Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / GetCertificateRequest.cs / 1 / GetCertificateRequest.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Security.Cryptography.X509Certificates; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary // Requests the certificate for the specified recipient. // internal class GetCertificateRequest : UIAgentRequest { string m_recipientIdentifier; // Stores the recipient identifier. X509Certificate2 m_certificate; // Stores reference to the certificate. public GetCertificateRequest( IntPtr rpcHandle, Stream inArgs, Stream outArgs, ClientUIRequest parent ) : base( rpcHandle, inArgs, outArgs, parent ) { } // // Summary // Reads the recipient identifier from the argument stream. // protected override void OnMarshalInArgs() { BinaryReader reader = new BinaryReader( InArgs, System.Text.Encoding.Unicode ); m_recipientIdentifier = Utility.DeserializeString( reader ); } // // Summary // Retrieves the certificate from the cache. // protected override void OnProcess() { m_certificate = ParentRequest.CertCacheFind( m_recipientIdentifier ); if( null == m_certificate ) { throw IDT.ThrowHelperError( new InfoCardArgumentException( SR.GetString( SR.NoCachedCertificateForRecipient ) ) ); } } // // Summary // Writes the encoded certificate to the argument stream. // protected override void OnMarshalOutArgs() { BinaryWriter writer = new BinaryWriter( OutArgs, System.Text.Encoding.Unicode ); Utility.SerializeBytes( writer, m_certificate.GetRawCertData() ); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
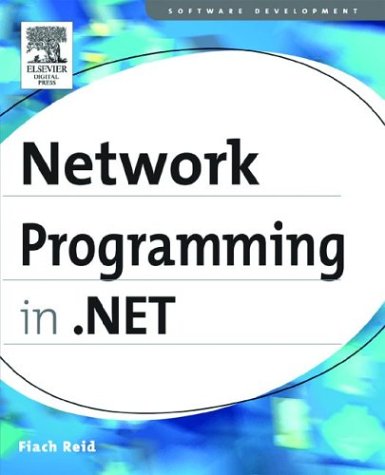
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageRanges.cs
- LabelDesigner.cs
- DoubleCollection.cs
- DbTypeMap.cs
- WebRequestModuleElement.cs
- XmlSchemaExternal.cs
- XmlTextReaderImplHelpers.cs
- DeploymentSectionCache.cs
- DBSqlParserTable.cs
- NonParentingControl.cs
- TableAutomationPeer.cs
- metadatamappinghashervisitor.cs
- VariableQuery.cs
- ParserStack.cs
- UpdateEventArgs.cs
- WebBrowserSiteBase.cs
- ListView.cs
- ContextMarshalException.cs
- DataBindEngine.cs
- ValidationHelper.cs
- Filter.cs
- ImageSource.cs
- WebPartDisplayModeCancelEventArgs.cs
- ResourcePool.cs
- UpdatePanelTriggerCollection.cs
- DataControlFieldCollection.cs
- PropertyReferenceSerializer.cs
- MachineKeyConverter.cs
- KeyGestureConverter.cs
- TemplatingOptionsDialog.cs
- PerformanceCountersBase.cs
- SqlNodeAnnotation.cs
- ListItemConverter.cs
- ContactManager.cs
- QilExpression.cs
- Select.cs
- WebGetAttribute.cs
- RowsCopiedEventArgs.cs
- ETagAttribute.cs
- ClassValidator.cs
- EditingMode.cs
- TagNameToTypeMapper.cs
- Vector3DCollection.cs
- ContractUtils.cs
- Group.cs
- WmlValidatorAdapter.cs
- DataGridRelationshipRow.cs
- BrowsableAttribute.cs
- ContentPlaceHolder.cs
- MiniLockedBorderGlyph.cs
- Processor.cs
- AlternateView.cs
- LoginView.cs
- StrongNameMembershipCondition.cs
- X509Extension.cs
- OracleTimeSpan.cs
- InternalTypeHelper.cs
- DoubleMinMaxAggregationOperator.cs
- RuleRef.cs
- RegisteredDisposeScript.cs
- CompositeCollection.cs
- TimeoutConverter.cs
- RenderData.cs
- MessageQueuePermission.cs
- DataSourceView.cs
- PagerSettings.cs
- MediaTimeline.cs
- X500Name.cs
- Ipv6Element.cs
- DeflateStream.cs
- StringSorter.cs
- Debugger.cs
- DataGridViewCellValidatingEventArgs.cs
- AdapterDictionary.cs
- DirtyTextRange.cs
- GPPOINT.cs
- ServiceNameElement.cs
- DataStreams.cs
- MissingMethodException.cs
- DirectionalLight.cs
- SqlMethodCallConverter.cs
- NamespaceMapping.cs
- SoapInteropTypes.cs
- PersonalizationEntry.cs
- JournalEntryListConverter.cs
- BitSet.cs
- Margins.cs
- PointAnimationBase.cs
- X509Certificate2.cs
- RightsManagementInformation.cs
- UriParserTemplates.cs
- Binding.cs
- SQLByteStorage.cs
- TextRangeAdaptor.cs
- ObfuscationAttribute.cs
- XamlPointCollectionSerializer.cs
- PageEventArgs.cs
- TopClause.cs
- ProfileBuildProvider.cs
- WebPartCancelEventArgs.cs