Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / ActivitiesCollection.cs / 1305376 / ActivitiesCollection.cs
namespace System.Workflow.ComponentModel { using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel.Design.Serialization; using System.Workflow.ComponentModel.Serialization; #region Class ActivityCollectionItemList [DesignerSerializer(typeof(ActivityCollectionMarkupSerializer), typeof(WorkflowMarkupSerializer))] public sealed class ActivityCollection : List, IList , IList { private Activity owner = null; internal event EventHandler ListChanging; public event EventHandler ListChanged; public ActivityCollection(Activity owner) { if (owner == null) throw new ArgumentNullException("owner"); if (!(owner is Activity)) throw new ArgumentException(SR.GetString(SR.Error_UnexpectedArgumentType, typeof(Activity).FullName), "owner"); this.owner = owner; } private void FireListChanging(ActivityCollectionChangeEventArgs eventArgs) { if (this.ListChanging != null) this.ListChanging(this, eventArgs); } private void FireListChanged(ActivityCollectionChangeEventArgs eventArgs) { if (this.ListChanged != null) this.ListChanged(this, eventArgs); } internal Activity Owner { get { return this.owner; } } internal void InnerAdd(Activity activity) { base.Add(activity); } #region IList Members void IList .RemoveAt(int index) { if (index < 0 || index >= base.Count) throw new ArgumentOutOfRangeException("Index"); Activity item = base[index]; ActivityCollectionChangeEventArgs args = new ActivityCollectionChangeEventArgs(index, item, null, this.owner, ActivityCollectionChangeAction.Remove); FireListChanging(args); base.RemoveAt(index); FireListChanged(args); } void IList .Insert(int index, Activity item) { if (index < 0 || index > base.Count) throw new ArgumentOutOfRangeException("index"); if (item == null) throw new ArgumentNullException("item"); ActivityCollectionChangeEventArgs args = new ActivityCollectionChangeEventArgs(index, null, item, this.owner, ActivityCollectionChangeAction.Add); FireListChanging(args); base.Insert(index, item); FireListChanged(args); } Activity IList .this[int index] { get { return base[index]; } set { if (value == null) throw new ArgumentNullException("item"); Activity oldItem = base[index]; ActivityCollectionChangeEventArgs args = new ActivityCollectionChangeEventArgs(index, oldItem, value, this.owner, ActivityCollectionChangeAction.Replace); FireListChanging(args); base[index] = value; FireListChanged(args); } } int IList .IndexOf(Activity item) { return base.IndexOf(item); } #endregion #region ICollection Members bool ICollection .IsReadOnly { get { return false; } } bool ICollection .Contains(Activity item) { return base.Contains(item); } bool ICollection .Remove(Activity item) { if (!base.Contains(item)) return false; int index = base.IndexOf(item); if (index >= 0) { ActivityCollectionChangeEventArgs args = new ActivityCollectionChangeEventArgs(index, item, null, this.owner, ActivityCollectionChangeAction.Remove); FireListChanging(args); base.Remove(item); FireListChanged(args); return true; } return false; } void ICollection .Clear() { ICollection children = base.GetRange(0, base.Count); ActivityCollectionChangeEventArgs args = new ActivityCollectionChangeEventArgs(-1, children, null, this.owner, ActivityCollectionChangeAction.Remove); FireListChanging(args); base.Clear(); FireListChanged(args); } void ICollection .Add(Activity item) { if (item == null) throw new ArgumentNullException("item"); ActivityCollectionChangeEventArgs args = new ActivityCollectionChangeEventArgs(base.Count, null, item, this.owner, ActivityCollectionChangeAction.Add); FireListChanging(args); base.Add(item); FireListChanged(args); } int ICollection .Count { get { return base.Count; } } void ICollection .CopyTo(Activity[] array, int arrayIndex) { base.CopyTo(array, arrayIndex); } #endregion #region IEnumerable Members IEnumerator IEnumerable .GetEnumerator() { return base.GetEnumerator(); } #endregion #region Member Implementations public new int Count { get { return ((ICollection )this).Count; } } public new void Add(Activity item) { ((IList )this).Add(item); } public new void Clear() { ((IList )this).Clear(); } public new void Insert(int index, Activity item) { ((IList )this).Insert(index, item); } public new bool Remove(Activity item) { return ((IList )this).Remove(item); } public new void RemoveAt(int index) { ((IList )this).RemoveAt(index); } public new Activity this[int index] { get { return ((IList )this)[index]; } set { ((IList )this)[index] = value; } } public Activity this[string key] { get { for (int index = 0; index < this.Count; index++) if ((this[index].Name.Equals(key) || this[index].QualifiedName.Equals(key))) return this[index]; return null; } } public new int IndexOf(Activity item) { return ((IList )this).IndexOf(item); } public new bool Contains(Activity item) { return ((IList )this).Contains(item); } public new IEnumerator GetEnumerator() { return ((IList )this).GetEnumerator(); } #endregion #region IList Members int IList.Add(object value) { if (!(value is Activity)) throw new Exception(SR.GetString(SR.Error_InvalidListItem, this.GetType().GetGenericArguments()[0].FullName)); ((IList )this).Add((Activity)value); return this.Count - 1; } void IList.Clear() { ((IList )this).Clear(); } bool IList.Contains(object value) { if (!(value is Activity)) throw new Exception(SR.GetString(SR.Error_InvalidListItem, this.GetType().GetGenericArguments()[0].FullName)); return (((IList )this).Contains((Activity)value)); } int IList.IndexOf(object value) { if (!(value is Activity)) throw new Exception(SR.GetString(SR.Error_InvalidListItem, this.GetType().GetGenericArguments()[0].FullName)); return ((IList )this).IndexOf((Activity)value); } void IList.Insert(int index, object value) { if (!(value is Activity)) throw new Exception(SR.GetString(SR.Error_InvalidListItem, this.GetType().GetGenericArguments()[0].FullName)); ((IList )this).Insert(index, (Activity)value); } bool IList.IsFixedSize { get { return false; } } bool IList.IsReadOnly { get { return ((IList )this).IsReadOnly; } } void IList.Remove(object value) { if (!(value is Activity)) throw new Exception(SR.GetString(SR.Error_InvalidListItem, this.GetType().GetGenericArguments()[0].FullName)); ((IList )this).Remove((Activity)value); } object IList.this[int index] { get { return ((IList )this)[index]; } set { if (!(value is Activity)) throw new Exception(SR.GetString(SR.Error_InvalidListItem, this.GetType().GetGenericArguments()[0].FullName)); ((IList )this)[index] = (Activity)value; } } #endregion #region ICollection Members void ICollection.CopyTo(Array array, int index) { for (int loop = 0; loop < this.Count; loop++) array.SetValue(this[loop], loop + index); } bool ICollection.IsSynchronized { get { return false; } } object ICollection.SyncRoot { get { return this; } } #endregion #region IEnumerable Members IEnumerator IEnumerable.GetEnumerator() { return (IEnumerator)((IList )this).GetEnumerator(); } #endregion } #endregion public enum ActivityCollectionChangeAction { Add = 0x00, Remove = 0x01, Replace = 0x02 } public sealed class ActivityCollectionChangeEventArgs: EventArgs { private int index = 0; private ICollection addedItems = null; private ICollection removedItems = null; private object owner = null; private ActivityCollectionChangeAction action = ActivityCollectionChangeAction.Add; public ActivityCollectionChangeEventArgs(int index, ICollection removedItems, ICollection addedItems, object owner, ActivityCollectionChangeAction action) { this.index = index; this.removedItems = removedItems; this.addedItems = addedItems; this.action = action; this.owner = owner; } public ActivityCollectionChangeEventArgs(int index, Activity removedActivity, Activity addedActivity, object owner, ActivityCollectionChangeAction action) { this.index = index; if (removedActivity != null) { this.removedItems = new List (); ((List )this.removedItems).Add(removedActivity); } if (addedActivity != null) { this.addedItems = new List (); ((List )this.addedItems).Add(addedActivity); } this.action = action; this.owner = owner; } public IList RemovedItems { get { return (this.removedItems != null) ? new List (this.removedItems).AsReadOnly() : new List ().AsReadOnly(); } } public IList AddedItems { get { return (this.addedItems != null) ? new List (this.addedItems).AsReadOnly() : new List ().AsReadOnly(); } } public object Owner { get { return this.owner; } } public int Index { get { return this.index; } } public ActivityCollectionChangeAction Action { get { return this.action; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
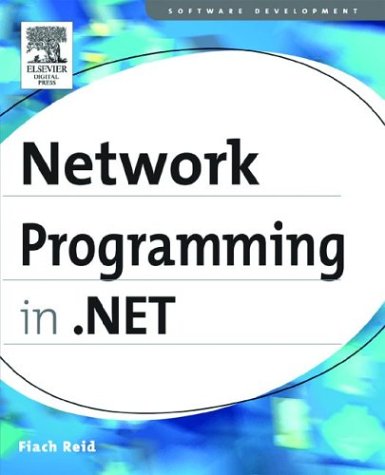
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Simplifier.cs
- HiddenField.cs
- AssemblyName.cs
- ConversionContext.cs
- DataFormats.cs
- WindowsUpDown.cs
- XmlSchemaSimpleContent.cs
- SqlCacheDependencyDatabase.cs
- CommonDialog.cs
- ApplicationServicesHostFactory.cs
- ManagementEventArgs.cs
- ByteKeyFrameCollection.cs
- DbProviderFactoriesConfigurationHandler.cs
- ComponentFactoryHelpers.cs
- InputMethodStateChangeEventArgs.cs
- MasterPageParser.cs
- CalendarDesigner.cs
- XmlSortKeyAccumulator.cs
- TypeDescriptionProviderAttribute.cs
- BatchWriter.cs
- Binding.cs
- _DomainName.cs
- ProfileService.cs
- SystemIcmpV6Statistics.cs
- SecurityContextSecurityTokenParameters.cs
- EmptyEnumerator.cs
- ProcessProtocolHandler.cs
- MatrixTransform.cs
- DataGridViewColumn.cs
- ComponentConverter.cs
- LinkClickEvent.cs
- PersistenceTask.cs
- Effect.cs
- ItemCollection.cs
- ListItemConverter.cs
- ProfileGroupSettings.cs
- WindowsGraphics2.cs
- EncodingInfo.cs
- TagElement.cs
- XamlRtfConverter.cs
- StatusBarPanel.cs
- FtpCachePolicyElement.cs
- SqlBuffer.cs
- PersonalizationAdministration.cs
- ContainerFilterService.cs
- Knowncolors.cs
- HashRepartitionStream.cs
- ObjectDataSourceView.cs
- DefaultEventAttribute.cs
- DbProviderFactoriesConfigurationHandler.cs
- SignedInfo.cs
- EntryPointNotFoundException.cs
- XmlCDATASection.cs
- SweepDirectionValidation.cs
- UnsafeNativeMethodsMilCoreApi.cs
- SafeNativeMethods.cs
- SignedPkcs7.cs
- DataBoundControlAdapter.cs
- ToolStripStatusLabel.cs
- CodeIndexerExpression.cs
- ListDictionaryInternal.cs
- SplineKeyFrames.cs
- X509CertificateCollection.cs
- DeflateStream.cs
- configsystem.cs
- ItemContainerGenerator.cs
- HttpWriter.cs
- Graph.cs
- Pkcs7Signer.cs
- WorkflowInstanceSuspendedRecord.cs
- IUnknownConstantAttribute.cs
- MsmqIntegrationElement.cs
- XmlExtensionFunction.cs
- HtmlTitle.cs
- TransformPatternIdentifiers.cs
- Stack.cs
- CustomErrorsSectionWrapper.cs
- HtmlHistory.cs
- OleDbStruct.cs
- InputBuffer.cs
- DispatcherHooks.cs
- SoapObjectReader.cs
- SqlCommandSet.cs
- ThrowHelper.cs
- Accessible.cs
- UserControlCodeDomTreeGenerator.cs
- TimerEventSubscription.cs
- ResponseStream.cs
- CellCreator.cs
- _ListenerRequestStream.cs
- MenuItemStyleCollection.cs
- SetIterators.cs
- RuntimeIdentifierPropertyAttribute.cs
- XamlInt32CollectionSerializer.cs
- DispatcherHooks.cs
- InvalidAsynchronousStateException.cs
- parserscommon.cs
- DynamicMethod.cs
- SpoolingTask.cs
- DocumentsTrace.cs