Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / Effects / Generated / OuterGlowBitmapEffect.cs / 1 / OuterGlowBitmapEffect.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see [....]/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; // These types are aliased to match the unamanaged names used in interop using BOOL = System.UInt32; using WORD = System.UInt16; using Float = System.Single; namespace System.Windows.Media.Effects { sealed partial class OuterGlowBitmapEffect : BitmapEffect { #region Constructors //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Shadows inherited Clone() with a strongly typed /// version for convenience. /// public new OuterGlowBitmapEffect Clone() { return (OuterGlowBitmapEffect)base.Clone(); } ////// Shadows inherited CloneCurrentValue() with a strongly typed /// version for convenience. /// public new OuterGlowBitmapEffect CloneCurrentValue() { return (OuterGlowBitmapEffect)base.CloneCurrentValue(); } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ private static void GlowColorPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(GlowColorProperty); } private static void GlowSizePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(GlowSizeProperty); } private static void NoisePropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(NoiseProperty); } private static void OpacityPropertyChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { OuterGlowBitmapEffect target = ((OuterGlowBitmapEffect) d); target.PropertyChanged(OpacityProperty); } #region Public Properties ////// GlowColor - Color. Default value is Colors.Gold. /// public Color GlowColor { get { return (Color) GetValue(GlowColorProperty); } set { SetValueInternal(GlowColorProperty, value); } } ////// GlowSize - double. Default value is 5.0. /// public double GlowSize { get { return (double) GetValue(GlowSizeProperty); } set { SetValueInternal(GlowSizeProperty, value); } } ////// Noise - double. Default value is 0.0. /// public double Noise { get { return (double) GetValue(NoiseProperty); } set { SetValueInternal(NoiseProperty, value); } } ////// Opacity - double. Default value is 1.0. /// public double Opacity { get { return (double) GetValue(OpacityProperty); } set { SetValueInternal(OpacityProperty, value); } } #endregion Public Properties //----------------------------------------------------- // // Protected Methods // //------------------------------------------------------ #region Protected Methods ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new OuterGlowBitmapEffect(); } #endregion ProtectedMethods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties #endregion Internal Properties //----------------------------------------------------- // // Dependency Properties // //------------------------------------------------------ #region Dependency Properties ////// The DependencyProperty for the OuterGlowBitmapEffect.GlowColor property. /// public static readonly DependencyProperty GlowColorProperty = RegisterProperty("GlowColor", typeof(Color), typeof(OuterGlowBitmapEffect), Colors.Gold, new PropertyChangedCallback(GlowColorPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the OuterGlowBitmapEffect.GlowSize property. /// public static readonly DependencyProperty GlowSizeProperty = RegisterProperty("GlowSize", typeof(double), typeof(OuterGlowBitmapEffect), 5.0, new PropertyChangedCallback(GlowSizePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the OuterGlowBitmapEffect.Noise property. /// public static readonly DependencyProperty NoiseProperty = RegisterProperty("Noise", typeof(double), typeof(OuterGlowBitmapEffect), 0.0, new PropertyChangedCallback(NoisePropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); ////// The DependencyProperty for the OuterGlowBitmapEffect.Opacity property. /// public static readonly DependencyProperty OpacityProperty = RegisterProperty("Opacity", typeof(double), typeof(OuterGlowBitmapEffect), 1.0, new PropertyChangedCallback(OpacityPropertyChanged), null, /* isIndependentlyAnimated = */ true, /* coerceValueCallback */ null); #endregion Dependency Properties //------------------------------------------------------ // // Internal Fields // //----------------------------------------------------- #region Internal Fields internal static Color s_GlowColor = Colors.Gold; internal const double c_GlowSize = 5.0; internal const double c_Noise = 0.0; internal const double c_Opacity = 1.0; #endregion Internal Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
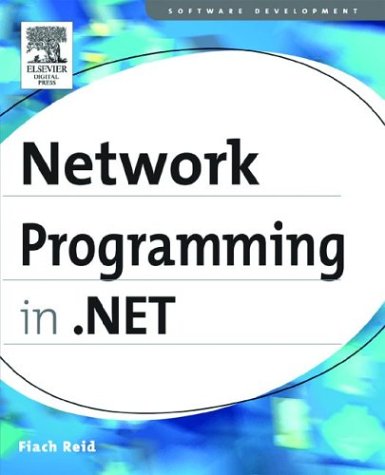
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServiceProviders.cs
- clipboard.cs
- ControlTemplate.cs
- ImageSourceValueSerializer.cs
- HistoryEventArgs.cs
- updateconfighost.cs
- DuplicateWaitObjectException.cs
- OutputScopeManager.cs
- VisualTreeHelper.cs
- ReaderWriterLockSlim.cs
- mactripleDES.cs
- RegistrySecurity.cs
- Animatable.cs
- DocumentsTrace.cs
- CutCopyPasteHelper.cs
- TableCellAutomationPeer.cs
- Authorization.cs
- BinaryObjectReader.cs
- BitmapEffect.cs
- WindowsImpersonationContext.cs
- OutputScopeManager.cs
- PropertyCollection.cs
- TextShapeableCharacters.cs
- OleDbFactory.cs
- EntityDataSourceQueryBuilder.cs
- SettingsBindableAttribute.cs
- SqlUDTStorage.cs
- PeerContact.cs
- DecimalStorage.cs
- RectAnimationUsingKeyFrames.cs
- DelayedRegex.cs
- BrowsableAttribute.cs
- TextCharacters.cs
- QilTernary.cs
- X509ChainPolicy.cs
- UniqueConstraint.cs
- StyleHelper.cs
- RepeaterCommandEventArgs.cs
- UrlAuthFailedErrorFormatter.cs
- SupportingTokenBindingElement.cs
- Single.cs
- FixedFlowMap.cs
- FileNotFoundException.cs
- PropertyRecord.cs
- rsa.cs
- Point3DAnimation.cs
- CodeTypeReferenceSerializer.cs
- HttpConfigurationContext.cs
- TableItemProviderWrapper.cs
- CategoryValueConverter.cs
- StartUpEventArgs.cs
- ExpressionVisitor.cs
- ConnectionStringsExpressionBuilder.cs
- MailDefinition.cs
- ComboBoxAutomationPeer.cs
- Message.cs
- UInt64.cs
- DataGridViewAutoSizeModeEventArgs.cs
- MILUtilities.cs
- XPathNodeList.cs
- BitmapEffectGroup.cs
- HwndKeyboardInputProvider.cs
- ServiceDurableInstance.cs
- AstTree.cs
- ObfuscationAttribute.cs
- BindingNavigator.cs
- Encoder.cs
- HeaderedContentControl.cs
- FlagsAttribute.cs
- TableLayoutSettingsTypeConverter.cs
- HtmlTableCellCollection.cs
- UriExt.cs
- ToolboxItemSnapLineBehavior.cs
- XmlElementAttribute.cs
- BaseParser.cs
- WebControlsSection.cs
- SettingsPropertyNotFoundException.cs
- XmlParserContext.cs
- MsmqOutputMessage.cs
- WindowsRegion.cs
- TextFormatterImp.cs
- SymbolDocumentGenerator.cs
- ImageClickEventArgs.cs
- SoapAttributeAttribute.cs
- FrameworkElementAutomationPeer.cs
- DataSvcMapFileSerializer.cs
- SubstitutionDesigner.cs
- AsyncMethodInvoker.cs
- ConfigXmlSignificantWhitespace.cs
- Scanner.cs
- SecUtil.cs
- SQLRoleProvider.cs
- WindowsFormsLinkLabel.cs
- TemplateInstanceAttribute.cs
- IResourceProvider.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- RegexRunnerFactory.cs
- DataGridViewRowStateChangedEventArgs.cs
- LowerCaseStringConverter.cs
- PatternMatcher.cs