Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DLinq / Dlinq / SqlClient / Query / SqlCrossApplyToCrossJoin.cs / 1 / SqlCrossApplyToCrossJoin.cs
using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; namespace System.Data.Linq.SqlClient { using System.Data.Linq; ////// Turn CROSS APPLY into CROSS JOIN when the right side /// of the apply doesn't reference anything on the left side. /// /// Any query which has a CROSS APPLY which cannot be converted to /// a CROSS JOIN is annotated so that we can give a meaningful /// error message later for SQL2K. /// internal class SqlCrossApplyToCrossJoin { internal static SqlNode Reduce(SqlNode node, SqlNodeAnnotations annotations) { Reducer r = new Reducer(); r.Annotations = annotations; return r.Visit(node); } class Reducer : SqlVisitor { internal SqlNodeAnnotations Annotations; internal override SqlSource VisitJoin(SqlJoin join) { if (join.JoinType == SqlJoinType.CrossApply) { // Look down the left side to see what table aliases are produced. HashSetp = SqlGatherProducedAliases.Gather(join.Left); // Look down the right side to see what table aliases are consumed. HashSet c = SqlGatherConsumedAliases.Gather(join.Right); // Look at each consumed alias and see if they are mentioned in produced. if (p.Overlaps(c)) { Annotations.Add(join, new SqlServerCompatibilityAnnotation(Strings.SourceExpressionAnnotation(join.SourceExpression), SqlProvider.ProviderMode.Sql2000)); // Can't reduce because this consumed alias is produced on the left. return base.VisitJoin(join); } // Can turn this into a CROSS JOIN join.JoinType = SqlJoinType.Cross; return VisitJoin(join); } return base.VisitJoin(join); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; namespace System.Data.Linq.SqlClient { using System.Data.Linq; /// /// Turn CROSS APPLY into CROSS JOIN when the right side /// of the apply doesn't reference anything on the left side. /// /// Any query which has a CROSS APPLY which cannot be converted to /// a CROSS JOIN is annotated so that we can give a meaningful /// error message later for SQL2K. /// internal class SqlCrossApplyToCrossJoin { internal static SqlNode Reduce(SqlNode node, SqlNodeAnnotations annotations) { Reducer r = new Reducer(); r.Annotations = annotations; return r.Visit(node); } class Reducer : SqlVisitor { internal SqlNodeAnnotations Annotations; internal override SqlSource VisitJoin(SqlJoin join) { if (join.JoinType == SqlJoinType.CrossApply) { // Look down the left side to see what table aliases are produced. HashSetp = SqlGatherProducedAliases.Gather(join.Left); // Look down the right side to see what table aliases are consumed. HashSet c = SqlGatherConsumedAliases.Gather(join.Right); // Look at each consumed alias and see if they are mentioned in produced. if (p.Overlaps(c)) { Annotations.Add(join, new SqlServerCompatibilityAnnotation(Strings.SourceExpressionAnnotation(join.SourceExpression), SqlProvider.ProviderMode.Sql2000)); // Can't reduce because this consumed alias is produced on the left. return base.VisitJoin(join); } // Can turn this into a CROSS JOIN join.JoinType = SqlJoinType.Cross; return VisitJoin(join); } return base.VisitJoin(join); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
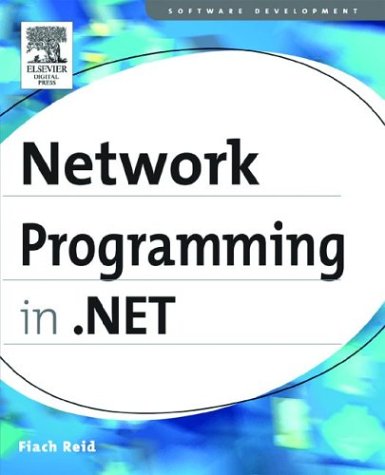
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MenuEventArgs.cs
- HTTPNotFoundHandler.cs
- BrowserCapabilitiesCodeGenerator.cs
- SchemaConstraints.cs
- BorderGapMaskConverter.cs
- TabItemWrapperAutomationPeer.cs
- SamlAuthenticationClaimResource.cs
- ApplicationTrust.cs
- SecurityRuntime.cs
- DataGridViewComboBoxColumn.cs
- PageTheme.cs
- DataTableMapping.cs
- PreservationFileReader.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- Matrix.cs
- Matrix3D.cs
- DataGridViewImageCell.cs
- Missing.cs
- XmlSchemaAll.cs
- WebSysDefaultValueAttribute.cs
- ItemChangedEventArgs.cs
- PerfCounterSection.cs
- ConvertEvent.cs
- RectAnimationUsingKeyFrames.cs
- Int32EqualityComparer.cs
- DocumentCollection.cs
- DataGridViewRow.cs
- sqlinternaltransaction.cs
- InsufficientMemoryException.cs
- ClaimTypes.cs
- CanonicalFontFamilyReference.cs
- CleanUpVirtualizedItemEventArgs.cs
- XmlAnyElementAttributes.cs
- WrappedDispatcherException.cs
- FilteredDataSetHelper.cs
- StyleBamlRecordReader.cs
- DeferredRunTextReference.cs
- ListBase.cs
- EdmProperty.cs
- ContentDesigner.cs
- ListViewItemCollectionEditor.cs
- TextEditorTyping.cs
- BrowserDefinition.cs
- SimpleBitVector32.cs
- TypeDescriptionProviderAttribute.cs
- StringTraceRecord.cs
- SocketInformation.cs
- BitStack.cs
- CreateParams.cs
- BinaryObjectInfo.cs
- XPathDocumentIterator.cs
- Interop.cs
- NumberAction.cs
- OutputScopeManager.cs
- SafeIUnknown.cs
- EncodingFallbackAwareXmlTextWriter.cs
- XmlSequenceWriter.cs
- JapaneseCalendar.cs
- HtmlLink.cs
- DataGridViewCellStyle.cs
- ConfigXmlCDataSection.cs
- TakeOrSkipWhileQueryOperator.cs
- TextRange.cs
- HwndSourceKeyboardInputSite.cs
- CdpEqualityComparer.cs
- RelationshipEndCollection.cs
- PrincipalPermission.cs
- SemaphoreSecurity.cs
- pingexception.cs
- SplineQuaternionKeyFrame.cs
- _ListenerAsyncResult.cs
- WindowsTab.cs
- SchemaObjectWriter.cs
- IDispatchConstantAttribute.cs
- XAMLParseException.cs
- Pair.cs
- SharedPersonalizationStateInfo.cs
- SEHException.cs
- GestureRecognitionResult.cs
- TokenBasedSet.cs
- CompiledELinqQueryState.cs
- FileDialog.cs
- RawAppCommandInputReport.cs
- ObjectParameter.cs
- UserControl.cs
- SynchronizationFilter.cs
- XmlExpressionDumper.cs
- ServiceNameCollection.cs
- WebBrowserBase.cs
- SmtpNtlmAuthenticationModule.cs
- TypeGeneratedEventArgs.cs
- unsafenativemethodsother.cs
- DelayLoadType.cs
- Model3DGroup.cs
- FileSystemEventArgs.cs
- SmiMetaDataProperty.cs
- Label.cs
- IndexedWhereQueryOperator.cs
- DataGridPreparingCellForEditEventArgs.cs
- CachingHintValidation.cs