Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / Serialization / System / Runtime / Serialization / DataContractSerializer.cs / 1305376 / DataContractSerializer.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Runtime.Serialization { using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.IO; using System.Reflection; using System.Text; using System.Xml; using System.Xml.Serialization; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Runtime.CompilerServices; using DataContractDictionary = System.Collections.Generic.Dictionary; public sealed class DataContractSerializer : XmlObjectSerializer { Type rootType; DataContract rootContract; // post-surrogate bool needsContractNsAtRoot; XmlDictionaryString rootName; XmlDictionaryString rootNamespace; int maxItemsInObjectGraph; bool ignoreExtensionDataObject; bool preserveObjectReferences; IDataContractSurrogate dataContractSurrogate; ReadOnlyCollection knownTypeCollection; internal IList knownTypeList; internal DataContractDictionary knownDataContracts; DataContractResolver dataContractResolver; public DataContractSerializer(Type type) : this(type, null) { } public DataContractSerializer(Type type, IEnumerable knownTypes) : this(type, knownTypes, int.MaxValue, false, false, null) { } public DataContractSerializer(Type type, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { Initialize(type, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate); } public DataContractSerializer(Type type, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate, DataContractResolver dataContractResolver) : this (type, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate) { this.dataContractResolver = dataContractResolver; } public DataContractSerializer(Type type, string rootName, string rootNamespace) : this(type, rootName, rootNamespace, null) { } public DataContractSerializer(Type type, string rootName, string rootNamespace, IEnumerable knownTypes) : this(type, rootName, rootNamespace, knownTypes, int.MaxValue, false, false, null) { } public DataContractSerializer(Type type, string rootName, string rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { XmlDictionary dictionary = new XmlDictionary(2); Initialize(type, dictionary.Add(rootName), dictionary.Add(DataContract.GetNamespace(rootNamespace)), knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate); } public DataContractSerializer(Type type, string rootName, string rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate, DataContractResolver dataContractResolver) : this(type, rootName, rootNamespace, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate) { this.dataContractResolver = dataContractResolver; } public DataContractSerializer(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace) : this(type, rootName, rootNamespace, null) { } public DataContractSerializer(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace, IEnumerable knownTypes) : this(type, rootName, rootNamespace, knownTypes, int.MaxValue, false, false, null) { } public DataContractSerializer(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { Initialize(type, rootName, rootNamespace, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate); } public DataContractSerializer(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate, DataContractResolver dataContractResolver) : this(type, rootName, rootNamespace, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate) { this.dataContractResolver = dataContractResolver; } void Initialize(Type type, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { CheckNull(type, "type"); this.rootType = type; if (knownTypes != null) { this.knownTypeList = new List (); foreach (Type knownType in knownTypes) { this.knownTypeList.Add(knownType); } } if (maxItemsInObjectGraph < 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("maxItemsInObjectGraph", SR.GetString(SR.ValueMustBeNonNegative))); this.maxItemsInObjectGraph = maxItemsInObjectGraph; this.ignoreExtensionDataObject = ignoreExtensionDataObject; this.preserveObjectReferences = preserveObjectReferences; this.dataContractSurrogate = dataContractSurrogate; } void Initialize(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { Initialize(type, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate); // validate root name and namespace are both non-null this.rootName = rootName; this.rootNamespace = rootNamespace; } public ReadOnlyCollection KnownTypes { get { if (knownTypeCollection == null) { if (knownTypeList != null) { knownTypeCollection = new ReadOnlyCollection (knownTypeList); } else { knownTypeCollection = new ReadOnlyCollection (Globals.EmptyTypeArray); } } return knownTypeCollection; } } internal override DataContractDictionary KnownDataContracts { get { if (this.knownDataContracts == null && this.knownTypeList != null) { // This assignment may be performed concurrently and thus is a race condition. // It's safe, however, because at worse a new (and identical) dictionary of // data contracts will be created and re-assigned to this field. Introduction // of a lock here could lead to deadlocks. this.knownDataContracts = XmlObjectSerializerContext.GetDataContractsForKnownTypes(this.knownTypeList); } return this.knownDataContracts; } } public int MaxItemsInObjectGraph { get { return maxItemsInObjectGraph; } } public IDataContractSurrogate DataContractSurrogate { get { return dataContractSurrogate; } } public bool PreserveObjectReferences { get { return preserveObjectReferences; } } public bool IgnoreExtensionDataObject { get { return ignoreExtensionDataObject; } } public DataContractResolver DataContractResolver { get { return dataContractResolver; } } DataContract RootContract { get { if (rootContract == null) { rootContract = DataContract.GetDataContract(((dataContractSurrogate == null) ? rootType : GetSurrogatedType(dataContractSurrogate, rootType))); needsContractNsAtRoot = CheckIfNeedsContractNsAtRoot(rootName, rootNamespace, rootContract); } return rootContract; } } internal override void InternalWriteObject(XmlWriterDelegator writer, object graph) { InternalWriteObject(writer, graph, null); } internal override void InternalWriteObject(XmlWriterDelegator writer, object graph, DataContractResolver dataContractResolver) { InternalWriteStartObject(writer, graph); InternalWriteObjectContent(writer, graph, dataContractResolver); InternalWriteEndObject(writer); } public override void WriteObject(XmlWriter writer, object graph) { WriteObjectHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteStartObject(XmlWriter writer, object graph) { WriteStartObjectHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteObjectContent(XmlWriter writer, object graph) { WriteObjectContentHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteEndObject(XmlWriter writer) { WriteEndObjectHandleExceptions(new XmlWriterDelegator(writer)); } public override void WriteStartObject(XmlDictionaryWriter writer, object graph) { WriteStartObjectHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteObjectContent(XmlDictionaryWriter writer, object graph) { WriteObjectContentHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteEndObject(XmlDictionaryWriter writer) { WriteEndObjectHandleExceptions(new XmlWriterDelegator(writer)); } public void WriteObject(XmlDictionaryWriter writer, object graph, DataContractResolver dataContractResolver) { WriteObjectHandleExceptions(new XmlWriterDelegator(writer), graph, dataContractResolver); } public override object ReadObject(XmlReader reader) { return ReadObjectHandleExceptions(new XmlReaderDelegator(reader), true /*verifyObjectName*/); } public override object ReadObject(XmlReader reader, bool verifyObjectName) { return ReadObjectHandleExceptions(new XmlReaderDelegator(reader), verifyObjectName); } public override bool IsStartObject(XmlReader reader) { return IsStartObjectHandleExceptions(new XmlReaderDelegator(reader)); } public override object ReadObject(XmlDictionaryReader reader, bool verifyObjectName) { return ReadObjectHandleExceptions(new XmlReaderDelegator(reader), verifyObjectName); } public override bool IsStartObject(XmlDictionaryReader reader) { return IsStartObjectHandleExceptions(new XmlReaderDelegator(reader)); } public object ReadObject(XmlDictionaryReader reader, bool verifyObjectName, DataContractResolver dataContractResolver) { return ReadObjectHandleExceptions(new XmlReaderDelegator(reader), verifyObjectName, dataContractResolver); } internal override void InternalWriteStartObject(XmlWriterDelegator writer, object graph) { WriteRootElement(writer, RootContract, rootName, rootNamespace, needsContractNsAtRoot); } internal override void InternalWriteObjectContent(XmlWriterDelegator writer, object graph) { InternalWriteObjectContent(writer, graph, null); } internal void InternalWriteObjectContent(XmlWriterDelegator writer, object graph, DataContractResolver dataContractResolver) { if (MaxItemsInObjectGraph == 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.ExceededMaxItemsQuota, MaxItemsInObjectGraph))); DataContract contract = RootContract; Type declaredType = contract.UnderlyingType; Type graphType = (graph == null) ? declaredType : graph.GetType(); if (dataContractSurrogate != null) graph = SurrogateToDataContractType(dataContractSurrogate, graph, declaredType, ref graphType); if (dataContractResolver == null) dataContractResolver = this.DataContractResolver; if (graph == null) { if (IsRootXmlAny(rootName, contract)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.IsAnyCannotBeNull, declaredType))); WriteNull(writer); } else { if (declaredType == graphType) { if (contract.CanContainReferences) { XmlObjectSerializerWriteContext context = XmlObjectSerializerWriteContext.CreateContext(this, contract, dataContractResolver); context.HandleGraphAtTopLevel(writer, graph, contract); context.SerializeWithoutXsiType(contract, writer, graph, declaredType.TypeHandle); } else { contract.WriteXmlValue(writer, graph, null); } } else { XmlObjectSerializerWriteContext context = null; if (IsRootXmlAny(rootName, contract)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.IsAnyCannotBeSerializedAsDerivedType, graphType, contract.UnderlyingType))); contract = GetDataContract(contract, declaredType, graphType); context = XmlObjectSerializerWriteContext.CreateContext(this, RootContract, dataContractResolver); if (contract.CanContainReferences) { context.HandleGraphAtTopLevel(writer, graph, contract); } context.OnHandleIsReference(writer, contract, graph); context.SerializeWithXsiTypeAtTopLevel(contract, writer, graph, declaredType.TypeHandle, graphType); } } } internal static DataContract GetDataContract(DataContract declaredTypeContract, Type declaredType, Type objectType) { if (declaredType.IsInterface && CollectionDataContract.IsCollectionInterface(declaredType)) { return declaredTypeContract; } else if (declaredType.IsArray)//Array covariance is not supported in XSD { return declaredTypeContract; } else { return DataContract.GetDataContract(objectType.TypeHandle, objectType, SerializationMode.SharedContract); } } internal override void InternalWriteEndObject(XmlWriterDelegator writer) { if (!IsRootXmlAny(rootName, RootContract)) { writer.WriteEndElement(); } } internal override object InternalReadObject(XmlReaderDelegator xmlReader, bool verifyObjectName) { return InternalReadObject(xmlReader, verifyObjectName, null); } internal override object InternalReadObject(XmlReaderDelegator xmlReader, bool verifyObjectName, DataContractResolver dataContractResolver) { if (MaxItemsInObjectGraph == 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.ExceededMaxItemsQuota, MaxItemsInObjectGraph))); if (dataContractResolver == null) dataContractResolver = this.DataContractResolver; if (verifyObjectName) { if (!InternalIsStartObject(xmlReader)) { XmlDictionaryString expectedName; XmlDictionaryString expectedNs; if (rootName == null) { expectedName = RootContract.TopLevelElementName; expectedNs = RootContract.TopLevelElementNamespace; } else { expectedName = rootName; expectedNs = rootNamespace; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationExceptionWithReaderDetails(SR.GetString(SR.ExpectingElement, expectedNs, expectedName), xmlReader)); } } else if (!IsStartElement(xmlReader)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationExceptionWithReaderDetails(SR.GetString(SR.ExpectingElementAtDeserialize, XmlNodeType.Element), xmlReader)); } DataContract contract = RootContract; if (contract.IsPrimitive && object.ReferenceEquals(contract.UnderlyingType, rootType) /*handle Nullable differently*/) { return contract.ReadXmlValue(xmlReader, null); } if (IsRootXmlAny(rootName, contract)) { return XmlObjectSerializerReadContext.ReadRootIXmlSerializable(xmlReader, contract as XmlDataContract, false /*isMemberType*/); } XmlObjectSerializerReadContext context = XmlObjectSerializerReadContext.CreateContext(this, contract, dataContractResolver); return context.InternalDeserialize(xmlReader, rootType, contract, null, null); } internal override bool InternalIsStartObject(XmlReaderDelegator reader) { return IsRootElement(reader, RootContract, rootName, rootNamespace); } internal override Type GetSerializeType(object graph) { return (graph == null) ? rootType : graph.GetType(); } internal override Type GetDeserializeType() { return rootType; } internal static object SurrogateToDataContractType(IDataContractSurrogate dataContractSurrogate, object oldObj, Type surrogatedDeclaredType, ref Type objType) { object obj = DataContractSurrogateCaller.GetObjectToSerialize(dataContractSurrogate, oldObj, objType, surrogatedDeclaredType); if (obj != oldObj) { if (obj == null) objType = Globals.TypeOfObject; else objType = obj.GetType(); } return obj; } internal static Type GetSurrogatedType(IDataContractSurrogate dataContractSurrogate, Type type) { return DataContractSurrogateCaller.GetDataContractType(dataContractSurrogate, DataContract.UnwrapNullableType(type)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.Runtime.Serialization { using System; using System.Collections; using System.Diagnostics; using System.Globalization; using System.IO; using System.Reflection; using System.Text; using System.Xml; using System.Xml.Serialization; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Runtime.CompilerServices; using DataContractDictionary = System.Collections.Generic.Dictionary ; public sealed class DataContractSerializer : XmlObjectSerializer { Type rootType; DataContract rootContract; // post-surrogate bool needsContractNsAtRoot; XmlDictionaryString rootName; XmlDictionaryString rootNamespace; int maxItemsInObjectGraph; bool ignoreExtensionDataObject; bool preserveObjectReferences; IDataContractSurrogate dataContractSurrogate; ReadOnlyCollection knownTypeCollection; internal IList knownTypeList; internal DataContractDictionary knownDataContracts; DataContractResolver dataContractResolver; public DataContractSerializer(Type type) : this(type, null) { } public DataContractSerializer(Type type, IEnumerable knownTypes) : this(type, knownTypes, int.MaxValue, false, false, null) { } public DataContractSerializer(Type type, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { Initialize(type, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate); } public DataContractSerializer(Type type, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate, DataContractResolver dataContractResolver) : this (type, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate) { this.dataContractResolver = dataContractResolver; } public DataContractSerializer(Type type, string rootName, string rootNamespace) : this(type, rootName, rootNamespace, null) { } public DataContractSerializer(Type type, string rootName, string rootNamespace, IEnumerable knownTypes) : this(type, rootName, rootNamespace, knownTypes, int.MaxValue, false, false, null) { } public DataContractSerializer(Type type, string rootName, string rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { XmlDictionary dictionary = new XmlDictionary(2); Initialize(type, dictionary.Add(rootName), dictionary.Add(DataContract.GetNamespace(rootNamespace)), knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate); } public DataContractSerializer(Type type, string rootName, string rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate, DataContractResolver dataContractResolver) : this(type, rootName, rootNamespace, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate) { this.dataContractResolver = dataContractResolver; } public DataContractSerializer(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace) : this(type, rootName, rootNamespace, null) { } public DataContractSerializer(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace, IEnumerable knownTypes) : this(type, rootName, rootNamespace, knownTypes, int.MaxValue, false, false, null) { } public DataContractSerializer(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { Initialize(type, rootName, rootNamespace, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate); } public DataContractSerializer(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate, DataContractResolver dataContractResolver) : this(type, rootName, rootNamespace, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate) { this.dataContractResolver = dataContractResolver; } void Initialize(Type type, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { CheckNull(type, "type"); this.rootType = type; if (knownTypes != null) { this.knownTypeList = new List (); foreach (Type knownType in knownTypes) { this.knownTypeList.Add(knownType); } } if (maxItemsInObjectGraph < 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("maxItemsInObjectGraph", SR.GetString(SR.ValueMustBeNonNegative))); this.maxItemsInObjectGraph = maxItemsInObjectGraph; this.ignoreExtensionDataObject = ignoreExtensionDataObject; this.preserveObjectReferences = preserveObjectReferences; this.dataContractSurrogate = dataContractSurrogate; } void Initialize(Type type, XmlDictionaryString rootName, XmlDictionaryString rootNamespace, IEnumerable knownTypes, int maxItemsInObjectGraph, bool ignoreExtensionDataObject, bool preserveObjectReferences, IDataContractSurrogate dataContractSurrogate) { Initialize(type, knownTypes, maxItemsInObjectGraph, ignoreExtensionDataObject, preserveObjectReferences, dataContractSurrogate); // validate root name and namespace are both non-null this.rootName = rootName; this.rootNamespace = rootNamespace; } public ReadOnlyCollection KnownTypes { get { if (knownTypeCollection == null) { if (knownTypeList != null) { knownTypeCollection = new ReadOnlyCollection (knownTypeList); } else { knownTypeCollection = new ReadOnlyCollection (Globals.EmptyTypeArray); } } return knownTypeCollection; } } internal override DataContractDictionary KnownDataContracts { get { if (this.knownDataContracts == null && this.knownTypeList != null) { // This assignment may be performed concurrently and thus is a race condition. // It's safe, however, because at worse a new (and identical) dictionary of // data contracts will be created and re-assigned to this field. Introduction // of a lock here could lead to deadlocks. this.knownDataContracts = XmlObjectSerializerContext.GetDataContractsForKnownTypes(this.knownTypeList); } return this.knownDataContracts; } } public int MaxItemsInObjectGraph { get { return maxItemsInObjectGraph; } } public IDataContractSurrogate DataContractSurrogate { get { return dataContractSurrogate; } } public bool PreserveObjectReferences { get { return preserveObjectReferences; } } public bool IgnoreExtensionDataObject { get { return ignoreExtensionDataObject; } } public DataContractResolver DataContractResolver { get { return dataContractResolver; } } DataContract RootContract { get { if (rootContract == null) { rootContract = DataContract.GetDataContract(((dataContractSurrogate == null) ? rootType : GetSurrogatedType(dataContractSurrogate, rootType))); needsContractNsAtRoot = CheckIfNeedsContractNsAtRoot(rootName, rootNamespace, rootContract); } return rootContract; } } internal override void InternalWriteObject(XmlWriterDelegator writer, object graph) { InternalWriteObject(writer, graph, null); } internal override void InternalWriteObject(XmlWriterDelegator writer, object graph, DataContractResolver dataContractResolver) { InternalWriteStartObject(writer, graph); InternalWriteObjectContent(writer, graph, dataContractResolver); InternalWriteEndObject(writer); } public override void WriteObject(XmlWriter writer, object graph) { WriteObjectHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteStartObject(XmlWriter writer, object graph) { WriteStartObjectHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteObjectContent(XmlWriter writer, object graph) { WriteObjectContentHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteEndObject(XmlWriter writer) { WriteEndObjectHandleExceptions(new XmlWriterDelegator(writer)); } public override void WriteStartObject(XmlDictionaryWriter writer, object graph) { WriteStartObjectHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteObjectContent(XmlDictionaryWriter writer, object graph) { WriteObjectContentHandleExceptions(new XmlWriterDelegator(writer), graph); } public override void WriteEndObject(XmlDictionaryWriter writer) { WriteEndObjectHandleExceptions(new XmlWriterDelegator(writer)); } public void WriteObject(XmlDictionaryWriter writer, object graph, DataContractResolver dataContractResolver) { WriteObjectHandleExceptions(new XmlWriterDelegator(writer), graph, dataContractResolver); } public override object ReadObject(XmlReader reader) { return ReadObjectHandleExceptions(new XmlReaderDelegator(reader), true /*verifyObjectName*/); } public override object ReadObject(XmlReader reader, bool verifyObjectName) { return ReadObjectHandleExceptions(new XmlReaderDelegator(reader), verifyObjectName); } public override bool IsStartObject(XmlReader reader) { return IsStartObjectHandleExceptions(new XmlReaderDelegator(reader)); } public override object ReadObject(XmlDictionaryReader reader, bool verifyObjectName) { return ReadObjectHandleExceptions(new XmlReaderDelegator(reader), verifyObjectName); } public override bool IsStartObject(XmlDictionaryReader reader) { return IsStartObjectHandleExceptions(new XmlReaderDelegator(reader)); } public object ReadObject(XmlDictionaryReader reader, bool verifyObjectName, DataContractResolver dataContractResolver) { return ReadObjectHandleExceptions(new XmlReaderDelegator(reader), verifyObjectName, dataContractResolver); } internal override void InternalWriteStartObject(XmlWriterDelegator writer, object graph) { WriteRootElement(writer, RootContract, rootName, rootNamespace, needsContractNsAtRoot); } internal override void InternalWriteObjectContent(XmlWriterDelegator writer, object graph) { InternalWriteObjectContent(writer, graph, null); } internal void InternalWriteObjectContent(XmlWriterDelegator writer, object graph, DataContractResolver dataContractResolver) { if (MaxItemsInObjectGraph == 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.ExceededMaxItemsQuota, MaxItemsInObjectGraph))); DataContract contract = RootContract; Type declaredType = contract.UnderlyingType; Type graphType = (graph == null) ? declaredType : graph.GetType(); if (dataContractSurrogate != null) graph = SurrogateToDataContractType(dataContractSurrogate, graph, declaredType, ref graphType); if (dataContractResolver == null) dataContractResolver = this.DataContractResolver; if (graph == null) { if (IsRootXmlAny(rootName, contract)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.IsAnyCannotBeNull, declaredType))); WriteNull(writer); } else { if (declaredType == graphType) { if (contract.CanContainReferences) { XmlObjectSerializerWriteContext context = XmlObjectSerializerWriteContext.CreateContext(this, contract, dataContractResolver); context.HandleGraphAtTopLevel(writer, graph, contract); context.SerializeWithoutXsiType(contract, writer, graph, declaredType.TypeHandle); } else { contract.WriteXmlValue(writer, graph, null); } } else { XmlObjectSerializerWriteContext context = null; if (IsRootXmlAny(rootName, contract)) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.IsAnyCannotBeSerializedAsDerivedType, graphType, contract.UnderlyingType))); contract = GetDataContract(contract, declaredType, graphType); context = XmlObjectSerializerWriteContext.CreateContext(this, RootContract, dataContractResolver); if (contract.CanContainReferences) { context.HandleGraphAtTopLevel(writer, graph, contract); } context.OnHandleIsReference(writer, contract, graph); context.SerializeWithXsiTypeAtTopLevel(contract, writer, graph, declaredType.TypeHandle, graphType); } } } internal static DataContract GetDataContract(DataContract declaredTypeContract, Type declaredType, Type objectType) { if (declaredType.IsInterface && CollectionDataContract.IsCollectionInterface(declaredType)) { return declaredTypeContract; } else if (declaredType.IsArray)//Array covariance is not supported in XSD { return declaredTypeContract; } else { return DataContract.GetDataContract(objectType.TypeHandle, objectType, SerializationMode.SharedContract); } } internal override void InternalWriteEndObject(XmlWriterDelegator writer) { if (!IsRootXmlAny(rootName, RootContract)) { writer.WriteEndElement(); } } internal override object InternalReadObject(XmlReaderDelegator xmlReader, bool verifyObjectName) { return InternalReadObject(xmlReader, verifyObjectName, null); } internal override object InternalReadObject(XmlReaderDelegator xmlReader, bool verifyObjectName, DataContractResolver dataContractResolver) { if (MaxItemsInObjectGraph == 0) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationException(SR.GetString(SR.ExceededMaxItemsQuota, MaxItemsInObjectGraph))); if (dataContractResolver == null) dataContractResolver = this.DataContractResolver; if (verifyObjectName) { if (!InternalIsStartObject(xmlReader)) { XmlDictionaryString expectedName; XmlDictionaryString expectedNs; if (rootName == null) { expectedName = RootContract.TopLevelElementName; expectedNs = RootContract.TopLevelElementNamespace; } else { expectedName = rootName; expectedNs = rootNamespace; } throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationExceptionWithReaderDetails(SR.GetString(SR.ExpectingElement, expectedNs, expectedName), xmlReader)); } } else if (!IsStartElement(xmlReader)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(XmlObjectSerializer.CreateSerializationExceptionWithReaderDetails(SR.GetString(SR.ExpectingElementAtDeserialize, XmlNodeType.Element), xmlReader)); } DataContract contract = RootContract; if (contract.IsPrimitive && object.ReferenceEquals(contract.UnderlyingType, rootType) /*handle Nullable differently*/) { return contract.ReadXmlValue(xmlReader, null); } if (IsRootXmlAny(rootName, contract)) { return XmlObjectSerializerReadContext.ReadRootIXmlSerializable(xmlReader, contract as XmlDataContract, false /*isMemberType*/); } XmlObjectSerializerReadContext context = XmlObjectSerializerReadContext.CreateContext(this, contract, dataContractResolver); return context.InternalDeserialize(xmlReader, rootType, contract, null, null); } internal override bool InternalIsStartObject(XmlReaderDelegator reader) { return IsRootElement(reader, RootContract, rootName, rootNamespace); } internal override Type GetSerializeType(object graph) { return (graph == null) ? rootType : graph.GetType(); } internal override Type GetDeserializeType() { return rootType; } internal static object SurrogateToDataContractType(IDataContractSurrogate dataContractSurrogate, object oldObj, Type surrogatedDeclaredType, ref Type objType) { object obj = DataContractSurrogateCaller.GetObjectToSerialize(dataContractSurrogate, oldObj, objType, surrogatedDeclaredType); if (obj != oldObj) { if (obj == null) objType = Globals.TypeOfObject; else objType = obj.GetType(); } return obj; } internal static Type GetSurrogatedType(IDataContractSurrogate dataContractSurrogate, Type type) { return DataContractSurrogateCaller.GetDataContractType(dataContractSurrogate, DataContract.UnwrapNullableType(type)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
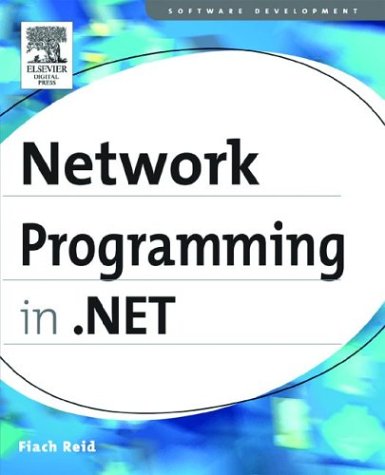
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DeflateStreamAsyncResult.cs
- ListViewInsertEventArgs.cs
- NamedPermissionSet.cs
- ComponentTray.cs
- PartBasedPackageProperties.cs
- VBCodeProvider.cs
- ValidationEventArgs.cs
- Run.cs
- DocumentOrderComparer.cs
- QuaternionAnimation.cs
- ConnectionsZoneDesigner.cs
- MsmqIntegrationBindingElement.cs
- TextContainer.cs
- DbProviderServices.cs
- PersonalizablePropertyEntry.cs
- NoClickablePointException.cs
- CheckBoxStandardAdapter.cs
- ConfigurationSectionCollection.cs
- TdsRecordBufferSetter.cs
- CodeAccessSecurityEngine.cs
- Figure.cs
- ClientRoleProvider.cs
- TemplateBuilder.cs
- ClassHandlersStore.cs
- CreateDataSourceDialog.cs
- TypeDescriptorContext.cs
- HwndMouseInputProvider.cs
- ToolStripLocationCancelEventArgs.cs
- RowTypeElement.cs
- BeginStoryboard.cs
- DateTimeUtil.cs
- BaseCollection.cs
- UniqueIdentifierService.cs
- TypeDependencyAttribute.cs
- TraceContextRecord.cs
- BamlRecords.cs
- HandlerFactoryWrapper.cs
- DesignerActionUI.cs
- TextBoxBase.cs
- SqlDataSourceAdvancedOptionsForm.cs
- InkCanvasAutomationPeer.cs
- DataGridCommandEventArgs.cs
- ImplicitInputBrush.cs
- CharacterMetricsDictionary.cs
- WSSecurityPolicy.cs
- ToolBarButton.cs
- TargetInvocationException.cs
- ToolboxDataAttribute.cs
- LightweightCodeGenerator.cs
- OdbcError.cs
- SoapAttributeAttribute.cs
- CollectionConverter.cs
- DbSourceCommand.cs
- HttpValueCollection.cs
- AnnotationComponentManager.cs
- XmlElementAttributes.cs
- EmptyCollection.cs
- SystemParameters.cs
- Separator.cs
- WinEventHandler.cs
- Maps.cs
- DrawListViewColumnHeaderEventArgs.cs
- PopupRoot.cs
- PathFigureCollection.cs
- HttpPostClientProtocol.cs
- DbParameterHelper.cs
- BoundPropertyEntry.cs
- StdValidatorsAndConverters.cs
- DiscoveryDocumentLinksPattern.cs
- DoubleAnimationUsingKeyFrames.cs
- DiscardableAttribute.cs
- SqlDeflator.cs
- BaseServiceProvider.cs
- InputMethodStateChangeEventArgs.cs
- DefaultPerformanceCounters.cs
- PathGradientBrush.cs
- BaseDataListPage.cs
- ScrollBarAutomationPeer.cs
- Parameter.cs
- HttpResponseHeader.cs
- CheckBox.cs
- WS2007FederationHttpBindingElement.cs
- Regex.cs
- ModuleBuilderData.cs
- DecoderFallback.cs
- TimersDescriptionAttribute.cs
- TCPClient.cs
- PathParser.cs
- InputLangChangeRequestEvent.cs
- ColorContext.cs
- StaticExtensionConverter.cs
- WsiProfilesElement.cs
- DataGridPagingPage.cs
- MenuItem.cs
- panel.cs
- CallId.cs
- CodeCastExpression.cs
- WebZone.cs
- XomlSerializationHelpers.cs
- XPathConvert.cs