Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Web / OutgoingWebResponseContext.cs / 1 / OutgoingWebResponseContext.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- #pragma warning disable 1634, 1691 namespace System.ServiceModel.Web { using System; using System.Globalization; using System.Diagnostics.CodeAnalysis; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.ServiceModel.Dispatcher; using System.Net; using System.Collections.ObjectModel; using System.Collections.Specialized; public class OutgoingWebResponseContext { OperationContext operationContext; internal OutgoingWebResponseContext(OperationContext operationContext) { Fx.Assert(operationContext != null, "operationContext is null"); this.operationContext = operationContext; } public long ContentLength { get { return long.Parse(this.MessageProperty.Headers[HttpResponseHeader.ContentLength], CultureInfo.InvariantCulture); } set { this.MessageProperty.Headers[HttpResponseHeader.ContentLength] = value.ToString(CultureInfo.InvariantCulture); } } public string ContentType { get { return this.MessageProperty.Headers[HttpResponseHeader.ContentType]; } set { this.MessageProperty.Headers[HttpResponseHeader.ContentType] = value; } } public string ETag { get { return this.MessageProperty.Headers[HttpResponseHeader.ETag]; } set { this.MessageProperty.Headers[HttpResponseHeader.ETag] = value; } } public WebHeaderCollection Headers { get { return this.MessageProperty.Headers; } } public DateTime LastModified { get { return DateTime.Parse(this.MessageProperty.Headers[HttpResponseHeader.LastModified], CultureInfo.InvariantCulture); } set { this.MessageProperty.Headers[HttpResponseHeader.LastModified] = (value.Kind == DateTimeKind.Utc ? value.ToString("R", CultureInfo.InvariantCulture) : value.ToUniversalTime().ToString("R", CultureInfo.InvariantCulture)); } } public string Location { get { return this.MessageProperty.Headers[HttpResponseHeader.Location]; } set { this.MessageProperty.Headers[HttpResponseHeader.Location] = value; } } public HttpStatusCode StatusCode { get {return this.MessageProperty.StatusCode; } set {this.MessageProperty.StatusCode = value; } } public string StatusDescription { get {return this.MessageProperty.StatusDescription; } set {this.MessageProperty.StatusDescription = value; } } public bool SuppressEntityBody { get { return this.MessageProperty.SuppressEntityBody; } set { this.MessageProperty.SuppressEntityBody = value; } } HttpResponseMessageProperty MessageProperty { get { if (!operationContext.OutgoingMessageProperties.ContainsKey(HttpResponseMessageProperty.Name)) { operationContext.OutgoingMessageProperties.Add(HttpResponseMessageProperty.Name, new HttpResponseMessageProperty()); } return operationContext.OutgoingMessageProperties[HttpResponseMessageProperty.Name] as HttpResponseMessageProperty; } } public void SetStatusAsCreated(Uri locationUri) { if (locationUri == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("locationUri"); } this.StatusCode = HttpStatusCode.Created; this.Location = locationUri.ToString(); } public void SetStatusAsNotFound() { this.StatusCode = HttpStatusCode.NotFound; } public void SetStatusAsNotFound(string description) { this.StatusCode = HttpStatusCode.NotFound; this.StatusDescription = description; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
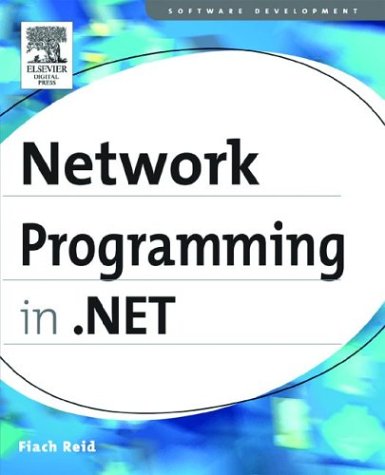
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DoubleKeyFrameCollection.cs
- SqlBulkCopy.cs
- Baml6Assembly.cs
- PackagePart.cs
- LinqDataSourceInsertEventArgs.cs
- Visual3D.cs
- TcpChannelListener.cs
- GZipUtils.cs
- CharacterMetrics.cs
- ThreadStartException.cs
- BitmapDecoder.cs
- XhtmlBasicObjectListAdapter.cs
- SqlDependencyListener.cs
- StringBlob.cs
- LoginCancelEventArgs.cs
- EventWaitHandle.cs
- PostBackTrigger.cs
- DataObjectPastingEventArgs.cs
- HotSpotCollection.cs
- TextRunCacheImp.cs
- SolidBrush.cs
- Dump.cs
- FtpRequestCacheValidator.cs
- HandlerBase.cs
- DiscoveryMessageSequenceGenerator.cs
- WebPartEventArgs.cs
- SqlFactory.cs
- AppDomainManager.cs
- LinqDataSourceEditData.cs
- GridViewSortEventArgs.cs
- FileStream.cs
- XmlFormatWriterGenerator.cs
- SpeechAudioFormatInfo.cs
- ServiceNameElement.cs
- RemoteWebConfigurationHostServer.cs
- RSAPKCS1KeyExchangeFormatter.cs
- NativeWindow.cs
- CompoundFileReference.cs
- NamespaceListProperty.cs
- XmlSubtreeReader.cs
- Baml6ConstructorInfo.cs
- StylusPointProperty.cs
- OperatorExpressions.cs
- Geometry.cs
- MessageSecurityTokenVersion.cs
- RegistrationServices.cs
- TextBoxAutoCompleteSourceConverter.cs
- CompModHelpers.cs
- X509CertificateCollection.cs
- ListItemConverter.cs
- HtmlInputPassword.cs
- UpdateRecord.cs
- COM2PropertyDescriptor.cs
- SignedPkcs7.cs
- SQLMembershipProvider.cs
- BypassElement.cs
- ErrorEventArgs.cs
- CompositeActivityValidator.cs
- SaveFileDialog.cs
- SQLInt64.cs
- LocalValueEnumerator.cs
- DataSourceXmlClassAttribute.cs
- ImageBrush.cs
- GridViewUpdateEventArgs.cs
- SourceItem.cs
- ParserStreamGeometryContext.cs
- SlipBehavior.cs
- Win32KeyboardDevice.cs
- ColorPalette.cs
- MissingManifestResourceException.cs
- Exception.cs
- ListBase.cs
- SpellerError.cs
- ElementsClipboardData.cs
- FileEnumerator.cs
- EventLogPermissionEntry.cs
- ManifestBasedResourceGroveler.cs
- XmlReflectionImporter.cs
- ConfigXmlCDataSection.cs
- BreakRecordTable.cs
- Size.cs
- TrackingProfileSerializer.cs
- AsyncPostBackErrorEventArgs.cs
- ScriptResourceAttribute.cs
- NativeMethods.cs
- KeyTime.cs
- XhtmlBasicTextViewAdapter.cs
- Types.cs
- DrawListViewSubItemEventArgs.cs
- UnregisterInfo.cs
- OleDbConnectionFactory.cs
- XmlUtilWriter.cs
- EncodingNLS.cs
- FontCollection.cs
- MultiPageTextView.cs
- ExpressionEditorSheet.cs
- LoginName.cs
- HtmlInputRadioButton.cs
- SystemUnicastIPAddressInformation.cs
- srgsitem.cs