Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Input / Stylus / StylusPointPropertyId.cs / 1 / StylusPointPropertyId.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Input; using System.Windows.Media; using System.Collections.Generic; namespace System.Windows.Input { ////// StylusPointPropertyIds /// ///internal static class StylusPointPropertyIds { /// /// The x-coordinate in the tablet coordinate space. /// ///public static readonly Guid X = new Guid(0x598A6A8F, 0x52C0, 0x4BA0, 0x93, 0xAF, 0xAF, 0x35, 0x74, 0x11, 0xA5, 0x61); /// /// The y-coordinate in the tablet coordinate space. /// ///public static readonly Guid Y = new Guid(0xB53F9F75, 0x04E0, 0x4498, 0xA7, 0xEE, 0xC3, 0x0D, 0xBB, 0x5A, 0x90, 0x11); /// /// The z-coordinate or distance of the pen tip from the tablet surface. /// ///public static readonly Guid Z = new Guid(0x735ADB30, 0x0EBB, 0x4788, 0xA0, 0xE4, 0x0F, 0x31, 0x64, 0x90, 0x05, 0x5D); /// /// The width value of touch on the tablet surface. /// ///public static readonly Guid Width = new Guid(0xbaabe94d, 0x2712, 0x48f5, 0xbe, 0x9d, 0x8f, 0x8b, 0x5e, 0xa0, 0x71, 0x1a); /// /// The height value of touch on the tablet surface. /// ///public static readonly Guid Height = new Guid(0xe61858d2, 0xe447, 0x4218, 0x9d, 0x3f, 0x18, 0x86, 0x5c, 0x20, 0x3d, 0xf4); /// /// SystemTouch /// ///public static readonly Guid SystemTouch = new Guid(0xe706c804, 0x57f0, 0x4f00, 0x8a, 0x0c, 0x85, 0x3d, 0x57, 0x78, 0x9b, 0xe9); /// /// The current status of the pen pointer. /// ///public static readonly Guid PacketStatus = new Guid(0x6E0E07BF, 0xAFE7, 0x4CF7, 0x87, 0xD1, 0xAF, 0x64, 0x46, 0x20, 0x84, 0x18); /// /// Identifies the packet. /// ///public static readonly Guid SerialNumber = new Guid(0x78A81B56, 0x0935, 0x4493, 0xBA, 0xAE, 0x00, 0x54, 0x1A, 0x8A, 0x16, 0xC4); /// /// Downward pressure of the pen tip on the tablet surface. /// ///public static readonly Guid NormalPressure = new Guid(0x7307502D, 0xF9F4, 0x4E18, 0xB3, 0xF2, 0x2C, 0xE1, 0xB1, 0xA3, 0x61, 0x0C); /// /// Diagonal pressure of the pen tip on the tablet surface. /// ///public static readonly Guid TangentPressure = new Guid(0x6DA4488B, 0x5244, 0x41EC, 0x90, 0x5B, 0x32, 0xD8, 0x9A, 0xB8, 0x08, 0x09); /// /// Pressure on a pressure sensitive button. /// ///public static readonly Guid ButtonPressure = new Guid(0x8B7FEFC4, 0x96AA, 0x4BFE, 0xAC, 0x26, 0x8A, 0x5F, 0x0B, 0xE0, 0x7B, 0xF5); /// /// The x-tilt orientation is the angle between the y,z-plane and the pen and y-axis plane. /// ///public static readonly Guid XTiltOrientation = new Guid(0xA8D07B3A, 0x8BF0, 0x40B0, 0x95, 0xA9, 0xB8, 0x0A, 0x6B, 0xB7, 0x87, 0xBF); /// /// The y-tilt orientation is the angle between the x,z-plane and the pen and x-axis plane. /// ///public static readonly Guid YTiltOrientation = new Guid(0x0E932389, 0x1D77, 0x43AF, 0xAC, 0x00, 0x5B, 0x95, 0x0D, 0x6D, 0x4B, 0x2D); /// /// Clockwise rotation of the pen about the z axis through a full circular range. /// ///public static readonly Guid AzimuthOrientation = new Guid(0x029123B4, 0x8828, 0x410B, 0xB2, 0x50, 0xA0, 0x53, 0x65, 0x95, 0xE5, 0xDC); /// /// Angle between the axis of the pen and the surface of the tablet. /// ///public static readonly Guid AltitudeOrientation = new Guid(0x82DEC5C7, 0xF6BA, 0x4906, 0x89, 0x4F, 0x66, 0xD6, 0x8D, 0xFC, 0x45, 0x6C); /// /// Clockwise rotation of the pen about its own axis. /// ///public static readonly Guid TwistOrientation = new Guid(0x0D324960, 0x13B2, 0x41E4, 0xAC, 0xE6, 0x7A, 0xE9, 0xD4, 0x3D, 0x2D, 0x3B); /// /// Identifies whether the tip is above or below a horizontal line that is perpendicular to the writing surface. Requires 3D digitizer. /// ///public static readonly Guid PitchRotation = new Guid(0x7F7E57B7, 0xBE37, 0x4BE1, 0xA3, 0x56, 0x7A, 0x84, 0x16, 0x0E, 0x18, 0x93); /// /// Clockwise rotation of the pen about its own axis. Requires 3D digitizer. /// ///public static readonly Guid RollRotation = new Guid(0x5D5D5E56, 0x6BA9, 0x4C5B, 0x9F, 0xB0, 0x85, 0x1C, 0x91, 0x71, 0x4E, 0x56); /// /// Yaw identifies whether the tip is turning left or right around the center of its horzontal axis (pen is horizontal). Requires 3D digitizer. /// ///public static readonly Guid YawRotation = new Guid(0x6A849980, 0x7C3A, 0x45B7, 0xAA, 0x82, 0x90, 0xA2, 0x62, 0x95, 0x0E, 0x89); /// /// Identifies the tip button of a stylus. Used for identifying StylusButtons in StylusPointDescription. /// ///public static readonly Guid TipButton = new Guid(0x39143d3, 0x78cb, 0x449c, 0xa8, 0xe7, 0x67, 0xd1, 0x88, 0x64, 0xc3, 0x32); /// /// Identifies the button on the barrel of a stylus. Used for identifying StylusButtons in StylusPointDescription. /// ///public static readonly Guid BarrelButton = new Guid(0xf0720328, 0x663b, 0x418f, 0x85, 0xa6, 0x95, 0x31, 0xae, 0x3e, 0xcd, 0xfa); /// /// Identifies the secondary tip barrel button of a stylus. Used for identifying StylusButtons in StylusPointDescription. /// ///public static readonly Guid SecondaryTipButton = new Guid(0x67743782, 0xee5, 0x419a, 0xa1, 0x2b, 0x27, 0x3a, 0x9e, 0xc0, 0x8f, 0x3d); /// /// Called by the StylusPointProperty constructor. /// Any new Guids in this static class should be added here /// /// guid internal static bool IsKnownId(Guid guid) { if (guid == X || guid == Y || guid == Z || guid == Width || guid == Height || guid == SystemTouch || guid == PacketStatus || guid == SerialNumber || guid == NormalPressure || guid == TangentPressure || guid == ButtonPressure || guid == XTiltOrientation || guid == YTiltOrientation || guid == AzimuthOrientation || guid == AltitudeOrientation || guid == TwistOrientation || guid == PitchRotation || guid == RollRotation || guid == YawRotation || guid == TipButton || guid == BarrelButton || guid == SecondaryTipButton) { return true; } return false; } ////// Called by the StylusPointProperty constructor. /// Any new Guids in this static class should be added here /// /// guid internal static string GetStringRepresentation(Guid guid) { if (guid == X) { return "X"; } if (guid == Y) { return "Y"; } if (guid == Z) { return "Z"; } if (guid == Width) { return "Width"; } if (guid == Height) { return "Height"; } if (guid == SystemTouch) { return "SystemTouch"; } if (guid == PacketStatus) { return "PacketStatus"; } if (guid == SerialNumber) { return "SerialNumber"; } if (guid == NormalPressure) { return "NormalPressure"; } if (guid == TangentPressure) { return "TangentPressure"; } if (guid == ButtonPressure) { return "ButtonPressure"; } if (guid == XTiltOrientation) { return "XTiltOrientation"; } if (guid == YTiltOrientation) { return "YTiltOrientation"; } if (guid == AzimuthOrientation) { return "AzimuthOrientation"; } if (guid == AltitudeOrientation) { return "AltitudeOrientation"; } if (guid == TwistOrientation) { return "TwistOrientation"; } if (guid == PitchRotation) { return "PitchRotation"; } if (guid == RollRotation) { return "RollRotation"; } if (guid == AltitudeOrientation) { return "AltitudeOrientation"; } if (guid == YawRotation) { return "YawRotation"; } if (guid == TipButton) { return "TipButton"; } if (guid == BarrelButton) { return "BarrelButton"; } if (guid == SecondaryTipButton) { return "SecondaryTipButton"; } return "Unknown"; } ////// Called by the StylusPointProperty constructor. /// Any new button Guids in this static class should be added here /// /// guid internal static bool IsKnownButton(Guid guid) { if (guid == TipButton || guid == BarrelButton || guid == SecondaryTipButton) { return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
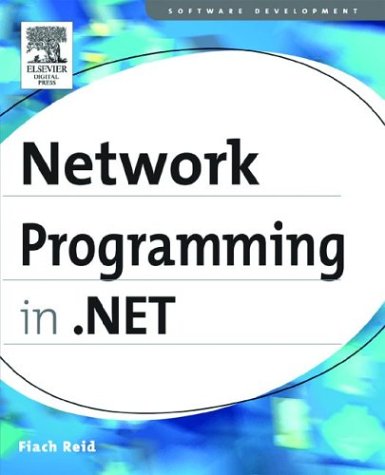
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- NameTable.cs
- SortExpressionBuilder.cs
- ZoomPercentageConverter.cs
- LexicalChunk.cs
- XmlWriterTraceListener.cs
- CodeGenerationManager.cs
- ProviderException.cs
- DigitalSignature.cs
- InputProcessorProfiles.cs
- SoapAttributeAttribute.cs
- WriteableOnDemandPackagePart.cs
- Pointer.cs
- autovalidator.cs
- ExpressionEditorAttribute.cs
- MemoryMappedViewAccessor.cs
- ObjectMemberMapping.cs
- IPAddress.cs
- COM2IPerPropertyBrowsingHandler.cs
- XsltLoader.cs
- SynchronizedInputHelper.cs
- CollectionViewGroupInternal.cs
- TraceUtility.cs
- PageHandlerFactory.cs
- NumericExpr.cs
- HttpValueCollection.cs
- HighContrastHelper.cs
- ConfigXmlAttribute.cs
- AppModelKnownContentFactory.cs
- RemoteDebugger.cs
- ContentElement.cs
- _CommandStream.cs
- ConnectionPoolManager.cs
- RuntimeCompatibilityAttribute.cs
- XmlSchemaObjectCollection.cs
- FileBasedResourceGroveler.cs
- RedBlackList.cs
- GridViewCellAutomationPeer.cs
- Misc.cs
- WebPartUserCapability.cs
- StylusPointDescription.cs
- SqlRowUpdatingEvent.cs
- SoapSchemaImporter.cs
- CreateUserErrorEventArgs.cs
- TextContainer.cs
- SectionInput.cs
- ForeignConstraint.cs
- WebPartVerb.cs
- RoutedPropertyChangedEventArgs.cs
- clipboard.cs
- Int64Storage.cs
- HebrewCalendar.cs
- TableRowGroup.cs
- Scene3D.cs
- ProfilePropertyNameValidator.cs
- TypeDescriptionProviderAttribute.cs
- EmbossBitmapEffect.cs
- TypeNameParser.cs
- SdlChannelSink.cs
- GenericArgumentsUpdater.cs
- TextBoxBaseDesigner.cs
- UpdateException.cs
- SafeHandles.cs
- VSWCFServiceContractGenerator.cs
- VerificationException.cs
- GridItemCollection.cs
- SqlDataAdapter.cs
- CodeSnippetStatement.cs
- CreateUserWizardAutoFormat.cs
- CodeCatchClause.cs
- RelationshipNavigation.cs
- TransformGroup.cs
- AxisAngleRotation3D.cs
- DecoratedNameAttribute.cs
- UnionCqlBlock.cs
- EncodingDataItem.cs
- ImplicitInputBrush.cs
- DescendantOverDescendantQuery.cs
- CapabilitiesAssignment.cs
- Timeline.cs
- ActivityDesigner.cs
- ConsoleKeyInfo.cs
- AnnotationHighlightLayer.cs
- RowUpdatedEventArgs.cs
- PixelFormatConverter.cs
- BrowsableAttribute.cs
- WorkflowView.cs
- LayoutEngine.cs
- ProvidersHelper.cs
- ProfilePropertySettings.cs
- Page.cs
- Convert.cs
- SerialStream.cs
- CookieHandler.cs
- DocumentsTrace.cs
- cookieexception.cs
- ServiceDocument.cs
- CatalogPartChrome.cs
- TextTreeNode.cs
- GraphicsPathIterator.cs
- SerializationAttributes.cs