Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / MS / Internal / IO / Packaging / CompoundFile / CompressionTransform.cs / 1 / CompressionTransform.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // This class implements IDataTransform for the Compression transform. // // History: // 06/04/2002: [....]: Initial implementation. // 05/29/2003: [....]: Ported to WCP tree. // 05/17/2005: [....]: Port to Office-compatible block-based format // 05/30/2005: [....]: Moved to System.IO.Packaging namespace // 12/14/2005: [....]: Moved to MS.Internal.IO.Packaging.CompoundFile namespace // //----------------------------------------------------------------------------- using System; using System.Collections; // for IDictionary using System.IO; // for Stream using System.IO.Packaging; using System.Globalization; // for CultureInfo using System.Windows; // ExceptionStringTable using MS.Internal.IO.Packaging; // CompoundFileEmulationStream using MS.Internal.IO.Packaging.CompoundFile; //using System.Windows; namespace MS.Internal.IO.Packaging.CompoundFile { ////// CompressionTransform for use in Compound File DataSpaces /// internal class CompressionTransform : IDataTransform { #region IDataTransform ////// Transform readiness /// public bool IsReady { get { return true; } } ////// No configuration parameters for this transform /// public bool FixedSettings { get { return true; } } ////// Returns the type name identifier string. /// public object TransformIdentifier { get { return CompressionTransform.ClassTransformIdentifier; } } ////// Expose the transform identifier for the use of the DataSpaceManager. /// internal static string ClassTransformIdentifier { get { return "{86DE7F2B-DDCE-486d-B016-405BBE82B8BC}"; } } //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- ////// Given output stream returns stream for encoding/decoding /// /// the encoded stream that this transform acts on /// Dictionary object used to store any additional context information ///the stream that implements the transform ////// This method is used only by the DataSpaceManager, so we declare it as an explicit /// interface implementation to hide it from the public interface. /// Stream IDataTransform.GetTransformedStream( Stream encodedStream, IDictionary transformContext ) { Stream tempStream = new SparseMemoryStream(_lowWaterMark, _highWaterMark); tempStream = new CompressEmulationStream(encodedStream, tempStream, 0, new CompoundFileDeflateTransform()); // return a VersionedStream that works with the VersionedStreamOwner // to verify/update our FormatVersion info return new VersionedStream(tempStream, _versionedStreamOwner); } #endregion ////// constructor /// /// environment ///this should only be used by the DataSpaceManager class public CompressionTransform(TransformEnvironment myEnvironment) { _transformEnvironment = myEnvironment; // Create a wrapper that manages persistence and comparison of FormatVersion // in our InstanceData stream. We can read/write to this stream as needed (though CompressionTransform // does not because we don't house any non-FormatVersion data in the instance data stream). // We need to give out our current code version so it can compare with any file version as appropriate. _versionedStreamOwner = new VersionedStreamOwner( _transformEnvironment.GetPrimaryInstanceData(), new FormatVersion(_featureName, _minimumReaderVersion, _minimumUpdaterVersion, _currentFeatureVersion)); } //------------------------------------------------------ // // Private Data // //----------------------------------------------------- private TransformEnvironment _transformEnvironment; private VersionedStreamOwner _versionedStreamOwner; // our instance data stream wrapped private static readonly string _featureName = "Microsoft.Metadata.CompressionTransform"; private static readonly VersionPair _currentFeatureVersion = new VersionPair(1, 0); private static readonly VersionPair _minimumReaderVersion = new VersionPair(1, 0); private static readonly VersionPair _minimumUpdaterVersion = new VersionPair(1, 0); private const long _lowWaterMark = 0x19000; // we definitely would like to keep everything under 100 KB in memory private const long _highWaterMark = 0xA00000; // we would like to keep everything over 10 MB on disk } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
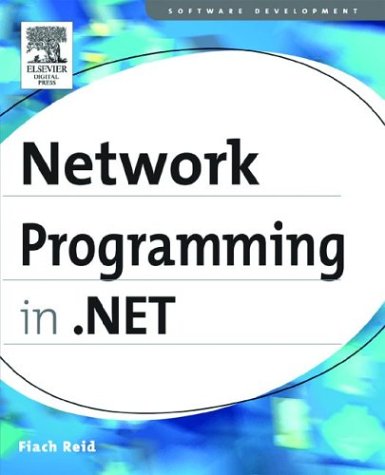
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EarlyBoundInfo.cs
- Manipulation.cs
- FtpRequestCacheValidator.cs
- CompoundFileStreamReference.cs
- TypefaceMap.cs
- ByteRangeDownloader.cs
- ServicesSection.cs
- DocumentOrderComparer.cs
- VideoDrawing.cs
- LinkDescriptor.cs
- ImportContext.cs
- TabControlToolboxItem.cs
- BitmapInitialize.cs
- CapabilitiesAssignment.cs
- WSTransactionSection.cs
- SafeEventLogWriteHandle.cs
- TextTreeTextNode.cs
- Cell.cs
- SecurityTokenProvider.cs
- LayoutDump.cs
- ReachFixedPageSerializerAsync.cs
- DtdParser.cs
- StrokeCollection.cs
- HitTestFilterBehavior.cs
- XmlnsCompatibleWithAttribute.cs
- XmlCharCheckingWriter.cs
- BitArray.cs
- DockPattern.cs
- CachingHintValidation.cs
- WebPartConnectionsCancelEventArgs.cs
- BCryptHashAlgorithm.cs
- HtmlElementErrorEventArgs.cs
- DecimalAnimation.cs
- LineVisual.cs
- TiffBitmapDecoder.cs
- WindowsAuthenticationEventArgs.cs
- ListViewGroupConverter.cs
- ValidationPropertyAttribute.cs
- UniformGrid.cs
- GridViewHeaderRowPresenter.cs
- ServiceObjectContainer.cs
- DataGridViewCell.cs
- ParagraphVisual.cs
- SqlDataSourceConfigureFilterForm.cs
- EntityCommandDefinition.cs
- CmsInterop.cs
- InputReferenceExpression.cs
- UnknownExceptionActionHelper.cs
- MessageVersion.cs
- Parameter.cs
- TCEAdapterGenerator.cs
- TemplateKeyConverter.cs
- HtmlTableCell.cs
- Empty.cs
- PKCS1MaskGenerationMethod.cs
- DataRelation.cs
- SqlErrorCollection.cs
- TypeValidationEventArgs.cs
- SignerInfo.cs
- TiffBitmapEncoder.cs
- GlobalDataBindingHandler.cs
- Double.cs
- ExecutionTracker.cs
- PointLightBase.cs
- TextContainerChangeEventArgs.cs
- WebResponse.cs
- StringAttributeCollection.cs
- BitmapImage.cs
- AutomationElement.cs
- LiteralTextParser.cs
- BooleanConverter.cs
- GroupPartitionExpr.cs
- MergeFilterQuery.cs
- FirstMatchCodeGroup.cs
- HybridWebProxyFinder.cs
- AddInIpcChannel.cs
- GroupItemAutomationPeer.cs
- SelectionEditingBehavior.cs
- LoginNameDesigner.cs
- SQLConvert.cs
- TextRangeProviderWrapper.cs
- XmlEntityReference.cs
- RuntimeConfig.cs
- EventLogPermissionEntryCollection.cs
- ProxyAttribute.cs
- DbMetaDataColumnNames.cs
- ParameterBuilder.cs
- Environment.cs
- UIElementParaClient.cs
- ComplexBindingPropertiesAttribute.cs
- Crc32Helper.cs
- SortedList.cs
- AttributeSetAction.cs
- OdbcReferenceCollection.cs
- SQLBinaryStorage.cs
- DataServiceQueryProvider.cs
- SamlAuthorizationDecisionStatement.cs
- ConfigurationPropertyCollection.cs
- PolicyUnit.cs
- SqlNodeAnnotation.cs