuedTokenClientCredential.cs
- DataTableCollection.cs
- CodeDirectoryCompiler.cs
- UIElementParaClient.cs
- GridViewSortEventArgs.cs
- DSACryptoServiceProvider.cs
- NumberAction.cs
- HistoryEventArgs.cs
- Renderer.cs
- LocalizabilityAttribute.cs
- EntityDesignerBuildProvider.cs
- WindowsHyperlink.cs
- ServiceReference.cs
- HttpResponseHeader.cs
- ControlUtil.cs
- BufferedWebEventProvider.cs
- DataGridCellsPanel.cs
- VectorAnimationBase.cs
- TextRunCacheImp.cs
- RepeaterItem.cs
- ProxyElement.cs
- SiteMapDataSourceView.cs
- WebSysDefaultValueAttribute.cs
- ModuleConfigurationInfo.cs
- ServiceReference.cs
- MessageQueueAccessControlEntry.cs
- HostedElements.cs
- UriPrefixTable.cs
- ActionNotSupportedException.cs
- XamlPoint3DCollectionSerializer.cs
- WmpBitmapEncoder.cs
- ProfileService.cs
- Pair.cs
- sqlstateclientmanager.cs
- _NTAuthentication.cs
- GeometryConverter.cs
- AssemblyBuilder.cs