Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / UIAutomation / UIAutomationClient / MS / Internal / Automation / WindowVisualStateTracker.cs / 1 / WindowVisualStateTracker.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class used to track the visual appearance of Windows and make sure any events // are propogated to that new UI. // // History: // 01/05/2005 : Miw Created // //--------------------------------------------------------------------------- using System; using System.Windows.Automation; using MS.Win32; namespace MS.Internal.Automation { // Class used to track new UI appearing and make sure any events // are propogated to that new UI. internal class WindowVisualStateTracker : WinEventWrap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal WindowVisualStateTracker() : base(new int[] { NativeMethods.EVENT_OBJECT_LOCATIONCHANGE }) { // Intentionally not setting the callback for the base WinEventWrap since the WinEventProc override // in this class calls RaiseEventInThisClientOnly to actually raise the event to the client. } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void WinEventProc(int eventId, IntPtr hwnd, int idObject, int idChild, uint eventTime) { // ignore any event not pertaining directly to the window if (idObject != UnsafeNativeMethods.OBJID_WINDOW) { return; } // Ignore if this is a bogus hwnd (shouldn't happen) if (hwnd == IntPtr.Zero) { return; } OnStateChange(hwnd, idObject, idChild); } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods private void OnStateChange(IntPtr hwnd, int idObject, int idChild) { NativeMethods.HWND nativeHwnd = NativeMethods.HWND.Cast(hwnd); // Ignore windows that have been destroyed if (!SafeNativeMethods.IsWindow(nativeHwnd)) { return; } AutomationElement rawEl = AutomationElement.FromHandle(hwnd); // Raise this event only for elements with the WindowPattern. object patternObject; if (!rawEl.TryGetCurrentPattern(WindowPattern.Pattern, out patternObject)) return; Object windowVisualState = rawEl.GetPatternPropertyValue(WindowPattern.WindowVisualStateProperty, false); // if has no state value just return if (!(windowVisualState is WindowVisualState)) { return; } WindowVisualState state = (WindowVisualState)windowVisualState; // Filter... avoid duplicate events if (hwnd == _lastHwnd && state == _lastState) { return; } AutomationPropertyChangedEventArgs e = new AutomationPropertyChangedEventArgs( WindowPattern.WindowVisualStateProperty, null, state); ClientEventManager.RaiseEventInThisClientOnly(AutomationElement.AutomationPropertyChangedEvent, rawEl, e); // save the last hwnd/rect for filtering out duplicates _lastHwnd = hwnd; _lastState = state; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private WindowVisualState _lastState; // keep track of last visual state private IntPtr _lastHwnd; // and hwnd for dup checking #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Class used to track the visual appearance of Windows and make sure any events // are propogated to that new UI. // // History: // 01/05/2005 : Miw Created // //--------------------------------------------------------------------------- using System; using System.Windows.Automation; using MS.Win32; namespace MS.Internal.Automation { // Class used to track new UI appearing and make sure any events // are propogated to that new UI. internal class WindowVisualStateTracker : WinEventWrap { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors internal WindowVisualStateTracker() : base(new int[] { NativeMethods.EVENT_OBJECT_LOCATIONCHANGE }) { // Intentionally not setting the callback for the base WinEventWrap since the WinEventProc override // in this class calls RaiseEventInThisClientOnly to actually raise the event to the client. } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods internal override void WinEventProc(int eventId, IntPtr hwnd, int idObject, int idChild, uint eventTime) { // ignore any event not pertaining directly to the window if (idObject != UnsafeNativeMethods.OBJID_WINDOW) { return; } // Ignore if this is a bogus hwnd (shouldn't happen) if (hwnd == IntPtr.Zero) { return; } OnStateChange(hwnd, idObject, idChild); } #endregion Internal Methods //------------------------------------------------------ // // Private Methods // //------------------------------------------------------ #region Private Methods private void OnStateChange(IntPtr hwnd, int idObject, int idChild) { NativeMethods.HWND nativeHwnd = NativeMethods.HWND.Cast(hwnd); // Ignore windows that have been destroyed if (!SafeNativeMethods.IsWindow(nativeHwnd)) { return; } AutomationElement rawEl = AutomationElement.FromHandle(hwnd); // Raise this event only for elements with the WindowPattern. object patternObject; if (!rawEl.TryGetCurrentPattern(WindowPattern.Pattern, out patternObject)) return; Object windowVisualState = rawEl.GetPatternPropertyValue(WindowPattern.WindowVisualStateProperty, false); // if has no state value just return if (!(windowVisualState is WindowVisualState)) { return; } WindowVisualState state = (WindowVisualState)windowVisualState; // Filter... avoid duplicate events if (hwnd == _lastHwnd && state == _lastState) { return; } AutomationPropertyChangedEventArgs e = new AutomationPropertyChangedEventArgs( WindowPattern.WindowVisualStateProperty, null, state); ClientEventManager.RaiseEventInThisClientOnly(AutomationElement.AutomationPropertyChangedEvent, rawEl, e); // save the last hwnd/rect for filtering out duplicates _lastHwnd = hwnd; _lastState = state; } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private WindowVisualState _lastState; // keep track of last visual state private IntPtr _lastHwnd; // and hwnd for dup checking #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
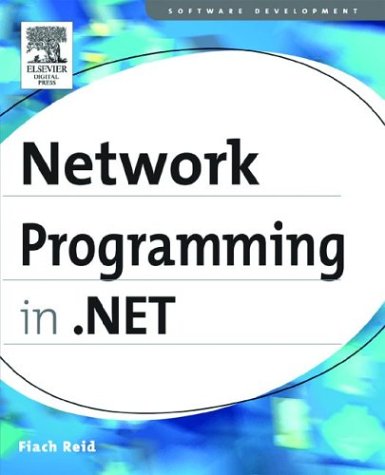
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ParserContext.cs
- FormViewUpdatedEventArgs.cs
- RootBrowserWindow.cs
- XslAst.cs
- InkPresenterAutomationPeer.cs
- ItemsControl.cs
- CompiledRegexRunner.cs
- MimeTypeAttribute.cs
- AppDomainProtocolHandler.cs
- HtmlTableRow.cs
- OrderedDictionary.cs
- ServiceObjectContainer.cs
- HtmlDocument.cs
- XamlTreeBuilderBamlRecordWriter.cs
- WebUtil.cs
- XsltLoader.cs
- AssemblyNameUtility.cs
- TimeStampChecker.cs
- ToolStripLabel.cs
- SqlDataRecord.cs
- CompilerErrorCollection.cs
- SqlConnectionString.cs
- TaiwanCalendar.cs
- AddingNewEventArgs.cs
- PersonalizationDictionary.cs
- IndexOutOfRangeException.cs
- AutomationElementCollection.cs
- TranslateTransform3D.cs
- COM2EnumConverter.cs
- WebServiceHandlerFactory.cs
- DetailsViewRow.cs
- XmlDocumentSerializer.cs
- ArgIterator.cs
- SemanticKeyElement.cs
- IncrementalCompileAnalyzer.cs
- DataGridViewDataErrorEventArgs.cs
- SessionParameter.cs
- DataControlField.cs
- PartialTrustHelpers.cs
- RuleSettings.cs
- xamlnodes.cs
- ReflectEventDescriptor.cs
- DbTransaction.cs
- StylusButtonEventArgs.cs
- WinInet.cs
- SQLConvert.cs
- CollectionBase.cs
- StringPropertyBuilder.cs
- TrustManagerPromptUI.cs
- ExceptionValidationRule.cs
- Axis.cs
- TextFormatterHost.cs
- DbConnectionFactory.cs
- ResourceCategoryAttribute.cs
- ProcessModuleCollection.cs
- MemberPathMap.cs
- TempFiles.cs
- PropertyEmitter.cs
- SegmentInfo.cs
- NoneExcludedImageIndexConverter.cs
- ProcessModelInfo.cs
- SvcMapFileSerializer.cs
- XmlSerializationWriter.cs
- GridViewSelectEventArgs.cs
- ConnectionPoint.cs
- WinInetCache.cs
- UnionCodeGroup.cs
- EmbossBitmapEffect.cs
- GridViewRowCollection.cs
- Control.cs
- MimeFormatter.cs
- VScrollProperties.cs
- MainMenu.cs
- StylusOverProperty.cs
- WindowsListViewScroll.cs
- HttpClientProtocol.cs
- RecognizerBase.cs
- OdbcPermission.cs
- HwndSource.cs
- IconBitmapDecoder.cs
- DataGridCaption.cs
- QualifierSet.cs
- ToolStripPanel.cs
- XmlSiteMapProvider.cs
- GridLength.cs
- EdmItemCollection.cs
- CodeChecksumPragma.cs
- NativeMethods.cs
- MediaTimeline.cs
- BasicHttpBindingElement.cs
- Point3DAnimationUsingKeyFrames.cs
- AuthorizationRuleCollection.cs
- ImageField.cs
- _UriTypeConverter.cs
- PropertyGridEditorPart.cs
- BypassElement.cs
- SystemGatewayIPAddressInformation.cs
- ClientEndpointLoader.cs
- StringAnimationUsingKeyFrames.cs
- RuntimeTransactionHandle.cs