Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / SMSvcHost / System / ServiceModel / Activation / App.cs / 1 / App.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System; using System.ServiceModel.Channels; using System.Collections.Generic; using System.Diagnostics; using System.Security.Principal; using System.ServiceModel; class App { string appKey; AppPool appPool; int siteId; IActivatedMessageQueue messageQueue; string path; bool requestBlocked; bool hasInvalidBinding; AppAction pendingAction; internal App(string appKey, string path, int siteId, AppPool appPool, bool requestsBlocked) : base() { Debug.Print("App.ctor(appKey:" + appKey + " path:" + path + " appPoolId:" + appPool.AppPoolId + ")"); this.appKey = appKey; this.path = path; this.appPool = appPool; this.siteId = siteId; this.requestBlocked = requestsBlocked; } internal AppAction PendingAction { get { return this.pendingAction; } } internal void SetPendingAction(AppAction action) { if (action != null) { DiagnosticUtility.DebugAssert(this.pendingAction == null, "There is already a pending action."); } this.pendingAction = action; } internal void RegisterQueue(IActivatedMessageQueue messageQueue) { if (this.messageQueue != null) { DiagnosticUtility.DebugAssert("a message queue was already registered"); throw DiagnosticUtility.ExceptionUtility.ThrowHelperInternal(false); } this.messageQueue = messageQueue; } internal string AppKey { get { return appKey; } } internal AppPool AppPool { get { return appPool; } } internal int SiteId { get { return siteId; } } internal IActivatedMessageQueue MessageQueue { get { return messageQueue; } } internal string Path { get { return path; } set { this.path = value; } } internal void OnAppPoolChanged(AppPool newAppPool) { this.appPool = newAppPool; } internal void SetRequestBlocked(bool requestBlocked) { if (this.requestBlocked != requestBlocked) { this.requestBlocked = requestBlocked; OnStateChanged(); } } internal void OnAppPoolStateChanged() { OnStateChanged(); } internal void OnDeleted(bool appPoolDeleted) { messageQueue.Delete(); } internal bool IsEnabled { get { return this.appPool.IsEnabled && !this.requestBlocked && !this.hasInvalidBinding; } } internal void OnInvalidBinding(bool hasInvalidBinding) { this.hasInvalidBinding = hasInvalidBinding; OnStateChanged(); } void OnStateChanged() { messageQueue.SetEnabledState(this.IsEnabled); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
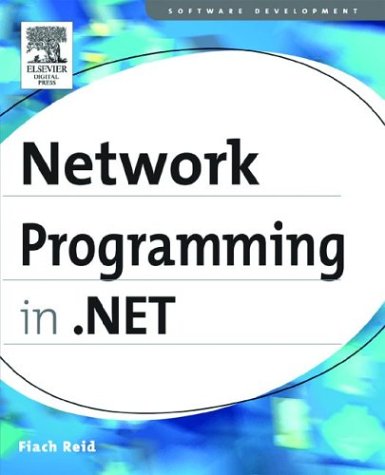
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextParaLineResult.cs
- DesignerSerializationManager.cs
- nulltextcontainer.cs
- CloudCollection.cs
- DefaultBinder.cs
- NavigationExpr.cs
- CheckBox.cs
- IndependentAnimationStorage.cs
- x509store.cs
- CatalogZone.cs
- ToolStripControlHost.cs
- DataGridRelationshipRow.cs
- PermissionToken.cs
- dtdvalidator.cs
- EmptyReadOnlyDictionaryInternal.cs
- ComboBox.cs
- AnnotationAuthorChangedEventArgs.cs
- RequestResizeEvent.cs
- HtmlInputSubmit.cs
- AutomationPropertyInfo.cs
- SupportsEventValidationAttribute.cs
- ProvidersHelper.cs
- SymbolPair.cs
- ExpandedWrapper.cs
- BezierSegment.cs
- DataGridTableCollection.cs
- LinqDataSourceUpdateEventArgs.cs
- X509WindowsSecurityToken.cs
- SafeRsaProviderHandle.cs
- DataStorage.cs
- PluggableProtocol.cs
- LocalBuilder.cs
- FillRuleValidation.cs
- ExpandSegment.cs
- XmlParser.cs
- InkCanvasInnerCanvas.cs
- ObjectStateEntry.cs
- SyndicationSerializer.cs
- ListGeneralPage.cs
- AssertHelper.cs
- PartialCachingControl.cs
- ProxyGenerationError.cs
- TemplateControl.cs
- CounterCreationDataCollection.cs
- SerializerDescriptor.cs
- ObfuscateAssemblyAttribute.cs
- ItemsPresenter.cs
- SocketInformation.cs
- ContextMenu.cs
- PenLineJoinValidation.cs
- CodeSnippetExpression.cs
- CodeDefaultValueExpression.cs
- ControlDesignerState.cs
- RegisteredExpandoAttribute.cs
- TraceEventCache.cs
- VectorConverter.cs
- TdsParserSessionPool.cs
- TrailingSpaceComparer.cs
- ZipIOExtraField.cs
- OdbcConnectionString.cs
- Point3D.cs
- NullableFloatAverageAggregationOperator.cs
- CanonicalFontFamilyReference.cs
- GPPOINTF.cs
- SmtpClient.cs
- NamedElement.cs
- SoapRpcServiceAttribute.cs
- NonParentingControl.cs
- EntityDataSourceChangedEventArgs.cs
- CommandEventArgs.cs
- ResetableIterator.cs
- MetadataUtilsSmi.cs
- SessionParameter.cs
- _TimerThread.cs
- Resources.Designer.cs
- EnumDataContract.cs
- CursorConverter.cs
- ReflectionPermission.cs
- mediaclock.cs
- Assert.cs
- DesignerToolboxInfo.cs
- RegexGroupCollection.cs
- ConditionCollection.cs
- XmlAttributeOverrides.cs
- CmsInterop.cs
- SystemIPGlobalStatistics.cs
- DetailsView.cs
- QilDataSource.cs
- MessageSecurityProtocolFactory.cs
- SqlNode.cs
- AnnouncementDispatcherAsyncResult.cs
- AlternationConverter.cs
- SpecialNameAttribute.cs
- WorkflowOperationBehavior.cs
- TextParentUndoUnit.cs
- XmlSchemaObject.cs
- StateItem.cs
- ToolBarTray.cs
- tibetanshape.cs
- CategoriesDocumentFormatter.cs