Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.ServiceModel.Web / System / ServiceModel / Dispatcher / WebErrorHandler.cs / 1407647 / WebErrorHandler.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- #pragma warning disable 1634, 1691 namespace System.ServiceModel.Dispatcher { using System; using System.Diagnostics; using System.Net; using System.Runtime; using System.Runtime.Serialization; using System.Runtime.Serialization.Json; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.ServiceModel.Syndication; using System.ServiceModel.Web; using System.Xml.Linq; using System.Xml.Serialization; using System.ServiceModel.Diagnostics; class WebErrorHandler : IErrorHandler { WebHttpBehavior webHttpBehavior; ContractDescription contractDescription; bool includeExceptionDetailInFaults; public WebErrorHandler(WebHttpBehavior webHttpBehavior, ContractDescription contractDescription, bool includeExceptionDetailInFaults) { this.webHttpBehavior = webHttpBehavior; this.contractDescription = contractDescription; this.includeExceptionDetailInFaults = includeExceptionDetailInFaults; } public bool HandleError(Exception error) { return false; } public void ProvideFault(Exception error, MessageVersion version, ref Message fault) { if (version != MessageVersion.None || error == null) { return; } // If the exception is not derived from FaultException and the fault message is already present // then only another error handler could have provided the fault so we should not replace it FaultException errorAsFaultException = error as FaultException; if (errorAsFaultException == null && fault != null) { return; } try { if (error is IWebFaultException) { IWebFaultException webFaultException = (IWebFaultException)error; WebOperationContext context = WebOperationContext.Current; context.OutgoingResponse.StatusCode = webFaultException.StatusCode; string operationName; if (OperationContext.Current.IncomingMessageProperties.TryGetValue(WebHttpDispatchOperationSelector.HttpOperationNamePropertyName, out operationName)) { OperationDescription description = this.contractDescription.Operations.Find(operationName); bool isXmlSerializerFaultFormat = WebHttpBehavior.IsXmlSerializerFaultFormat(description); if (isXmlSerializerFaultFormat && WebOperationContext.Current.OutgoingResponse.Format == WebMessageFormat.Json) { throw System.ServiceModel.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.GetString(SR2.JsonFormatRequiresDataContract, description.Name, description.DeclaringContract.Name, description.DeclaringContract.Namespace))); } WebMessageFormat? nullableFormat = !isXmlSerializerFaultFormat ? context.OutgoingResponse.Format : WebMessageFormat.Xml; WebMessageFormat format = nullableFormat.HasValue ? nullableFormat.Value : this.webHttpBehavior.GetResponseFormat(description); if (webFaultException.DetailObject != null) { switch (format) { case WebMessageFormat.Json: fault = context.CreateJsonResponse(webFaultException.DetailObject, new DataContractJsonSerializer(webFaultException.DetailType, webFaultException.KnownTypes)); break; case WebMessageFormat.Xml: if (isXmlSerializerFaultFormat) { fault = context.CreateXmlResponse(webFaultException.DetailObject, new XmlSerializer(webFaultException.DetailType, webFaultException.KnownTypes)); } else { fault = context.CreateXmlResponse(webFaultException.DetailObject, new DataContractSerializer(webFaultException.DetailType, webFaultException.KnownTypes)); } break; } } else { HttpResponseMessageProperty property; if (OperationContext.Current.OutgoingMessageProperties.TryGetValue (HttpResponseMessageProperty.Name, out property) && property != null) { property.SuppressEntityBody = true; } if (format == WebMessageFormat.Json) { fault.Properties.Add(WebBodyFormatMessageProperty.Name, WebBodyFormatMessageProperty.JsonProperty); } } } else { throw System.ServiceModel.DiagnosticUtility.ExceptionUtility.ThrowHelperError(new InvalidOperationException(SR2.OperationNameNotFound)); } } else { fault = CreateHtmlResponse(error); } } catch (Exception ex) { if (Fx.IsFatal(ex)) { throw; } if (System.ServiceModel.DiagnosticUtility.ShouldTraceWarning) { System.ServiceModel.DiagnosticUtility.ExceptionUtility.TraceHandledException(new InvalidOperationException(SR2.GetString(SR2.HelpPageFailedToCreateErrorMessage)), TraceEventType.Warning); } WebOperationContext.Current.OutgoingResponse.StatusCode = HttpStatusCode.BadRequest; fault = CreateHtmlResponse(ex); } } Message CreateHtmlResponse(Exception error) { // Note: WebOperationContext may not be present in case of an invalid HTTP request Uri helpUri = null; if (WebOperationContext.Current != null) { helpUri = this.webHttpBehavior.HelpUri != null ? UriTemplate.RewriteUri(this.webHttpBehavior.HelpUri, WebOperationContext.Current.IncomingRequest.Headers[HttpRequestHeader.Host]) : null; } StreamBodyWriter bodyWriter; if (this.includeExceptionDetailInFaults) { bodyWriter = StreamBodyWriter.CreateStreamBodyWriter(s => HelpHtmlBuilder.CreateServerErrorPage(helpUri, error).Save(s, SaveOptions.OmitDuplicateNamespaces)); } else { bodyWriter = StreamBodyWriter.CreateStreamBodyWriter(s => HelpHtmlBuilder.CreateServerErrorPage(helpUri, null).Save(s, SaveOptions.OmitDuplicateNamespaces)); } Message response = new HttpStreamMessage(bodyWriter); response.Properties.Add(WebBodyFormatMessageProperty.Name, WebBodyFormatMessageProperty.RawProperty); HttpResponseMessageProperty responseProperty = GetResponseProperty(WebOperationContext.Current, response); if (!responseProperty.HasStatusCodeBeenSet) { responseProperty.StatusCode = HttpStatusCode.BadRequest; } responseProperty.Headers[HttpResponseHeader.ContentType] = Atom10Constants.HtmlMediaType; return response; } static HttpResponseMessageProperty GetResponseProperty(WebOperationContext currentContext, Message response) { HttpResponseMessageProperty responseProperty; if (currentContext != null) { responseProperty = currentContext.OutgoingResponse.MessageProperty; } else { responseProperty = new HttpResponseMessageProperty(); response.Properties.Add(HttpResponseMessageProperty.Name, responseProperty); } return responseProperty; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
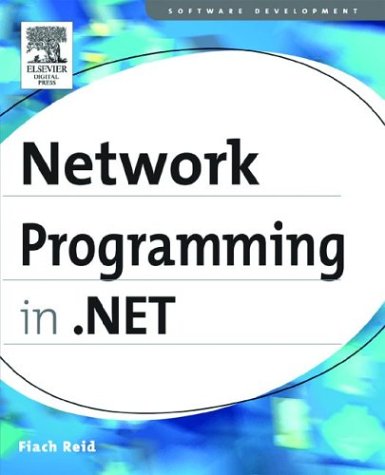
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Screen.cs
- ExpressionWriter.cs
- DataGridViewButtonColumn.cs
- Style.cs
- WebPartEventArgs.cs
- DrawingAttributesDefaultValueFactory.cs
- ModelItemKeyValuePair.cs
- ExtenderProviderService.cs
- NetworkInformationPermission.cs
- UnionExpr.cs
- RelationshipWrapper.cs
- TextElementAutomationPeer.cs
- MembershipUser.cs
- ContextMenuStrip.cs
- UpdateManifestForBrowserApplication.cs
- VirtualDirectoryMappingCollection.cs
- BufferModesCollection.cs
- DataGridViewRowsRemovedEventArgs.cs
- SafeArrayRankMismatchException.cs
- EncryptedKey.cs
- CustomLineCap.cs
- ResourcePermissionBaseEntry.cs
- BuildProviderCollection.cs
- SynchronizationHandlesCodeDomSerializer.cs
- StdValidatorsAndConverters.cs
- ToolStripContainerActionList.cs
- RangeBaseAutomationPeer.cs
- MenuItemBinding.cs
- Thickness.cs
- HelpEvent.cs
- RemotingHelper.cs
- XamlReader.cs
- UIPropertyMetadata.cs
- XPathPatternBuilder.cs
- FileDialog_Vista.cs
- DataGridViewComboBoxEditingControl.cs
- ComponentChangedEvent.cs
- CatalogPart.cs
- AttachmentService.cs
- RestHandler.cs
- JsonEnumDataContract.cs
- PanelStyle.cs
- WebPartCatalogAddVerb.cs
- MailDefinitionBodyFileNameEditor.cs
- SystemInfo.cs
- NamespaceImport.cs
- TraceXPathNavigator.cs
- AutomationIdentifier.cs
- compensatingcollection.cs
- Signature.cs
- EmptyElement.cs
- DependencyPropertyHelper.cs
- SecurityTokenAuthenticator.cs
- GetPageCompletedEventArgs.cs
- BuildProvidersCompiler.cs
- VectorCollectionConverter.cs
- ModelVisual3D.cs
- XmlNamespaceMapping.cs
- IndicCharClassifier.cs
- TimeSpanConverter.cs
- UrlParameterWriter.cs
- MarginsConverter.cs
- ListMarkerLine.cs
- ToolStripDropDownDesigner.cs
- RayHitTestParameters.cs
- OleDbInfoMessageEvent.cs
- DomNameTable.cs
- SourceFileInfo.cs
- PersonalizationDictionary.cs
- RequestNavigateEventArgs.cs
- ClipboardData.cs
- SHA1CryptoServiceProvider.cs
- DataGridTableCollection.cs
- Viewport3DAutomationPeer.cs
- PkcsUtils.cs
- VisualProxy.cs
- StackSpiller.Temps.cs
- SectionXmlInfo.cs
- MdImport.cs
- StrokeFIndices.cs
- BamlBinaryReader.cs
- DocumentSchemaValidator.cs
- HtmlInputButton.cs
- CommentAction.cs
- PerformanceCounterPermissionEntry.cs
- FormsAuthenticationUserCollection.cs
- ConfigXmlText.cs
- HttpsChannelListener.cs
- WebPartConnectionsDisconnectVerb.cs
- ComponentResourceManager.cs
- XmlCollation.cs
- DbDataRecord.cs
- DataServiceException.cs
- ChangesetResponse.cs
- ServiceModelPerformanceCounters.cs
- Cloud.cs
- ValueType.cs
- DataAdapter.cs
- SharedStatics.cs
- ConfigurationManagerInternal.cs