Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / Advanced / ImageFormat.cs / 1 / ImageFormat.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /*************************************************************************\ * * Copyright (c) 1998-1999, Microsoft Corp. All Rights Reserved. * * Module Name: * * ImageFormat.cs * * Abstract: * * Image format constant types * * Revision History: * * 12/14/1998 [....] * Created it. * \**************************************************************************/ namespace System.Drawing.Imaging { using System; using System.Diagnostics; using System.Drawing; using System.ComponentModel; /** * Image format constants */ ////// /// Specifies the format of the image. /// [TypeConverterAttribute(typeof(ImageFormatConverter))] public sealed class ImageFormat { // Format IDs // private static ImageFormat undefined = new ImageFormat(new Guid("{b96b3ca9-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat memoryBMP = new ImageFormat(new Guid("{b96b3caa-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat bmp = new ImageFormat(new Guid("{b96b3cab-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat emf = new ImageFormat(new Guid("{b96b3cac-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat wmf = new ImageFormat(new Guid("{b96b3cad-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat jpeg = new ImageFormat(new Guid("{b96b3cae-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat png = new ImageFormat(new Guid("{b96b3caf-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat gif = new ImageFormat(new Guid("{b96b3cb0-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat tiff = new ImageFormat(new Guid("{b96b3cb1-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat exif = new ImageFormat(new Guid("{b96b3cb2-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat photoCD = new ImageFormat(new Guid("{b96b3cb3-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat flashPIX = new ImageFormat(new Guid("{b96b3cb4-0728-11d3-9d7b-0000f81ef32e}")); private static ImageFormat icon = new ImageFormat(new Guid("{b96b3cb5-0728-11d3-9d7b-0000f81ef32e}")); private Guid guid; ////// /// Initializes a new instance of the public ImageFormat(Guid guid) { this.guid = guid; } ///class with the specified GUID. /// /// /// Specifies a global unique identifier (GUID) /// that represents this public Guid Guid { get { return guid;} } ///. /// /// /// Specifies a memory bitmap image format. /// public static ImageFormat MemoryBmp { get { return memoryBMP;} } ////// /// Specifies the bitmap image format. /// public static ImageFormat Bmp { get { return bmp;} } ////// /// Specifies the enhanced Windows metafile /// image format. /// public static ImageFormat Emf { get { return emf;} } ////// /// Specifies the Windows metafile image /// format. /// public static ImageFormat Wmf { get { return wmf;} } ////// /// Specifies the GIF image format. /// public static ImageFormat Gif { get { return gif;} } ////// /// Specifies the JPEG image format. /// public static ImageFormat Jpeg { get { return jpeg;} } ////// /// public static ImageFormat Png { get { return png;} } ////// Specifies the W3C PNG image format. /// ////// /// Specifies the Tag Image File /// Format (TIFF) image format. /// public static ImageFormat Tiff { get { return tiff;} } ////// /// Specifies the Exchangable Image Format /// (EXIF). /// public static ImageFormat Exif { get { return exif;} } ////// /// public static ImageFormat Icon { get { return icon;} } ////// Specifies the Windows icon image format. /// ////// /// Returns a value indicating whether the /// specified object is an public override bool Equals(object o) { ImageFormat format = o as ImageFormat; if (format == null) return false; return this.guid == format.guid; } ///equivalent to this . /// /// /// public override int GetHashCode() { return this.guid.GetHashCode(); } #if !FEATURE_PAL // Find any random encoder which supports this format internal ImageCodecInfo FindEncoder() { ImageCodecInfo[] codecs = ImageCodecInfo.GetImageEncoders(); foreach (ImageCodecInfo codec in codecs) { if (codec.FormatID.Equals(this.guid)) return codec; } return null; } #endif ////// Returns a hash code. /// ////// /// Converts this public override string ToString() { if (this == memoryBMP) return "MemoryBMP"; if (this == bmp) return "Bmp"; if (this == emf) return "Emf"; if (this == wmf) return "Wmf"; if (this == gif) return "Gif"; if (this == jpeg) return "Jpeg"; if (this == png) return "Png"; if (this == tiff) return "Tiff"; if (this == exif) return "Exif"; if (this == icon) return "Icon"; return "[ImageFormat: " + guid + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.to a human-readable string. ///
Link Menu
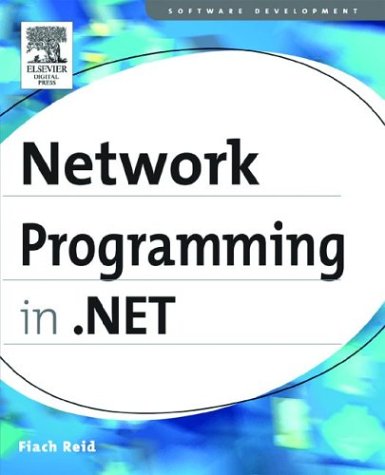
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HttpFormatExtensions.cs
- XmlSchemaExporter.cs
- FixedPageAutomationPeer.cs
- XmlUtilWriter.cs
- VSWCFServiceContractGenerator.cs
- CachedCompositeFamily.cs
- SqlInternalConnection.cs
- SecurityTokenInclusionMode.cs
- InstalledFontCollection.cs
- SignatureDescription.cs
- AspNetCacheProfileAttribute.cs
- SoapAttributeAttribute.cs
- AncillaryOps.cs
- DesignerProperties.cs
- IteratorDescriptor.cs
- ListParaClient.cs
- ComEventsHelper.cs
- SiteMapDataSource.cs
- ManipulationDevice.cs
- EntryPointNotFoundException.cs
- Trigger.cs
- DbConnectionHelper.cs
- IndentedWriter.cs
- DataGridViewHeaderCell.cs
- StyleSheet.cs
- ColorConverter.cs
- TextEffectCollection.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- SmtpFailedRecipientsException.cs
- TextFindEngine.cs
- UpdatePanelControlTrigger.cs
- UnhandledExceptionEventArgs.cs
- TextSimpleMarkerProperties.cs
- IListConverters.cs
- TextSearch.cs
- SMSvcHost.cs
- JsonObjectDataContract.cs
- DataGridGeneralPage.cs
- Geometry.cs
- BidOverLoads.cs
- ScriptResourceInfo.cs
- CompatibleComparer.cs
- HealthMonitoringSection.cs
- TemplatePagerField.cs
- SQLDoubleStorage.cs
- SqlFlattener.cs
- TypeElement.cs
- SettingsAttributes.cs
- TypeInitializationException.cs
- InternalDuplexChannelFactory.cs
- ChtmlPageAdapter.cs
- LocalizedNameDescriptionPair.cs
- QueueException.cs
- DbProviderFactories.cs
- _Win32.cs
- WebPartUtil.cs
- MultiSelectRootGridEntry.cs
- localization.cs
- DataProviderNameConverter.cs
- SqlPersonalizationProvider.cs
- HeaderLabel.cs
- Faults.cs
- XsltInput.cs
- Crypto.cs
- FieldInfo.cs
- KeyMatchBuilder.cs
- SoundPlayerAction.cs
- IdentitySection.cs
- SiteMapPath.cs
- MemberRelationshipService.cs
- OracleParameterCollection.cs
- WebDescriptionAttribute.cs
- DataServiceHost.cs
- PackWebRequest.cs
- CompoundFileStorageReference.cs
- WebControlsSection.cs
- UserControlCodeDomTreeGenerator.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- WebResourceAttribute.cs
- MarkupCompilePass1.cs
- KeyBinding.cs
- CustomAttribute.cs
- GestureRecognitionResult.cs
- RelationshipDetailsRow.cs
- MD5Cng.cs
- TableItemStyle.cs
- SessionStateItemCollection.cs
- SmiXetterAccessMap.cs
- TextComposition.cs
- BinaryMethodMessage.cs
- TimelineGroup.cs
- X509ClientCertificateAuthentication.cs
- ComponentResourceKey.cs
- XPathAncestorIterator.cs
- ZipFileInfoCollection.cs
- FontInfo.cs
- TransportConfigurationTypeElementCollection.cs
- ToolboxSnapDragDropEventArgs.cs
- DefaultAssemblyResolver.cs
- TextBox.cs