Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / MS / Internal / AppModel / AppModelKnownContentFactory.cs / 1 / AppModelKnownContentFactory.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Provides a method to turn a baml stream into an object. // // // History: // 11/22/04: [....]: Initial Creation // // //--------------------------------------------------------------------------- using System; using System.IO; using System.Net; // WebPermission. using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Markup; using System.Windows.Navigation; using MS.Internal.Controls; using MS.Internal.Navigation; using MS.Internal.Utility; using MS.Internal.Resources; using System.IO.Packaging; using MS.Internal.PresentationFramework; using System.ComponentModel; namespace MS.Internal.AppModel { // !!!! Note: Those methods are registered as MimeObjectFactory.StreamToObjectFactoryDelegate. The caller expects the // delgate to close stream. internal static class AppModelKnownContentFactory { //// Creates an object instance from a Baml stream and it's Uri // internal static object BamlConverter(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { asyncObjectConverter = null; // If this stream comes from outside the application throw // if (!BaseUriHelper.IsPackApplicationUri(baseUri)) { throw new InvalidOperationException(SR.Get(SRID.BamlIsNotSupportedOutsideOfApplicationResources)); } // If this stream comes from a content file also throw Uri partUri = PackUriHelper.GetPartUri(baseUri); string partName, assemblyName, assemblyVersion, assemblyKey; BaseUriHelper.GetAssemblyNameAndPart(partUri, out partName, out assemblyName, out assemblyVersion, out assemblyKey); if (ContentFileHelper.IsContentFile(partName)) { throw new InvalidOperationException(SR.Get(SRID.BamlIsNotSupportedOutsideOfApplicationResources)); } ParserContext pc = new ParserContext(); pc.BaseUri = baseUri; pc.SkipJournaledProperties = isJournalNavigation; return Application.LoadBamlStreamWithSyncInfo(stream, pc); } //// Creates an object instance from a Xaml stream and it's Uri // ////// Critical - Keep track of this, as sandboxing is meant to be a way for an application to /// isolate xaml content from the hosting application. /// TreatAsSafe - the act of putting this content in a new webbrowser control (and by extention, a new PresentationHost) /// isolates the xaml content from the rest of the application. /// [SecurityCritical, SecurityTreatAsSafe] internal static object XamlConverter(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { asyncObjectConverter = null; if (sandboxExternalContent) { if (SecurityHelper.AreStringTypesEqual(baseUri.Scheme, BaseUriHelper.PackAppBaseUri.Scheme)) { baseUri = BaseUriHelper.ConvertPackUriToAbsoluteExternallyVisibleUri(baseUri); } stream.Close(); return new WebBrowser(baseUri);; } else { ParserContext pc = new ParserContext(); pc.BaseUri = baseUri; pc.SkipJournaledProperties = isJournalNavigation; pc.XamlTypeMapper = XmlParserDefaults.DefaultMapper; if (allowAsync) { XamlReader xr = new XamlReader(); asyncObjectConverter = xr; xr.LoadCompleted += new AsyncCompletedEventHandler(OnParserComplete); // XamlReader.Load will close the stream. return xr.LoadAsync(stream, pc); } else { // XamlReader.Load will close the stream. return XamlReader.Load(stream, pc); } } } private static void OnParserComplete(object sender, AsyncCompletedEventArgs args) { // We can get this event from cancellation. We do not care about the error if there is any // that happened as a result of cancellation. if ((! args.Cancelled) && (args.Error != null)) { throw args.Error; } } internal static object HtmlXappConverter(Stream stream, Uri baseUri, bool canUseTopLevelBrowser, bool sandboxExternalContent, bool allowAsync, bool isJournalNavigation, out XamlReader asyncObjectConverter) { asyncObjectConverter = null; if (canUseTopLevelBrowser) { return null; } if (SecurityHelper.AreStringTypesEqual(baseUri.Scheme, BaseUriHelper.PackAppBaseUri.Scheme)) { baseUri = BaseUriHelper.ConvertPackUriToAbsoluteExternallyVisibleUri(baseUri); } stream.Close(); return new WebBrowser(baseUri); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
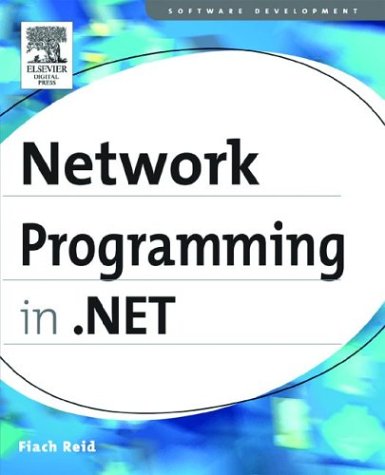
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BindableAttribute.cs
- ReadOnlyDataSourceView.cs
- VectorValueSerializer.cs
- Menu.cs
- LocalizableAttribute.cs
- Message.cs
- XmlChildNodes.cs
- RemoteWebConfigurationHostServer.cs
- DataGridTextBoxColumn.cs
- PeerTransportListenAddressValidatorAttribute.cs
- GridViewPageEventArgs.cs
- CurrentTimeZone.cs
- Descriptor.cs
- NominalTypeEliminator.cs
- WebBodyFormatMessageProperty.cs
- MLangCodePageEncoding.cs
- CodeTypeMember.cs
- TimelineGroup.cs
- CodeDOMProvider.cs
- TextAdaptor.cs
- ReadOnlyCollection.cs
- MemoryFailPoint.cs
- COAUTHINFO.cs
- WindowsTokenRoleProvider.cs
- Attributes.cs
- Quaternion.cs
- WindowsScrollBarBits.cs
- FontFamilyValueSerializer.cs
- JobPageOrder.cs
- DirectoryRedirect.cs
- QilChoice.cs
- NavigationWindowAutomationPeer.cs
- DataKeyCollection.cs
- GenerateTemporaryAssemblyTask.cs
- UnsafeCollabNativeMethods.cs
- XslAstAnalyzer.cs
- SamlAttribute.cs
- Command.cs
- HostedHttpContext.cs
- DesignTimeVisibleAttribute.cs
- WebPartConnectionsConnectVerb.cs
- _ChunkParse.cs
- SystemIcons.cs
- ExtendedPropertyDescriptor.cs
- CryptoHelper.cs
- DataViewSetting.cs
- FormsAuthenticationEventArgs.cs
- HttpProtocolImporter.cs
- ValueProviderWrapper.cs
- SqlParameterizer.cs
- XPathBinder.cs
- TypeReference.cs
- StylusPlugInCollection.cs
- UnsafeNativeMethods.cs
- MsmqChannelListenerBase.cs
- DataGridCellsPanel.cs
- XmlNodeWriter.cs
- MatchingStyle.cs
- SuppressIldasmAttribute.cs
- WorkflowMarkupSerializationManager.cs
- NavigationProperty.cs
- CodeExpressionStatement.cs
- ElementFactory.cs
- documentsequencetextview.cs
- Component.cs
- FileUtil.cs
- SqlCrossApplyToCrossJoin.cs
- LineSegment.cs
- DataControlFieldHeaderCell.cs
- AssociationTypeEmitter.cs
- ACL.cs
- XmlUnspecifiedAttribute.cs
- DateTimePicker.cs
- FrameAutomationPeer.cs
- x509store.cs
- FontConverter.cs
- TemplatePropertyEntry.cs
- XmlIgnoreAttribute.cs
- SchemaMapping.cs
- ImageSource.cs
- FixedSchema.cs
- PointAnimationClockResource.cs
- ApplicationId.cs
- SchemaSetCompiler.cs
- SecurityTokenTypes.cs
- ToolStripSeparator.cs
- MultipleViewPattern.cs
- WebPartDescription.cs
- TransactedBatchContext.cs
- CodeTypeDelegate.cs
- SafeNativeMethods.cs
- Int64Animation.cs
- DoubleLinkListEnumerator.cs
- CalendarDay.cs
- PrintDialog.cs
- ThicknessAnimationBase.cs
- ListViewItemEventArgs.cs
- MethodBuilder.cs
- TypeContext.cs
- NetworkInterface.cs