Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / Compilation / WCFModel / ProxyGenerationError.cs / 1305376 / ProxyGenerationError.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // This code is shared between ndp\fx\src\xsp\System\Web\Extensions\Compilation\WCFModel // and wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // Please make sure the code files between those two directories are alway in [....] when you make any changes to this code. // And always test these code in both places before check in. // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Diagnostics; using System.Globalization; using System.IO; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Configuration; using System.ServiceModel.Description; using System.Xml; using System.Xml.Schema; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// This class represents an error message happens when we generate code /// ///#if WEB_EXTENSIONS_CODE internal class ProxyGenerationError #else [CLSCompliant(true)] public class ProxyGenerationError #endif { private bool m_IsWarning; private string m_Message; private string m_MetadataFile; private int m_LineNumber; private int m_LinePosition; private GeneratorState m_ErrorGeneratorState; /// /// Constructor /// /// MetadataConversionError ///public ProxyGenerationError(MetadataConversionError errorMessage) { m_ErrorGeneratorState = GeneratorState.GenerateCode; m_IsWarning = errorMessage.IsWarning; m_Message = errorMessage.Message; m_MetadataFile = String.Empty; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An IOException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, Exception errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An IOException /// An IOException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, Exception errorException, bool isWarning) { m_ErrorGeneratorState = generatorState; m_IsWarning = isWarning; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlException errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// Constructor /// /// /// /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlSchemaException errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// Constructor /// /// /// /// An XmlException /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlSchemaException errorException, bool isWarning) { m_ErrorGeneratorState = generatorState; m_IsWarning = isWarning; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// This property represents when an error message happens /// ////// public GeneratorState ErrorGeneratorState { get { return m_ErrorGeneratorState; } } /// /// True: if it is a warning message, otherwise, an error /// ////// public bool IsWarning { get { return m_IsWarning; } } /// /// Line Number when error happens /// ////// public int LineNumber { get { return m_LineNumber; } } /// /// Column Number in the line when error happens /// ////// public int LinePosition { get { return m_LinePosition; } } /// /// return the error message /// ////// public string Message { get { return m_Message; } } /// /// return the error message /// ////// public string MetadataFile { get { return m_MetadataFile; } } /// /// This enum represents when an error message happens /// ///public enum GeneratorState { LoadMetadata = 0, MergeMetadata = 1, GenerateCode = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (C) Microsoft Corporation. All Rights Reserved. // //----------------------------------------------------------------------------- // // This code is shared between ndp\fx\src\xsp\System\Web\Extensions\Compilation\WCFModel // and wizard\vsdesigner\designer\microsoft\vsdesigner\WCFModel. // Please make sure the code files between those two directories are alway in [....] when you make any changes to this code. // And always test these code in both places before check in. // The code under ndp\fx\src\xsp\System\Web\Extensions\Compilation\XmlSerializer might have to be regerenated when // the format of the svcmap file is changed, or class structure has been changed in this directory. Please follow the HowTo file // under Compilation directory to see how to regerenate that code. // using System; using System.Diagnostics; using System.Globalization; using System.IO; using System.ServiceModel; using System.ServiceModel.Channels; using System.ServiceModel.Configuration; using System.ServiceModel.Description; using System.Xml; using System.Xml.Schema; #if WEB_EXTENSIONS_CODE namespace System.Web.Compilation.WCFModel #else namespace Microsoft.VSDesigner.WCFModel #endif { ////// This class represents an error message happens when we generate code /// ///#if WEB_EXTENSIONS_CODE internal class ProxyGenerationError #else [CLSCompliant(true)] public class ProxyGenerationError #endif { private bool m_IsWarning; private string m_Message; private string m_MetadataFile; private int m_LineNumber; private int m_LinePosition; private GeneratorState m_ErrorGeneratorState; /// /// Constructor /// /// MetadataConversionError ///public ProxyGenerationError(MetadataConversionError errorMessage) { m_ErrorGeneratorState = GeneratorState.GenerateCode; m_IsWarning = errorMessage.IsWarning; m_Message = errorMessage.Message; m_MetadataFile = String.Empty; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An IOException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, Exception errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An IOException /// An IOException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, Exception errorException, bool isWarning) { m_ErrorGeneratorState = generatorState; m_IsWarning = isWarning; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = -1; m_LinePosition = -1; } /// /// Constructor /// /// /// /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlException errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// Constructor /// /// /// /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlSchemaException errorException) { m_ErrorGeneratorState = generatorState; m_IsWarning = false; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// Constructor /// /// /// /// An XmlException /// An XmlException ///public ProxyGenerationError(GeneratorState generatorState, string fileName, XmlSchemaException errorException, bool isWarning) { m_ErrorGeneratorState = generatorState; m_IsWarning = isWarning; m_Message = errorException.Message; m_MetadataFile = fileName; m_LineNumber = errorException.LineNumber; m_LinePosition = errorException.LinePosition; } /// /// This property represents when an error message happens /// ////// public GeneratorState ErrorGeneratorState { get { return m_ErrorGeneratorState; } } /// /// True: if it is a warning message, otherwise, an error /// ////// public bool IsWarning { get { return m_IsWarning; } } /// /// Line Number when error happens /// ////// public int LineNumber { get { return m_LineNumber; } } /// /// Column Number in the line when error happens /// ////// public int LinePosition { get { return m_LinePosition; } } /// /// return the error message /// ////// public string Message { get { return m_Message; } } /// /// return the error message /// ////// public string MetadataFile { get { return m_MetadataFile; } } /// /// This enum represents when an error message happens /// ///public enum GeneratorState { LoadMetadata = 0, MergeMetadata = 1, GenerateCode = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
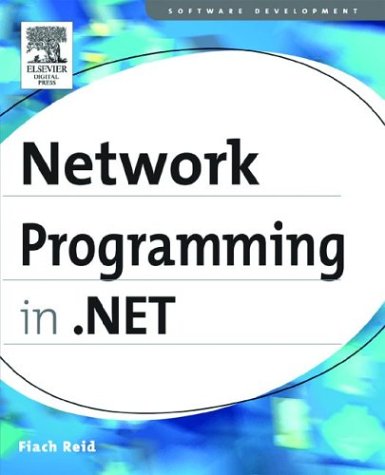
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GPStream.cs
- QilInvokeLateBound.cs
- SqlDataSourceConfigureSelectPanel.cs
- RootBrowserWindowProxy.cs
- Vector3D.cs
- EncoderBestFitFallback.cs
- InkPresenter.cs
- SqlDataSourceDesigner.cs
- Image.cs
- LoadMessageLogger.cs
- TdsValueSetter.cs
- ADMembershipUser.cs
- LambdaCompiler.cs
- SolidBrush.cs
- MenuRendererClassic.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- AdapterDictionary.cs
- ObjectItemCachedAssemblyLoader.cs
- Grant.cs
- XmlSchemaExternal.cs
- XamlReaderHelper.cs
- ImageFormatConverter.cs
- XmlEncoding.cs
- LineMetrics.cs
- ReadOnlyNameValueCollection.cs
- XmlQueryContext.cs
- Triangle.cs
- HebrewNumber.cs
- SelectionUIHandler.cs
- ReachDocumentPageSerializerAsync.cs
- Properties.cs
- DrawingGroupDrawingContext.cs
- OutgoingWebRequestContext.cs
- DataGridViewCellStyleConverter.cs
- PenLineJoinValidation.cs
- GridViewAutomationPeer.cs
- AdRotator.cs
- DataServiceEntityAttribute.cs
- XmlChildEnumerator.cs
- EventLogPermissionEntry.cs
- ExtensionQuery.cs
- CryptoProvider.cs
- XamlParser.cs
- CheckBoxFlatAdapter.cs
- GridViewColumnCollection.cs
- LeafCellTreeNode.cs
- GridEntryCollection.cs
- DataGridViewMethods.cs
- ClientScriptManagerWrapper.cs
- PointUtil.cs
- UriTemplateEquivalenceComparer.cs
- Decoder.cs
- SecurityDescriptor.cs
- EnumerableRowCollectionExtensions.cs
- ComplusTypeValidator.cs
- WebPageTraceListener.cs
- HwndHost.cs
- WindowsScroll.cs
- DesignerSerializerAttribute.cs
- NativeMethodsCLR.cs
- RemoteWebConfigurationHostServer.cs
- Choices.cs
- TextViewBase.cs
- SessionState.cs
- ApplicationDirectory.cs
- AccessViolationException.cs
- WebPartsPersonalizationAuthorization.cs
- QilXmlWriter.cs
- ScrollPattern.cs
- InheritanceUI.cs
- DataGridViewImageCell.cs
- HttpClientCertificate.cs
- FrameworkElement.cs
- DropTarget.cs
- PersonalizationStateInfo.cs
- CheckBoxList.cs
- NetTcpBindingElement.cs
- ChannelListenerBase.cs
- PageCatalogPart.cs
- _Rfc2616CacheValidators.cs
- ConfigurationPropertyAttribute.cs
- ProviderConnectionPoint.cs
- ColorConvertedBitmap.cs
- SessionStateModule.cs
- SystemKeyConverter.cs
- COM2IVsPerPropertyBrowsingHandler.cs
- WebDescriptionAttribute.cs
- Clipboard.cs
- ValueProviderWrapper.cs
- TileModeValidation.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- LinqDataSourceHelper.cs
- Catch.cs
- ToolBar.cs
- BackgroundFormatInfo.cs
- InkCanvasFeedbackAdorner.cs
- XmlElementAttribute.cs
- IndexedGlyphRun.cs
- QilTernary.cs
- PropertyTabChangedEvent.cs