Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Configuration / System / Configuration / AppSettingsSection.cs / 1305376 / AppSettingsSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; public sealed class AppSettingsSection : ConfigurationSection { private static ConfigurationPropertyCollection s_properties; private static ConfigurationProperty s_propAppSettings; private static ConfigurationProperty s_propFile; private KeyValueInternalCollection _KeyValueCollection = null; private static ConfigurationPropertyCollection EnsureStaticPropertyBag() { if (s_properties == null) { s_propAppSettings = new ConfigurationProperty(null, typeof(KeyValueConfigurationCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); s_propFile = new ConfigurationProperty("file", typeof(string), String.Empty, ConfigurationPropertyOptions.None); ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); properties.Add(s_propAppSettings); properties.Add(s_propFile); s_properties = properties; } return s_properties; } public AppSettingsSection() { EnsureStaticPropertyBag(); } protected internal override ConfigurationPropertyCollection Properties { get { return EnsureStaticPropertyBag(); } } protected internal override object GetRuntimeObject() { SetReadOnly(); return this.InternalSettings; // return the read only object } internal NameValueCollection InternalSettings { get { if (_KeyValueCollection == null) { _KeyValueCollection = new KeyValueInternalCollection(this); } return (NameValueCollection)_KeyValueCollection; } } [ConfigurationProperty("", IsDefaultCollection = true)] public KeyValueConfigurationCollection Settings { get { return (KeyValueConfigurationCollection)base[s_propAppSettings]; } } [ConfigurationProperty("file", DefaultValue = "")] public string File { get { string fileValue = (string)base[s_propFile]; if (fileValue == null) { return String.Empty; } return fileValue; } set { base[s_propFile] = value; } } protected internal override void Reset(ConfigurationElement parentSection) { _KeyValueCollection = null; base.Reset(parentSection); if (!String.IsNullOrEmpty((string)base[s_propFile])) { // don't inherit from the parent SetPropertyValue(s_propFile,null,true); // ignore the lock to prevent inheritence } } protected internal override bool IsModified() { return base.IsModified(); } protected internal override string SerializeSection(ConfigurationElement parentElement, string name, ConfigurationSaveMode saveMode) { return base.SerializeSection(parentElement, name, saveMode); } protected internal override void DeserializeElement(XmlReader reader, bool serializeCollectionKey) { string ElementName = reader.Name; base.DeserializeElement(reader, serializeCollectionKey); if ((File != null) && (File.Length > 0)) { string sourceFileFullPath; string configFileDirectory; string configFile; // Determine file location configFile = ElementInformation.Source; if (String.IsNullOrEmpty(configFile)) { sourceFileFullPath = File; } else { configFileDirectory = System.IO.Path.GetDirectoryName(configFile); sourceFileFullPath = System.IO.Path.Combine(configFileDirectory, File); } if (System.IO.File.Exists(sourceFileFullPath)) { int lineOffset = 0; string rawXml = null; using (Stream sourceFileStream = new FileStream(sourceFileFullPath, FileMode.Open, FileAccess.Read, FileShare.Read)) { using (XmlUtil xmlUtil = new XmlUtil(sourceFileStream, sourceFileFullPath, true)) { if (xmlUtil.Reader.Name != ElementName) { throw new ConfigurationErrorsException( SR.GetString(SR.Config_name_value_file_section_file_invalid_root, ElementName), xmlUtil); } lineOffset = xmlUtil.Reader.LineNumber; rawXml = xmlUtil.CopySection(); // Detect if there is any XML left over after the section while (!xmlUtil.Reader.EOF) { XmlNodeType t = xmlUtil.Reader.NodeType; if (t != XmlNodeType.Comment) { throw new ConfigurationErrorsException(SR.GetString(SR.Config_source_file_format), xmlUtil); } xmlUtil.Reader.Read(); } } } ConfigXmlReader internalReader = new ConfigXmlReader(rawXml, sourceFileFullPath, lineOffset); internalReader.Read(); if (internalReader.MoveToNextAttribute()) { throw new ConfigurationErrorsException(SR.GetString(SR.Config_base_unrecognized_attribute, internalReader.Name), (XmlReader)internalReader); } internalReader.MoveToElement(); base.DeserializeElement(internalReader, serializeCollectionKey); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; public sealed class AppSettingsSection : ConfigurationSection { private static ConfigurationPropertyCollection s_properties; private static ConfigurationProperty s_propAppSettings; private static ConfigurationProperty s_propFile; private KeyValueInternalCollection _KeyValueCollection = null; private static ConfigurationPropertyCollection EnsureStaticPropertyBag() { if (s_properties == null) { s_propAppSettings = new ConfigurationProperty(null, typeof(KeyValueConfigurationCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); s_propFile = new ConfigurationProperty("file", typeof(string), String.Empty, ConfigurationPropertyOptions.None); ConfigurationPropertyCollection properties = new ConfigurationPropertyCollection(); properties.Add(s_propAppSettings); properties.Add(s_propFile); s_properties = properties; } return s_properties; } public AppSettingsSection() { EnsureStaticPropertyBag(); } protected internal override ConfigurationPropertyCollection Properties { get { return EnsureStaticPropertyBag(); } } protected internal override object GetRuntimeObject() { SetReadOnly(); return this.InternalSettings; // return the read only object } internal NameValueCollection InternalSettings { get { if (_KeyValueCollection == null) { _KeyValueCollection = new KeyValueInternalCollection(this); } return (NameValueCollection)_KeyValueCollection; } } [ConfigurationProperty("", IsDefaultCollection = true)] public KeyValueConfigurationCollection Settings { get { return (KeyValueConfigurationCollection)base[s_propAppSettings]; } } [ConfigurationProperty("file", DefaultValue = "")] public string File { get { string fileValue = (string)base[s_propFile]; if (fileValue == null) { return String.Empty; } return fileValue; } set { base[s_propFile] = value; } } protected internal override void Reset(ConfigurationElement parentSection) { _KeyValueCollection = null; base.Reset(parentSection); if (!String.IsNullOrEmpty((string)base[s_propFile])) { // don't inherit from the parent SetPropertyValue(s_propFile,null,true); // ignore the lock to prevent inheritence } } protected internal override bool IsModified() { return base.IsModified(); } protected internal override string SerializeSection(ConfigurationElement parentElement, string name, ConfigurationSaveMode saveMode) { return base.SerializeSection(parentElement, name, saveMode); } protected internal override void DeserializeElement(XmlReader reader, bool serializeCollectionKey) { string ElementName = reader.Name; base.DeserializeElement(reader, serializeCollectionKey); if ((File != null) && (File.Length > 0)) { string sourceFileFullPath; string configFileDirectory; string configFile; // Determine file location configFile = ElementInformation.Source; if (String.IsNullOrEmpty(configFile)) { sourceFileFullPath = File; } else { configFileDirectory = System.IO.Path.GetDirectoryName(configFile); sourceFileFullPath = System.IO.Path.Combine(configFileDirectory, File); } if (System.IO.File.Exists(sourceFileFullPath)) { int lineOffset = 0; string rawXml = null; using (Stream sourceFileStream = new FileStream(sourceFileFullPath, FileMode.Open, FileAccess.Read, FileShare.Read)) { using (XmlUtil xmlUtil = new XmlUtil(sourceFileStream, sourceFileFullPath, true)) { if (xmlUtil.Reader.Name != ElementName) { throw new ConfigurationErrorsException( SR.GetString(SR.Config_name_value_file_section_file_invalid_root, ElementName), xmlUtil); } lineOffset = xmlUtil.Reader.LineNumber; rawXml = xmlUtil.CopySection(); // Detect if there is any XML left over after the section while (!xmlUtil.Reader.EOF) { XmlNodeType t = xmlUtil.Reader.NodeType; if (t != XmlNodeType.Comment) { throw new ConfigurationErrorsException(SR.GetString(SR.Config_source_file_format), xmlUtil); } xmlUtil.Reader.Read(); } } } ConfigXmlReader internalReader = new ConfigXmlReader(rawXml, sourceFileFullPath, lineOffset); internalReader.Read(); if (internalReader.MoveToNextAttribute()) { throw new ConfigurationErrorsException(SR.GetString(SR.Config_base_unrecognized_attribute, internalReader.Name), (XmlReader)internalReader); } internalReader.MoveToElement(); base.DeserializeElement(internalReader, serializeCollectionKey); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
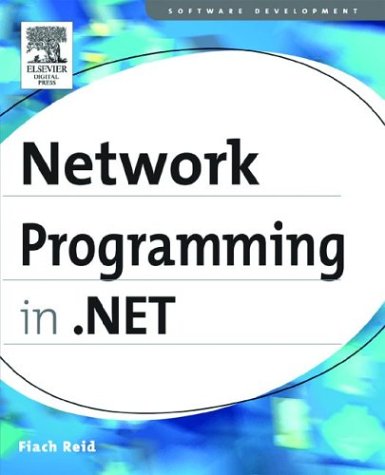
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DefaultAssemblyResolver.cs
- DataGridViewCellStyleChangedEventArgs.cs
- XmlHierarchyData.cs
- TextModifier.cs
- ClientConfigurationSystem.cs
- EntitySqlQueryCacheEntry.cs
- xdrvalidator.cs
- Char.cs
- FileDialogCustomPlacesCollection.cs
- BindingWorker.cs
- DataGridViewLinkCell.cs
- CircleHotSpot.cs
- XMLDiffLoader.cs
- DiffuseMaterial.cs
- UnsettableComboBox.cs
- TdsParserSessionPool.cs
- GroupDescription.cs
- AccessDataSource.cs
- _KerberosClient.cs
- odbcmetadatacolumnnames.cs
- X509ChainElement.cs
- SiteMapPath.cs
- DnsEndPoint.cs
- ContentControl.cs
- BrowserDefinitionCollection.cs
- TreeView.cs
- UserControlBuildProvider.cs
- AccessKeyManager.cs
- ConfigUtil.cs
- TextLineResult.cs
- SqlCacheDependency.cs
- DynamicValueConverter.cs
- ObjectListFieldCollection.cs
- WebException.cs
- ChangePassword.cs
- UrlMappingsModule.cs
- UnsafeNativeMethods.cs
- XmlSignatureProperties.cs
- HybridObjectCache.cs
- XmlSchemas.cs
- PriorityBinding.cs
- HttpApplicationFactory.cs
- QuotedStringWriteStateInfo.cs
- FileStream.cs
- DecoderNLS.cs
- PrivilegedConfigurationManager.cs
- ZipIOCentralDirectoryBlock.cs
- ExceptionHandler.cs
- ZipIOBlockManager.cs
- ElementUtil.cs
- ToolStripItemTextRenderEventArgs.cs
- WebRequest.cs
- WindowsComboBox.cs
- XamlWriter.cs
- TextEditor.cs
- StringDictionaryCodeDomSerializer.cs
- EventSetter.cs
- WebHttpSecurityModeHelper.cs
- SourceLineInfo.cs
- DictionaryTraceRecord.cs
- BufferedGraphicsContext.cs
- HttpStreamMessageEncoderFactory.cs
- Overlapped.cs
- NotSupportedException.cs
- TextSimpleMarkerProperties.cs
- OleStrCAMarshaler.cs
- HttpFileCollection.cs
- TreeView.cs
- HttpPostedFile.cs
- TokenBasedSetEnumerator.cs
- UInt16.cs
- RelOps.cs
- ResetableIterator.cs
- cryptoapiTransform.cs
- UncommonField.cs
- Model3DGroup.cs
- RadioButtonAutomationPeer.cs
- PathFigureCollectionConverter.cs
- ReflectionPermission.cs
- EntityConnectionStringBuilder.cs
- PageRouteHandler.cs
- ColumnWidthChangingEvent.cs
- SelfIssuedAuthProofToken.cs
- CTreeGenerator.cs
- UnmanagedMemoryStreamWrapper.cs
- VectorAnimationUsingKeyFrames.cs
- WebPartCatalogCloseVerb.cs
- UserControlBuildProvider.cs
- IsolatedStorageFileStream.cs
- ReflectTypeDescriptionProvider.cs
- Expression.cs
- Rotation3DAnimationBase.cs
- ListViewSortEventArgs.cs
- XmlSchemaRedefine.cs
- NoResizeHandleGlyph.cs
- PerformanceCounterPermission.cs
- SelectorAutomationPeer.cs
- Delegate.cs
- ThreadNeutralSemaphore.cs
- PrintingPermission.cs