Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / Util / UnitControl.cs / 1 / UnitControl.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // UnitControl.cs // // 12/22/98: Created: [....] // namespace System.Web.UI.Design.Util { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System.Design; using System.Globalization; using System; using System.ComponentModel; using System.Windows.Forms; using System.Drawing; using Microsoft.Win32; ////// /// UnitControl /// Provides a UI to edit a unit, i.e., its value and type. /// Additionally allows placing restrictions on the types of units that /// that can be entered. /// ///[System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode)] internal sealed class UnitControl : Panel { /////////////////////////////////////////////////////////////////////////// // Constants // UI Layout constants private const int EDIT_X_SIZE = 44; private const int COMBO_X_SIZE = 40; private const int SEPARATOR_X_SIZE = 4; private const int CTL_Y_SIZE = 21; // Units public const int UNIT_PX = 0; public const int UNIT_PT = 1; public const int UNIT_PC = 2; public const int UNIT_MM = 3; public const int UNIT_CM = 4; public const int UNIT_IN = 5; public const int UNIT_EM = 6; public const int UNIT_EX = 7; public const int UNIT_PERCENT = 8; public const int UNIT_NONE = 9; private static readonly string[] UNIT_VALUES = new string[] { "px", "pt", "pc", "mm", "cm", "in", "em", "ex", "%", "" }; /////////////////////////////////////////////////////////////////////////// // Members private NumberEdit valueEdit; private ComboBox unitCombo; private bool allowPercent = true; private bool allowNonUnit = false; private int defaultUnit = UNIT_PT; private int minValue = 0; private int maxValue = 0xFFFF; private bool validateMinMax = false; private EventHandler onChangedHandler = null; private bool initMode = false; private bool internalChange = false; private bool valueChanged = false; /////////////////////////////////////////////////////////////////////////// // Constructor /// /// /// Createa a new UnitControl. /// public UnitControl() { initMode = true; Size = new Size((EDIT_X_SIZE + COMBO_X_SIZE + SEPARATOR_X_SIZE), CTL_Y_SIZE); InitControl(); InitUI(); initMode = false; } /////////////////////////////////////////////////////////////////////////// // Properties ////// /// Controls whether the unit value can be negative /// public bool AllowNegativeValues { get { return valueEdit.AllowNegative; } set { valueEdit.AllowNegative = value; } } ////// /// Controls whether the unit value can be a unit-less /// public bool AllowNonUnitValues { get { return allowNonUnit; } set { if (value == allowNonUnit) return; if (value && !allowPercent) { Debug.Fail("AllowPercentValues must be set to true first"); throw new Exception(); } allowNonUnit = value; if (allowNonUnit) unitCombo.Items.Add(UNIT_VALUES[UNIT_NONE]); else unitCombo.Items.Remove(UNIT_VALUES[UNIT_NONE]); } } ////// /// Controls whether the unit value can be a percent value /// public bool AllowPercentValues { get { return allowPercent; } set { if (value == allowPercent) return; if (!value && allowNonUnit) { Debug.Fail("AllowNonUnitValues must be set to false first"); throw new Exception(); } allowPercent = value; if (allowPercent) unitCombo.Items.Add(UNIT_VALUES[UNIT_PERCENT]); else unitCombo.Items.Remove(UNIT_VALUES[UNIT_PERCENT]); } } ////// /// The default unit to be used /// public int DefaultUnit { get { return defaultUnit; } set { Debug.Assert((value >= UNIT_PX) && (value <= UNIT_NONE) && (allowNonUnit || (value != UNIT_NONE)) || (allowPercent || (value != UNIT_PERCENT)), "Invalid default unit"); defaultUnit = value; } } public int MaxValue { get { return maxValue; } set { maxValue = value; } } public int MinValue { get { return minValue; } set { minValue = value; } } protected override void OnEnabledChanged(EventArgs e) { base.OnEnabledChanged(e); valueEdit.Enabled = Enabled; unitCombo.Enabled = Enabled; } public bool ValidateMinMax { get { return validateMinMax; } set { validateMinMax = value; } } ////// /// The unit reflecting the value and unit type within the UI. /// Returns null if no unit is selected. /// public string Value { get { string value = GetValidatedValue(); if (value == null) { value = valueEdit.Text; } else { valueEdit.Text = value; OnValueTextChanged(valueEdit, EventArgs.Empty); } int unit = unitCombo.SelectedIndex; if ((value.Length == 0) || (unit == -1)) return null; return value + UNIT_VALUES[unit]; } set { initMode = true; InitUI(); if (value != null) { string temp = value.Trim().ToLower(CultureInfo.InvariantCulture); int len = temp.Length; int unit = -1; int iLastDigit = -1; char ch; // find the end of the number part for (int i = 0; i < len; i++) { ch = temp[i]; if (!(((ch >= '0') && (ch <= '9')) || (NumberFormatInfo.CurrentInfo.NumberDecimalSeparator.Contains(ch.ToString(CultureInfo.CurrentCulture))) || (NumberFormatInfo.CurrentInfo.NegativeSign.Contains(ch.ToString(CultureInfo.CurrentCulture))) && (valueEdit.AllowNegative))) break; else iLastDigit = i; } if (iLastDigit != -1) { // detect the type of unit if ((iLastDigit + 1) < len) { int maxUnit = allowPercent ? UNIT_PERCENT : UNIT_EX; string unitString = temp.Substring(iLastDigit+1); for (int i = 0; i <= maxUnit; i++) { if (UNIT_VALUES[i].Equals(unitString)) { unit = i; break; } } } else if (allowNonUnit) unit = UNIT_NONE; if (unit != -1) { valueEdit.Text = temp.Substring(0, iLastDigit+1); unitCombo.SelectedIndex = unit; } } } initMode = false; } } public string UnitAccessibleName { get { if (unitCombo != null) { return unitCombo.AccessibleName; } return String.Empty; } set { if (unitCombo != null) { unitCombo.AccessibleName = value; } } } public string UnitAccessibleDescription { get { if (unitCombo != null) { return unitCombo.AccessibleDescription; } return String.Empty; } set { if (unitCombo != null) { unitCombo.AccessibleDescription = value; } } } public string ValueAccessibleName { get { if (valueEdit != null) { return valueEdit.AccessibleName; } return String.Empty; } set { if (valueEdit != null) { valueEdit.AccessibleName = value; } } } public string ValueAccessibleDescription { get { if (valueEdit != null) { return valueEdit.AccessibleDescription; } return String.Empty; } set { if (valueEdit != null) { valueEdit.AccessibleDescription = value; } } } /////////////////////////////////////////////////////////////////////////// // Events public event EventHandler Changed { add { onChangedHandler += value; } remove { onChangedHandler -= value; } } /////////////////////////////////////////////////////////////////////////// // Implementation ////// /// private string GetValidatedValue() { string validValue = null; if (validateMinMax) { string value = valueEdit.Text; if (value.Length != 0) { try { if (!value.Contains(NumberFormatInfo.CurrentInfo.NumberDecimalSeparator)) { int valueNum = Int32.Parse(value, CultureInfo.CurrentCulture); if (valueNum < minValue) validValue = minValue.ToString(NumberFormatInfo.CurrentInfo); else if (valueNum > maxValue) validValue = maxValue.ToString(NumberFormatInfo.CurrentInfo); } else { float valueNum = Single.Parse(value, CultureInfo.CurrentCulture); if (valueNum < (float)minValue) validValue = minValue.ToString(NumberFormatInfo.CurrentInfo); else if (valueNum > (float)maxValue) validValue = maxValue.ToString(NumberFormatInfo.CurrentInfo); } } catch { validValue = maxValue.ToString(NumberFormatInfo.CurrentInfo); } } } return validValue; } ////// /// Create the contained controls and initialize their settings /// private void InitControl() { int editWidth = this.Width - (COMBO_X_SIZE + SEPARATOR_X_SIZE); if (editWidth < 0) editWidth = 0; valueEdit = new NumberEdit(); valueEdit.Location = new Point(0, 0); valueEdit.Size = new Size(editWidth, CTL_Y_SIZE); valueEdit.TabIndex = 0; valueEdit.MaxLength = 10; valueEdit.TextChanged += new EventHandler(this.OnValueTextChanged); valueEdit.LostFocus += new EventHandler(this.OnValueLostFocus); unitCombo = new ComboBox(); unitCombo.DropDownStyle = ComboBoxStyle.DropDownList; unitCombo.Location = new Point(editWidth + SEPARATOR_X_SIZE, 0); unitCombo.Size = new Size(COMBO_X_SIZE, CTL_Y_SIZE); unitCombo.TabIndex = 1; unitCombo.MaxDropDownItems = 9; unitCombo.SelectedIndexChanged += new EventHandler(this.OnUnitSelectedIndexChanged); this.Controls.Clear(); this.Controls.AddRange(new Control[] { unitCombo, valueEdit}); for (int i = UNIT_PX; i <= UNIT_EX; i++) unitCombo.Items.Add(UNIT_VALUES[i]); if (allowPercent) unitCombo.Items.Add(UNIT_VALUES[UNIT_PERCENT]); if (allowNonUnit) unitCombo.Items.Add(UNIT_VALUES[UNIT_NONE]); } ////// /// Initialize the controls to their default state /// private void InitUI() { valueEdit.Text = String.Empty; unitCombo.SelectedIndex = -1; } ////// /// Fires the "changed" event. /// private void OnChanged(EventArgs e) { if (onChangedHandler != null) onChangedHandler(this, e); } /////////////////////////////////////////////////////////////////////////// // Event Handlers protected override void OnGotFocus(EventArgs e) { valueEdit.Focus(); } ////// /// Handles changes in the value edit control. /// If there is no unit selected, and a value is entered, the default unit is /// selected. If the value is cleared, the unit is also deselected. /// private void OnValueTextChanged(object source, EventArgs e) { if (initMode) return; string text = valueEdit.Text; if (text.Length == 0) { internalChange = true; unitCombo.SelectedIndex = -1; internalChange = false; } else { if (unitCombo.SelectedIndex == -1) { internalChange = true; unitCombo.SelectedIndex = defaultUnit; internalChange = false; } } valueChanged = true; OnChanged(null); } private void OnValueLostFocus(object source, EventArgs e) { if (valueChanged) { string value = GetValidatedValue(); if (value != null) { valueEdit.Text = value; OnValueTextChanged(valueEdit, EventArgs.Empty); } valueChanged = false; OnChanged(EventArgs.Empty); } } ////// /// Handles the event when the unit combobox selection changes. /// Fires a changed event. /// private void OnUnitSelectedIndexChanged(object source, EventArgs e) { if (initMode || internalChange) return; OnChanged(EventArgs.Empty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
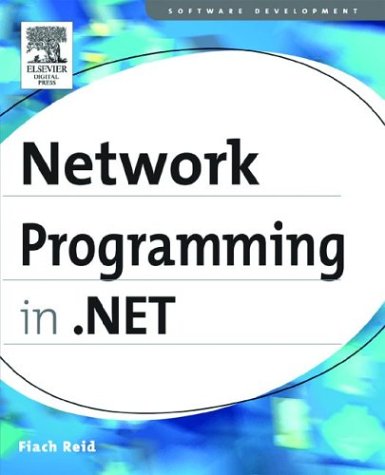
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AddInSegmentDirectoryNotFoundException.cs
- FrameworkObject.cs
- ThrowOnMultipleAssignment.cs
- PackWebRequestFactory.cs
- AnnotationAuthorChangedEventArgs.cs
- OleDbStruct.cs
- Vector3DCollection.cs
- Mapping.cs
- CacheEntry.cs
- TagMapInfo.cs
- SqlClientMetaDataCollectionNames.cs
- StylusShape.cs
- GridViewRowPresenterBase.cs
- SimpleBitVector32.cs
- CodeGroup.cs
- SerializationStore.cs
- TimerEventSubscriptionCollection.cs
- XamlSerializerUtil.cs
- CompilerHelpers.cs
- ComboBox.cs
- ScrollChrome.cs
- HostTimeoutsElement.cs
- AsymmetricSignatureDeformatter.cs
- UdpDuplexChannel.cs
- ExeContext.cs
- VirtualizingStackPanel.cs
- Dispatcher.cs
- SafeNativeMethods.cs
- XmlTypeMapping.cs
- FigureParagraph.cs
- DataMemberFieldConverter.cs
- Domain.cs
- DataGridViewCheckBoxColumn.cs
- ImageFormatConverter.cs
- AsyncPostBackTrigger.cs
- AttachedPropertyBrowsableForTypeAttribute.cs
- RowToFieldTransformer.cs
- ObjectStateEntry.cs
- DefaultWorkflowSchedulerService.cs
- InvalidFilterCriteriaException.cs
- BlockCollection.cs
- TemplatePagerField.cs
- WebPartCloseVerb.cs
- HMACMD5.cs
- SemaphoreFullException.cs
- NameTable.cs
- CreateUserWizardStep.cs
- HelpKeywordAttribute.cs
- MultilineStringConverter.cs
- Wizard.cs
- RectangleHotSpot.cs
- DbConnectionPoolCounters.cs
- MsmqInputChannelBase.cs
- Context.cs
- DispatcherExceptionFilterEventArgs.cs
- WorkflowValidationFailedException.cs
- FontEditor.cs
- IPGlobalProperties.cs
- EntityCommandCompilationException.cs
- RectKeyFrameCollection.cs
- FixedSchema.cs
- Substitution.cs
- TextDecorationLocationValidation.cs
- DoubleCollection.cs
- TextTreeTextBlock.cs
- WinFormsSecurity.cs
- DataMember.cs
- LogConverter.cs
- IconHelper.cs
- DelegateSerializationHolder.cs
- CellLabel.cs
- DbException.cs
- EventRecord.cs
- ChannelManager.cs
- DiscoveryReferences.cs
- SystemColors.cs
- TextElementEnumerator.cs
- LinqDataSourceStatusEventArgs.cs
- InstanceDataCollectionCollection.cs
- TypeSystem.cs
- OutputCacheSection.cs
- EntityKey.cs
- EndpointBehaviorElement.cs
- XmlDocumentType.cs
- MachineKeySection.cs
- DesignTimeTemplateParser.cs
- EventLogWatcher.cs
- Base64Encoding.cs
- HashAlgorithm.cs
- ComponentCommands.cs
- WinEventWrap.cs
- Utilities.cs
- IndexedDataBuffer.cs
- Converter.cs
- TextSelectionHighlightLayer.cs
- TitleStyle.cs
- ProcessHostMapPath.cs
- SerialPort.cs
- ActivityCodeGenerator.cs
- Function.cs