Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / MS / Internal / Utilities.cs / 1305600 / Utilities.cs
/**************************************************************************\ Copyright Microsoft Corporation. All Rights Reserved. \**************************************************************************/ namespace MS.Internal { using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using MS.Win32; ////// General utility class for macro-type functions. /// internal static class Utilities { private static readonly Version _osVersion = Environment.OSVersion.Version; internal static bool IsOSVistaOrNewer { get { return _osVersion >= new Version(6, 0); } } internal static bool IsOSWindows7OrNewer { get { return _osVersion >= new Version(6, 1); } } internal static bool IsCompositionEnabled { ////// Critical -- calls into an unsafe native method. /// TreatAsSafe -- the call to DwmIsCompositionEnabled simply returns a boolean /// through an out parameter, it is safe information to expose /// [SecurityCritical, SecurityTreatAsSafe] get { if (!IsOSVistaOrNewer) { return false; } Int32 isDesktopCompositionEnabled = 0; UnsafeNativeMethods.HRESULT.Check(UnsafeNativeMethods.DwmIsCompositionEnabled(out isDesktopCompositionEnabled)); return isDesktopCompositionEnabled != 0; } } internal static void SafeDispose(ref T disposable) where T : IDisposable { // Dispose can safely be called on an object multiple times. IDisposable t = disposable; disposable = default(T); if (null != t) { t.Dispose(); } } /// /// Critical - Suppresses unmanaged code security. Calls Marshal.ReleaseComObject which has a LinkDemand. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] internal static void SafeRelease(ref T comObject) where T : class { T t = comObject; comObject = default(T); if (null != t) { Debug.Assert(Marshal.IsComObject(t)); Marshal.ReleaseComObject(t); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /**************************************************************************\ Copyright Microsoft Corporation. All Rights Reserved. \**************************************************************************/ namespace MS.Internal { using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security; using MS.Win32; /// /// General utility class for macro-type functions. /// internal static class Utilities { private static readonly Version _osVersion = Environment.OSVersion.Version; internal static bool IsOSVistaOrNewer { get { return _osVersion >= new Version(6, 0); } } internal static bool IsOSWindows7OrNewer { get { return _osVersion >= new Version(6, 1); } } internal static bool IsCompositionEnabled { ////// Critical -- calls into an unsafe native method. /// TreatAsSafe -- the call to DwmIsCompositionEnabled simply returns a boolean /// through an out parameter, it is safe information to expose /// [SecurityCritical, SecurityTreatAsSafe] get { if (!IsOSVistaOrNewer) { return false; } Int32 isDesktopCompositionEnabled = 0; UnsafeNativeMethods.HRESULT.Check(UnsafeNativeMethods.DwmIsCompositionEnabled(out isDesktopCompositionEnabled)); return isDesktopCompositionEnabled != 0; } } internal static void SafeDispose(ref T disposable) where T : IDisposable { // Dispose can safely be called on an object multiple times. IDisposable t = disposable; disposable = default(T); if (null != t) { t.Dispose(); } } /// /// Critical - Suppresses unmanaged code security. Calls Marshal.ReleaseComObject which has a LinkDemand. /// [SuppressUnmanagedCodeSecurity, SecurityCritical] internal static void SafeRelease(ref T comObject) where T : class { T t = comObject; comObject = default(T); if (null != t) { Debug.Assert(Marshal.IsComObject(t)); Marshal.ReleaseComObject(t); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
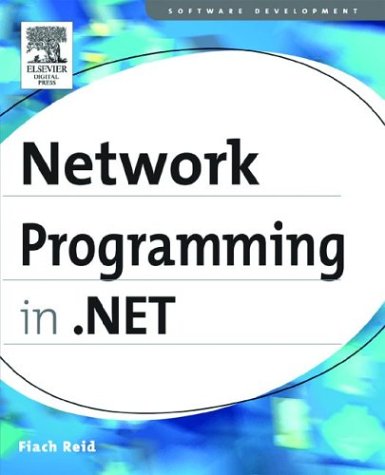
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolStripItem.cs
- XmlReader.cs
- NetMsmqSecurityElement.cs
- Section.cs
- SourceLocationProvider.cs
- URLString.cs
- SqlConnectionPoolProviderInfo.cs
- Accessible.cs
- WindowVisualStateTracker.cs
- CompilationLock.cs
- BuildProviderCollection.cs
- ReservationCollection.cs
- DataBindingCollectionConverter.cs
- ComPlusDiagnosticTraceSchemas.cs
- RelatedImageListAttribute.cs
- SqlErrorCollection.cs
- IList.cs
- DateTimeOffsetStorage.cs
- KnownColorTable.cs
- InputMethodStateChangeEventArgs.cs
- HttpWriter.cs
- SortAction.cs
- DataGridViewRowHeaderCell.cs
- GeneralTransform3D.cs
- cryptoapiTransform.cs
- DetailsViewInsertEventArgs.cs
- DefinitionUpdate.cs
- GeometryModel3D.cs
- DataGridViewColumnTypePicker.cs
- SkewTransform.cs
- DataObject.cs
- PropertyValueUIItem.cs
- OSFeature.cs
- EditingCommands.cs
- ThreadExceptionDialog.cs
- ListBox.cs
- ScrollBar.cs
- HttpWebRequestElement.cs
- WindowsFont.cs
- VectorConverter.cs
- sqlstateclientmanager.cs
- TraceSection.cs
- FontFaceLayoutInfo.cs
- UInt16Storage.cs
- ComboBox.cs
- ExtendedTransformFactory.cs
- DoubleAnimationUsingKeyFrames.cs
- ConfigXmlReader.cs
- CodeBlockBuilder.cs
- FaultPropagationRecord.cs
- CustomAttributeBuilder.cs
- MasterPageBuildProvider.cs
- HtmlGenericControl.cs
- PropertyConverter.cs
- DBCSCodePageEncoding.cs
- PassportAuthenticationEventArgs.cs
- TiffBitmapEncoder.cs
- DrawingBrush.cs
- ContextQuery.cs
- ObjectDataSource.cs
- UIElementHelper.cs
- CodeDomLoader.cs
- ComboBox.cs
- ChannelManager.cs
- InkCanvasAutomationPeer.cs
- Table.cs
- QilName.cs
- CacheSection.cs
- DiscoveryClientChannelFactory.cs
- XmlNodeList.cs
- UserControlCodeDomTreeGenerator.cs
- DataObject.cs
- SemaphoreFullException.cs
- ICollection.cs
- StylusSystemGestureEventArgs.cs
- SslStream.cs
- MarshalByRefObject.cs
- FamilyTypefaceCollection.cs
- SynchronizationContext.cs
- HasCopySemanticsAttribute.cs
- EncryptedData.cs
- JsonGlobals.cs
- RowVisual.cs
- SwitchElementsCollection.cs
- SqlCommand.cs
- ISAPIWorkerRequest.cs
- GenerateScriptTypeAttribute.cs
- XmlSerializationWriter.cs
- EasingFunctionBase.cs
- FormatterConverter.cs
- Table.cs
- MiniConstructorInfo.cs
- ManagementInstaller.cs
- HelpKeywordAttribute.cs
- TrustLevelCollection.cs
- PrintPageEvent.cs
- CustomLineCap.cs
- ClientUrlResolverWrapper.cs
- Positioning.cs
- FixedStringLookup.cs