Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / RenderDataDrawingContext.cs / 1305600 / RenderDataDrawingContext.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: RenderDataDrawingContext.cs // // History: // [....]: 04/19/2003 // Created it based on the RenderDataDrawingContext used in the AvPhat branch. // [....]: 07/02/2003 // Renamed to RenderDataDrawingContext, which derives from DrawingContext // [....]: 07/02/2003 // Renamed to RenderDataDrawingContext, which derives from DrawingContext // This class no longer understand Visuals, and simply produces RenderData. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Security.Permissions; using System.Windows; using System.Windows.Threading; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Diagnostics; using MS.Internal; using System.Security; //----------------------------------------------------------------------------- // This section lists various things that we could improve on the DrawingContxt // class. // // - Remove the isAnimated flag from being propagated everywhere. Rather mark // the pail when we add an animated argument. //----------------------------------------------------------------------------- namespace System.Windows.Media { ////// Drawing context. /// internal partial class RenderDataDrawingContext : DrawingContext, IDisposable { #region Constructors ////// Creates a drawing context which is associated with a given Dispatcher /// internal RenderDataDrawingContext() { } #endregion Constructors #region Public Methods internal RenderData GetRenderData() { return _renderData; } ////// Closes the DrawingContext and flushes the content. /// Afterwards the DrawingContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// public override void Close() { VerifyApiNonstructuralChange(); ((IDisposable)this).Dispose(); } ////// This is the same as the Close call: /// Closes the DrawingContext and flushes the content. /// Afterwards the DrawingContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// protected override void DisposeCore() { if (!_disposed) { EnsureCorrectNesting(); CloseCore(_renderData); _disposed = true; } } #endregion Public Methods #region Protected Methods ////// CloseCore - Implemented be derivees to Close the context. /// This will only be called once (if ever) per instance. /// /// The render data produced by this RenderDataDrawingContext. protected virtual void CloseCore(RenderData renderData) {} #endregion Protected Methods #region Private Methods ////// EnsureRenderData - this method ensures that the _renderData variable is initialized. /// The render data's _buffer will be lazily instantiated via the Draw* methods which all /// call WriteDataRecord. /// private void EnsureRenderData() { if (_renderData == null) { _renderData = new RenderData(); } } ////// This verifies that the API can be called for access which doesn't affect structure. /// protected override void VerifyApiNonstructuralChange() { base.VerifyApiNonstructuralChange(); if (_disposed) { throw new ObjectDisposedException("RenderDataDrawingContext"); } } ////// This method checks if there were any Push calls that were not matched by /// the corresponding Pop. If this is the case, this method adds corresponding /// number of Pop instructions to the render data. /// private void EnsureCorrectNesting() { if (_renderData != null && _stackDepth > 0) { int stackDepth = _stackDepth; for (int i = 0; i < stackDepth; i++) { Pop(); } } _stackDepth = 0; } #endregion Private Methods #region Fields private RenderData _renderData; private bool _disposed; private int _stackDepth; #endregion Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: RenderDataDrawingContext.cs // // History: // [....]: 04/19/2003 // Created it based on the RenderDataDrawingContext used in the AvPhat branch. // [....]: 07/02/2003 // Renamed to RenderDataDrawingContext, which derives from DrawingContext // [....]: 07/02/2003 // Renamed to RenderDataDrawingContext, which derives from DrawingContext // This class no longer understand Visuals, and simply produces RenderData. // //----------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Security.Permissions; using System.Windows; using System.Windows.Threading; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Diagnostics; using MS.Internal; using System.Security; //----------------------------------------------------------------------------- // This section lists various things that we could improve on the DrawingContxt // class. // // - Remove the isAnimated flag from being propagated everywhere. Rather mark // the pail when we add an animated argument. //----------------------------------------------------------------------------- namespace System.Windows.Media { ////// Drawing context. /// internal partial class RenderDataDrawingContext : DrawingContext, IDisposable { #region Constructors ////// Creates a drawing context which is associated with a given Dispatcher /// internal RenderDataDrawingContext() { } #endregion Constructors #region Public Methods internal RenderData GetRenderData() { return _renderData; } ////// Closes the DrawingContext and flushes the content. /// Afterwards the DrawingContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// public override void Close() { VerifyApiNonstructuralChange(); ((IDisposable)this).Dispose(); } ////// This is the same as the Close call: /// Closes the DrawingContext and flushes the content. /// Afterwards the DrawingContext can not be used anymore. /// This call does not require all Push calls to have been Popped. /// ////// This call is illegal if this object has already been closed or disposed. /// protected override void DisposeCore() { if (!_disposed) { EnsureCorrectNesting(); CloseCore(_renderData); _disposed = true; } } #endregion Public Methods #region Protected Methods ////// CloseCore - Implemented be derivees to Close the context. /// This will only be called once (if ever) per instance. /// /// The render data produced by this RenderDataDrawingContext. protected virtual void CloseCore(RenderData renderData) {} #endregion Protected Methods #region Private Methods ////// EnsureRenderData - this method ensures that the _renderData variable is initialized. /// The render data's _buffer will be lazily instantiated via the Draw* methods which all /// call WriteDataRecord. /// private void EnsureRenderData() { if (_renderData == null) { _renderData = new RenderData(); } } ////// This verifies that the API can be called for access which doesn't affect structure. /// protected override void VerifyApiNonstructuralChange() { base.VerifyApiNonstructuralChange(); if (_disposed) { throw new ObjectDisposedException("RenderDataDrawingContext"); } } ////// This method checks if there were any Push calls that were not matched by /// the corresponding Pop. If this is the case, this method adds corresponding /// number of Pop instructions to the render data. /// private void EnsureCorrectNesting() { if (_renderData != null && _stackDepth > 0) { int stackDepth = _stackDepth; for (int i = 0; i < stackDepth; i++) { Pop(); } } _stackDepth = 0; } #endregion Private Methods #region Fields private RenderData _renderData; private bool _disposed; private int _stackDepth; #endregion Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
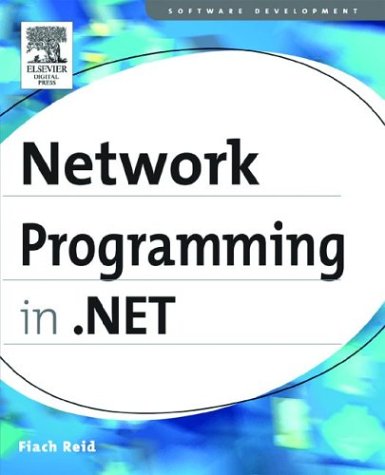
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _NegoStream.cs
- TiffBitmapEncoder.cs
- TextTreeTextNode.cs
- ParseChildrenAsPropertiesAttribute.cs
- COM2ExtendedTypeConverter.cs
- BackStopAuthenticationModule.cs
- HandlerFactoryWrapper.cs
- configsystem.cs
- ArrayElementGridEntry.cs
- XmlBoundElement.cs
- MissingFieldException.cs
- __Error.cs
- DropSource.cs
- EntityDataSourceReferenceGroup.cs
- ProgressBar.cs
- CrossSiteScriptingValidation.cs
- CapabilitiesUse.cs
- Timeline.cs
- EraserBehavior.cs
- FontInfo.cs
- EventHandlersStore.cs
- DetailsViewPagerRow.cs
- DrawingContext.cs
- TextElementEnumerator.cs
- FormCollection.cs
- InternalPermissions.cs
- ChannelBinding.cs
- DoubleAnimationBase.cs
- CommandEventArgs.cs
- PrintPreviewGraphics.cs
- SaveFileDialog.cs
- ExcCanonicalXml.cs
- SessionEndingCancelEventArgs.cs
- SectionVisual.cs
- RegexWriter.cs
- ItemDragEvent.cs
- EntityDataSourceStatementEditor.cs
- XmlKeywords.cs
- EncodingDataItem.cs
- StringAttributeCollection.cs
- WebZone.cs
- QueryException.cs
- ImageFormat.cs
- DetailsView.cs
- RegisteredScript.cs
- MsdtcClusterUtils.cs
- SystemIPGlobalProperties.cs
- IOThreadTimer.cs
- MemoryMappedViewAccessor.cs
- CalendarModeChangedEventArgs.cs
- SplineKeyFrames.cs
- ObjectStateFormatter.cs
- GeneralTransform3DGroup.cs
- ExceptionValidationRule.cs
- RandomNumberGenerator.cs
- ToolStripPanel.cs
- Property.cs
- OutputCacheModule.cs
- RNGCryptoServiceProvider.cs
- MailMessageEventArgs.cs
- X509CertificateCollection.cs
- ValidationErrorCollection.cs
- Soap12ProtocolReflector.cs
- ScrollChrome.cs
- RequiredFieldValidator.cs
- HtmlInputRadioButton.cs
- BinaryObjectWriter.cs
- CodeAccessSecurityEngine.cs
- SQLBoolean.cs
- figurelength.cs
- RunInstallerAttribute.cs
- NavigationPropertySingletonExpression.cs
- MulticastNotSupportedException.cs
- DecimalKeyFrameCollection.cs
- Menu.cs
- recordstatescratchpad.cs
- SymDocumentType.cs
- WebPartConnectionCollection.cs
- SizeIndependentAnimationStorage.cs
- EventLogPropertySelector.cs
- Debugger.cs
- EpmTargetPathSegment.cs
- IPEndPointCollection.cs
- ZipPackage.cs
- TypeUtil.cs
- ProcessThread.cs
- DecoderFallback.cs
- Int64Converter.cs
- ContentValidator.cs
- ChtmlTextWriter.cs
- StylusPointDescription.cs
- InputDevice.cs
- NetTcpSecurityElement.cs
- UserControl.cs
- IdleTimeoutMonitor.cs
- UserInitiatedNavigationPermission.cs
- Fonts.cs
- ObjectSecurity.cs
- KnowledgeBase.cs
- Membership.cs