Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Ink / StrokeNodeData.cs / 1305600 / StrokeNodeData.cs
//------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Windows; using System.Windows.Media; using System.Windows.Input; using System.Diagnostics; namespace MS.Internal.Ink { #region StrokeNodeData ////// This structure represents a node on a stroke spine. /// internal struct StrokeNodeData { #region Statics private static StrokeNodeData s_empty = new StrokeNodeData(); #endregion #region API (internal) ///Returns static object representing an unitialized node internal static StrokeNodeData Empty { get { return s_empty; } } ////// Constructor for nodes of a pressure insensitive stroke /// /// position of the node internal StrokeNodeData(Point position) { _position = position; _pressure = 1; } ////// Constructor for nodes with pressure data /// /// position of the node /// pressure scaling factor at the node internal StrokeNodeData(Point position, float pressure) { System.Diagnostics.Debug.Assert(DoubleUtil.GreaterThan((double)pressure, 0d)); _position = position; _pressure = pressure; } ///Tells whether the structre was properly initialized internal bool IsEmpty { get { Debug.Assert(DoubleUtil.AreClose(0, s_empty._pressure)); return DoubleUtil.AreClose(_pressure, s_empty._pressure); } } ///Position of the node internal Point Position { get { return _position; } } ///Pressure scaling factor at the node internal float PressureFactor { get { return _pressure; } } #endregion #region Privates private Point _position; private float _pressure; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Windows; using System.Windows.Media; using System.Windows.Input; using System.Diagnostics; namespace MS.Internal.Ink { #region StrokeNodeData ////// This structure represents a node on a stroke spine. /// internal struct StrokeNodeData { #region Statics private static StrokeNodeData s_empty = new StrokeNodeData(); #endregion #region API (internal) ///Returns static object representing an unitialized node internal static StrokeNodeData Empty { get { return s_empty; } } ////// Constructor for nodes of a pressure insensitive stroke /// /// position of the node internal StrokeNodeData(Point position) { _position = position; _pressure = 1; } ////// Constructor for nodes with pressure data /// /// position of the node /// pressure scaling factor at the node internal StrokeNodeData(Point position, float pressure) { System.Diagnostics.Debug.Assert(DoubleUtil.GreaterThan((double)pressure, 0d)); _position = position; _pressure = pressure; } ///Tells whether the structre was properly initialized internal bool IsEmpty { get { Debug.Assert(DoubleUtil.AreClose(0, s_empty._pressure)); return DoubleUtil.AreClose(_pressure, s_empty._pressure); } } ///Position of the node internal Point Position { get { return _position; } } ///Pressure scaling factor at the node internal float PressureFactor { get { return _pressure; } } #endregion #region Privates private Point _position; private float _pressure; #endregion } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
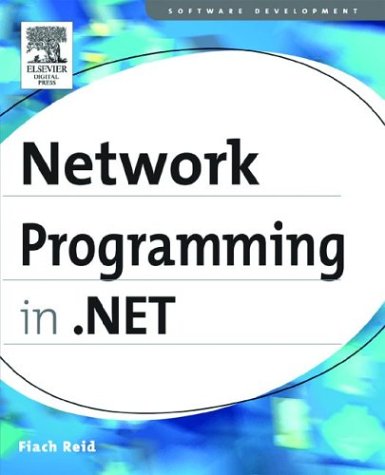
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ResizeGrip.cs
- ZipIORawDataFileBlock.cs
- CallbackHandler.cs
- RoleService.cs
- PrintControllerWithStatusDialog.cs
- DateTimeConverter.cs
- ObjectIDGenerator.cs
- TableLayoutCellPaintEventArgs.cs
- DbParameterCollectionHelper.cs
- TreeViewAutomationPeer.cs
- DynamicPropertyHolder.cs
- PopupRoot.cs
- Dictionary.cs
- _RequestCacheProtocol.cs
- ErrorHandlerModule.cs
- AsmxEndpointPickerExtension.cs
- ApplicationSecurityInfo.cs
- CodeTypeMember.cs
- KeysConverter.cs
- SymmetricAlgorithm.cs
- AtomMaterializerLog.cs
- ItemChangedEventArgs.cs
- TemplateContainer.cs
- StringFormat.cs
- ScalarType.cs
- SqlCaseSimplifier.cs
- ScrollChrome.cs
- TypeConstant.cs
- BinaryParser.cs
- FileDialog_Vista_Interop.cs
- TraceRecord.cs
- WorkflowInstanceRecord.cs
- Win32KeyboardDevice.cs
- EnterpriseServicesHelper.cs
- Transform3DCollection.cs
- Codec.cs
- ICspAsymmetricAlgorithm.cs
- SizeValueSerializer.cs
- filewebresponse.cs
- WebPartsSection.cs
- AutoSizeComboBox.cs
- GetIndexBinder.cs
- ClassicBorderDecorator.cs
- Clause.cs
- CompilationUnit.cs
- DbConnectionPoolCounters.cs
- IImplicitResourceProvider.cs
- RemoteAsymmetricSignatureFormatter.cs
- EdmType.cs
- input.cs
- SystemIPGlobalProperties.cs
- DoubleAnimationClockResource.cs
- MasterPageParser.cs
- KeysConverter.cs
- SqlProvider.cs
- PrintingPermissionAttribute.cs
- SqlDataSourceView.cs
- TreeSet.cs
- MsmqBindingElementBase.cs
- Profiler.cs
- EFColumnProvider.cs
- CodeConstructor.cs
- Not.cs
- VectorAnimation.cs
- AnonymousIdentificationSection.cs
- TemplatedAdorner.cs
- ChannelEndpointElement.cs
- ScaleTransform3D.cs
- FreezableOperations.cs
- TypeNameConverter.cs
- Utils.cs
- DataObject.cs
- KeyValuePairs.cs
- Qualifier.cs
- OutputCacheSection.cs
- ServiceModelActivity.cs
- _OverlappedAsyncResult.cs
- GrabHandleGlyph.cs
- Helper.cs
- AuthenticationModuleElementCollection.cs
- SqlUtil.cs
- SpellerError.cs
- SimpleExpression.cs
- KeyInterop.cs
- TextTrailingCharacterEllipsis.cs
- ObjectDisposedException.cs
- AssemblyAttributesGoHere.cs
- Win32MouseDevice.cs
- StringResourceManager.cs
- ExpressionVisitor.cs
- InputElement.cs
- EtwTrace.cs
- ELinqQueryState.cs
- DefinitionUpdate.cs
- BamlLocalizationDictionary.cs
- WebPartZone.cs
- SharedConnectionInfo.cs
- cryptoapiTransform.cs
- RenderDataDrawingContext.cs
- ExpressionsCollectionConverter.cs