Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media3D / Transform3DGroup.cs / 1305600 / Transform3DGroup.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: 3D transform collection. // // See spec at http://avalon/medialayer/Specifications/Avalon3D%20API%20Spec.mht // // History: // 06/11/2003 : t-gregr - Created // 01/19/2004 : [....] - Changed to Transform3DGroup // //--------------------------------------------------------------------------- using System; using System.Windows; using System.Windows.Media; using System.Windows.Media.Composition; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Reflection; using System.Collections; using System.Collections.Generic; using MS.Internal; using System.Windows.Media.Animation; using System.Globalization; using System.Text; using System.Runtime.InteropServices; using System.Windows.Markup; using System.Diagnostics; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Media3D { ////// 3D transform group. /// [ContentProperty("Children")] public sealed partial class Transform3DGroup : Transform3D { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default constructor. /// public Transform3DGroup() {} #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Return the current transformation value. /// public override Matrix3D Value { get { ReadPreamble(); Matrix3D transform = new Matrix3D(); Append(ref transform); return transform; } } ////// Whether the transform is affine. /// public override bool IsAffine { get { ReadPreamble(); Transform3DCollection children = Children; if (children != null) { for (int i = 0, count = children.Count; i < count; ++i) { Transform3D transform = children.Internal_GetItem(i); if (!transform.IsAffine) { return false; } } } return true; } } #endregion Public Methods //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ internal override void Append(ref Matrix3D matrix) { Transform3DCollection children = Children; if (children != null) { for (int i = 0, count = children.Count; i < count; i++) { children.Internal_GetItem(i).Append(ref matrix); } } } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
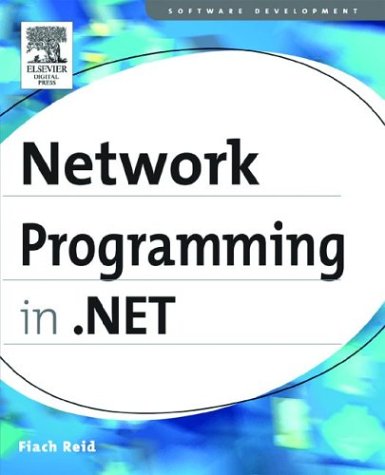
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RtfControlWordInfo.cs
- TraceSwitch.cs
- GridView.cs
- DoubleLinkList.cs
- FixedDocumentPaginator.cs
- SystemDropShadowChrome.cs
- XmlDataLoader.cs
- ArrayElementGridEntry.cs
- HttpRuntime.cs
- TreeView.cs
- RTTypeWrapper.cs
- CommandValueSerializer.cs
- Ray3DHitTestResult.cs
- TextElementEnumerator.cs
- ListDictionary.cs
- Operator.cs
- MarginCollapsingState.cs
- CodeEntryPointMethod.cs
- ObjectItemConventionAssemblyLoader.cs
- Thickness.cs
- StoreContentChangedEventArgs.cs
- PictureBox.cs
- HostingEnvironmentWrapper.cs
- GridViewDeleteEventArgs.cs
- ThreadStaticAttribute.cs
- VirtualDirectoryMapping.cs
- DataRow.cs
- MimeMapping.cs
- XmlDictionary.cs
- DocumentXmlWriter.cs
- RowUpdatedEventArgs.cs
- AccessDataSourceWizardForm.cs
- WhitespaceRuleLookup.cs
- DirectoryGroupQuery.cs
- LinkButton.cs
- KnownIds.cs
- UInt64Storage.cs
- SqlOuterApplyReducer.cs
- StyleCollection.cs
- CodeArrayIndexerExpression.cs
- X509ChainElement.cs
- MetadataPropertyCollection.cs
- ObjectListGeneralPage.cs
- TreeViewItemAutomationPeer.cs
- TdsParameterSetter.cs
- HostedElements.cs
- Context.cs
- MemoryPressure.cs
- WindowsListViewScroll.cs
- CustomError.cs
- X509CertificateCollection.cs
- SoapSchemaMember.cs
- SoapExtensionTypeElementCollection.cs
- Token.cs
- PageThemeBuildProvider.cs
- SwitchElementsCollection.cs
- WrappedDispatcherException.cs
- CmsInterop.cs
- SoapEnumAttribute.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ImageKeyConverter.cs
- LocalizationComments.cs
- CommandLibraryHelper.cs
- SqlDataSourceSelectingEventArgs.cs
- CodeGenerationManager.cs
- HttpWriter.cs
- Attributes.cs
- MasterPageParser.cs
- SendKeys.cs
- DeliveryRequirementsAttribute.cs
- EntityException.cs
- EmbeddedObject.cs
- BeginEvent.cs
- NetMsmqSecurityMode.cs
- SrgsItemList.cs
- Button.cs
- ToolStrip.cs
- TextPatternIdentifiers.cs
- DropDownButton.cs
- AttributeCollection.cs
- CompositeKey.cs
- BuildProvider.cs
- PropertyChangedEventArgs.cs
- TableLayoutColumnStyleCollection.cs
- IisTraceListener.cs
- PropertyGroupDescription.cs
- LocatorBase.cs
- MetadataPropertyCollection.cs
- COM2ColorConverter.cs
- Line.cs
- ManipulationLogic.cs
- ImageInfo.cs
- HtmlEmptyTagControlBuilder.cs
- CodeGroup.cs
- StringKeyFrameCollection.cs
- EntityStoreSchemaFilterEntry.cs
- CacheMemory.cs
- BoundField.cs
- XmlNodeReader.cs
- RequiredFieldValidator.cs