Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntityDesign / Design / System / Data / Entity / Design / EntityStoreSchemaFilterEntry.cs / 1 / EntityStoreSchemaFilterEntry.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Entity.Design.Common; namespace System.Data.Entity.Design { ////// This class represent a single filter entry /// public class EntityStoreSchemaFilterEntry { private string _catalog; private string _schema; private string _name; private EntityStoreSchemaFilterObjectTypes _types; private EntityStoreSchemaFilterEffect _effect; ////// Creates a EntityStoreSchemaFilterEntry /// /// The pattern to use to select the appropriate catalog or null to not limit by catalog. /// The pattern to use to select the appropriate schema or null to not limit by schema. /// The pattern to use to select the appropriate name or null to not limit by name. /// The type of objects to apply this filter to. /// The effect that this filter should have on the results. public EntityStoreSchemaFilterEntry(string catalog, string schema, string name, EntityStoreSchemaFilterObjectTypes types, EntityStoreSchemaFilterEffect effect) { if (types == EntityStoreSchemaFilterObjectTypes.None) { throw EDesignUtil.Argument("types"); } _catalog = catalog; _schema = schema; _name = name; _types = types; _effect = effect; } ////// Creates a EntityStoreSchemaFilterEntry /// /// The pattern to use to select the appropriate catalog or null to not limit by catalog. /// The pattern to use to select the appropriate schema or null to not limit by schema. /// The pattern to use to select the appropriate name or null to not limit by name. public EntityStoreSchemaFilterEntry(string catalog, string schema, string name) :this(catalog, schema, name, EntityStoreSchemaFilterObjectTypes.All, EntityStoreSchemaFilterEffect.Allow) { } ////// Gets the pattern that will be used to select the appropriate catalog. /// public string Catalog { [DebuggerStepThroughAttribute] get { return _catalog; } } ////// Gets the pattern that will be used to select the appropriate schema. /// public string Schema { [DebuggerStepThroughAttribute] get { return _schema; } } ////// Gets the pattern that will be used to select the appropriate name. /// public string Name { [DebuggerStepThroughAttribute] get { return _name; } } ////// Gets the types of objects that this filter applies to. /// public EntityStoreSchemaFilterObjectTypes Types { [DebuggerStepThroughAttribute] get { return _types; } } ////// Gets the effect that this filter has on results. /// public EntityStoreSchemaFilterEffect Effect { [DebuggerStepThroughAttribute] get { return _effect; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Data.Entity.Design.Common; namespace System.Data.Entity.Design { ////// This class represent a single filter entry /// public class EntityStoreSchemaFilterEntry { private string _catalog; private string _schema; private string _name; private EntityStoreSchemaFilterObjectTypes _types; private EntityStoreSchemaFilterEffect _effect; ////// Creates a EntityStoreSchemaFilterEntry /// /// The pattern to use to select the appropriate catalog or null to not limit by catalog. /// The pattern to use to select the appropriate schema or null to not limit by schema. /// The pattern to use to select the appropriate name or null to not limit by name. /// The type of objects to apply this filter to. /// The effect that this filter should have on the results. public EntityStoreSchemaFilterEntry(string catalog, string schema, string name, EntityStoreSchemaFilterObjectTypes types, EntityStoreSchemaFilterEffect effect) { if (types == EntityStoreSchemaFilterObjectTypes.None) { throw EDesignUtil.Argument("types"); } _catalog = catalog; _schema = schema; _name = name; _types = types; _effect = effect; } ////// Creates a EntityStoreSchemaFilterEntry /// /// The pattern to use to select the appropriate catalog or null to not limit by catalog. /// The pattern to use to select the appropriate schema or null to not limit by schema. /// The pattern to use to select the appropriate name or null to not limit by name. public EntityStoreSchemaFilterEntry(string catalog, string schema, string name) :this(catalog, schema, name, EntityStoreSchemaFilterObjectTypes.All, EntityStoreSchemaFilterEffect.Allow) { } ////// Gets the pattern that will be used to select the appropriate catalog. /// public string Catalog { [DebuggerStepThroughAttribute] get { return _catalog; } } ////// Gets the pattern that will be used to select the appropriate schema. /// public string Schema { [DebuggerStepThroughAttribute] get { return _schema; } } ////// Gets the pattern that will be used to select the appropriate name. /// public string Name { [DebuggerStepThroughAttribute] get { return _name; } } ////// Gets the types of objects that this filter applies to. /// public EntityStoreSchemaFilterObjectTypes Types { [DebuggerStepThroughAttribute] get { return _types; } } ////// Gets the effect that this filter has on results. /// public EntityStoreSchemaFilterEffect Effect { [DebuggerStepThroughAttribute] get { return _effect; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
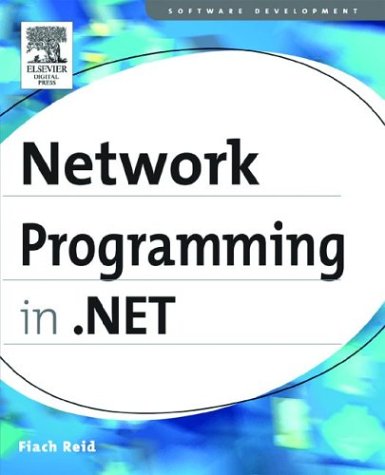
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextSerializer.cs
- InputLanguageCollection.cs
- TextAction.cs
- SiteMembershipCondition.cs
- XmlDataLoader.cs
- MappingItemCollection.cs
- MeasurementDCInfo.cs
- PagedDataSource.cs
- FrameworkElement.cs
- BamlBinaryWriter.cs
- HandleCollector.cs
- PersonalizableTypeEntry.cs
- StrokeNodeEnumerator.cs
- WebPartCatalogAddVerb.cs
- SpinLock.cs
- SimpleBitVector32.cs
- PreviousTrackingServiceAttribute.cs
- ProxySimple.cs
- RsaSecurityKey.cs
- ColumnResizeAdorner.cs
- InheritanceContextHelper.cs
- ArrangedElementCollection.cs
- RMPermissions.cs
- NativeObjectSecurity.cs
- DesignerActionUIStateChangeEventArgs.cs
- FileUtil.cs
- sqlnorm.cs
- CommonDialog.cs
- MatrixAnimationBase.cs
- SynchronizedInputProviderWrapper.cs
- ToolStripGripRenderEventArgs.cs
- CodeCatchClause.cs
- EmissiveMaterial.cs
- xsdvalidator.cs
- MethodMessage.cs
- DecimalConstantAttribute.cs
- SqlConnection.cs
- ResourceDescriptionAttribute.cs
- OutputScope.cs
- TaskFileService.cs
- DescendantBaseQuery.cs
- ValidationError.cs
- ManipulationStartingEventArgs.cs
- GridItem.cs
- SendParametersContent.cs
- C14NUtil.cs
- PriorityRange.cs
- TextParaClient.cs
- DbConvert.cs
- TreeNodeSelectionProcessor.cs
- TargetException.cs
- PerformanceCounterScope.cs
- controlskin.cs
- SecurityContext.cs
- Size.cs
- WebColorConverter.cs
- HttpGetProtocolReflector.cs
- SortableBindingList.cs
- BaseTemplateCodeDomTreeGenerator.cs
- SecurityHelper.cs
- ResourceManager.cs
- WebPartDisplayModeEventArgs.cs
- BindingContext.cs
- MachineKeySection.cs
- WebResourceAttribute.cs
- HMAC.cs
- RuntimeTransactionHandle.cs
- ColorConvertedBitmapExtension.cs
- MimeBasePart.cs
- TextAutomationPeer.cs
- WindowsListViewItemCheckBox.cs
- SourceChangedEventArgs.cs
- CodeGroup.cs
- StringCollection.cs
- SizeChangedInfo.cs
- SeverityFilter.cs
- PostBackOptions.cs
- XamlPoint3DCollectionSerializer.cs
- PropertyGeneratedEventArgs.cs
- AttachedPropertyBrowsableAttribute.cs
- RealProxy.cs
- NetStream.cs
- WebPartConnectionsCancelEventArgs.cs
- PolyLineSegment.cs
- SQLDecimalStorage.cs
- ConfigurationProperty.cs
- OdbcCommand.cs
- TextSpanModifier.cs
- SqlWriter.cs
- WorkItem.cs
- ZipArchive.cs
- CodePropertyReferenceExpression.cs
- GridViewUpdatedEventArgs.cs
- XXXOnTypeBuilderInstantiation.cs
- InputBinding.cs
- AttributeCollection.cs
- DataSourceXmlSerializationAttribute.cs
- SqlPersistenceProviderFactory.cs
- TreeNodeSelectionProcessor.cs
- ServiceReference.cs