Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / textformatting / TextCharacters.cs / 1305600 / TextCharacters.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2004 // // File: TextCharacters.cs // // Contents: Implementation of text symbol for characters // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 1-2-2004 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.Collections; using System.Collections.Generic; using System.Security; using System.Windows; using MS.Internal; using MS.Internal.Shaping; using MS.Internal.TextFormatting; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { ////// A specialized TextSymbols implemented by TextFormatter to produces /// a collection of TextCharacterShape – each represents a collection of /// character glyphs from distinct physical typeface. /// public class TextCharacters : TextRun, ITextSymbols, IShapeableTextCollector { private CharacterBufferReference _characterBufferReference; private int _length; private TextRunProperties _textRunProperties; #region Constructors ////// Construct a run of text content from character array /// public TextCharacters( char[] characterArray, int offsetToFirstChar, int length, TextRunProperties textRunProperties ) : this( new CharacterBufferReference(characterArray, offsetToFirstChar), length, textRunProperties ) {} ////// Construct a run for text content from string /// public TextCharacters( string characterString, TextRunProperties textRunProperties ) : this( characterString, 0, // offserToFirstChar (characterString == null) ? 0 : characterString.Length, textRunProperties ) {} ////// Construct a run for text content from string /// public TextCharacters( string characterString, int offsetToFirstChar, int length, TextRunProperties textRunProperties ) : this( new CharacterBufferReference(characterString, offsetToFirstChar), length, textRunProperties ) {} ////// Construct a run for text content from unsafe character string /// ////// Critical: This manipulates unsafe pointers and calls into the critical CharacterBufferReference ctor. /// PublicOK: The caller needs unmanaged code permission in order to pass unsafe pointers to us. /// [SecurityCritical] [CLSCompliant(false)] public unsafe TextCharacters( char* unsafeCharacterString, int length, TextRunProperties textRunProperties ) : this( new CharacterBufferReference(unsafeCharacterString, length), length, textRunProperties ) {} ////// Internal constructor of TextContent /// private TextCharacters( CharacterBufferReference characterBufferReference, int length, TextRunProperties textRunProperties ) { if (length <= 0) { throw new ArgumentOutOfRangeException("length", SR.Get(SRID.ParameterMustBeGreaterThanZero)); } if (textRunProperties == null) { throw new ArgumentNullException("textRunProperties"); } if (textRunProperties.Typeface == null) { throw new ArgumentNullException("textRunProperties.Typeface"); } if (textRunProperties.CultureInfo == null) { throw new ArgumentNullException("textRunProperties.CultureInfo"); } if (textRunProperties.FontRenderingEmSize <= 0) { throw new ArgumentOutOfRangeException("textRunProperties.FontRenderingEmSize", SR.Get(SRID.ParameterMustBeGreaterThanZero)); } _characterBufferReference = characterBufferReference; _length = length; _textRunProperties = textRunProperties; } #endregion #region TextRun implementation ////// Character buffer /// public sealed override CharacterBufferReference CharacterBufferReference { get { return _characterBufferReference; } } ////// Character length of the run /// ///public sealed override int Length { get { return _length; } } /// /// A set of properties shared by every characters in the run /// public sealed override TextRunProperties Properties { get { return _textRunProperties; } } #endregion #region ITextSymbols implementation ////// Break a run of text into individually shape items. /// Shape items are delimited by /// Change of writing system /// Change of glyph typeface /// IListITextSymbols.GetTextShapeableSymbols( GlyphingCache glyphingCache, CharacterBufferReference characterBufferReference, int length, bool rightToLeft, bool isRightToLeftParagraph, CultureInfo digitCulture, TextModifierScope textModifierScope, TextFormattingMode textFormattingMode, bool isSideways ) { if (characterBufferReference.CharacterBuffer == null) { throw new ArgumentNullException("characterBufferReference.CharacterBuffer"); } int offsetToFirstChar = characterBufferReference.OffsetToFirstChar - _characterBufferReference.OffsetToFirstChar; Debug.Assert(characterBufferReference.CharacterBuffer == _characterBufferReference.CharacterBuffer); Debug.Assert(offsetToFirstChar >= 0 && offsetToFirstChar < _length); if ( length < 0 || offsetToFirstChar + length > _length) { length = _length - offsetToFirstChar; } // Get the actual text run properties in effect, after invoking any // text modifiers that may be in scope. TextRunProperties textRunProperties = _textRunProperties; if (textModifierScope != null) { textRunProperties = textModifierScope.ModifyProperties(textRunProperties); } if (!rightToLeft) { // Fast loop early out for run with all non-complex characters // which can be optimized by not going thru shaping engine. int nominalLength; if (textRunProperties.Typeface.CheckFastPathNominalGlyphs( new CharacterBufferRange(characterBufferReference, length), textRunProperties.FontRenderingEmSize, 1.0, double.MaxValue, // widthMax true, // keepAWord digitCulture != null, CultureMapper.GetSpecificCulture(textRunProperties.CultureInfo), textFormattingMode, isSideways, out nominalLength ) && length == nominalLength) { return new TextShapeableCharacters[] { new TextShapeableCharacters( new CharacterBufferRange(characterBufferReference, nominalLength), textRunProperties, textRunProperties.FontRenderingEmSize, new MS.Internal.Text.TextInterface.ItemProps(), null, // shapeTypeface (no shaping required) false, // nullShape, textFormattingMode, isSideways ) }; } } IList shapeables = new List (2); glyphingCache.GetShapeableText( textRunProperties.Typeface, characterBufferReference, length, textRunProperties, digitCulture, isRightToLeftParagraph, shapeables, this as IShapeableTextCollector, textFormattingMode ); return shapeables; } #endregion #region IShapeableTextCollector implementation /// /// Add shapeable text object to the list /// void IShapeableTextCollector.Add( IListshapeables, CharacterBufferRange characterBufferRange, TextRunProperties textRunProperties, MS.Internal.Text.TextInterface.ItemProps textItem, ShapeTypeface shapeTypeface, double emScale, bool nullShape, TextFormattingMode textFormattingMode ) { Debug.Assert(shapeables != null); shapeables.Add( new TextShapeableCharacters( characterBufferRange, textRunProperties, textRunProperties.FontRenderingEmSize * emScale, textItem, shapeTypeface, nullShape, textFormattingMode, false ) ); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2004 // // File: TextCharacters.cs // // Contents: Implementation of text symbol for characters // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 1-2-2004 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Globalization; using System.Collections; using System.Collections.Generic; using System.Security; using System.Windows; using MS.Internal; using MS.Internal.Shaping; using MS.Internal.TextFormatting; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.TextFormatting { /// /// A specialized TextSymbols implemented by TextFormatter to produces /// a collection of TextCharacterShape – each represents a collection of /// character glyphs from distinct physical typeface. /// public class TextCharacters : TextRun, ITextSymbols, IShapeableTextCollector { private CharacterBufferReference _characterBufferReference; private int _length; private TextRunProperties _textRunProperties; #region Constructors ////// Construct a run of text content from character array /// public TextCharacters( char[] characterArray, int offsetToFirstChar, int length, TextRunProperties textRunProperties ) : this( new CharacterBufferReference(characterArray, offsetToFirstChar), length, textRunProperties ) {} ////// Construct a run for text content from string /// public TextCharacters( string characterString, TextRunProperties textRunProperties ) : this( characterString, 0, // offserToFirstChar (characterString == null) ? 0 : characterString.Length, textRunProperties ) {} ////// Construct a run for text content from string /// public TextCharacters( string characterString, int offsetToFirstChar, int length, TextRunProperties textRunProperties ) : this( new CharacterBufferReference(characterString, offsetToFirstChar), length, textRunProperties ) {} ////// Construct a run for text content from unsafe character string /// ////// Critical: This manipulates unsafe pointers and calls into the critical CharacterBufferReference ctor. /// PublicOK: The caller needs unmanaged code permission in order to pass unsafe pointers to us. /// [SecurityCritical] [CLSCompliant(false)] public unsafe TextCharacters( char* unsafeCharacterString, int length, TextRunProperties textRunProperties ) : this( new CharacterBufferReference(unsafeCharacterString, length), length, textRunProperties ) {} ////// Internal constructor of TextContent /// private TextCharacters( CharacterBufferReference characterBufferReference, int length, TextRunProperties textRunProperties ) { if (length <= 0) { throw new ArgumentOutOfRangeException("length", SR.Get(SRID.ParameterMustBeGreaterThanZero)); } if (textRunProperties == null) { throw new ArgumentNullException("textRunProperties"); } if (textRunProperties.Typeface == null) { throw new ArgumentNullException("textRunProperties.Typeface"); } if (textRunProperties.CultureInfo == null) { throw new ArgumentNullException("textRunProperties.CultureInfo"); } if (textRunProperties.FontRenderingEmSize <= 0) { throw new ArgumentOutOfRangeException("textRunProperties.FontRenderingEmSize", SR.Get(SRID.ParameterMustBeGreaterThanZero)); } _characterBufferReference = characterBufferReference; _length = length; _textRunProperties = textRunProperties; } #endregion #region TextRun implementation ////// Character buffer /// public sealed override CharacterBufferReference CharacterBufferReference { get { return _characterBufferReference; } } ////// Character length of the run /// ///public sealed override int Length { get { return _length; } } /// /// A set of properties shared by every characters in the run /// public sealed override TextRunProperties Properties { get { return _textRunProperties; } } #endregion #region ITextSymbols implementation ////// Break a run of text into individually shape items. /// Shape items are delimited by /// Change of writing system /// Change of glyph typeface /// IListITextSymbols.GetTextShapeableSymbols( GlyphingCache glyphingCache, CharacterBufferReference characterBufferReference, int length, bool rightToLeft, bool isRightToLeftParagraph, CultureInfo digitCulture, TextModifierScope textModifierScope, TextFormattingMode textFormattingMode, bool isSideways ) { if (characterBufferReference.CharacterBuffer == null) { throw new ArgumentNullException("characterBufferReference.CharacterBuffer"); } int offsetToFirstChar = characterBufferReference.OffsetToFirstChar - _characterBufferReference.OffsetToFirstChar; Debug.Assert(characterBufferReference.CharacterBuffer == _characterBufferReference.CharacterBuffer); Debug.Assert(offsetToFirstChar >= 0 && offsetToFirstChar < _length); if ( length < 0 || offsetToFirstChar + length > _length) { length = _length - offsetToFirstChar; } // Get the actual text run properties in effect, after invoking any // text modifiers that may be in scope. TextRunProperties textRunProperties = _textRunProperties; if (textModifierScope != null) { textRunProperties = textModifierScope.ModifyProperties(textRunProperties); } if (!rightToLeft) { // Fast loop early out for run with all non-complex characters // which can be optimized by not going thru shaping engine. int nominalLength; if (textRunProperties.Typeface.CheckFastPathNominalGlyphs( new CharacterBufferRange(characterBufferReference, length), textRunProperties.FontRenderingEmSize, 1.0, double.MaxValue, // widthMax true, // keepAWord digitCulture != null, CultureMapper.GetSpecificCulture(textRunProperties.CultureInfo), textFormattingMode, isSideways, out nominalLength ) && length == nominalLength) { return new TextShapeableCharacters[] { new TextShapeableCharacters( new CharacterBufferRange(characterBufferReference, nominalLength), textRunProperties, textRunProperties.FontRenderingEmSize, new MS.Internal.Text.TextInterface.ItemProps(), null, // shapeTypeface (no shaping required) false, // nullShape, textFormattingMode, isSideways ) }; } } IList shapeables = new List (2); glyphingCache.GetShapeableText( textRunProperties.Typeface, characterBufferReference, length, textRunProperties, digitCulture, isRightToLeftParagraph, shapeables, this as IShapeableTextCollector, textFormattingMode ); return shapeables; } #endregion #region IShapeableTextCollector implementation /// /// Add shapeable text object to the list /// void IShapeableTextCollector.Add( IListshapeables, CharacterBufferRange characterBufferRange, TextRunProperties textRunProperties, MS.Internal.Text.TextInterface.ItemProps textItem, ShapeTypeface shapeTypeface, double emScale, bool nullShape, TextFormattingMode textFormattingMode ) { Debug.Assert(shapeables != null); shapeables.Add( new TextShapeableCharacters( characterBufferRange, textRunProperties, textRunProperties.FontRenderingEmSize * emScale, textItem, shapeTypeface, nullShape, textFormattingMode, false ) ); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
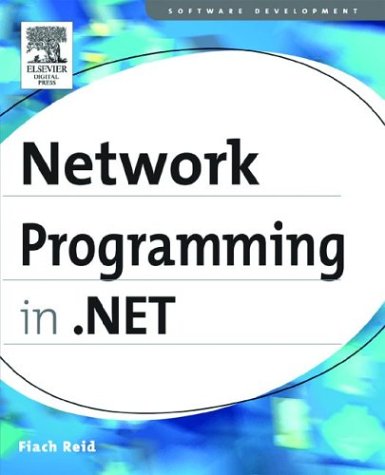
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Assert.cs
- EditorAttribute.cs
- DirectionalLight.cs
- ServiceReference.cs
- InstancePersistence.cs
- AnimationTimeline.cs
- FlowNode.cs
- ConfigurationValue.cs
- RewritingValidator.cs
- PersonalizationAdministration.cs
- autovalidator.cs
- CipherData.cs
- IteratorFilter.cs
- ArgumentNullException.cs
- WCFServiceClientProxyGenerator.cs
- complextypematerializer.cs
- ExclusiveHandleList.cs
- ConnectionPoint.cs
- HttpWriter.cs
- AllMembershipCondition.cs
- FieldAccessException.cs
- RecipientInfo.cs
- HttpRawResponse.cs
- MenuItemAutomationPeer.cs
- AnonymousIdentificationModule.cs
- ProxyWebPartManager.cs
- Add.cs
- AutoCompleteStringCollection.cs
- SqlUnionizer.cs
- Item.cs
- ValueExpressions.cs
- ContentElementAutomationPeer.cs
- UpdateInfo.cs
- GridViewRowEventArgs.cs
- PropertyKey.cs
- SEHException.cs
- CacheAxisQuery.cs
- AsyncOperationManager.cs
- _NtlmClient.cs
- MouseWheelEventArgs.cs
- ObjectDataSourceChooseMethodsPanel.cs
- XmlSerializerNamespaces.cs
- DynamicMethod.cs
- InvalidOperationException.cs
- ResXResourceReader.cs
- WebInvokeAttribute.cs
- BitmapVisualManager.cs
- TextElementAutomationPeer.cs
- SocketException.cs
- DropShadowEffect.cs
- ShellProvider.cs
- ResourceManager.cs
- sqlinternaltransaction.cs
- DbParameterHelper.cs
- TracingConnectionListener.cs
- ProfileModule.cs
- DataControlFieldCollection.cs
- SimpleWebHandlerParser.cs
- DiscreteKeyFrames.cs
- StrokeSerializer.cs
- LinqDataSourceDeleteEventArgs.cs
- SiteMapSection.cs
- PipelineModuleStepContainer.cs
- ImmutableCollection.cs
- CalendarDateRangeChangingEventArgs.cs
- RunWorkerCompletedEventArgs.cs
- XmlAttributeHolder.cs
- HttpAsyncResult.cs
- graph.cs
- ConfigurationValidatorBase.cs
- MenuAutomationPeer.cs
- LoopExpression.cs
- SymbolEqualComparer.cs
- Label.cs
- ZipFileInfo.cs
- DistinctQueryOperator.cs
- StrongName.cs
- KnownColorTable.cs
- TimeSpan.cs
- SessionEndingEventArgs.cs
- WorkflowFormatterBehavior.cs
- XhtmlBasicFormAdapter.cs
- ManagementOperationWatcher.cs
- MSHTMLHost.cs
- EntityDataSourceWizardForm.cs
- Int64Animation.cs
- ClientRolePrincipal.cs
- LogicalExpr.cs
- SoapIgnoreAttribute.cs
- TextEndOfLine.cs
- XsltFunctions.cs
- UrlMappingsSection.cs
- RepeaterItemEventArgs.cs
- CodeTypeParameterCollection.cs
- MessageProtectionOrder.cs
- OracleParameterCollection.cs
- StaticFileHandler.cs
- XPathNodeList.cs
- CompareInfo.cs
- Win32KeyboardDevice.cs