Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachVisualSerializer.cs / 1 / ReachVisualSerializer.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachVisualSerializer.cs Abstract: Author: [....] ([....]) 1-December-2004 Revision History: --*/ using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Markup; namespace System.Windows.Xps.Serialization { ////// /// internal class ReachVisualSerializer : ReachSerializer { ////// /// public ReachVisualSerializer( PackageSerializationManager manager ): base(manager) { } ////// /// public override void SerializeObject( object serializedObject ) { Visual v = serializedObject as Visual; if (v == null) { throw new ArgumentException(ReachSR.Get(ReachSRID.MustBeOfType, "serializedObject", typeof(Visual))); } XmlWriter pageWriter = ((XpsSerializationManager)SerializationManager). PackagingPolicy.AcquireXmlWriterForPage(); XmlWriter resWriter = ((XpsSerializationManager)SerializationManager). PackagingPolicy.AcquireXmlWriterForResourceDictionary(); SerializeTree(v, resWriter, pageWriter); } ////// /// internal override void SerializeObject( SerializablePropertyContext serializedProperty ) { // // Do nothing here. // We do not support serializing visuals that come in as // properties out of context of a FixedPage or a DocumentPage // } private void SerializeTree( Visual visual, XmlWriter resWriter, XmlWriter bodyWriter ) { Toolbox.StartEvent(Toolbox.DRXSERIALIZETREEGUID); Size fixedPageSize = ((XpsSerializationManager)SerializationManager).FixedPageSize; VisualTreeFlattener flattener = ((XpsSerializationManager)SerializationManager). VisualSerializationService.AcquireVisualTreeFlattener(resWriter, bodyWriter, fixedPageSize); StackcontextStack = new Stack (); if (flattener.StartVisual(visual)) { contextStack.Push(new NodeContext(visual)); } while (contextStack.Count > 0) { NodeContext ctx = contextStack.Peek(); Visual v = ctx.GetNextChild(); if (v != null) { if (flattener.StartVisual(v)) { contextStack.Push(new NodeContext(v)); } } else { contextStack.Pop(); flattener.EndVisual(); } } Toolbox.EndEvent(Toolbox.DRXSERIALIZETREEGUID); } /// /// /// internal override void PersistObjectData( SerializableObjectContext serializableObjectContext ) { // // Do nothing here // } ////// /// public override XmlWriter XmlWriter { get { if(base.XmlWriter == null) { base.XmlWriter = SerializationManager.AcquireXmlWriter(typeof(FixedPage)); } return base.XmlWriter; } set { base.XmlWriter = null; SerializationManager.ReleaseXmlWriter(typeof(FixedPage)); } } #region Internal Methods ////// /// internal bool SerializeDisguisedVisual( object serializedObject ) { Visual v = serializedObject as Visual; if (v == null) { throw new ArgumentException(ReachSR.Get(ReachSRID.MustBeOfType, "serializedObject", typeof(Visual))); } XmlWriter pageWriter = ((XpsSerializationManager)SerializationManager). PackagingPolicy.AcquireXmlWriterForPage(); XmlWriter resWriter = ((XpsSerializationManager)SerializationManager). PackagingPolicy.AcquireXmlWriterForResourceDictionary(); Size fixedPageSize = ((XpsSerializationManager)SerializationManager).FixedPageSize; VisualTreeFlattener flattener = ((XpsSerializationManager)SerializationManager). VisualSerializationService.AcquireVisualTreeFlattener(resWriter, pageWriter, fixedPageSize); return flattener.StartVisual(v); } #endregion Internal Methods }; class NodeContext { #region Constructor public NodeContext(Visual v) { nodeVisual = v; index = 0; } #endregion Constructor #region Public properties public Visual NodeVisual { get { return nodeVisual; } } #endregion Public properties #region Public methods public Visual GetNextChild() { Visual child = null; if (index < VisualTreeHelper.GetChildrenCount(nodeVisual)) { // VisualTreeFlattener will flatten Viewport3DVisual into an image. We shouldn't // be attempting to walk Visual3D children. child = (Visual) VisualTreeHelper.GetChild(nodeVisual, index); index++; } return child; } #endregion Public methods #region Private data private Visual nodeVisual; private int index; #endregion Private data } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
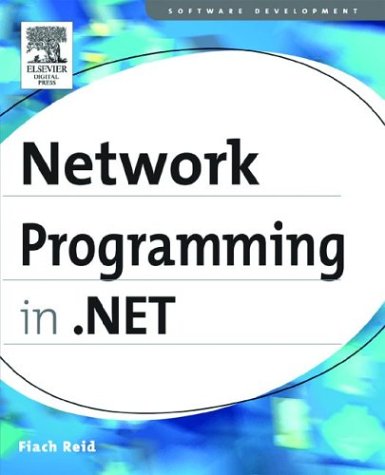
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StrongNameKeyPair.cs
- AddInServer.cs
- SecurityResources.cs
- CodeAttachEventStatement.cs
- RawStylusInput.cs
- BitmapMetadataEnumerator.cs
- XsltArgumentList.cs
- OutputScopeManager.cs
- X509Extension.cs
- Pipe.cs
- DataGridViewButtonColumn.cs
- IsolatedStorageFile.cs
- COMException.cs
- IsolatedStorage.cs
- JsonStringDataContract.cs
- TerminatorSinks.cs
- Rules.cs
- DiscoveryMessageSequenceGenerator.cs
- EntityReference.cs
- DataColumnCollection.cs
- DotAtomReader.cs
- ProfilePropertyNameValidator.cs
- VoiceSynthesis.cs
- WebPartsSection.cs
- PanelStyle.cs
- RelatedView.cs
- Nodes.cs
- GeometryHitTestResult.cs
- SiteMapHierarchicalDataSourceView.cs
- IssuedTokenServiceCredential.cs
- JapaneseLunisolarCalendar.cs
- TextParentUndoUnit.cs
- StylusPointPropertyId.cs
- DesignerActionService.cs
- coordinator.cs
- SafeFileMappingHandle.cs
- mediaeventshelper.cs
- FileDialog_Vista_Interop.cs
- WebPartConnectionsCancelVerb.cs
- __Error.cs
- RuleSettings.cs
- Tablet.cs
- SettingsProviderCollection.cs
- oledbmetadatacollectionnames.cs
- MenuItem.cs
- App.cs
- CommandField.cs
- RelativeSource.cs
- MembershipUser.cs
- IndexerNameAttribute.cs
- NumericUpDownAccelerationCollection.cs
- RelatedImageListAttribute.cs
- UriTemplateDispatchFormatter.cs
- TextEffect.cs
- ApplicationBuildProvider.cs
- TextServicesHost.cs
- XmlAnyAttributeAttribute.cs
- TextDecorationLocationValidation.cs
- ClientScriptManager.cs
- CellPartitioner.cs
- ApplicationProxyInternal.cs
- TextDecorationUnitValidation.cs
- PingOptions.cs
- RealProxy.cs
- ActivityWithResultWrapper.cs
- AnimationException.cs
- AddInAdapter.cs
- DependencyObject.cs
- ToolStripArrowRenderEventArgs.cs
- TypeInfo.cs
- MetadataAssemblyHelper.cs
- SqlErrorCollection.cs
- WebPartHeaderCloseVerb.cs
- ToolboxComponentsCreatedEventArgs.cs
- ModifierKeysValueSerializer.cs
- CommentEmitter.cs
- BuildDependencySet.cs
- TemplatedAdorner.cs
- sqlnorm.cs
- DNS.cs
- ChangePasswordDesigner.cs
- HttpWebRequest.cs
- GroupBoxAutomationPeer.cs
- QueryTreeBuilder.cs
- XmlObjectSerializerReadContext.cs
- TypeHelper.cs
- Inline.cs
- BuildProvider.cs
- ArrayExtension.cs
- XmlQueryCardinality.cs
- TextTreeTextNode.cs
- SizeConverter.cs
- ParameterToken.cs
- GridViewUpdatedEventArgs.cs
- TdsRecordBufferSetter.cs
- ConnectionPointCookie.cs
- BaseParaClient.cs
- SettingsAttributeDictionary.cs
- EdmError.cs
- XPathDescendantIterator.cs