Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WebForms / System / Web / UI / Design / WebFormsRootDesigner.cs / 1 / WebFormsRootDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design { using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Design; using System.Diagnostics; using System.Drawing; using System.Drawing.Design; using System.Globalization; using System.Resources; using System.Web.Compilation; using System.Web.UI; ////// /// public abstract class WebFormsRootDesigner : IRootDesigner, IDesignerFilter { private const string dummyProtocolAndServer = "file://foo"; private IComponent _component; private EventHandler _loadCompleteHandler; private IUrlResolutionService _urlResolutionService; private DesignerActionService _designerActionService; private DesignerActionUIService _designerActionUIService; private IImplicitResourceProvider _implicitResourceProvider; ////// /// public virtual IComponent Component { get { return _component; } set { _component = value; } } ///~WebFormsRootDesigner() { Dispose(false); } public CultureInfo CurrentCulture { get { return CultureInfo.CurrentCulture; } } /// /// /// public abstract string DocumentUrl { get; } ////// Returns whether the designer view is locked, keeping controls from being added. /// public abstract bool IsDesignerViewLocked { get; } ////// /// public abstract bool IsLoading { get; } ///public abstract WebFormsReferenceManager ReferenceManager { get; } protected ViewTechnology[] SupportedTechnologies { get { return new ViewTechnology[] { ViewTechnology.Default }; } } protected DesignerVerbCollection Verbs { get { return new DesignerVerbCollection(); } } /// /// /// protected internal virtual object GetService(Type serviceType) { if (_component != null) { ISite site = _component.Site; if (site != null) { return site.GetService(serviceType); } } return null; } protected object GetView(ViewTechnology viewTechnology) { return null; } ////// /// public event EventHandler LoadComplete { add { _loadCompleteHandler = (EventHandler)Delegate.Combine(_loadCompleteHandler, value); } remove { _loadCompleteHandler = (EventHandler)Delegate.Remove(_loadCompleteHandler, value); } } public abstract void AddClientScriptToDocument(ClientScriptItem scriptItem); ////// /// public abstract string AddControlToDocument(Control newControl, Control referenceControl, ControlLocation location); protected virtual DesignerActionService CreateDesignerActionService(IServiceProvider serviceProvider) { return new WebFormsDesignerActionService(serviceProvider); } ////// /// protected virtual IUrlResolutionService CreateUrlResolutionService() { return new UrlResolutionService(this); } ////// /// protected virtual void Dispose(bool disposing) { if (disposing) { IPropertyValueUIService propUIService = (IPropertyValueUIService)GetService(typeof(IPropertyValueUIService)); if (propUIService != null) { propUIService.RemovePropertyValueUIHandler(new PropertyValueUIHandler(OnGetUIValueItem)); } IServiceContainer serviceContainer = (IServiceContainer)GetService(typeof(IServiceContainer)); if (serviceContainer != null) { if (_urlResolutionService != null) { serviceContainer.RemoveService(typeof(IUrlResolutionService)); } serviceContainer.RemoveService(typeof(IImplicitResourceProvider)); if (_designerActionService != null) { _designerActionService.Dispose(); } _designerActionUIService.Dispose(); } _urlResolutionService = null; _component = null; } } ////// /// public virtual string GenerateEmptyDesignTimeHtml(Control control) { return GenerateErrorDesignTimeHtml(control, null, String.Empty); } ////// /// public virtual string GenerateErrorDesignTimeHtml(Control control, Exception e, string errorMessage) { string name = control.Site.Name; if (errorMessage == null) { errorMessage = String.Empty; } else { errorMessage = HttpUtility.HtmlEncode(errorMessage); } if (e != null) { errorMessage += "
" + HttpUtility.HtmlEncode(e.Message); } return String.Format(CultureInfo.InvariantCulture, ControlDesigner.ErrorDesignTimeHtmlTemplate, SR.GetString(SR.ControlDesigner_DesignTimeHtmlError), HttpUtility.HtmlEncode(name), errorMessage); } public abstract ClientScriptItemCollection GetClientScriptsInDocument(); ////// /// protected internal abstract void GetControlViewAndTag(Control control, out IControlDesignerView view, out IControlDesignerTag tag); ////// /// public virtual void Initialize(IComponent component) { ControlDesigner.VerifyInitializeArgument(component, typeof(TemplateControl)); _component = component; IServiceContainer serviceContainer = (IServiceContainer)GetService(typeof(IServiceContainer)); if (serviceContainer != null) { _urlResolutionService = CreateUrlResolutionService(); if (_urlResolutionService != null) { serviceContainer.AddService(typeof(IUrlResolutionService), _urlResolutionService); } _designerActionService = CreateDesignerActionService(_component.Site); Debug.Assert(_designerActionService != null, "Did not expecte CreateDesignerActionService to return null."); _designerActionUIService = new DesignerActionUIService(_component.Site); // Demand create the IImplicitResourceProvider service. ServiceCreatorCallback callback = new ServiceCreatorCallback(this.OnCreateService); serviceContainer.AddService(typeof(IImplicitResourceProvider), callback); } IPropertyValueUIService propUIService = (IPropertyValueUIService)GetService(typeof(IPropertyValueUIService)); if (propUIService != null) { propUIService.AddPropertyValueUIHandler(new PropertyValueUIHandler(OnGetUIValueItem)); } } ////// /// Demand creates some of the more infrequently used services we offer. /// private object OnCreateService(IServiceContainer container, Type serviceType) { if (serviceType == typeof(IImplicitResourceProvider)) { if (_implicitResourceProvider == null) { DesignTimeResourceProviderFactory designTimeProvider = ControlDesigner.GetDesignTimeResourceProviderFactory(Component.Site); IResourceProvider resProvider = designTimeProvider.CreateDesignTimeLocalResourceProvider(Component.Site); _implicitResourceProvider = resProvider as IImplicitResourceProvider; if (_implicitResourceProvider == null) { _implicitResourceProvider = new ImplicitResourceProvider(this); } } return _implicitResourceProvider; } Debug.Fail("Service type " + serviceType.FullName + " requested but we don't support it"); return null; } ////// /// private void OnGetUIValueItem(ITypeDescriptorContext context, PropertyDescriptor propDesc, ArrayList valueUIItemList) { // This only supports top-level properties. Properties such as Font.Bold // are not supported because there is no way to detect the parent chain // of ownership of complex properties. Control ctrl = context.Instance as Control; if (ctrl != null) { IDataBindingsAccessor dbAcc = (IDataBindingsAccessor)ctrl; if (dbAcc.HasDataBindings) { DataBinding db = dbAcc.DataBindings[propDesc.Name]; if (db != null) { valueUIItemList.Add(new DataBindingUIItem()); } } IExpressionsAccessor expAcc = (IExpressionsAccessor)ctrl; if (expAcc.HasExpressions) { ExpressionBinding eb = expAcc.Expressions[propDesc.Name]; if (eb != null) { if (eb.Generated) { valueUIItemList.Add(new ImplicitExpressionUIItem()); } else { valueUIItemList.Add(new ExpressionBindingUIItem()); } } } } } ////// /// protected virtual void OnLoadComplete(EventArgs e) { if (_loadCompleteHandler != null) { _loadCompleteHandler(this, e); } } ////// /// Allows a /// designer to filter the set of member attributes the /// component it is designing will expose through the /// TypeDescriptor object. /// protected virtual void PostFilterAttributes(IDictionary attributes) { } ////// /// Allows /// a designer to filter the set of events the /// component it is designing will expose through the /// TypeDescriptor object. /// protected virtual void PostFilterEvents(IDictionary events) { } ////// /// Allows /// a designer to filter the set of properties the /// component it is designing will expose through the /// TypeDescriptor object. /// protected virtual void PostFilterProperties(IDictionary properties) { } ////// /// Allows a designer /// to filter the set of member attributes the component /// it is designing will expose through the TypeDescriptor /// object. /// protected virtual void PreFilterAttributes(IDictionary attributes) { } ////// /// Allows a /// designer to filter the set of events the component /// it is designing will expose through the TypeDescriptor /// object. /// protected virtual void PreFilterEvents(IDictionary events) { } ////// /// Allows a /// designer to filter the set of properties the component /// it is designing will expose through the TypeDescriptor /// object. /// protected virtual void PreFilterProperties(IDictionary properties) { } public abstract void RemoveClientScriptFromDocument(string clientScriptId); public abstract void RemoveControlFromDocument(Control control); ////// /// public string ResolveUrl(string relativeUrl) { if (relativeUrl == null) { throw new ArgumentNullException("relativeUrl"); } string documentUrl = DocumentUrl; if ((documentUrl == null) || (documentUrl.Length == 0) || IsAppRelativePath(relativeUrl) || IsRooted(relativeUrl) || !IsAppRelativePath(documentUrl)) { // If there's no documentUrl or the path is already appRelative or the path is rooted or // the document URL (invalid) isn't app-relative, just return what they gave us return relativeUrl; } // Give this a fake protocol and server so Uri can resolve it documentUrl = documentUrl.Replace("~", dummyProtocolAndServer); #pragma warning disable 618 Uri docUri = new Uri(documentUrl, true); #pragma warning restore 618 Uri resolvedUri = new Uri(docUri, relativeUrl); string resolvedUrl = resolvedUri.ToString(); resolvedUrl = resolvedUrl.Replace(dummyProtocolAndServer, "~"); return resolvedUrl; } public virtual void SetControlID(Control control, string id) { ISite site = control.Site; site.Name = id; control.ID = id.Trim(); } #region Copied from UrlPath.cs private const char appRelativeCharacter = '~'; private static bool IsRooted(String basepath) { return(basepath == null || basepath.Length == 0 || basepath[0] == '/' || basepath[0] == '\\'); } private static bool IsAppRelativePath(string path) { return (path.Length >= 2 && path[0] == appRelativeCharacter && (path[1] == '/' || path[1] == '\\')); } #endregion #region IDesigner private implementation ////// /// DesignerVerbCollection IDesigner.Verbs { get { return Verbs; } } ////// /// void IDesigner.DoDefaultAction() { } #endregion #region IDesignerFilter implementation ////// /// /// Allows a designer to filter the set of /// attributes the component being designed will expose through the void IDesignerFilter.PostFilterAttributes(IDictionary attributes) { PostFilterAttributes(attributes); } ///object. /// /// /// /// Allows a designer to filter the set of events /// the component being designed will expose through the void IDesignerFilter.PostFilterEvents(IDictionary events) { PostFilterEvents(events); } ////// object. /// /// /// /// Allows a designer to filter the set of properties /// the component being designed will expose through the void IDesignerFilter.PostFilterProperties(IDictionary properties) { PostFilterProperties(properties); } ////// object. /// /// /// /// Allows a designer to filter the set of /// attributes the component being designed will expose through the void IDesignerFilter.PreFilterAttributes(IDictionary attributes) { PreFilterAttributes(attributes); } ////// object. /// /// /// /// Allows a designer to filter the set of events /// the component being designed will expose through the void IDesignerFilter.PreFilterEvents(IDictionary events) { PreFilterEvents(events); } ////// object. /// /// /// /// Allows a designer to filter the set of properties /// the component being designed will expose through the void IDesignerFilter.PreFilterProperties(IDictionary properties) { PreFilterProperties(properties); } #endregion #region IDisposable implementation ////// object. /// void IDisposable.Dispose() { Dispose(true); GC.SuppressFinalize(this); } #endregion #region IRootDesigner implementation /// /// ViewTechnology[] IRootDesigner.SupportedTechnologies { get { return SupportedTechnologies; } } /// /// object IRootDesigner.GetView(ViewTechnology viewTechnology) { return GetView(viewTechnology); } #endregion /// /// private sealed class DataBindingUIItem : PropertyValueUIItem { private static Bitmap _dataBindingBitmap; private static string _dataBindingToolTip; public DataBindingUIItem() : base(DataBindingUIItem.DataBindingBitmap, new PropertyValueUIItemInvokeHandler(OnValueUIItemInvoke), DataBindingUIItem.DataBindingToolTip) { } private static Bitmap DataBindingBitmap { get { if (_dataBindingBitmap == null) { _dataBindingBitmap = new Bitmap(typeof(WebFormsRootDesigner), "DataBindingGlyph.bmp"); _dataBindingBitmap.MakeTransparent(Color.Fuchsia); } return _dataBindingBitmap; } } private static string DataBindingToolTip { get { if (_dataBindingToolTip == null) { _dataBindingToolTip = SR.GetString(SR.DataBindingGlyph_ToolTip); } return _dataBindingToolTip; } } private static void OnValueUIItemInvoke(ITypeDescriptorContext context, PropertyDescriptor propDesc, PropertyValueUIItem invokedItem) { // No action is necessary when the icon is clicked } } ////// private sealed class ExpressionBindingUIItem : PropertyValueUIItem { private static Bitmap _expressionBindingBitmap; private static string _expressionBindingToolTip; public ExpressionBindingUIItem() : base(ExpressionBindingUIItem.ExpressionBindingBitmap, new PropertyValueUIItemInvokeHandler(OnValueUIItemInvoke), ExpressionBindingUIItem.ExpressionBindingToolTip) { } private static Bitmap ExpressionBindingBitmap { get { if (_expressionBindingBitmap == null) { _expressionBindingBitmap = new Bitmap(typeof(WebFormsRootDesigner), "ExpressionBindingGlyph.bmp"); _expressionBindingBitmap.MakeTransparent(Color.Fuchsia); } return _expressionBindingBitmap; } } private static string ExpressionBindingToolTip { get { if (_expressionBindingToolTip == null) { _expressionBindingToolTip = SR.GetString(SR.ExpressionBindingGlyph_ToolTip); } return _expressionBindingToolTip; } } private static void OnValueUIItemInvoke(ITypeDescriptorContext context, PropertyDescriptor propDesc, PropertyValueUIItem invokedItem) { // No action is necessary when the icon is clicked } } ////// private sealed class ImplicitExpressionUIItem : PropertyValueUIItem { private static Bitmap _expressionBindingBitmap; private static string _expressionBindingToolTip; public ImplicitExpressionUIItem() : base(ImplicitExpressionUIItem.ImplicitExpressionBindingBitmap, new PropertyValueUIItemInvokeHandler(OnValueUIItemInvoke), ImplicitExpressionUIItem.ImplicitExpressionBindingToolTip) { } private static Bitmap ImplicitExpressionBindingBitmap { get { if (_expressionBindingBitmap == null) { _expressionBindingBitmap = new Bitmap(typeof(WebFormsRootDesigner), "ImplicitExpressionBindingGlyph.bmp"); _expressionBindingBitmap.MakeTransparent(Color.Fuchsia); } return _expressionBindingBitmap; } } private static string ImplicitExpressionBindingToolTip { get { if (_expressionBindingToolTip == null) { _expressionBindingToolTip = SR.GetString(SR.ImplicitExpressionBindingGlyph_ToolTip); } return _expressionBindingToolTip; } } private static void OnValueUIItemInvoke(ITypeDescriptorContext context, PropertyDescriptor propDesc, PropertyValueUIItem invokedItem) { // No action is necessary when the icon is clicked } } ////// private sealed class UrlResolutionService : IUrlResolutionService { private WebFormsRootDesigner _owner; public UrlResolutionService(WebFormsRootDesigner owner) { _owner = owner; } string IUrlResolutionService.ResolveClientUrl(string relativeUrl) { if (relativeUrl == null) { throw new ArgumentNullException("relativeUrl"); } if (!IsAppRelativePath(relativeUrl)) { return relativeUrl; } string documentUrl = _owner.DocumentUrl; if ((documentUrl == null) || (documentUrl.Length == 0) || !IsAppRelativePath(documentUrl)) { // If there is no documentUrl or it isn't app-relative, // trim off the ~/ to make our best guess. return relativeUrl.Substring(2); } // Give this a fake protocol and server so Uri can resolve it documentUrl = documentUrl.Replace("~", dummyProtocolAndServer); #pragma warning disable 618 Uri docUri = new Uri(documentUrl, true); #pragma warning restore 618 // Give this a fake protocol and server so Uri can resolve it relativeUrl = relativeUrl.Replace("~", dummyProtocolAndServer); #pragma warning disable 618 Uri relativeUri = new Uri(relativeUrl, true); #pragma warning restore 618 string resultUrl = docUri.MakeRelativeUri(relativeUri).ToString(); // In the case that relativeUri and docUri can't be made relative // MakeRelative just returns relatveUri, so we need to remove the // dummyProcotolAndServer return resultUrl.Replace(dummyProtocolAndServer, String.Empty); } } private sealed class ImplicitResourceProvider : IImplicitResourceProvider { private WebFormsRootDesigner _owner; public ImplicitResourceProvider(WebFormsRootDesigner owner) { _owner = owner; } object IImplicitResourceProvider.GetObject(ImplicitResourceKey key, CultureInfo culture) { throw new NotSupportedException(); } ICollection IImplicitResourceProvider.GetImplicitResourceKeys(string keyPrefix) { IDictionary pageResources = GetPageResources(); return pageResources[keyPrefix] as ICollection; } private IDictionary GetPageResources() { if (_owner.Component == null) { return null; } IServiceProvider serviceProvider = _owner.Component.Site; if (serviceProvider == null) { return null; } DesignTimeResourceProviderFactory resourceProviderFactory = ControlDesigner.GetDesignTimeResourceProviderFactory(serviceProvider); if (resourceProviderFactory == null) { return null; } IResourceProvider resProvider = resourceProviderFactory.CreateDesignTimeLocalResourceProvider(serviceProvider); if (resProvider == null) { return null; } IResourceReader resReader = resProvider.ResourceReader; if (resReader == null) { return null; } IDictionary pageResources = new HybridDictionary(true); if (resReader != null) { // foreach (DictionaryEntry entry in resReader) { string key = (string)entry.Key; string filter = String.Empty; // A page resource key looks like [myfilter:]MyResKey.MyProp[.MySubProp] // Check if there is a filter if (key.IndexOf(':') > 0) { string[] parts = key.Split(':'); // Shouldn't be multiple ':'. If there is, ignore it if (parts.Length > 2) continue; filter = parts[0]; key = parts[1]; } int periodIndex = key.IndexOf('.'); // There should be at least one period, for the meta:resourcekey part. If not, ignore. if (periodIndex <= 0) continue; string resourceKey = key.Substring(0, periodIndex); // The rest of the string is the property (e.g. MyProp.MySubProp) string property = key.Substring(periodIndex + 1); // Check if we already have an entry for this resource key ArrayList controlResources = (ArrayList)pageResources[resourceKey]; // If not, create one if (controlResources == null) { controlResources = new ArrayList(); pageResources[resourceKey] = controlResources; } // Add an entry in the ArrayList for this property ImplicitResourceKey resKeyEntry = new ImplicitResourceKey(); resKeyEntry.Filter = filter; resKeyEntry.Property = property; resKeyEntry.KeyPrefix = resourceKey; controlResources.Add(resKeyEntry); } } return pageResources; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
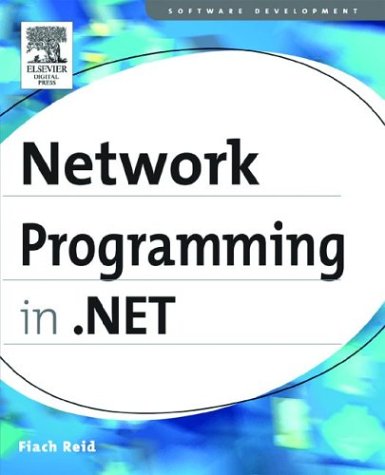
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ActivityContext.cs
- StoreAnnotationsMap.cs
- XmlIgnoreAttribute.cs
- XPathChildIterator.cs
- FixedSOMTableRow.cs
- HtmlFormParameterReader.cs
- ModelFunctionTypeElement.cs
- SecurityStandardsManager.cs
- CapabilitiesUse.cs
- Vector3DKeyFrameCollection.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- StatusBar.cs
- Oid.cs
- SqlConnectionPoolGroupProviderInfo.cs
- HtmlInputControl.cs
- ModuleConfigurationInfo.cs
- PagedControl.cs
- GridViewActionList.cs
- MSAAEventDispatcher.cs
- NameTable.cs
- ProfileInfo.cs
- storepermission.cs
- DoubleConverter.cs
- Decorator.cs
- MemoryPressure.cs
- WebBrowserDocumentCompletedEventHandler.cs
- SessionStateUtil.cs
- OperatingSystem.cs
- DefaultWorkflowSchedulerService.cs
- MatcherBuilder.cs
- InfocardExtendedInformationCollection.cs
- HtmlLinkAdapter.cs
- HiddenFieldPageStatePersister.cs
- CollectionContainer.cs
- FlowLayout.cs
- DataTableReader.cs
- MemberInitExpression.cs
- LinqDataSourceContextData.cs
- Enumerable.cs
- FileDialogCustomPlace.cs
- Window.cs
- ClientSettingsSection.cs
- JavaScriptSerializer.cs
- WinEventWrap.cs
- ScrollChrome.cs
- ClearTypeHintValidation.cs
- CannotUnloadAppDomainException.cs
- XmlSchemaExporter.cs
- Peer.cs
- FacetChecker.cs
- DateTimeFormat.cs
- DbFunctionCommandTree.cs
- RoutingExtensionElement.cs
- IpcManager.cs
- ADRoleFactoryConfiguration.cs
- BorderGapMaskConverter.cs
- DotExpr.cs
- Authorization.cs
- ElementInit.cs
- ZoneIdentityPermission.cs
- PeerApplicationLaunchInfo.cs
- HtmlSelect.cs
- WebBrowserSiteBase.cs
- BindingExpressionUncommonField.cs
- LineUtil.cs
- CatalogUtil.cs
- URL.cs
- Wildcard.cs
- WindowsFormsHostAutomationPeer.cs
- Module.cs
- VisualTreeHelper.cs
- RightsManagementEncryptedStream.cs
- SystemDiagnosticsSection.cs
- OracleRowUpdatedEventArgs.cs
- Convert.cs
- LocalizableAttribute.cs
- RunWorkerCompletedEventArgs.cs
- ServiceModelSectionGroup.cs
- ScrollProviderWrapper.cs
- CheckBoxPopupAdapter.cs
- WindowsAuthenticationEventArgs.cs
- UInt16Storage.cs
- PerformanceCounterPermission.cs
- TagPrefixCollection.cs
- Util.cs
- XmlEntityReference.cs
- ListViewCommandEventArgs.cs
- SingleAnimationBase.cs
- AudioFileOut.cs
- InvalidOperationException.cs
- ApplicationId.cs
- FixedSOMFixedBlock.cs
- TargetControlTypeAttribute.cs
- BaseAddressPrefixFilterElement.cs
- PageVisual.cs
- ChtmlTextWriter.cs
- DataListItemEventArgs.cs
- BitmapEffectInputData.cs
- embossbitmapeffect.cs
- InheritedPropertyChangedEventArgs.cs