Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / DataGridCheckBoxColumn.cs / 1305600 / DataGridCheckBoxColumn.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Input; namespace System.Windows.Controls { ////// A column that displays a check box. /// public class DataGridCheckBoxColumn : DataGridBoundColumn { static DataGridCheckBoxColumn() { ElementStyleProperty.OverrideMetadata(typeof(DataGridCheckBoxColumn), new FrameworkPropertyMetadata(DefaultElementStyle)); EditingElementStyleProperty.OverrideMetadata(typeof(DataGridCheckBoxColumn), new FrameworkPropertyMetadata(DefaultEditingElementStyle)); } #region Styles ////// The default value of the ElementStyle property. /// This value can be used as the BasedOn for new styles. /// public static Style DefaultElementStyle { get { if (_defaultElementStyle == null) { Style style = new Style(typeof(CheckBox)); // When not in edit mode, the end-user should not be able to toggle the state style.Setters.Add(new Setter(UIElement.IsHitTestVisibleProperty, false)); style.Setters.Add(new Setter(UIElement.FocusableProperty, false)); style.Setters.Add(new Setter(CheckBox.HorizontalAlignmentProperty, HorizontalAlignment.Center)); style.Setters.Add(new Setter(CheckBox.VerticalAlignmentProperty, VerticalAlignment.Top)); style.Seal(); _defaultElementStyle = style; } return _defaultElementStyle; } } ////// The default value of the EditingElementStyle property. /// This value can be used as the BasedOn for new styles. /// public static Style DefaultEditingElementStyle { get { if (_defaultEditingElementStyle == null) { Style style = new Style(typeof(CheckBox)); style.Setters.Add(new Setter(CheckBox.HorizontalAlignmentProperty, HorizontalAlignment.Center)); style.Setters.Add(new Setter(CheckBox.VerticalAlignmentProperty, VerticalAlignment.Top)); style.Seal(); _defaultEditingElementStyle = style; } return _defaultEditingElementStyle; } } #endregion #region Element Generation ////// Creates the visual tree for boolean based cells. /// protected override FrameworkElement GenerateElement(DataGridCell cell, object dataItem) { return GenerateCheckBox(/* isEditing = */ false, cell); } ////// Creates the visual tree for boolean based cells. /// protected override FrameworkElement GenerateEditingElement(DataGridCell cell, object dataItem) { return GenerateCheckBox(/* isEditing = */ true, cell); } private CheckBox GenerateCheckBox(bool isEditing, DataGridCell cell) { CheckBox checkBox = (cell != null) ? (cell.Content as CheckBox) : null; if (checkBox == null) { checkBox = new CheckBox(); } checkBox.IsThreeState = IsThreeState; ApplyStyle(isEditing, /* defaultToElementStyle = */ true, checkBox); ApplyBinding(checkBox, CheckBox.IsCheckedProperty); return checkBox; } protected internal override void RefreshCellContent(FrameworkElement element, string propertyName) { DataGridCell cell = element as DataGridCell; if (cell != null && string.Compare(propertyName, "IsThreeState", StringComparison.Ordinal) == 0) { var checkBox = cell.Content as CheckBox; if (checkBox != null) { checkBox.IsThreeState = IsThreeState; } } else { base.RefreshCellContent(element, propertyName); } } #endregion #region Editing ////// The DependencyProperty for the IsThreeState property. /// Flags: None /// Default Value: false /// public static readonly DependencyProperty IsThreeStateProperty = CheckBox.IsThreeStateProperty.AddOwner( typeof(DataGridCheckBoxColumn), new FrameworkPropertyMetadata(false, DataGridColumn.NotifyPropertyChangeForRefreshContent)); ////// The IsThreeState property determines whether the control supports two or three states. /// IsChecked property can be set to null as a third state when IsThreeState is true /// public bool IsThreeState { get { return (bool)GetValue(IsThreeStateProperty); } set { SetValue(IsThreeStateProperty, value); } } ////// Called when a cell has just switched to edit mode. /// /// A reference to element returned by GenerateEditingElement. /// The event args of the input event that caused the cell to go into edit mode. May be null. ///The unedited value of the cell. protected override object PrepareCellForEdit(FrameworkElement editingElement, RoutedEventArgs editingEventArgs) { CheckBox checkBox = editingElement as CheckBox; if (checkBox != null) { checkBox.Focus(); bool? uneditedValue = checkBox.IsChecked; // If a click or a space key invoked the begin edit, then do an immediate toggle if ((IsMouseLeftButtonDown(editingEventArgs) && IsMouseOver(checkBox, editingEventArgs)) || IsSpaceKeyDown(editingEventArgs)) { checkBox.IsChecked = (uneditedValue != true); } return uneditedValue; } return (bool?) false; } internal override void OnInput(InputEventArgs e) { // Space key down will begin edit mode if (IsSpaceKeyDown(e)) { BeginEdit(e); } } private static bool IsMouseLeftButtonDown(RoutedEventArgs e) { MouseButtonEventArgs mouseArgs = e as MouseButtonEventArgs; return (mouseArgs != null) && (mouseArgs.ChangedButton == MouseButton.Left) && (mouseArgs.ButtonState == MouseButtonState.Pressed); } private static bool IsMouseOver(CheckBox checkBox, RoutedEventArgs e) { // This element is new, so the IsMouseOver property will not have been updated // yet, but there is enough information to do a hit-test. return checkBox.InputHitTest(((MouseButtonEventArgs)e).GetPosition(checkBox)) != null; } private static bool IsSpaceKeyDown(RoutedEventArgs e) { KeyEventArgs keyArgs = e as KeyEventArgs; return (keyArgs != null) && ((keyArgs.KeyStates & KeyStates.Down) == KeyStates.Down) && (keyArgs.Key == Key.Space); } #endregion #region Data private static Style _defaultElementStyle; private static Style _defaultEditingElementStyle; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Input; namespace System.Windows.Controls { ////// A column that displays a check box. /// public class DataGridCheckBoxColumn : DataGridBoundColumn { static DataGridCheckBoxColumn() { ElementStyleProperty.OverrideMetadata(typeof(DataGridCheckBoxColumn), new FrameworkPropertyMetadata(DefaultElementStyle)); EditingElementStyleProperty.OverrideMetadata(typeof(DataGridCheckBoxColumn), new FrameworkPropertyMetadata(DefaultEditingElementStyle)); } #region Styles ////// The default value of the ElementStyle property. /// This value can be used as the BasedOn for new styles. /// public static Style DefaultElementStyle { get { if (_defaultElementStyle == null) { Style style = new Style(typeof(CheckBox)); // When not in edit mode, the end-user should not be able to toggle the state style.Setters.Add(new Setter(UIElement.IsHitTestVisibleProperty, false)); style.Setters.Add(new Setter(UIElement.FocusableProperty, false)); style.Setters.Add(new Setter(CheckBox.HorizontalAlignmentProperty, HorizontalAlignment.Center)); style.Setters.Add(new Setter(CheckBox.VerticalAlignmentProperty, VerticalAlignment.Top)); style.Seal(); _defaultElementStyle = style; } return _defaultElementStyle; } } ////// The default value of the EditingElementStyle property. /// This value can be used as the BasedOn for new styles. /// public static Style DefaultEditingElementStyle { get { if (_defaultEditingElementStyle == null) { Style style = new Style(typeof(CheckBox)); style.Setters.Add(new Setter(CheckBox.HorizontalAlignmentProperty, HorizontalAlignment.Center)); style.Setters.Add(new Setter(CheckBox.VerticalAlignmentProperty, VerticalAlignment.Top)); style.Seal(); _defaultEditingElementStyle = style; } return _defaultEditingElementStyle; } } #endregion #region Element Generation ////// Creates the visual tree for boolean based cells. /// protected override FrameworkElement GenerateElement(DataGridCell cell, object dataItem) { return GenerateCheckBox(/* isEditing = */ false, cell); } ////// Creates the visual tree for boolean based cells. /// protected override FrameworkElement GenerateEditingElement(DataGridCell cell, object dataItem) { return GenerateCheckBox(/* isEditing = */ true, cell); } private CheckBox GenerateCheckBox(bool isEditing, DataGridCell cell) { CheckBox checkBox = (cell != null) ? (cell.Content as CheckBox) : null; if (checkBox == null) { checkBox = new CheckBox(); } checkBox.IsThreeState = IsThreeState; ApplyStyle(isEditing, /* defaultToElementStyle = */ true, checkBox); ApplyBinding(checkBox, CheckBox.IsCheckedProperty); return checkBox; } protected internal override void RefreshCellContent(FrameworkElement element, string propertyName) { DataGridCell cell = element as DataGridCell; if (cell != null && string.Compare(propertyName, "IsThreeState", StringComparison.Ordinal) == 0) { var checkBox = cell.Content as CheckBox; if (checkBox != null) { checkBox.IsThreeState = IsThreeState; } } else { base.RefreshCellContent(element, propertyName); } } #endregion #region Editing ////// The DependencyProperty for the IsThreeState property. /// Flags: None /// Default Value: false /// public static readonly DependencyProperty IsThreeStateProperty = CheckBox.IsThreeStateProperty.AddOwner( typeof(DataGridCheckBoxColumn), new FrameworkPropertyMetadata(false, DataGridColumn.NotifyPropertyChangeForRefreshContent)); ////// The IsThreeState property determines whether the control supports two or three states. /// IsChecked property can be set to null as a third state when IsThreeState is true /// public bool IsThreeState { get { return (bool)GetValue(IsThreeStateProperty); } set { SetValue(IsThreeStateProperty, value); } } ////// Called when a cell has just switched to edit mode. /// /// A reference to element returned by GenerateEditingElement. /// The event args of the input event that caused the cell to go into edit mode. May be null. ///The unedited value of the cell. protected override object PrepareCellForEdit(FrameworkElement editingElement, RoutedEventArgs editingEventArgs) { CheckBox checkBox = editingElement as CheckBox; if (checkBox != null) { checkBox.Focus(); bool? uneditedValue = checkBox.IsChecked; // If a click or a space key invoked the begin edit, then do an immediate toggle if ((IsMouseLeftButtonDown(editingEventArgs) && IsMouseOver(checkBox, editingEventArgs)) || IsSpaceKeyDown(editingEventArgs)) { checkBox.IsChecked = (uneditedValue != true); } return uneditedValue; } return (bool?) false; } internal override void OnInput(InputEventArgs e) { // Space key down will begin edit mode if (IsSpaceKeyDown(e)) { BeginEdit(e); } } private static bool IsMouseLeftButtonDown(RoutedEventArgs e) { MouseButtonEventArgs mouseArgs = e as MouseButtonEventArgs; return (mouseArgs != null) && (mouseArgs.ChangedButton == MouseButton.Left) && (mouseArgs.ButtonState == MouseButtonState.Pressed); } private static bool IsMouseOver(CheckBox checkBox, RoutedEventArgs e) { // This element is new, so the IsMouseOver property will not have been updated // yet, but there is enough information to do a hit-test. return checkBox.InputHitTest(((MouseButtonEventArgs)e).GetPosition(checkBox)) != null; } private static bool IsSpaceKeyDown(RoutedEventArgs e) { KeyEventArgs keyArgs = e as KeyEventArgs; return (keyArgs != null) && ((keyArgs.KeyStates & KeyStates.Down) == KeyStates.Down) && (keyArgs.Key == Key.Space); } #endregion #region Data private static Style _defaultElementStyle; private static Style _defaultEditingElementStyle; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
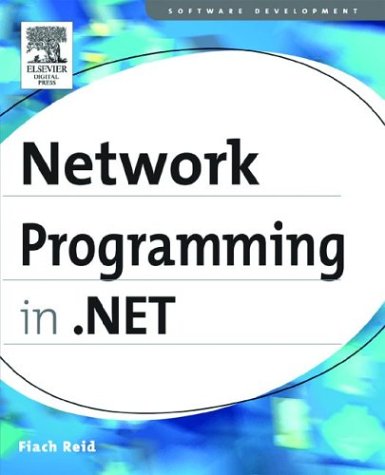
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ArcSegment.cs
- FileNotFoundException.cs
- TdsRecordBufferSetter.cs
- PageOutputQuality.cs
- HttpWriter.cs
- NumericUpDown.cs
- FixedSOMTableRow.cs
- TextWriterEngine.cs
- InheritanceAttribute.cs
- PrimarySelectionAdorner.cs
- DesignTimeType.cs
- Token.cs
- WindowProviderWrapper.cs
- StringArrayConverter.cs
- RequestCache.cs
- SupportingTokenListenerFactory.cs
- TransactionFlowOption.cs
- ElapsedEventArgs.cs
- pingexception.cs
- ISAPIRuntime.cs
- ReadOnlyDictionary.cs
- SmiMetaData.cs
- SingleAnimationUsingKeyFrames.cs
- UrlPath.cs
- MetadataPropertyvalue.cs
- IPipelineRuntime.cs
- DataGridCheckBoxColumn.cs
- SafeFileMappingHandle.cs
- SafeNativeMethods.cs
- XmlDataSourceView.cs
- ImageCodecInfo.cs
- TextRunCache.cs
- LogSwitch.cs
- DeclarativeCatalogPartDesigner.cs
- IsolatedStorageException.cs
- FieldNameLookup.cs
- OleDbConnectionPoolGroupProviderInfo.cs
- Win32Native.cs
- TemplateFactory.cs
- StylusCollection.cs
- StrongTypingException.cs
- TripleDESCryptoServiceProvider.cs
- Win32.cs
- CursorInteropHelper.cs
- ProfilePropertySettingsCollection.cs
- transactioncontext.cs
- TileModeValidation.cs
- PropertyConverter.cs
- ListMarkerLine.cs
- TextServicesContext.cs
- Stack.cs
- ForeignConstraint.cs
- WindowsFormsSynchronizationContext.cs
- ServerValidateEventArgs.cs
- SqlCommandBuilder.cs
- RectangleConverter.cs
- SectionRecord.cs
- ItemsControlAutomationPeer.cs
- ProcessProtocolHandler.cs
- ExpandCollapsePattern.cs
- EllipseGeometry.cs
- CollectionType.cs
- RoutedCommand.cs
- ConfigXmlSignificantWhitespace.cs
- ScalarType.cs
- UnSafeCharBuffer.cs
- JsonByteArrayDataContract.cs
- PointLight.cs
- DesignTimeValidationFeature.cs
- ProgressBarAutomationPeer.cs
- SafeBitVector32.cs
- AncillaryOps.cs
- WmlImageAdapter.cs
- PaintEvent.cs
- RepeaterItemEventArgs.cs
- Scheduling.cs
- VScrollBar.cs
- OrthographicCamera.cs
- DataStorage.cs
- cookiecontainer.cs
- ChangeBlockUndoRecord.cs
- AttachedPropertiesService.cs
- SchemaImporterExtensionElement.cs
- TrustManagerPromptUI.cs
- DataPagerFieldCollection.cs
- StreamHelper.cs
- ImportCatalogPart.cs
- PaginationProgressEventArgs.cs
- SubclassTypeValidatorAttribute.cs
- PenThreadWorker.cs
- ColorBlend.cs
- documentsequencetextpointer.cs
- SolidBrush.cs
- PenLineJoinValidation.cs
- HtmlFormParameterReader.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- _HelperAsyncResults.cs
- COAUTHINFO.cs
- StackSpiller.Bindings.cs
- SectionInput.cs