Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Printing / PrinterResolution.cs / 1305376 / PrinterResolution.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Globalization; ////// /// [Serializable] public class PrinterResolution { private int x; private int y; private PrinterResolutionKind kind; ///Retrieves /// the resolution supported by a printer. ////// /// public PrinterResolution() { this.kind = PrinterResolutionKind.Custom; } internal PrinterResolution(PrinterResolutionKind kind, int x, int y) { this.kind = kind; this.x = x; this.y = y; } ////// Initializes a new instance of the ///class with default properties. /// This constructor is required for the serialization of the class. /// /// /// public PrinterResolutionKind Kind { get { return kind;} set { //valid values are 0xfffffffc to 0x0 if (!ClientUtils.IsEnumValid(value, (int)value, (int)PrinterResolutionKind.High, (int)PrinterResolutionKind.Custom)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(PrinterResolutionKind)); } kind = value; } } ////// Gets /// a value indicating the kind of printer resolution. /// Setter added to enable serialization of the PrinterSettings object. /// ////// /// public int X { get { return x; } set { x = value; } } ////// Gets the printer resolution in the horizontal direction, /// in dots per inch. /// Setter added to enable serialization of the PrinterSettings object. /// ////// /// public int Y { get { return y; } set { y = value; } } ///Gets the printer resolution in the vertical direction, /// in dots per inch. /// Setter added to enable serialization of the PrinterSettings object. /// ////// /// /// public override string ToString() { if (kind != PrinterResolutionKind.Custom) return "[PrinterResolution " + TypeDescriptor.GetConverter(typeof(PrinterResolutionKind)).ConvertToString((int) Kind) + "]"; else return "[PrinterResolution" + " X=" + X.ToString(CultureInfo.InvariantCulture) + " Y=" + Y.ToString(CultureInfo.InvariantCulture) + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Provides some interesting information about the PrinterResolution in /// String form. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Globalization; ////// /// [Serializable] public class PrinterResolution { private int x; private int y; private PrinterResolutionKind kind; ///Retrieves /// the resolution supported by a printer. ////// /// public PrinterResolution() { this.kind = PrinterResolutionKind.Custom; } internal PrinterResolution(PrinterResolutionKind kind, int x, int y) { this.kind = kind; this.x = x; this.y = y; } ////// Initializes a new instance of the ///class with default properties. /// This constructor is required for the serialization of the class. /// /// /// public PrinterResolutionKind Kind { get { return kind;} set { //valid values are 0xfffffffc to 0x0 if (!ClientUtils.IsEnumValid(value, (int)value, (int)PrinterResolutionKind.High, (int)PrinterResolutionKind.Custom)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(PrinterResolutionKind)); } kind = value; } } ////// Gets /// a value indicating the kind of printer resolution. /// Setter added to enable serialization of the PrinterSettings object. /// ////// /// public int X { get { return x; } set { x = value; } } ////// Gets the printer resolution in the horizontal direction, /// in dots per inch. /// Setter added to enable serialization of the PrinterSettings object. /// ////// /// public int Y { get { return y; } set { y = value; } } ///Gets the printer resolution in the vertical direction, /// in dots per inch. /// Setter added to enable serialization of the PrinterSettings object. /// ////// /// /// public override string ToString() { if (kind != PrinterResolutionKind.Custom) return "[PrinterResolution " + TypeDescriptor.GetConverter(typeof(PrinterResolutionKind)).ConvertToString((int) Kind) + "]"; else return "[PrinterResolution" + " X=" + X.ToString(CultureInfo.InvariantCulture) + " Y=" + Y.ToString(CultureInfo.InvariantCulture) + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Provides some interesting information about the PrinterResolution in /// String form. /// ///
Link Menu
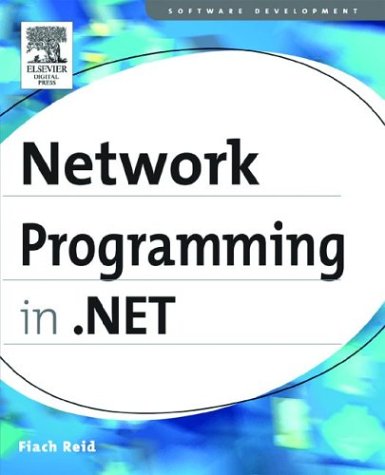
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpSysSettings.cs
- util.cs
- ComponentDispatcherThread.cs
- SQLRoleProvider.cs
- WebPartConnectionsCancelEventArgs.cs
- RemotingServices.cs
- CommonServiceBehaviorElement.cs
- CodeGroup.cs
- Animatable.cs
- Rotation3DAnimationUsingKeyFrames.cs
- PeerNameResolver.cs
- UIPropertyMetadata.cs
- DoubleLinkListEnumerator.cs
- TemplateColumn.cs
- IResourceProvider.cs
- PartManifestEntry.cs
- ColumnMap.cs
- InputScopeConverter.cs
- FrameworkReadOnlyPropertyMetadata.cs
- NumberFormatter.cs
- DSASignatureFormatter.cs
- OleCmdHelper.cs
- DelegatingTypeDescriptionProvider.cs
- WebServiceEnumData.cs
- RequiredFieldValidator.cs
- XpsFontSerializationService.cs
- dtdvalidator.cs
- ApplicationCommands.cs
- WebScriptMetadataInstanceContextProvider.cs
- XPathDocumentBuilder.cs
- TraceRecord.cs
- EntityDataSourceDataSelection.cs
- ActiveDocumentEvent.cs
- SQLUtility.cs
- LookupTables.cs
- SyntaxCheck.cs
- ByteAnimationBase.cs
- DbgUtil.cs
- ListControl.cs
- TagMapCollection.cs
- MouseButtonEventArgs.cs
- TextTreeExtractElementUndoUnit.cs
- StringAttributeCollection.cs
- GetCertificateRequest.cs
- XmlObjectSerializerWriteContext.cs
- ReflectionPermission.cs
- Timer.cs
- ContractMapping.cs
- ComPlusServiceLoader.cs
- SelfIssuedAuthRSAPKCS1SignatureDeformatter.cs
- RawStylusInput.cs
- DesignTimeTemplateParser.cs
- ValueQuery.cs
- ClientRoleProvider.cs
- PointHitTestResult.cs
- ServicePointManagerElement.cs
- RequestCacheManager.cs
- BeginCreateSecurityTokenRequest.cs
- SqlProviderManifest.cs
- SqlBuffer.cs
- AudioBase.cs
- Soap12FormatExtensions.cs
- mactripleDES.cs
- WebRequest.cs
- PropertyStore.cs
- wgx_exports.cs
- Scripts.cs
- RedBlackList.cs
- DataGridViewCellStateChangedEventArgs.cs
- ApplicationServiceHelper.cs
- MessageQueueException.cs
- AccessibleObject.cs
- ImpersonateTokenRef.cs
- EventPrivateKey.cs
- WCFBuildProvider.cs
- Dictionary.cs
- UpDownEvent.cs
- MLangCodePageEncoding.cs
- SmtpCommands.cs
- DateTimeSerializationSection.cs
- ClientEventManager.cs
- ChildDocumentBlock.cs
- LinqDataView.cs
- DataControlLinkButton.cs
- Point3DAnimation.cs
- FactoryId.cs
- Tokenizer.cs
- InternalsVisibleToAttribute.cs
- RegexCaptureCollection.cs
- SplayTreeNode.cs
- DeploymentSection.cs
- StylusShape.cs
- ObjectViewListener.cs
- GPStream.cs
- HandlerBase.cs
- PerformanceCounterManager.cs
- PseudoWebRequest.cs
- HttpProfileBase.cs
- Utils.cs
- InitiatorSessionSymmetricMessageSecurityProtocol.cs