Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Packaging / XpsDigitalSignature.cs / 1 / XpsDigitalSignature.cs
/*++ Copyright (C) 2004 - 2005 Microsoft Corporation All rights reserved. Module Name: XpsDigitalSignatureManger.cs Abstract: This is a wrapper class for the Package Digital Signature It provides binding information between the Xps Package and the Digital Signature such as what is the root objects signed and what are the signing restrictions Author: Brian Adelberg ([....]) 2-May-2005 Revision History: 07/12/2005: [....]: Reach -> Xps --*/ using MS.Internal; using System; using System.Windows.Documents; using System.IO.Packaging; using System.Security; // for SecurityCritical tag using System.Security.Permissions; // for LinkDemand using System.Security.Cryptography; using System.Security.Cryptography.X509Certificates; using System.Security.Cryptography.Xml; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Xml; namespace System.Windows.Xps.Packaging { ////// Wrapper class for the Pacakge Digital Signature /// Provides binding information between the Xps Package and the Digital Signature /// public class XpsDigitalSignature { ////// Constructs a XpsDigitalSignature instance to determine /// what was singed by the signature and if it is valid. /// /// /// The associated PacakgeDigitalSignature /// /// /// The associated package /// public XpsDigitalSignature( PackageDigitalSignature packageSignature, XpsDocument package ) { _packageSignature = packageSignature; _package = package; } #region Public properties ////// The Document Sequence Reader for /// the signed Document seqeuence part. /// If this is NULL it idicates that this signature /// only signed pages /// ///Value is the IXpsFixedDocumentSequenceReader for the signed Docuement Sequence Part public IXpsFixedDocumentSequenceReader SignedDocumentSequence { get { IXpsFixedDocumentSequenceReader seqReader = _package.FixedDocumentSequenceReader; IXpsFixedDocumentSequenceReader returnReader = null; if( seqReader != null ) { DictionarydependentList = new Dictionary (); List selectorList = new List (); _package.CollectSelfAndDependents( dependentList, selectorList, XpsDigSigPartAlteringRestrictions.None ); if( CollectionContainsCollection(_packageSignature.SignedParts, dependentList.Keys) && CollectionContainsCollection(dependentList.Keys, _packageSignature.SignedParts) && SelectorListContainsSelectorList(_packageSignature.SignedRelationshipSelectors, selectorList ) ) { returnReader = seqReader; } } return returnReader; } } /// /// returns true if changing the Signature Origin breaks the signature /// public bool SignatureOriginRestricted { get { bool restrictedFlag = false; foreach( PackageRelationshipSelector selector in _packageSignature.SignedRelationshipSelectors ) { if( selector.SourceUri == _package.CurrentXpsManager.GetSignatureOriginUri() && selector.SelectionCriteria == XpsS0Markup.DitialSignatureRelationshipType ) { restrictedFlag = true; break; } } return restrictedFlag; } } ////// returns true if changing the Document Properties breaks the signature /// public bool DocumentPropertiesRestricted { get { bool restrictedFlag = false; foreach( PackageRelationshipSelector selector in _packageSignature.SignedRelationshipSelectors ) { if( selector.SourceUri == PackUriHelper.PackageRootUri && selector.SelectionCriteria == XpsS0Markup.CorePropertiesRelationshipType ) { restrictedFlag = true; break; } } return restrictedFlag; } } ////// Id of the signature /// public Guid? Id { get { Guid? id = null; Signature sig = (Signature)_packageSignature.Signature; if( sig != null ) { try { string convertedId = XmlConvert.DecodeName(sig.Id); id = new Guid(convertedId); } catch( ArgumentNullException ) { id = null; } catch( FormatException ) { id = null; } } return id; } } ////// Certificate of signer embedded in container /// ///null if certificate was not embedded public X509Certificate SignerCertificate { get { return _packageSignature.Signer; } } ////// Time signature was created - not a trusted TimeStamp /// ///public DateTime SigningTime { get { return _packageSignature.SigningTime; } } /// /// encrypted hash value /// ///public byte[] SignatureValue { get { return _packageSignature.SignatureValue; } } /// /// Content Type of signature /// public String SignatureType { get { return _packageSignature.SignatureType; } } ////// True if the package contains the signatures certificate. /// public bool IsCertificateAvailable { get { return ( _packageSignature.CertificateEmbeddingOption == CertificateEmbeddingOption.InCertificatePart); } } #endregion Public properties #region Public methods ////// Verify /// ///cannot use this overload with signatures created without embedding their certs ///public VerifyResult Verify() { return _packageSignature.Verify(); } /// /// Verify /// ///cannot use this overload with signatures created without embedding their certs ////// /// Certificate to be used to verify /// public VerifyResult Verify(X509Certificate certificate) { return _packageSignature.Verify(certificate); } /// /// Verify Certificate /// Uses the certificate stored the signature /// ///the first error encountered when inspecting the certificate chain or NoError if the certificate is valid ////// Critical - The VerifyCertificate(certificate) method has a /// LinkDemand for Unrestricted. This is propogating that LinkDemand. /// PublicOK � VerifyCertificate has LinkDemand. /// [SecurityCritical] [SecurityPermissionAttribute(SecurityAction.LinkDemand, Unrestricted = true)] public X509ChainStatusFlags VerifyCertificate() { return VerifyCertificate(_packageSignature.Signer); } ////// Verify Certificate /// /// certificate to inspect ///the first error encountered when inspecting the certificate chain or NoError if the certificate is valid ////// Critical - The PackageDigitalSignatureManager.VerifyCertificate method has a /// LinkDemand for Unrestricted. This is propogating that LinkDemand. /// PublicOK � VerifyCertificate has LinkDemand. /// [SecurityCritical] [SecurityPermissionAttribute(SecurityAction.LinkDemand, Unrestricted = true)] public static X509ChainStatusFlags VerifyCertificate(X509Certificate certificate) { return PackageDigitalSignatureManager.VerifyCertificate(certificate); } #endregion Public methods #region Internal property internal PackageDigitalSignature PackageSignature { get { return _packageSignature; } } #endregion Internal property #region Private methods bool CollectionContainsCollection( ICollectioncontainingCollection, ICollection containedCollection ) { bool contained = true; // // Convert the containing collection to a hash table Dictionary hashTable = new Dictionary (); foreach( Uri uri in containingCollection ) { hashTable[uri] = uri; } // // Iteratate the contained collection // and cofirm existance in the contained collection // foreach( Uri uri in containedCollection ) { bool isOptional = IsOptional( uri ); if( !hashTable.ContainsKey( uri )&& !isOptional) { contained = false; break; } } return contained; } /// /// This returns true if the part can optionally be signed /// XML Paper Specification 10.2.1.1 Signing rules /// private bool IsOptional( Uri uri ) { string contentType = _package.CurrentXpsManager.MetroPackage.GetPart(uri).ContentType; return( OptionalSignedParts.ContainsKey( contentType ) ); } ////// This determines if the contained collection is a subset of the containting collection /// For each Source Uri in the Containging collection there must be the coorisponding /// selection criteria (relationship types) /// /// The super set collection /// The sub set collection ///returns true if is the contained collection is a subset of the containing collection bool SelectorListContainsSelectorList( ReadOnlyCollectioncontainingCollection, List containedCollection ) { bool contained = true; // // Convert the containing collection to a hash table Dictionary > uriHashTable = new Dictionary >(); foreach (PackageRelationshipSelector selector in containingCollection) { // // If the Source Uri is already in the hash table // pull out the existing relationship dictionary Dictionary relHash = null; if( uriHashTable.ContainsKey( selector.SourceUri ) ) { relHash = uriHashTable[selector.SourceUri]; } // // Else create a new one and add it into Uri Hash // else { relHash = new Dictionary (); uriHashTable[selector.SourceUri] = relHash; } // // The value is unused we are using this as a hash table // relHash[selector.SelectionCriteria] = 0; } // // Iteratate the contained collection // and cofirm existance in the contained collection // foreach (PackageRelationshipSelector selector in containedCollection) { // // If the source Uri is not in the hash this fails if (!uriHashTable.ContainsKey(selector.SourceUri)) { contained = false; break; } else { Dictionary relHash = uriHashTable[selector.SourceUri]; if (!relHash.ContainsKey(selector.SelectionCriteria)) { contained = false; break; } } } return contained; } Dictionary OptionalSignedParts { get { if( _optionalSignedTypes == null ) { _optionalSignedTypes = new Dictionary (); _optionalSignedTypes[XpsS0Markup.CoreDocumentPropertiesType.OriginalString] = ""; _optionalSignedTypes[XpsS0Markup.SignatureDefintionType.OriginalString] = ""; _optionalSignedTypes[XpsS0Markup.PrintTicketContentType.OriginalString] = ""; _optionalSignedTypes[XpsS0Markup.SigOriginContentType.OriginalString] = ""; _optionalSignedTypes[XpsS0Markup.SigCertContentType.OriginalString] = ""; _optionalSignedTypes[XpsS0Markup.DiscardContentType.OriginalString] = ""; _optionalSignedTypes[XpsS0Markup.RelationshipContentType.OriginalString] = ""; } return _optionalSignedTypes; } } #endregion Private methods #region Private data private PackageDigitalSignature _packageSignature; private XpsDocument _package; static private Dictionary _optionalSignedTypes; #endregion Private data } /// /// Flags indicating which parts are to be exluded /// from a digital signature /// May be or'ed together /// [FlagsAttribute] public enum XpsDigSigPartAlteringRestrictions { ////// all depedent parts will be signed /// None = 0x00000000, ////// Meta data will be exluded /// CoreMetadata = 0x00000001, ////// Annotations will be exluded /// Annotations = 0x00000002, ////// The signature will be exluded /// SignatureOrigin = 0x00000004 }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
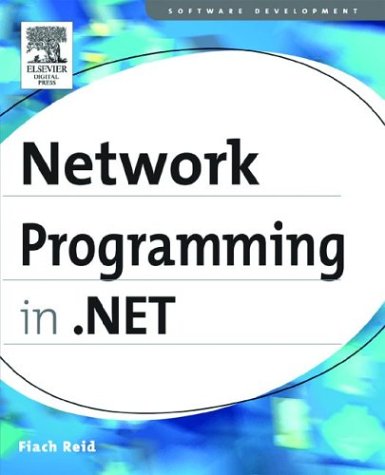
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DurationConverter.cs
- ContentType.cs
- OdbcConnectionHandle.cs
- FieldAccessException.cs
- RootNamespaceAttribute.cs
- Tokenizer.cs
- Int32.cs
- SeverityFilter.cs
- CheckableControlBaseAdapter.cs
- ResponseStream.cs
- DataSourceSerializationException.cs
- WebEventTraceProvider.cs
- SplineKeyFrames.cs
- Vector3D.cs
- GridEntry.cs
- AliasExpr.cs
- PaintValueEventArgs.cs
- SqlTransaction.cs
- InvalidCastException.cs
- GridViewDeletedEventArgs.cs
- ISAPIRuntime.cs
- ClientEventManager.cs
- DataGridViewAutoSizeColumnsModeEventArgs.cs
- SystemPens.cs
- GlobalizationSection.cs
- RecognizedPhrase.cs
- FixedSOMLineRanges.cs
- XsltFunctions.cs
- dataSvcMapFileLoader.cs
- SessionParameter.cs
- PartialToken.cs
- PersistChildrenAttribute.cs
- AddressHeaderCollectionElement.cs
- DbInsertCommandTree.cs
- BindingsCollection.cs
- Fonts.cs
- XmlAttribute.cs
- Reference.cs
- MatrixAnimationUsingPath.cs
- QilBinary.cs
- SchemaCollectionPreprocessor.cs
- EditingCommands.cs
- SmiConnection.cs
- DataFormats.cs
- Formatter.cs
- AppDomainProtocolHandler.cs
- TraceUtils.cs
- InputBuffer.cs
- DoubleAnimation.cs
- PrefixQName.cs
- ValueProviderWrapper.cs
- BlockUIContainer.cs
- UriExt.cs
- NumberFormatter.cs
- NodeFunctions.cs
- PrinterResolution.cs
- XmlEntityReference.cs
- TagMapCollection.cs
- CodeStatementCollection.cs
- RestHandler.cs
- OletxResourceManager.cs
- CodeEntryPointMethod.cs
- MessageQueuePermissionAttribute.cs
- DuplicateWaitObjectException.cs
- ValidationService.cs
- RegexCompilationInfo.cs
- NamespaceList.cs
- BuiltInExpr.cs
- filewebrequest.cs
- NonVisualControlAttribute.cs
- XmlTextWriter.cs
- TableCell.cs
- DefaultSettingsSection.cs
- Regex.cs
- QilExpression.cs
- PowerStatus.cs
- EntitySetRetriever.cs
- IDReferencePropertyAttribute.cs
- RefreshPropertiesAttribute.cs
- MyContact.cs
- VisualTreeFlattener.cs
- ConstraintConverter.cs
- TrackPointCollection.cs
- ByteAnimationUsingKeyFrames.cs
- Block.cs
- Converter.cs
- EncryptedHeader.cs
- SolidBrush.cs
- ListViewGroup.cs
- SkewTransform.cs
- ZipIOExtraField.cs
- NumberFormatter.cs
- Utils.cs
- PeerNameRecord.cs
- PageAsyncTask.cs
- XmlNodeReader.cs
- NumericUpDown.cs
- TransformerTypeCollection.cs
- XmlWellformedWriter.cs
- CustomAttributeSerializer.cs