Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / MS / Internal / Shaping / IndicCharClassifier.cs / 2 / IndicCharClassifier.cs
//---------------------------------------------------------------------- // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2003 // // File: IndicCharClassifier.cs // // Contents: Implementation of Indic shaping engine and its factory // // Created: 03-04-2007 [....] ([....]) // //----------------------------------------------------------------------- // #define VALIDATE_CLUSTER_PARAMETERS using System; using System.Security; using System.Security.Permissions; using System.Diagnostics; using System.Collections; using System.Globalization; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; using MS.Internal.FontCache; using MS.Internal.FontFace; using MS.Internal.PresentationCore; namespace MS.Internal.Shaping { internal enum IndicScriptIndices : byte { Devanagari, Bengali, Gurmukhi, Gujarati, Oriya, Tamil, Telugu, Kannada, Malayalam }; ////// IndicCharClassifier - /// internal class IndicCharClassifier : ShaperCharacterClassifier { internal const byte CharsPerIndicScript = 0x80; internal const ushort RaOffset = 0x30; internal const ushort HalantOffset = 0x4d; private IndicScriptIndices _scriptIx; private DependentCharacterList[] _scriptMatraInvalidVowels; private DependentCharacterList[] _compositeChars; private byte[] _charFormsInfo; bool _isFormsDiscoveryDone = false; public IndicCharClassifier( ScriptTags scriptTag, GlyphTypeface fontFace ) : base(scriptTag, fontFace) { _scriptIx = GetScriptIx(scriptTag); _unknownClass = (byte)IndicCharClass.Reserved; _spaceClass = (byte)IndicCharClass.Neutral; _zwControlClass = (byte)IndicCharClass.Neutral; _zwjClass = (byte)IndicCharClass.ZWJ; _zwnjClass = (byte)IndicCharClass.ZWNJ; _shyClass = (byte)IndicCharClass.Reserved; _firstChar = '\u0900'; // this should be set to the script's first Unicode char _lastChar = '\u0d7f'; // this should be set to the script's last Unicode char _xorRange = 0x0080; // this range is used in GetCharClass _xorMask = (ushort) ((ushort)_firstChar + ((ushort)_scriptIx << 7)); // this mask is used in GetCharClass (scriptIx * 0x80) _charClassTable = _indicCharClasses; _compositeChars = GetCompositeCharactersList(); _scriptMatraInvalidVowels = GetInvalidVowelsList(); _charFormsInfo = null; } //////IndicCharClassifier.ToShapeInfo - returns CharShapeInfo for the Unicode character /// public override CharShapeInfo ToShapeInfo (char unicodeChar ) { CharShapeInfo charShape = base.ToShapeInfo(unicodeChar); // if the character is returned as "unknown", check for vedic characters if (charShape == (CharShapeInfo.IsStartOfCluster | (CharShapeInfo)_unknownClass)) { if (((ushort)unicodeChar >= 0x0951 && (ushort)unicodeChar <= 0x0965) && ((ushort)unicodeChar <= 0x00955 || (ushort)unicodeChar >= 0x00964)) { // all Indic text can include Devanagari Anudatta or Udatta... charShape = (CharShapeInfo) _charClassTable[(int)unicodeChar - _firstChar]; } } return charShape; } ////// componentRepoInfo - returns the repositioning class info. /// public IndicRepositioningClass ToRepositioningClass ( char unicodeChar ) { CharShapeInfo charShape = ToShapeInfo(unicodeChar); Debug.Assert((charShape & (CharShapeInfo) IndicCharClass.IncludesPositioningInfo) != 0,"\"Includes Positioning Info\" flag not set."); charShape = (CharShapeInfo)((ushort)charShape >> IndicShape.RepositioningInfoShift); return (IndicRepositioningClass)charShape; } //////IndicCharClassifier.ToFormInfo - returns IndicFormFlags for the Unicode character /// public IndicFormFlags ToFormInfo (char unicodeChar ) { IndicFormFlags charFormInfo = 0; if (((ushort)unicodeChar ^ _xorMask) < _xorRange) { if (_charFormsInfo == null) { charFormInfo = (IndicFormFlags)_indicCharFormsInfo[unicodeChar - _firstChar]; } else { charFormInfo = (IndicFormFlags)_charFormsInfo[unicodeChar - FirstCharacterInScriptRange]; } } return charFormInfo; } ////// ToDecompositionList - returns the decomposition character sequence for the given character /// (if one exists) /// public char[] ToDecompositionList ( char unicodeChar ) { return GetDependentCharacterList(unicodeChar, _compositeChars); } ////// GetInvalidVowelList - returns the decomposition character sequence for the given character /// (if one exists) /// public char[] GetMatraInvalidVowelList ( char unicodeChar ) { return GetDependentCharacterList(unicodeChar, _scriptMatraInvalidVowels); } public IndicRepositioningClass HalantRepositioningClass { get { return ToRepositioningClass( (char)(_xorMask + HalantOffset) ); } } public IndicScriptIndices ScriptIx { get { return _scriptIx; } } public char FirstCharacterInScriptRange { get { return (char)_xorMask; } } public char[][] TamilAkhands { get { return _tamilAkhands; } } public char HalantCharacter { get { return (char)(_xorMask + HalantOffset); } } ////// Critical - calls critical code /// [SecurityCritical] public bool GetFontCharacterFormsInfo (IndicFontClient fontClient) { if (!_isFormsDiscoveryDone) { byte[] fontCharacterForms = new byte[IndicCharClassifier.CharsPerIndicScript]; for (int i = 0; i < IndicCharClassifier.CharsPerIndicScript; ++i) { fontCharacterForms[i] = (byte)ToFormInfo((char)(_xorMask + i)); } // see if font has anything to add. (If so, keep the new info) if (fontClient.GetCharacterFormsInfo(fontCharacterForms)) { _charFormsInfo = fontCharacterForms; } _isFormsDiscoveryDone = true; } return true; } private IndicScriptIndices GetScriptIx (ScriptTags scriptTag) { switch (scriptTag) { case ScriptTags.Devanagari: return IndicScriptIndices.Devanagari; case ScriptTags.Bengali: return IndicScriptIndices.Bengali; case ScriptTags.Gurmukhi: return IndicScriptIndices.Gurmukhi; case ScriptTags.Gujarati: return IndicScriptIndices.Gujarati; case ScriptTags.Oriya: return IndicScriptIndices.Oriya; case ScriptTags.Tamil: return IndicScriptIndices.Tamil; case ScriptTags.Telugu: return IndicScriptIndices.Telugu; case ScriptTags.Kannada: return IndicScriptIndices.Kannada; case ScriptTags.Malayalam: return IndicScriptIndices.Malayalam; case ScriptTags.Devanagari_v2: return IndicScriptIndices.Devanagari; case ScriptTags.Bengali_v2: return IndicScriptIndices.Bengali; case ScriptTags.Gurmukhi_v2: return IndicScriptIndices.Gurmukhi; case ScriptTags.Gujarati_v2: return IndicScriptIndices.Gujarati; case ScriptTags.Oriya_v2: return IndicScriptIndices.Oriya; case ScriptTags.Tamil_v2: return IndicScriptIndices.Tamil; case ScriptTags.Telugu_v2: return IndicScriptIndices.Telugu; case ScriptTags.Kannada_v2: return IndicScriptIndices.Kannada; case ScriptTags.Malayalam_v2: return IndicScriptIndices.Malayalam; default: throw new NotSupportedException(); } } private DependentCharacterList[] GetCompositeCharactersList () { switch (_scriptIx) { case IndicScriptIndices.Devanagari: return null; case IndicScriptIndices.Bengali: return _bengaliSplitMatras; case IndicScriptIndices.Gurmukhi: return null; case IndicScriptIndices.Gujarati: return null; case IndicScriptIndices.Oriya: return _oriyaSplitMatras; case IndicScriptIndices.Tamil: return _tamilSplitMatras; case IndicScriptIndices.Telugu: return _teluguSplitMatras; case IndicScriptIndices.Kannada: return _kannadaSplitMatras; case IndicScriptIndices.Malayalam: return _malayalamSplitMatras; default: throw new NotSupportedException(); } } private DependentCharacterList[] GetInvalidVowelsList () { switch (_scriptIx) { case IndicScriptIndices.Devanagari: return _hindiMatraInvalidVowels; case IndicScriptIndices.Bengali: return _bengaliMatraInvalidVowels; case IndicScriptIndices.Gurmukhi: return _gurmukhiMatraInvalidVowels; case IndicScriptIndices.Gujarati: return _gujaratiMatraInvalidVowels; case IndicScriptIndices.Oriya: return _oriyaMatraInvalidVowels; case IndicScriptIndices.Tamil: return null; case IndicScriptIndices.Telugu: return _teluguMatraInvalidVowels; case IndicScriptIndices.Kannada: return _kannadaMatraInvalidVowels; case IndicScriptIndices.Malayalam: return _malayalamMatraInvalidVowels; default: throw new NotSupportedException(); } } // Bengali Script split matras private static readonly DependentCharacterList[] _bengaliSplitMatras = { new DependentCharacterList( '\u09cb', new char[] {'\u09c7','\u09be'} ), new DependentCharacterList( '\u09cc', new char[] {'\u09c7','\u09d7'} ) }; // Bengali invalid vowel matras private static readonly DependentCharacterList[] _bengaliMatraInvalidVowels = { new DependentCharacterList( '\u09be', new char[] {'\u0985'} ), new DependentCharacterList( '\u09c3', new char[] {'\u098b'} ), new DependentCharacterList( '\u09e2', new char[] {'\u098c'} ), }; // Gujarati invalid vowel matras private static readonly DependentCharacterList[] _gujaratiMatraInvalidVowels = { new DependentCharacterList( '\u0abe', new char[] {'\u0a85'} ), new DependentCharacterList( '\u0ac5', new char[] {'\u0a85','\u0a86'} ), new DependentCharacterList( '\u0ac7', new char[] {'\u0a85','\u0a86'} ), new DependentCharacterList( '\u0ac8', new char[] {'\u0a85','\u0a86'} ), new DependentCharacterList( '\u0ac9', new char[] {'\u0a85'} ), new DependentCharacterList( '\u0acb', new char[] {'\u0a85'} ), new DependentCharacterList( '\u0acc', new char[] {'\u0a85'} ), }; // Gurmukhi invalid vowel matras private static readonly DependentCharacterList[] _gurmukhiMatraInvalidVowels = { new DependentCharacterList( '\u0a3e', new char[] {'\u0a05'} ), new DependentCharacterList( '\u0a3f', new char[] {'\u0a72'} ), new DependentCharacterList( '\u0a40', new char[] {'\u0a72'} ), new DependentCharacterList( '\u0a41', new char[] {'\u0a73'} ), new DependentCharacterList( '\u0a42', new char[] {'\u0a73'} ), new DependentCharacterList( '\u0a47', new char[] {'\u0a72'} ), new DependentCharacterList( '\u0a48', new char[] {'\u0a05'} ), new DependentCharacterList( '\u0a4c', new char[] {'\u0a05'} ) }; // Hindi (Devanagari) invalid vowel matras private static readonly DependentCharacterList[] _hindiMatraInvalidVowels = { new DependentCharacterList( '\u093e', new char[] {'\u0905'} ), new DependentCharacterList( '\u0945', new char[] {'\u0906','\u090f'} ), new DependentCharacterList( '\u0946', new char[] {'\u0905','\u0906','\u090f'} ), new DependentCharacterList( '\u0947', new char[] {'\u0906','\u090f'} ), new DependentCharacterList( '\u0948', new char[] {'\u0906'} ), new DependentCharacterList( '\u0949', new char[] {'\u0905'} ), new DependentCharacterList( '\u094a', new char[] {'\u0905'} ), new DependentCharacterList( '\u094b', new char[] {'\u0905'} ), new DependentCharacterList( '\u094c', new char[] {'\u0905'} ) }; // Kannada Script split matras private static readonly DependentCharacterList[] _kannadaSplitMatras = { new DependentCharacterList('\u0cc0',new char[] {'\u0cbf','\u0cd5'}), new DependentCharacterList('\u0cc7',new char[] {'\u0cc6','\u0cd5'}), new DependentCharacterList('\u0cc8',new char[] {'\u0cc6','\u0cd6'}), new DependentCharacterList('\u0cca',new char[] {'\u0cc6','\u0cc2'}), new DependentCharacterList('\u0ccb',new char[] {'\u0cc6','\u0cc2','\u0cd5'}), }; // Kannada invalid vowel matras private static readonly DependentCharacterList[] _kannadaMatraInvalidVowels = { new DependentCharacterList( '\u0ccc', new char[] {'\u0c92'} ) }; // Malayalam Script split matras private static readonly DependentCharacterList[] _malayalamSplitMatras = { new DependentCharacterList('\u0d4a', new char[] {'\u0d46','\u0d3e'}), new DependentCharacterList('\u0d4b', new char[] {'\u0d47','\u0d3e'}), new DependentCharacterList('\u0d4c', new char[] {'\u0d46','\u0d57'}), }; // Malayalam invalid vowel matras private static readonly DependentCharacterList[] _malayalamMatraInvalidVowels = { new DependentCharacterList( '\u0d3e', new char[] {'\u0d12'} ), new DependentCharacterList( '\u0d46', new char[] {'\u0d0e'} ), new DependentCharacterList( '\u0d57', new char[] {'\u0d07','\u0d09','\u0d12'} ), }; // Oriya Script split matras private static readonly DependentCharacterList[] _oriyaSplitMatras = { new DependentCharacterList('\u0b48', new char[] {'\u0b47','\u0b56'}), new DependentCharacterList('\u0b4b', new char[] {'\u0b47','\u0b3e'}), new DependentCharacterList('\u0b4c', new char[] {'\u0b47','\u0b57'}), }; // Oriya invalid vowel matras private static readonly DependentCharacterList[] _oriyaMatraInvalidVowels = { new DependentCharacterList( '\u0b3e', new char[] {'\u0b05'} ) }; private static readonly DependentCharacterList[] _tamilSplitMatras = { // Tamil Script split matras new DependentCharacterList('\u0b94', new char[] {'\u0b92','\u0bd7'}), new DependentCharacterList('\u0bca', new char[] {'\u0bc6','\u0bbe'}), new DependentCharacterList('\u0bcb', new char[] {'\u0bc7','\u0bbe'}), new DependentCharacterList('\u0bcc', new char[] {'\u0bc6','\u0bd7'}), }; private static readonly char[][] _tamilAkhands = { // Tamil Script akhands new char[] {'\u0b95','\u0bcd','\u0bb7'}, new char[] {'\u0bb6','\u0bcd','\u0bb0','\u0bc0'}, new char[] {'\u0bb8','\u0bcd','\u0bb0','\u0bc0'}, }; // Telugu Script split matras private static readonly DependentCharacterList[] _teluguSplitMatras = { new DependentCharacterList('\u0c48', new char[] {'\u0c46','\u0c56'}), }; // Telugu invalid vowel matras private static readonly DependentCharacterList[] _teluguMatraInvalidVowels = { new DependentCharacterList( '\u0c3e', new char[] {'\u0c0b','\u0c0c'} ), new DependentCharacterList( '\u0c4c', new char[] {'\u0c12'} ), new DependentCharacterList( '\u0c55', new char[] {'\u0c12'} ) }; #region Classification Tables private const byte RepositioningClassShift = (byte)IndicRepositioningClass.RepositioningClassShift; private const byte UndefinedChar = (byte)IndicCharClass.Reserved; private const byte Neutral = (byte)IndicCharClass.Neutral; private const byte Consonant = (byte)IndicCharClass.Consonant; private const byte NuktaConsonant = (byte)IndicCharClass.NuktaConsonant; private const byte Ra = (byte)(IndicCharClass.Ra | IndicCharClass.IncludesPositioningInfo); private const byte Vowel = (byte)IndicCharClass.Vowel; private const byte Nukta = (byte)IndicCharClass.Nukta; private const byte Halant = (byte)(IndicCharClass.Halant | IndicCharClass.IncludesPositioningInfo); private const byte Vedic = (byte)(IndicCharClass.Vedic | IndicCharClass.IncludesPositioningInfo); private const byte VowelSign = (byte)(IndicCharClass.VowelSign | IndicCharClass.IncludesPositioningInfo); private const byte Matra = (byte)(IndicCharClass.Matra | IndicCharClass.IncludesPositioningInfo); private const byte SplitMatra = (byte)IndicCharClass.Matra; private const byte RepositionAtStart = ((byte)IndicRepositioningClass.AtStart << RepositioningClassShift); private const byte RepositionBeforeMain = ((byte)IndicRepositioningClass.BeforeMain << RepositioningClassShift); private const byte RepositionAfterMain = ((byte)IndicRepositioningClass.AfterMain << RepositioningClassShift); private const byte RepositionBeforeSub = ((byte)IndicRepositioningClass.BeforeSub << RepositioningClassShift); private const byte RepositionAfterSub = ((byte)IndicRepositioningClass.AfterSub << RepositioningClassShift); private const byte RepositionBeforePost = ((byte)IndicRepositioningClass.BeforePost << RepositioningClassShift); private const byte RepositionAfterPost = ((byte)IndicRepositioningClass.AfterPost << RepositioningClassShift); private const byte RepositionBeforeEnd = ((byte)IndicRepositioningClass.BeforeEnd << RepositioningClassShift); private static readonly byte[] _indicCharClasses = { // All the Devanagari Unicode chars (U+900 - U+97F) classified //090 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, //091 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, Vowel, Vowel, Vowel, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //092 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, NuktaConsonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //093 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionBeforePost, NuktaConsonant, Consonant, Consonant, NuktaConsonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, Nukta, Neutral, Matra|RepositionAfterSub, Matra|RepositionAtStart, //094 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Halant|RepositionAfterSub, UndefinedChar, UndefinedChar, //095 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Neutral, Vedic|RepositionBeforeEnd, Vedic|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, UndefinedChar, UndefinedChar, UndefinedChar, NuktaConsonant, NuktaConsonant, NuktaConsonant, NuktaConsonant, NuktaConsonant, NuktaConsonant, NuktaConsonant, NuktaConsonant, //096 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //097 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Neutral, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Consonant, Consonant, Neutral, Consonant, Consonant, // All the Bengali Unicode chars (U+980 - U+9FF) classified //098 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, VowelSign|RepositionAfterPost, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, UndefinedChar, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, UndefinedChar, UndefinedChar, Vowel, //099 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, UndefinedChar, UndefinedChar, Vowel, Vowel, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //09A 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //09B 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionAfterSub, UndefinedChar, Consonant, UndefinedChar, UndefinedChar, UndefinedChar, Consonant, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, Nukta, Neutral, Matra|RepositionAfterPost, Matra|RepositionAtStart, //09C 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Matra|RepositionAfterPost, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, UndefinedChar, Matra|RepositionAtStart, Matra|RepositionAtStart, UndefinedChar, UndefinedChar, SplitMatra, SplitMatra, Halant|RepositionAfterPost, Consonant, UndefinedChar, //09D 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAfterPost, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, NuktaConsonant, NuktaConsonant, UndefinedChar, NuktaConsonant, //09E 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, UndefinedChar, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //09F 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionAfterSub, Consonant, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, // All the Gurmukhi Unicode chars (U+a00 - U+a7F) classified //0A0 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, VowelSign|RepositionAfterPost, VowelSign|RepositionAfterPost, VowelSign|RepositionBeforeEnd, UndefinedChar, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Vowel, //0A1 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, UndefinedChar, UndefinedChar, Vowel, Vowel, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0A2 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0A3 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionBeforePost, UndefinedChar, Consonant, NuktaConsonant, UndefinedChar, Consonant, NuktaConsonant, UndefinedChar, Consonant, Consonant, UndefinedChar, UndefinedChar, Nukta, UndefinedChar, Matra|RepositionAfterPost, Matra|RepositionAtStart, //0A4 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Matra|RepositionAfterPost, Matra|RepositionAfterPost, Matra|RepositionAfterPost, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAfterPost, Matra|RepositionAfterPost, UndefinedChar, UndefinedChar, Matra|RepositionAfterPost, Matra|RepositionAfterPost, Halant|RepositionAfterSub, UndefinedChar, UndefinedChar, //0A5 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, NuktaConsonant, NuktaConsonant, NuktaConsonant, Consonant, UndefinedChar, NuktaConsonant, UndefinedChar, //0A6 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //0A7 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F VowelSign|RepositionAfterPost, VowelSign|RepositionAfterPost, Vowel, Vowel, Neutral, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, // All the Gujarati Unicode chars (U+a80 - U+afF) classified //0A8 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, UndefinedChar, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, UndefinedChar, Vowel, //0A9 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, UndefinedChar, Vowel, Vowel, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0AA 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0AB 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionBeforePost, UndefinedChar, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, Nukta, Neutral, Matra|RepositionAfterSub, Matra|RepositionAtStart, //0AC 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Halant|RepositionAfterSub, UndefinedChar, UndefinedChar, //0AD 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Neutral, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, //0AE 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, UndefinedChar, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //0AF 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, Neutral, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, // All the Oriya Unicode chars (U+B00 - U+B7F) classified //0B0 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, VowelSign|RepositionBeforeSub, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, UndefinedChar, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, UndefinedChar, UndefinedChar, Vowel, //0B1 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, UndefinedChar, UndefinedChar, Vowel, Vowel, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0B2 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0B3 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionAfterMain, UndefinedChar, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, Nukta, Neutral, Matra|RepositionAfterPost, Matra|RepositionAfterMain, //0B4 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Matra|RepositionAfterPost, Matra|RepositionAfterSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAtStart, SplitMatra, UndefinedChar, UndefinedChar, SplitMatra, SplitMatra, Halant|RepositionAfterPost, UndefinedChar, UndefinedChar, //0B5 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAfterMain, Matra|RepositionAfterPost, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, NuktaConsonant, NuktaConsonant, UndefinedChar, Consonant, //0B6 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //0B7 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Neutral, Consonant, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, // All the Tamil Unicode chars (U+B80 - U+BFF) classified //0B8 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, VowelSign|RepositionBeforeEnd, Neutral, //Vowel, UndefinedChar, Vowel, Consonant, Vowel, Vowel, Vowel, Vowel, UndefinedChar, UndefinedChar, UndefinedChar, Vowel, Vowel, //0B9 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, UndefinedChar, Vowel, Vowel, Vowel, Consonant, UndefinedChar, UndefinedChar, UndefinedChar, Consonant, Consonant, UndefinedChar, Consonant, UndefinedChar, Consonant, Consonant, //0BA 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, Consonant, Consonant, UndefinedChar, UndefinedChar, UndefinedChar, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, UndefinedChar, Consonant, Consonant, //0BB 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionAfterPost, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAfterPost, Matra|RepositionAfterPost, //0BC 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Matra|RepositionAfterSub, Matra|RepositionAfterPost, Matra|RepositionAfterPost, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionBeforeMain, Matra|RepositionBeforeMain, Matra|RepositionBeforeMain, UndefinedChar, SplitMatra, SplitMatra, SplitMatra, Halant|RepositionAfterPost, UndefinedChar, UndefinedChar, //0BD 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAfterPost, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, //0BE 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //0BF 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, // All the Telugu Unicode chars (U+c00 - U+c7F) classified //0C0 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, UndefinedChar, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, UndefinedChar, Vowel, Vowel, //0C1 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, UndefinedChar, Vowel, Vowel, Vowel, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0C2 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0C3 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionAfterPost, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, //0C4 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, SplitMatra, UndefinedChar, Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, Halant|RepositionAfterPost, UndefinedChar, UndefinedChar, //0C5 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, //0C6 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //0C7 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, // All the Kannada Unicode chars (U+C80 - U+CFF) classified //0C8 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, UndefinedChar, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, UndefinedChar, Vowel, Vowel, //0C9 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, UndefinedChar, Vowel, Vowel, Vowel, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0CA 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0CB 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionAfterPost, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, Nukta, Neutral, Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, //0CC 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F SplitMatra, Matra|RepositionBeforeSub, Matra|RepositionBeforeSub, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, Matra|RepositionBeforeSub, SplitMatra, SplitMatra, UndefinedChar, SplitMatra, SplitMatra, Matra|RepositionBeforeSub, Halant|RepositionBeforeSub, UndefinedChar, UndefinedChar, //0CD 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAfterSub, Matra|RepositionAfterSub, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Consonant, UndefinedChar, //0CE 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //0CF 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, // All the Malayalam Unicode chars (U+d00 - U+d7F) classified //0D0 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, VowelSign|RepositionBeforeEnd, VowelSign|RepositionBeforeEnd, UndefinedChar, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, Vowel, UndefinedChar, Vowel, Vowel, //0D1 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, UndefinedChar, Vowel, Vowel, Vowel, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0D2 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, //0D3 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Ra|RepositionAfterMain, Ra|RepositionAfterMain, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, Consonant, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAfterPost, Matra|RepositionAfterPost, //0D4 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Matra|RepositionAfterPost, Matra|RepositionAfterPost, Matra|RepositionAfterPost, Matra|RepositionAfterPost, UndefinedChar, UndefinedChar, Matra|RepositionAtStart, Matra|RepositionAtStart, Matra|RepositionAtStart, UndefinedChar, SplitMatra, SplitMatra, SplitMatra, Halant|RepositionAfterPost, UndefinedChar, UndefinedChar, //0D5 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Matra|RepositionAfterPost, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, //0D6 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Vowel, Vowel, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, Neutral, //0D7 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, UndefinedChar, }; #endregion // end of Classification Tables #region Character Form Tables private const byte PreBaseComponent = (byte)IndicFormFlags.PreBaseMatra; private const byte AboveBaseComponent = (byte)IndicFormFlags.AboveBaseMatra; private const byte BelowBaseComponent = (byte)IndicFormFlags.BelowBaseMatra; private const byte PostBaseComponent = (byte)IndicFormFlags.PostBaseMatra; private const byte PreBaseMatra = (byte)IndicFormFlags.PreBaseMatra; private const byte AboveBaseMatra = (byte)IndicFormFlags.AboveBaseMatra; private const byte BelowBaseMatra = (byte)IndicFormFlags.BelowBaseMatra; private const byte PostBaseMatra = (byte)IndicFormFlags.PostBaseMatra; private const byte Reph = (byte)IndicFormFlags.RephForm; private const byte Vattu = (byte)IndicFormFlags.VattuForm; private const byte InitialChar = (byte)IndicFormFlags.InitForm; private const byte PostBaseReph = (byte)IndicFormFlags.PostbaseReph; private const byte DravidianBase = (byte)IndicFormFlags.DravidianBase; private const byte SubForm = (byte)IndicFormFlags.SubForm; private const byte PostForm = (byte)IndicFormFlags.PostForm; private static readonly byte[] _indicCharFormsInfo = { // All the Devanagari Unicode chars (U+900 - U+97F) forms info //090 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //091 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //092 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //093 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Reph | Vattu, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, PreBaseMatra, //094 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F PostBaseMatra, BelowBaseMatra, BelowBaseMatra, BelowBaseMatra, BelowBaseMatra, AboveBaseMatra, AboveBaseMatra, AboveBaseMatra, AboveBaseMatra, PostBaseMatra, PostBaseMatra, PostBaseMatra, PostBaseMatra, 0, 0, 0, //095 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //096 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, BelowBaseMatra, BelowBaseMatra, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //097 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // All the Bengali Unicode chars (U+980 - U+9FF) consonant/matra forms (the consonant // forms will be updated from the font) //098 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //099 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //09A 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, SubForm, 0, 0, PostForm, //09B 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F InitialChar | Reph | SubForm, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, PreBaseMatra, //09C 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F PostBaseMatra, BelowBaseMatra, BelowBaseMatra, BelowBaseMatra, BelowBaseMatra, 0, 0, PreBaseMatra|InitialChar, PreBaseMatra|InitialChar, 0, 0, InitialChar|PreBaseComponent|PostBaseComponent, InitialChar|PreBaseComponent|PostBaseComponent, 0, 0, 0, //09D 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, 0, 0, 0, 0, 0, 0, 0, 0, //09E 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, BelowBaseMatra, BelowBaseMatra, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //09F 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Reph | SubForm, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // All the Gurmukhi Unicode chars (U+a00 - U+a7F) classified //0A0 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0A1 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0A2 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, PostForm, //0A3 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F SubForm, 0, 0, 0, 0, SubForm, 0, 0, 0, SubForm, 0, 0, 0, 0, PostBaseMatra, PreBaseMatra, //0A4 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F PostBaseMatra, BelowBaseMatra, BelowBaseMatra, 0, 0, 0, 0, AboveBaseMatra, AboveBaseMatra, 0, 0, AboveBaseMatra, AboveBaseMatra, 0, 0, 0, //0A5 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0A6 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0A7 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // All the Gujarati Unicode chars (U+a80 - U+afF) classified //0A8 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0A9 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0AA 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0AB 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Reph | Vattu, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, PreBaseMatra, //0AC 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F PostBaseMatra, BelowBaseMatra, BelowBaseMatra, BelowBaseMatra, BelowBaseMatra, AboveBaseMatra, 0, AboveBaseMatra, AboveBaseMatra, PostBaseMatra, 0, PostBaseMatra, PostBaseMatra, 0, 0, 0, //0AD 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0AE 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, BelowBaseMatra, BelowBaseMatra, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0AF 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // All the Oriya Unicode chars (U+B00 - U+B7F) classified //0B0 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0B1 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0B2 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, SubForm, 0, 0, 0, SubForm, 0, 0, 0, SubForm, SubForm, SubForm, PostForm, //0B3 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Reph | SubForm, 0, SubForm, SubForm, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, AboveBaseMatra, //0B4 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F PostBaseMatra, BelowBaseMatra, BelowBaseMatra, BelowBaseMatra, 0, 0, 0, PreBaseMatra, PreBaseComponent|AboveBaseComponent, 0, 0, PreBaseComponent|PostBaseComponent, PreBaseComponent|AboveBaseComponent|PostBaseComponent, 0, 0, 0, //0B5 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, AboveBaseMatra, PostBaseMatra|AboveBaseComponent, 0, 0, 0, 0, 0, 0, 0, PostForm, //0B6 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0B7 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // All the Tamil Unicode chars (U+B80 - U+BFF) classified //0B8 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0B9 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0BA 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0BB 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, PostBaseMatra, //0BC 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F AboveBaseMatra, BelowBaseMatra, BelowBaseMatra, 0, 0, 0, PreBaseMatra, PreBaseMatra, PreBaseMatra, 0, PreBaseComponent|PostBaseComponent, PreBaseComponent|PostBaseComponent, PreBaseComponent|PostBaseComponent, 0, 0, 0, //0BD 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, 0, 0, 0, 0, 0, 0, 0, 0, //0BE 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0BF 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // All the Telugu Unicode chars (U+c00 - U+c7F) classified //0C0 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0C1 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, //0C2 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, 0, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, //0C3 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F SubForm, SubForm, SubForm, SubForm, 0, SubForm, SubForm, SubForm, SubForm, SubForm, 0, 0, 0, 0, AboveBaseMatra, AboveBaseMatra, //0C4 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F AboveBaseMatra, PostBaseMatra, PostBaseMatra, PostBaseMatra , PostBaseMatra, 0, AboveBaseMatra, AboveBaseMatra, AboveBaseComponent|BelowBaseComponent, 0, AboveBaseMatra, AboveBaseMatra, AboveBaseMatra, 0, 0, 0, //0C5 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, AboveBaseMatra, BelowBaseMatra, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0C6 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0C7 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // All the Kannada Unicode chars (U+C80 - U+CFF) classified //0C8 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0C9 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, //0CA 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, 0, SubForm, SubForm, SubForm, SubForm, SubForm, SubForm, //0CB 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F Reph | SubForm, SubForm, SubForm, SubForm, 0, SubForm, SubForm, SubForm, SubForm, SubForm, 0, 0, 0, 0, PostBaseMatra, AboveBaseMatra, //0CC 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F AboveBaseComponent|PostBaseComponent, PostBaseMatra, PostBaseMatra, PostBaseMatra, PostBaseMatra, 0, AboveBaseMatra, AboveBaseComponent|PostBaseComponent, AboveBaseComponent|PostBaseComponent, 0, AboveBaseComponent|PostBaseComponent, AboveBaseComponent|PostBaseComponent, AboveBaseMatra, 0, 0, 0, //0CD 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, PostBaseMatra, PostBaseMatra, 0, 0, 0, 0, 0, 0, 0, SubForm, 0, //0CE 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0CF 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, // All the Malayalam Unicode chars (U+d00 - U+d7F) classified //0D0 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0D1 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0D2 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, PostForm, //0D3 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F PostForm|PostBaseReph, DravidianBase, SubForm, DravidianBase, DravidianBase, PostForm, 0, 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, PostBaseMatra, //0D4 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F PostBaseMatra, PostBaseMatra, PostBaseMatra, PostBaseMatra, 0, 0, PreBaseMatra, PreBaseMatra, PreBaseMatra, 0, PreBaseComponent|PostBaseComponent, PreBaseComponent|PostBaseComponent, PreBaseComponent|PostBaseComponent, 0, 0, 0, //0D5 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, PostBaseMatra, 0, 0, 0, 0, 0, 0, 0, 0, //0D6 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, //0D7 0, 4, 8, C 1, 5, 9, D 2, 6, A, E 3, 7, B, F 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, }; #endregion // Character Form Tables } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
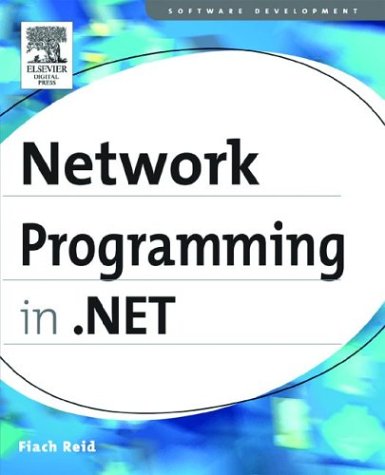
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlLanguageConverter.cs
- EncodingTable.cs
- ButtonFlatAdapter.cs
- PasswordPropertyTextAttribute.cs
- securitycriticaldata.cs
- PagePropertiesChangingEventArgs.cs
- uribuilder.cs
- WebPartDisplayModeEventArgs.cs
- PerfCounters.cs
- ConditionBrowserDialog.cs
- WindowsFormsHostAutomationPeer.cs
- QilReference.cs
- DBConcurrencyException.cs
- Semaphore.cs
- CharacterMetrics.cs
- HtmlMeta.cs
- DataKeyCollection.cs
- CompositeDataBoundControl.cs
- SqlIdentifier.cs
- QueryOutputWriter.cs
- DeferredRunTextReference.cs
- ProcessModule.cs
- ControlValuePropertyAttribute.cs
- PolicyLevel.cs
- AdRotatorDesigner.cs
- SqlDesignerDataSourceView.cs
- WizardSideBarListControlItemEventArgs.cs
- Soap11ServerProtocol.cs
- WebPartUtil.cs
- PartitionResolver.cs
- InputReport.cs
- LockRecoveryTask.cs
- BamlRecordWriter.cs
- SchemaNamespaceManager.cs
- TitleStyle.cs
- XPathNodeHelper.cs
- QueryableDataSourceEditData.cs
- NetCodeGroup.cs
- StaticFileHandler.cs
- XhtmlBasicCommandAdapter.cs
- CompensationExtension.cs
- CompositeScriptReference.cs
- ScrollContentPresenter.cs
- FormViewCommandEventArgs.cs
- ControlCollection.cs
- ObjectReaderCompiler.cs
- BrowserCapabilitiesFactoryBase.cs
- RouteItem.cs
- Process.cs
- ModulesEntry.cs
- CustomGrammar.cs
- TextFormatterImp.cs
- SqlGenericUtil.cs
- WindowAutomationPeer.cs
- DefaultEvaluationContext.cs
- ContextMarshalException.cs
- ColorPalette.cs
- QilSortKey.cs
- StagingAreaInputItem.cs
- SourceFileInfo.cs
- BuildProvider.cs
- ListViewItem.cs
- SingleObjectCollection.cs
- SocketElement.cs
- ScriptComponentDescriptor.cs
- SqlNodeAnnotation.cs
- Slider.cs
- EDesignUtil.cs
- WindowInteropHelper.cs
- ActivityTypeDesigner.xaml.cs
- TypeHelpers.cs
- ArraySubsetEnumerator.cs
- NetNamedPipeSecurityElement.cs
- TransformerConfigurationWizardBase.cs
- DrawListViewColumnHeaderEventArgs.cs
- MethodMessage.cs
- WebPartEventArgs.cs
- MultiPageTextView.cs
- StylusOverProperty.cs
- FontDriver.cs
- EventsTab.cs
- DataGridViewColumnStateChangedEventArgs.cs
- SmiEventSink_Default.cs
- MsmqIntegrationReceiveParameters.cs
- TokenBasedSetEnumerator.cs
- Clause.cs
- ListViewHitTestInfo.cs
- PolicyUtility.cs
- Monitor.cs
- UTF7Encoding.cs
- DataBinder.cs
- SystemUnicastIPAddressInformation.cs
- _NegoState.cs
- ToolBarOverflowPanel.cs
- DiagnosticTrace.cs
- AesManaged.cs
- RoleServiceManager.cs
- SqlInternalConnection.cs
- SuppressMessageAttribute.cs
- InvokeBase.cs