Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / ImageSourceConverter.cs / 2 / ImageSourceConverter.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved. // // File: ImageSourceConverter.cs // //----------------------------------------------------------------------------- using System; using System.IO; using System.Security; using System.Security.Permissions; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using MS.Internal; using System.Diagnostics; using System.Windows.Media; using System.Globalization; using System.Runtime.InteropServices; using System.Windows.Markup; using MMCF = System.IO.Packaging; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; using System.Windows.Media.Imaging; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media { #region ImageSourceConverter ////// ImageSourceConverter /// public class ImageSourceConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string) || sourceType == typeof(Stream) || sourceType == typeof(Uri) || sourceType == typeof(byte[])) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for some instances if (context != null && context.Instance != null) { if (!(context.Instance is ImageSource)) { throw new ArgumentException(SR.Get(SRID.General_Expected_Type, "ImageSource"), "context.Instance"); } #pragma warning suppress 6506 // context is obviously not null ImageSource value = (ImageSource)context.Instance; #pragma warning suppress 6506 // value is obviously not null return value.CanSerializeToString(); } return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a ImageSource from the given object. /// ////// The ImageSource which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a ImageSource. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of ImageSource. ////// Critical: Code eventually calls into unsafe code /// TreatAsASafe: Inputs are verified /// [SecurityCritical, SecurityTreatAsSafe] public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } if (((value is string) && (!string.IsNullOrEmpty((string)value))) || (value is Uri)) { UriHolder uriHolder = TypeConverterHelper.GetUriFromUriContext(context, value); return BitmapFrame.CreateFromUriOrStream( uriHolder.BaseUri, uriHolder.OriginalUri, null, BitmapCreateOptions.None, BitmapCacheOption.Default, null ); } else if (value is byte[]) { byte[] bytes = (byte[])value; if (bytes != null) { Stream memStream = null; // // this might be a magical OLE thing, try that first. // memStream = GetBitmapStream(bytes); if (memStream == null) { // // guess not. Try plain memory. // memStream = new MemoryStream(bytes); } return BitmapFrame.Create( memStream, BitmapCreateOptions.None, BitmapCacheOption.Default ); } } else if (value is Stream) { Stream stream = (Stream)value; return BitmapFrame.Create( stream, BitmapCreateOptions.None, BitmapCacheOption.Default ); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of ImageSource to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of ImageSource, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the ImageSource instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is ImageSource) { ImageSource instance = (ImageSource)value; if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for some instances if (context != null && context.Instance != null) { #pragma warning disable 6506 if (!instance.CanSerializeToString()) { throw new NotSupportedException(SR.Get(SRID.Converter_ConvertToNotSupported)); } #pragma warning restore 6506 } // Delegate to the formatting/culture-aware ConvertToString method. return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } /// Try to get a bitmap out of a byte array. This is an ole format that Access uses. /// this fails very quickly so we can try this first without a big perf hit. ////// Critical - does unmanaged memory manipulation /// [SecurityCritical] private unsafe Stream GetBitmapStream(byte[] rawData) { Debug.Assert(rawData != null, "rawData is null."); fixed (byte* pByte = rawData) { IntPtr addr = (IntPtr)pByte; if (addr == IntPtr.Zero) { return null; } // // This will be pHeader.signature, but we try to // do this first so we avoid building the structure when we shouldn't // if (Marshal.ReadInt16(addr) != 0x1c15) { return null; } // // The data is one of these OBJECTHEADER dudes. It's an encoded format that Access uses to push images // into the DB. It's not particularly documented, here is a KB: // // http://support.microsoft.com/default.aspx?scid=KB;EN-US;Q175261 // OBJECTHEADER pHeader = (OBJECTHEADER)Marshal.PtrToStructure(addr, typeof(OBJECTHEADER)); // // "PBrush" should be the 6 chars after position 12 as well. // string strPBrush = System.Text.Encoding.ASCII.GetString(rawData, pHeader.headersize + 12, 6); if (strPBrush != "PBrush") { return null; } // OK, now we can safely trust that we've got a bitmap. byte[] searchArray = System.Text.Encoding.ASCII.GetBytes("BM"); // // Search for "BMP" in the data which is the start of our bitmap data... // // 18 is from (12+6) above. // for (int i = pHeader.headersize + 18; i < pHeader.headersize + 510; i++) { if (searchArray[0] == pByte[i] && searchArray[1] == pByte[i+1]) { // // found the bitmap data. // return new MemoryStream(rawData, i, rawData.Length - i); } } } return null; } // // For pulling encoded IPictures out of Access Databases // [StructLayout(LayoutKind.Sequential)] private struct OBJECTHEADER { public short signature; // this looks like it's always 0x1c15 public short headersize; // how big all this goo ends up being. after this is the actual object data. public short objectType; // we don't care about anything else...they don't seem to be meaningful anyway. public short nameLen; public short classLen; public short nameOffset; public short classOffset; public short width; public short height; public IntPtr pInfo; } } #endregion // ImageSourceConverter } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
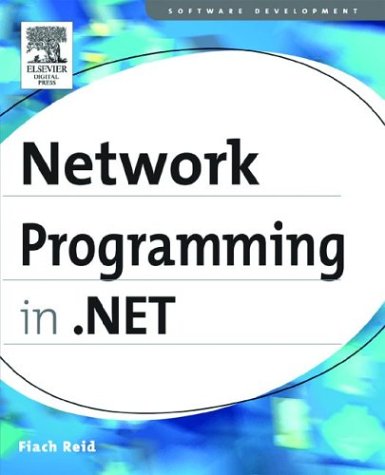
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WindowsTooltip.cs
- FileStream.cs
- SqlPersonalizationProvider.cs
- WindowsProgressbar.cs
- InvalidCastException.cs
- DateTimeOffset.cs
- ServiceMemoryGates.cs
- MissingManifestResourceException.cs
- WebConfigurationHostFileChange.cs
- BasicHttpSecurityElement.cs
- Claim.cs
- SetterBase.cs
- PageTextBox.cs
- PolygonHotSpot.cs
- GridViewRowPresenter.cs
- DiscoveryVersion.cs
- EventMappingSettingsCollection.cs
- ParenthesizePropertyNameAttribute.cs
- InputScopeAttribute.cs
- AssociationProvider.cs
- PathFigureCollection.cs
- DiscoveryCallbackBehavior.cs
- Matrix3DStack.cs
- TreeNode.cs
- WindowsFormsHost.cs
- URL.cs
- NaturalLanguageHyphenator.cs
- MessageQueuePermissionAttribute.cs
- RightsManagementEncryptedStream.cs
- AmbientValueAttribute.cs
- BrowserCapabilitiesFactory35.cs
- ErrorTableItemStyle.cs
- EdgeModeValidation.cs
- TableItemStyle.cs
- RectangleF.cs
- XmlHierarchicalDataSourceView.cs
- HttpGetProtocolReflector.cs
- BrushMappingModeValidation.cs
- SortedList.cs
- AnnotationComponentManager.cs
- BuildProviderAppliesToAttribute.cs
- rsa.cs
- ResourceSetExpression.cs
- HostExecutionContextManager.cs
- ComboBoxAutomationPeer.cs
- MergeExecutor.cs
- LinkUtilities.cs
- DocumentXPathNavigator.cs
- DSACryptoServiceProvider.cs
- PeerMaintainer.cs
- GeneralTransform.cs
- ScrollItemProviderWrapper.cs
- TextContainerHelper.cs
- DataObjectFieldAttribute.cs
- GridViewRowEventArgs.cs
- EventMap.cs
- GenericWebPart.cs
- SoapIncludeAttribute.cs
- TdsParserStaticMethods.cs
- SqlProcedureAttribute.cs
- SmtpClient.cs
- ObjectDataSource.cs
- HostedBindingBehavior.cs
- ConversionHelper.cs
- PersonalizableAttribute.cs
- HostedAspNetEnvironment.cs
- WebZone.cs
- StoragePropertyMapping.cs
- CalendarData.cs
- SharingService.cs
- ExpressionVisitorHelpers.cs
- PeerNameResolver.cs
- KeyConverter.cs
- SqlSelectClauseBuilder.cs
- ObjectQueryState.cs
- ConfigXmlText.cs
- CompilerErrorCollection.cs
- SqlTrackingWorkflowInstance.cs
- DataGridViewTopLeftHeaderCell.cs
- GridViewAutomationPeer.cs
- TextParaClient.cs
- FormViewUpdateEventArgs.cs
- SkewTransform.cs
- CellLabel.cs
- FileRecordSequenceCompletedAsyncResult.cs
- ResourcesChangeInfo.cs
- TableDetailsCollection.cs
- TraceContext.cs
- OutgoingWebResponseContext.cs
- PreviousTrackingServiceAttribute.cs
- ComponentEvent.cs
- TextPointer.cs
- SqlDeflator.cs
- SafeRightsManagementHandle.cs
- DayRenderEvent.cs
- URLIdentityPermission.cs
- ServiceProviders.cs
- XmlDictionaryString.cs
- LinkTarget.cs
- OracleConnectionStringBuilder.cs