Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Xml / System / Xml / Dom / XPathNodeList.cs / 1305376 / XPathNodeList.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml { using System.Xml.XPath; using System.Diagnostics; using System.Collections; using System.Collections.Generic; internal class XPathNodeList: XmlNodeList { Listlist; XPathNodeIterator nodeIterator; bool done; public XPathNodeList(XPathNodeIterator nodeIterator) { this.nodeIterator = nodeIterator; this.list = new List (); this.done = false; } public override int Count { get { if (! done) { ReadUntil(Int32.MaxValue); } return list.Count; } } private static readonly object[] nullparams = {}; private XmlNode GetNode(XPathNavigator n) { IHasXmlNode iHasNode = (IHasXmlNode) n; return iHasNode.GetNode(); } internal int ReadUntil(int index) { int count = list.Count; while (! done && count <= index) { if (nodeIterator.MoveNext()) { XmlNode n = GetNode(nodeIterator.Current); if (n != null) { list.Add(n); count++; } } else { done = true; break; } } return count; } public override XmlNode Item(int index) { if (list.Count <= index) { ReadUntil(index); } if (index < 0 || list.Count <= index) { return null; } return list[index]; } public override IEnumerator GetEnumerator() { return new XmlNodeListEnumerator(this); } } internal class XmlNodeListEnumerator : IEnumerator { XPathNodeList list; int index; bool valid; public XmlNodeListEnumerator(XPathNodeList list) { this.list = list; this.index = -1; this.valid = false; } public void Reset() { index = -1; } public bool MoveNext() { index++; int count = list.ReadUntil(index + 1); // read past for delete-node case if (count - 1 < index) { return false; } valid = (list[index] != null); return valid; } public object Current { get { if (valid) { return list[index]; } return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
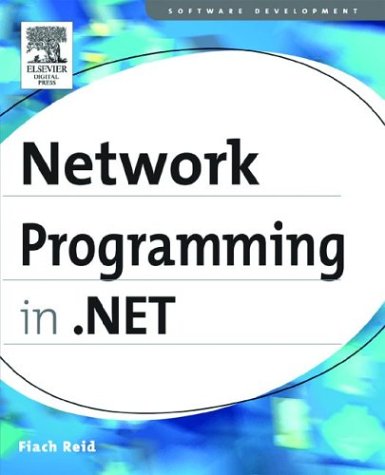
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateControlCodeDomTreeGenerator.cs
- ObjectDataProvider.cs
- CharConverter.cs
- GZipStream.cs
- XmlSerializerObjectSerializer.cs
- IxmlLineInfo.cs
- Trace.cs
- ImageIndexConverter.cs
- SystemUnicastIPAddressInformation.cs
- StringSource.cs
- HtmlFormWrapper.cs
- ExpandCollapseProviderWrapper.cs
- Accessors.cs
- WorkflowInlining.cs
- KnownAssembliesSet.cs
- ConsumerConnectionPoint.cs
- NetMsmqSecurityMode.cs
- HandleValueEditor.cs
- EnterpriseServicesHelper.cs
- ToolBarButtonDesigner.cs
- QilXmlReader.cs
- ContentOperations.cs
- VirtualPathExtension.cs
- DataKey.cs
- CellNormalizer.cs
- _SingleItemRequestCache.cs
- ErasingStroke.cs
- TogglePatternIdentifiers.cs
- _SslSessionsCache.cs
- RtfControlWordInfo.cs
- ZipIOExtraField.cs
- Parser.cs
- CheckBox.cs
- SqlDataSourceCustomCommandPanel.cs
- RegexMatchCollection.cs
- DynamicValidator.cs
- DataSource.cs
- ImageField.cs
- AnonymousIdentificationModule.cs
- NumericPagerField.cs
- ProxyManager.cs
- MemberMemberBinding.cs
- ValidationSummary.cs
- AppDomainUnloadedException.cs
- WorkflowInstance.cs
- RightsManagementLicense.cs
- ExceptionUtil.cs
- Point3D.cs
- Process.cs
- TextWriter.cs
- ClientEventManager.cs
- MouseWheelEventArgs.cs
- ArrayTypeMismatchException.cs
- SocketInformation.cs
- InheritablePropertyChangeInfo.cs
- Point4D.cs
- VisualProxy.cs
- PtsCache.cs
- Base64Stream.cs
- LogicalExpr.cs
- ServiceContractViewControl.Designer.cs
- UrlMappingsSection.cs
- CodeAttributeArgumentCollection.cs
- X509CertificateChain.cs
- SelectedDatesCollection.cs
- Container.cs
- DataBindingCollectionEditor.cs
- DataGridLinkButton.cs
- MimeParameters.cs
- OdbcConnectionOpen.cs
- PropertyMapper.cs
- DataGridrowEditEndingEventArgs.cs
- XmlParserContext.cs
- XsltInput.cs
- DependencyStoreSurrogate.cs
- XXXInfos.cs
- MembershipSection.cs
- MemberListBinding.cs
- TextServicesDisplayAttribute.cs
- TrackBar.cs
- WebControl.cs
- CapabilitiesRule.cs
- WsatConfiguration.cs
- ToolboxItemImageConverter.cs
- ColumnCollection.cs
- WebReference.cs
- ColorInterpolationModeValidation.cs
- SizeIndependentAnimationStorage.cs
- X509AsymmetricSecurityKey.cs
- WebHttpEndpoint.cs
- VectorCollectionConverter.cs
- CachedRequestParams.cs
- XmlExpressionDumper.cs
- PreProcessor.cs
- InputBuffer.cs
- ClientScriptManager.cs
- ResourceReferenceExpressionConverter.cs
- UnitControl.cs
- OdbcRowUpdatingEvent.cs
- InternalMappingException.cs