Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / MsmqIntegration / MsmqIntegrationBindingElement.cs / 1 / MsmqIntegrationBindingElement.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.MsmqIntegration { using System.Net.Security; using System.Runtime.Serialization; using System.ServiceModel.Channels; using System.Collections.Generic; public sealed class MsmqIntegrationBindingElement : MsmqBindingElementBase { MsmqMessageSerializationFormat serializationFormat; Type[] targetSerializationTypes; public MsmqIntegrationBindingElement() { this.serializationFormat = MsmqIntegrationDefaults.SerializationFormat; } MsmqIntegrationBindingElement(MsmqIntegrationBindingElement other) : base(other) { this.serializationFormat = other.serializationFormat; if (other.targetSerializationTypes != null) { this.targetSerializationTypes = other.targetSerializationTypes.Clone() as Type[]; } } public override string Scheme { get { return "msmq.formatname"; } } internal override MsmqUri.IAddressTranslator AddressTranslator { get { return MsmqUri.FormatNameAddressTranslator; } } // applicable on: client, server public MsmqMessageSerializationFormat SerializationFormat { get { return this.serializationFormat; } set { if (!MsmqMessageSerializationFormatHelper.IsDefined(value)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ArgumentOutOfRangeException("value")); } this.serializationFormat = value; } } // applicable on: receiver public Type[] TargetSerializationTypes { get { if (null == this.targetSerializationTypes) return null; else return this.targetSerializationTypes.Clone() as Type[]; } set { if (null == value) this.targetSerializationTypes = null; else this.targetSerializationTypes = value.Clone() as Type[]; } } public override BindingElement Clone() { return new MsmqIntegrationBindingElement(this); } public override bool CanBuildChannelFactory(BindingContext context) { return typeof(TChannel) == typeof(IOutputChannel); } public override bool CanBuildChannelListener (BindingContext context) { return typeof(TChannel) == typeof(IInputChannel); } public override IChannelFactory BuildChannelFactory (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (typeof(TChannel) != typeof(IOutputChannel)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("TChannel", SR.GetString(SR.ChannelTypeNotSupported, typeof(TChannel))); } MsmqChannelFactoryBase factory = new MsmqIntegrationChannelFactory(this, context); MsmqVerifier.VerifySender (factory); return (IChannelFactory )(object)factory; } public override IChannelListener BuildChannelListener (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (typeof(TChannel) != typeof(IInputChannel)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgument("TChannel", SR.GetString(SR.ChannelTypeNotSupported, typeof(TChannel))); } MsmqIntegrationReceiveParameters receiveParameters = new MsmqIntegrationReceiveParameters(this); MsmqIntegrationChannelListener listener = new MsmqIntegrationChannelListener(this, context, receiveParameters); MsmqVerifier.VerifyReceiver(receiveParameters, listener.Uri); return (IChannelListener )(object)listener; } public override T GetProperty (BindingContext context) { if (context == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("context"); } if (typeof(T) == typeof(MessageVersion)) { return (T)(object)(MessageVersion.None); } else { return base.GetProperty (context); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
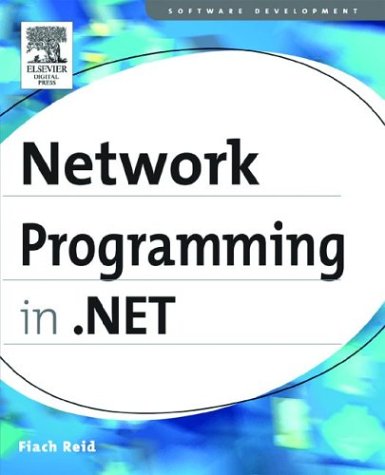
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- KnowledgeBase.cs
- SiteMapProvider.cs
- Mapping.cs
- CfgRule.cs
- ZeroOpNode.cs
- RuntimeCompatibilityAttribute.cs
- WeakReferenceList.cs
- FileFormatException.cs
- ISessionStateStore.cs
- DataBoundControl.cs
- hwndwrapper.cs
- WhitespaceRule.cs
- ViewManager.cs
- StructuredProperty.cs
- MachineKeyConverter.cs
- CanonicalXml.cs
- CompilationSection.cs
- CqlParser.cs
- TextParagraphCache.cs
- ActivitiesCollection.cs
- DictionaryKeyPropertyAttribute.cs
- NativeMethods.cs
- SocketStream.cs
- XmlResolver.cs
- SafeNativeMethods.cs
- HwndAppCommandInputProvider.cs
- SafeNativeMethodsMilCoreApi.cs
- StatusBarAutomationPeer.cs
- PersistenceProvider.cs
- MetadataItemCollectionFactory.cs
- MouseDevice.cs
- TableLayout.cs
- BooleanConverter.cs
- PartialCachingAttribute.cs
- ResourcesBuildProvider.cs
- ListItemParagraph.cs
- CompilerState.cs
- ShaderRenderModeValidation.cs
- UnmanagedMarshal.cs
- AuthenticationManager.cs
- DispatcherExceptionFilterEventArgs.cs
- AssemblyResourceLoader.cs
- DeferredRunTextReference.cs
- __Error.cs
- XmlException.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- _AutoWebProxyScriptWrapper.cs
- BindingMAnagerBase.cs
- ButtonFieldBase.cs
- QEncodedStream.cs
- BitmapEffect.cs
- XmlSchema.cs
- XmlSchemaSimpleContent.cs
- SingleAnimationUsingKeyFrames.cs
- DataGridBeginningEditEventArgs.cs
- WebPartConnectionsDisconnectVerb.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- KoreanLunisolarCalendar.cs
- UInt64.cs
- SafeViewOfFileHandle.cs
- SimpleType.cs
- IncrementalReadDecoders.cs
- AttributeExtensions.cs
- LinqDataSourceSelectEventArgs.cs
- RelationshipEndMember.cs
- HierarchicalDataSourceConverter.cs
- PathStreamGeometryContext.cs
- DbParameterCollectionHelper.cs
- NamedObject.cs
- HttpResponseBase.cs
- LinkLabel.cs
- XmlBindingWorker.cs
- ClrProviderManifest.cs
- TextRenderer.cs
- InitializationEventAttribute.cs
- WaitHandle.cs
- XmlUtil.cs
- tooltip.cs
- GenericsInstances.cs
- SoapFault.cs
- OutputCacheProfileCollection.cs
- StringInfo.cs
- ReferenceCountedObject.cs
- QuaternionRotation3D.cs
- XhtmlTextWriter.cs
- TextEditorTyping.cs
- CreateUserWizardStep.cs
- GetPageNumberCompletedEventArgs.cs
- LongMinMaxAggregationOperator.cs
- TreeNodeSelectionProcessor.cs
- IgnoreSectionHandler.cs
- VisemeEventArgs.cs
- BlockCollection.cs
- Repeater.cs
- EndpointAddressMessageFilter.cs
- XamlHostingConfiguration.cs
- DateTimeConstantAttribute.cs
- QueueAccessMode.cs
- BasicDesignerLoader.cs
- Polygon.cs