Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / VisualBasicSettingsHandler.cs / 1305376 / VisualBasicSettingsHandler.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System.ComponentModel; using Microsoft.VisualBasic.Activities; using System.Activities.Debugger; using System.Activities.Presentation.Model; using System.Runtime; using System.Linq; //the class does several things: //1. make sure a special property "Imports" (implemented using VisualBasicSettings attached properties) is added to the root object before it's loaded into ModelTree //2. make sure the "root workflow" of the root object always have the same VisualBasicSettings static class VisualBasicSettingsHandler { static public void PreviewLoadRoot(object sender, WorkflowDesigner.PreviewLoadEventArgs args) { VisualBasicSettings settings = VisualBasic.GetSettings(args.Instance); if (settings == null) { settings = new VisualBasicSettings(); VisualBasic.SetSettings(args.Instance, settings); } IDebuggableWorkflowTree root = args.Instance as IDebuggableWorkflowTree; if (root != null) { Activity rootActivity = root.GetWorkflowRoot(); if (rootActivity != null) { VisualBasic.SetSettings(rootActivity, settings); } args.Context.Services.Subscribe(manager => manager.Root.PropertyChanged += new PropertyChangedEventHandler(OnRootPropertyChanged)); } TypeDescriptor.AddProvider(new RootModelTypeDescriptionProvider(args.Instance), args.Instance); } static void OnRootPropertyChanged(object sender, PropertyChangedEventArgs e) { ModelItem rootModel = sender as ModelItem; Fx.Assert(rootModel != null, "sender item could not be null"); ModelProperty changedProperty = rootModel.Properties[e.PropertyName]; if (changedProperty == null) { return; } object changedPropertyValue = changedProperty.ComputedValue; if (changedPropertyValue == null) { return; } IDebuggableWorkflowTree root = rootModel.GetCurrentValue() as IDebuggableWorkflowTree; Fx.Assert(root != null, "root must be a IDebuggableWorkflowTree"); if (root.GetWorkflowRoot() == changedPropertyValue) { VisualBasicSettings settings = VisualBasic.GetSettings(root); VisualBasic.SetSettings(changedPropertyValue, settings); } } } class RootModelTypeDescriptionProvider : TypeDescriptionProvider { public RootModelTypeDescriptionProvider(object instance) : base(TypeDescriptor.GetProvider(instance)) { } public override ICustomTypeDescriptor GetTypeDescriptor(Type objectType, object instance) { ICustomTypeDescriptor defaultDescriptor = base.GetTypeDescriptor(objectType, instance); return new RootModelTypeDescriptor(defaultDescriptor, instance); } } class RootModelTypeDescriptor : CustomTypeDescriptor { object root; NamespaceListPropertyDescriptor importDescriptor; public RootModelTypeDescriptor(ICustomTypeDescriptor parent, object root) : base(parent) { this.root = root; } PropertyDescriptor ImportDescriptor { get { if (this.importDescriptor == null) { this.importDescriptor = new NamespaceListPropertyDescriptor(this.root); } return this.importDescriptor; } } public override PropertyDescriptorCollection GetProperties() { return GetProperties(null); } public override PropertyDescriptorCollection GetProperties(Attribute[] attributes) { return new PropertyDescriptorCollection(base.GetProperties(attributes).Cast () .Union(new PropertyDescriptor[] { this.ImportDescriptor }).ToArray()); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
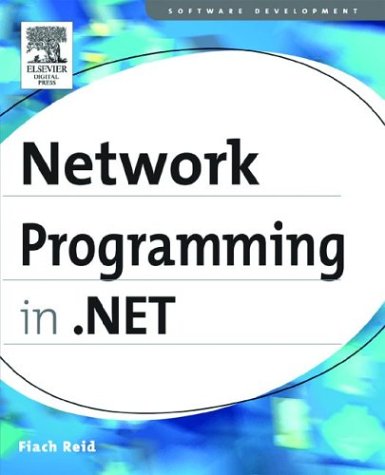
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthorizationSection.cs
- BoundField.cs
- ColumnResult.cs
- SqlDataSourceQueryEditor.cs
- ListViewSortEventArgs.cs
- SqlInternalConnectionSmi.cs
- sortedlist.cs
- Resources.Designer.cs
- EntityContainerEntitySet.cs
- VirtualPath.cs
- LineGeometry.cs
- TemplateXamlParser.cs
- MulticastNotSupportedException.cs
- TreeBuilderBamlTranslator.cs
- MemoryStream.cs
- XmlSchemaCollection.cs
- QilFunction.cs
- NumberFormatInfo.cs
- PeerCustomResolverBindingElement.cs
- PictureBoxDesigner.cs
- EmptyQuery.cs
- QilName.cs
- PackagePart.cs
- HttpCachePolicy.cs
- ManagedIStream.cs
- ManagedCodeMarkers.cs
- XmlElement.cs
- SettingsProperty.cs
- ConfigurationSection.cs
- Activity.cs
- RolePrincipal.cs
- XmlBinaryWriterSession.cs
- HeaderedContentControl.cs
- InstallerTypeAttribute.cs
- FormsIdentity.cs
- Convert.cs
- EventLogTraceListener.cs
- SatelliteContractVersionAttribute.cs
- Int32Animation.cs
- DataSourceHelper.cs
- FileUtil.cs
- ProgressPage.cs
- EdmTypeAttribute.cs
- MenuRenderer.cs
- WebReferencesBuildProvider.cs
- ZipIOLocalFileHeader.cs
- OperandQuery.cs
- StyleSelector.cs
- EntityDataSourceViewSchema.cs
- LinkLabel.cs
- XPathDocumentNavigator.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- TargetPerspective.cs
- MediaSystem.cs
- FormView.cs
- CreateInstanceBinder.cs
- ControlsConfig.cs
- UIntPtr.cs
- CryptoKeySecurity.cs
- ObjectStateFormatter.cs
- QilInvoke.cs
- AsyncPostBackErrorEventArgs.cs
- NumberFunctions.cs
- InvalidDataContractException.cs
- SplitterDesigner.cs
- ConfigurationLockCollection.cs
- NativeMethods.cs
- Int16Converter.cs
- PathSegmentCollection.cs
- ProxyWebPart.cs
- SafeHandle.cs
- ButtonBaseAutomationPeer.cs
- Update.cs
- CatalogPartChrome.cs
- HttpWebResponse.cs
- EncryptedXml.cs
- WebPartVerb.cs
- DataGridCheckBoxColumn.cs
- SamlConditions.cs
- ButtonChrome.cs
- ListViewItem.cs
- XmlArrayItemAttribute.cs
- DefaultAsyncDataDispatcher.cs
- ConfigurationValidatorBase.cs
- BrowsableAttribute.cs
- MobileControlBuilder.cs
- Stack.cs
- QilBinary.cs
- ToolboxItemFilterAttribute.cs
- SrgsRule.cs
- BuildProvidersCompiler.cs
- InputLanguageSource.cs
- MembershipValidatePasswordEventArgs.cs
- PrivilegedConfigurationManager.cs
- DependencyPropertyKind.cs
- HMACMD5.cs
- EntityDesignerDataSourceView.cs
- SafeWaitHandle.cs
- MatcherBuilder.cs
- SelectionHighlightInfo.cs