Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Utils / Set.cs / 1305376 / Set.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // Util.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; namespace System.Linq.Parallel { ////// A set for various operations. Shamelessly stolen from LINQ's source code. /// @ internal class Set { int[] buckets; Slot[] slots; int count; int freeList; IEqualityComparer comparer; internal Set() : this(null) { } internal Set(IEqualityComparer comparer) { if (comparer == null) comparer = EqualityComparer .Default; this.comparer = comparer; buckets = new int[7]; slots = new Slot[7]; freeList = -1; } // If value is not in set, add it and return true; otherwise return false internal bool Add(TElement value) { return !Find(value, true); } // Check whether value is in set internal bool Contains(TElement value) { return Find(value, false); } // If value is in set, remove it and return true; otherwise return false internal bool Remove(TElement value) { int hashCode = comparer.GetHashCode(value) & 0x7FFFFFFF; int bucket = hashCode % buckets.Length; int last = -1; for (int i = buckets[bucket] - 1; i >= 0; last = i, i = slots[i].next) { if (slots[i].hashCode == hashCode && comparer.Equals(slots[i].value, value)) { if (last < 0) { buckets[bucket] = slots[i].next + 1; } else { slots[last].next = slots[i].next; } slots[i].hashCode = -1; slots[i].value = default(TElement); slots[i].next = freeList; freeList = i; return true; } } return false; } internal bool Find(TElement value, bool add) { int hashCode = comparer.GetHashCode(value) & 0x7FFFFFFF; for (int i = buckets[hashCode % buckets.Length] - 1; i >= 0; i = slots[i].next) { if (slots[i].hashCode == hashCode && comparer.Equals(slots[i].value, value)) return true; } if (add) { int index; if (freeList >= 0) { index = freeList; freeList = slots[index].next; } else { if (count == slots.Length) Resize(); index = count; count++; } int bucket = hashCode % buckets.Length; slots[index].hashCode = hashCode; slots[index].value = value; slots[index].next = buckets[bucket] - 1; buckets[bucket] = index + 1; } return false; } void Resize() { int newSize = checked(count * 2 + 1); int[] newBuckets = new int[newSize]; Slot[] newSlots = new Slot[newSize]; Array.Copy(slots, 0, newSlots, 0, count); for (int i = 0; i < count; i++) { int bucket = newSlots[i].hashCode % newSize; newSlots[i].next = newBuckets[bucket] - 1; newBuckets[bucket] = i + 1; } buckets = newBuckets; slots = newSlots; } internal struct Slot { internal int hashCode; internal TElement value; internal int next; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
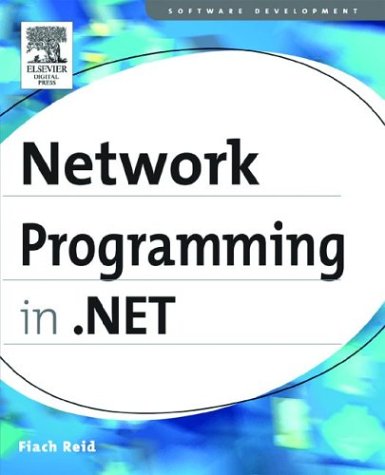
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseTreeIterator.cs
- safemediahandle.cs
- CompatibleIComparer.cs
- FontStyles.cs
- WebPartsSection.cs
- CfgSemanticTag.cs
- ILGenerator.cs
- RegistrationServices.cs
- CacheHelper.cs
- BidPrivateBase.cs
- SignatureResourcePool.cs
- AttributedMetaModel.cs
- Italic.cs
- EdmItemCollection.cs
- ParameterElementCollection.cs
- FileVersion.cs
- DeviceOverridableAttribute.cs
- ListControlConvertEventArgs.cs
- ReadingWritingEntityEventArgs.cs
- PartitionResolver.cs
- Section.cs
- CachedFontFamily.cs
- BooleanSwitch.cs
- RestHandlerFactory.cs
- CellParagraph.cs
- XmlAnyAttributeAttribute.cs
- TreeViewAutomationPeer.cs
- TextOnlyOutput.cs
- TextCompositionEventArgs.cs
- AttributeParameterInfo.cs
- SrgsDocumentParser.cs
- Window.cs
- ColorMatrix.cs
- Activator.cs
- ServiceModelTimeSpanValidator.cs
- ScriptingJsonSerializationSection.cs
- InplaceBitmapMetadataWriter.cs
- SecureConversationVersion.cs
- StateRuntime.cs
- Tile.cs
- MaterialGroup.cs
- ClientConfigurationSystem.cs
- SHA256Cng.cs
- HandlerFactoryCache.cs
- DataServiceClientException.cs
- DeclarativeCatalogPart.cs
- EnglishPluralizationService.cs
- SecureEnvironment.cs
- SetStoryboardSpeedRatio.cs
- OleDbConnection.cs
- NetWebProxyFinder.cs
- ResXResourceWriter.cs
- WindowsGraphicsCacheManager.cs
- WebSysDisplayNameAttribute.cs
- ValueTypeFixupInfo.cs
- XamlPathDataSerializer.cs
- XmlSchemaAttributeGroupRef.cs
- Literal.cs
- AutomationPropertyInfo.cs
- WindowsContainer.cs
- RemotingConfiguration.cs
- XmlSchemaAttributeGroup.cs
- CodeBinaryOperatorExpression.cs
- MarkupExtensionReturnTypeAttribute.cs
- NativeMethods.cs
- InstanceBehavior.cs
- LinqDataSourceInsertEventArgs.cs
- SessionStateContainer.cs
- VirtualizingPanel.cs
- DataControlButton.cs
- HelpKeywordAttribute.cs
- QuerySettings.cs
- LogStream.cs
- BuildProvidersCompiler.cs
- FolderBrowserDialogDesigner.cs
- MatrixUtil.cs
- WindowsFormsLinkLabel.cs
- smtppermission.cs
- SymLanguageVendor.cs
- ScrollViewerAutomationPeer.cs
- BoolLiteral.cs
- XPathExpr.cs
- ObjectCloneHelper.cs
- DoubleAnimationClockResource.cs
- XhtmlBasicListAdapter.cs
- Ports.cs
- Transform3D.cs
- _SslState.cs
- UserControlParser.cs
- StrokeDescriptor.cs
- FileRecordSequence.cs
- PathFigureCollectionValueSerializer.cs
- TraceHandlerErrorFormatter.cs
- webbrowsersite.cs
- ConstraintEnumerator.cs
- QuaternionIndependentAnimationStorage.cs
- DocumentAutomationPeer.cs
- ValidationPropertyAttribute.cs
- PerformanceCounterPermissionAttribute.cs
- SubclassTypeValidatorAttribute.cs