Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / HybridWebProxyFinder.cs / 1305376 / HybridWebProxyFinder.cs
using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Net { // This class behaves the same as WinHttpWebProxyFinder. The only difference is that in cases where // the script location has a scheme != HTTP, it falls back to NetWebProxyFinder which supports // also other schemes like FILE and FTP. // The mid-term goal for WinHttp is to support at least FILE scheme since it was already requested // by customers. The long term goal for System.Net is to use WinHttp only and remove this class // as well as NetWebProxyFinder. internal sealed class HybridWebProxyFinder : IWebProxyFinder { private NetWebProxyFinder netFinder; private WinHttpWebProxyFinder winHttpFinder; private BaseWebProxyFinder currentFinder; private AutoWebProxyScriptEngine engine; public HybridWebProxyFinder(AutoWebProxyScriptEngine engine) { this.engine = engine; this.winHttpFinder = new WinHttpWebProxyFinder(engine); this.currentFinder = winHttpFinder; } public bool IsValid { get { return currentFinder.IsValid; } } public bool GetProxies(Uri destination, out IListproxyList) { if (currentFinder.GetProxies(destination, out proxyList)) { return true; } if (currentFinder.IsUnrecognizedScheme && (currentFinder == winHttpFinder)) { // If WinHttpWebProxyFinder failed because the script location has a != HTTP scheme, // fall back to NetWebProxyFinder which supports also other schemes. if (netFinder == null) { netFinder = new NetWebProxyFinder(engine); } currentFinder = netFinder; return currentFinder.GetProxies(destination, out proxyList); } // Something else went wrong. Falling back to NetWebProxyFinder wouldn't help. return false; } public void Abort() { // Abort only the current finder. There is no need to abort the other one (which is either // uninitialized, i.e. not used yet, or we have an unrecognized-scheme state, which should // not be changed). currentFinder.Abort(); } public void Reset() { winHttpFinder.Reset(); if (netFinder != null) { netFinder.Reset(); } // Some settings changed, so let's reset the current finder to WinHttpWebProxyFinder, since // now it may work (if it didn't already before). currentFinder = winHttpFinder; } public void Dispose() { Dispose(true); } private void Dispose(bool disposing) { if (disposing) { winHttpFinder.Dispose(); if (netFinder != null) { netFinder.Dispose(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Diagnostics; namespace System.Net { // This class behaves the same as WinHttpWebProxyFinder. The only difference is that in cases where // the script location has a scheme != HTTP, it falls back to NetWebProxyFinder which supports // also other schemes like FILE and FTP. // The mid-term goal for WinHttp is to support at least FILE scheme since it was already requested // by customers. The long term goal for System.Net is to use WinHttp only and remove this class // as well as NetWebProxyFinder. internal sealed class HybridWebProxyFinder : IWebProxyFinder { private NetWebProxyFinder netFinder; private WinHttpWebProxyFinder winHttpFinder; private BaseWebProxyFinder currentFinder; private AutoWebProxyScriptEngine engine; public HybridWebProxyFinder(AutoWebProxyScriptEngine engine) { this.engine = engine; this.winHttpFinder = new WinHttpWebProxyFinder(engine); this.currentFinder = winHttpFinder; } public bool IsValid { get { return currentFinder.IsValid; } } public bool GetProxies(Uri destination, out IList proxyList) { if (currentFinder.GetProxies(destination, out proxyList)) { return true; } if (currentFinder.IsUnrecognizedScheme && (currentFinder == winHttpFinder)) { // If WinHttpWebProxyFinder failed because the script location has a != HTTP scheme, // fall back to NetWebProxyFinder which supports also other schemes. if (netFinder == null) { netFinder = new NetWebProxyFinder(engine); } currentFinder = netFinder; return currentFinder.GetProxies(destination, out proxyList); } // Something else went wrong. Falling back to NetWebProxyFinder wouldn't help. return false; } public void Abort() { // Abort only the current finder. There is no need to abort the other one (which is either // uninitialized, i.e. not used yet, or we have an unrecognized-scheme state, which should // not be changed). currentFinder.Abort(); } public void Reset() { winHttpFinder.Reset(); if (netFinder != null) { netFinder.Reset(); } // Some settings changed, so let's reset the current finder to WinHttpWebProxyFinder, since // now it may work (if it didn't already before). currentFinder = winHttpFinder; } public void Dispose() { Dispose(true); } private void Dispose(bool disposing) { if (disposing) { winHttpFinder.Dispose(); if (netFinder != null) { netFinder.Dispose(); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
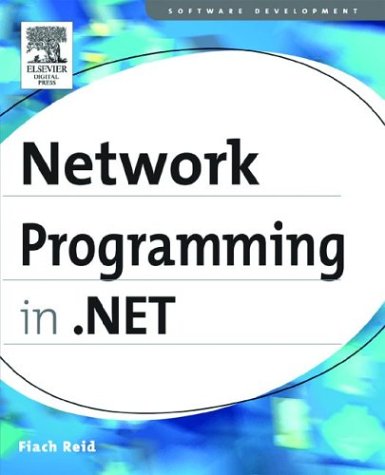
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Tokenizer.cs
- SqlError.cs
- PerfCounters.cs
- DataServiceException.cs
- ProviderCommandInfoUtils.cs
- odbcmetadatafactory.cs
- ZipPackagePart.cs
- DataServiceProcessingPipeline.cs
- Completion.cs
- Paragraph.cs
- XmlSerializerVersionAttribute.cs
- AssemblyResourceLoader.cs
- Trace.cs
- TreeNodeEventArgs.cs
- WebPartDisplayModeEventArgs.cs
- PreloadedPackages.cs
- translator.cs
- DataGridTable.cs
- HttpConfigurationContext.cs
- UnmanagedMemoryStream.cs
- SystemResources.cs
- IgnoreSection.cs
- GroupItemAutomationPeer.cs
- MachineKeyValidationConverter.cs
- FreeFormPanel.cs
- EpmSyndicationContentDeSerializer.cs
- DataGridViewImageCell.cs
- FaultImportOptions.cs
- HierarchicalDataTemplate.cs
- GeneralTransform3DGroup.cs
- PreviewKeyDownEventArgs.cs
- SqlDependency.cs
- EntityDataSourceUtil.cs
- ListViewItemMouseHoverEvent.cs
- HtmlCalendarAdapter.cs
- ParseElement.cs
- Int64Storage.cs
- WebPartConnectionsConfigureVerb.cs
- SoapObjectWriter.cs
- SchemaManager.cs
- OutputCacheModule.cs
- DataGridViewDataConnection.cs
- StringResourceManager.cs
- TransformerConfigurationWizardBase.cs
- TextDecorationCollectionConverter.cs
- ObjectSpanRewriter.cs
- EventTrigger.cs
- LeftCellWrapper.cs
- LayoutTableCell.cs
- MetaTableHelper.cs
- latinshape.cs
- BufferedGraphics.cs
- TreeIterator.cs
- ProgressChangedEventArgs.cs
- XamlInterfaces.cs
- XmlUnspecifiedAttribute.cs
- CapabilitiesRule.cs
- SettingsPropertyWrongTypeException.cs
- TextSpanModifier.cs
- HttpModuleCollection.cs
- ListenerElementsCollection.cs
- listitem.cs
- Mappings.cs
- RoutedPropertyChangedEventArgs.cs
- SByte.cs
- EventHandlers.cs
- Selector.cs
- _Events.cs
- ImagingCache.cs
- DataGridViewRowStateChangedEventArgs.cs
- VariableQuery.cs
- WindowsComboBox.cs
- EmptyQuery.cs
- ListGeneralPage.cs
- Transform.cs
- InstalledFontCollection.cs
- XhtmlBasicPageAdapter.cs
- ToolConsole.cs
- DocumentDesigner.cs
- MissingSatelliteAssemblyException.cs
- RadialGradientBrush.cs
- WorkflowCreationContext.cs
- StringInfo.cs
- PolyBezierSegment.cs
- GenericEnumConverter.cs
- ClipboardData.cs
- ButtonChrome.cs
- BinaryConverter.cs
- WrapPanel.cs
- AnimatedTypeHelpers.cs
- LinearGradientBrush.cs
- _ListenerRequestStream.cs
- TabItem.cs
- Enum.cs
- ComplusEndpointConfigContainer.cs
- InheritanceContextHelper.cs
- SimpleBitVector32.cs
- DataGridViewRowConverter.cs
- EntityAdapter.cs
- BamlCollectionHolder.cs