Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / DynamicData / DynamicData / DefaultAutoFieldGenerator.cs / 1305376 / DefaultAutoFieldGenerator.cs
namespace System.Web.DynamicData { using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Web.DynamicData.Util; using System.Web.UI; using System.Web.UI.WebControls; public class DefaultAutoFieldGenerator : IAutoFieldGenerator { private IMetaTable _metaTable; public DefaultAutoFieldGenerator(MetaTable table) : this((IMetaTable)table) { } internal DefaultAutoFieldGenerator(IMetaTable table) { if (table == null) { throw new ArgumentNullException("table"); } _metaTable = table; } public ICollection GenerateFields(Control control) { DataBoundControlMode mode = GetMode(control); ContainerType containerType = GetControlContainerType(control); // Auto-generate fields from metadata. Listfields = new List (); foreach (MetaColumn column in _metaTable.GetScaffoldColumns(mode, containerType)) { fields.Add(CreateField(column, containerType, mode)); } return fields; } protected virtual DynamicField CreateField(MetaColumn column, ContainerType containerType, DataBoundControlMode mode) { string headerText = (containerType == ContainerType.List ? column.ShortDisplayName : column.DisplayName); var field = new DynamicField() { DataField = column.Name, HeaderText = headerText }; // Turn wrapping off by default so that error messages don't show up on the next line. field.ItemStyle.Wrap = false; return field; } internal static ContainerType GetControlContainerType(Control control) { #if !ORYX_VNEXT if (control is IDataBoundListControl || control is Repeater) { return ContainerType.List; } else if (control is IDataBoundItemControl) { return ContainerType.Item; } #else if (control is GridView || control is ListView || control is Repeater) { return ContainerType.List; } if (control is FormView || control is DetailsView) { return ContainerType.Item; } #endif return ContainerType.List; } internal static DataBoundControlMode GetMode(Control control) { #if ORYX_VNEXT return DataControlHelper.GetControlAdapter(control).Mode; #else // Only item controls have distinct modes IDataBoundItemControl itemControl = control as IDataBoundItemControl; if (itemControl != null && GetControlContainerType(control) != ContainerType.List) { return itemControl.Mode; } return DataBoundControlMode.ReadOnly; #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Web.DynamicData { using System.Collections; using System.Collections.Generic; using System.Diagnostics; using System.Web.DynamicData.Util; using System.Web.UI; using System.Web.UI.WebControls; public class DefaultAutoFieldGenerator : IAutoFieldGenerator { private IMetaTable _metaTable; public DefaultAutoFieldGenerator(MetaTable table) : this((IMetaTable)table) { } internal DefaultAutoFieldGenerator(IMetaTable table) { if (table == null) { throw new ArgumentNullException("table"); } _metaTable = table; } public ICollection GenerateFields(Control control) { DataBoundControlMode mode = GetMode(control); ContainerType containerType = GetControlContainerType(control); // Auto-generate fields from metadata. List fields = new List (); foreach (MetaColumn column in _metaTable.GetScaffoldColumns(mode, containerType)) { fields.Add(CreateField(column, containerType, mode)); } return fields; } protected virtual DynamicField CreateField(MetaColumn column, ContainerType containerType, DataBoundControlMode mode) { string headerText = (containerType == ContainerType.List ? column.ShortDisplayName : column.DisplayName); var field = new DynamicField() { DataField = column.Name, HeaderText = headerText }; // Turn wrapping off by default so that error messages don't show up on the next line. field.ItemStyle.Wrap = false; return field; } internal static ContainerType GetControlContainerType(Control control) { #if !ORYX_VNEXT if (control is IDataBoundListControl || control is Repeater) { return ContainerType.List; } else if (control is IDataBoundItemControl) { return ContainerType.Item; } #else if (control is GridView || control is ListView || control is Repeater) { return ContainerType.List; } if (control is FormView || control is DetailsView) { return ContainerType.Item; } #endif return ContainerType.List; } internal static DataBoundControlMode GetMode(Control control) { #if ORYX_VNEXT return DataControlHelper.GetControlAdapter(control).Mode; #else // Only item controls have distinct modes IDataBoundItemControl itemControl = control as IDataBoundItemControl; if (itemControl != null && GetControlContainerType(control) != ContainerType.List) { return itemControl.Mode; } return DataBoundControlMode.ReadOnly; #endif } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
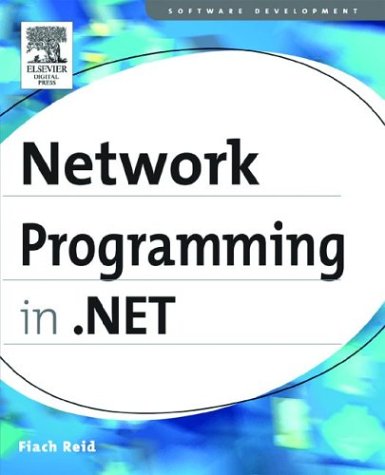
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RangeBase.cs
- IIS7UserPrincipal.cs
- AttributeSetAction.cs
- GenerateScriptTypeAttribute.cs
- ConnectionPoint.cs
- TextBox.cs
- PageThemeParser.cs
- OneOfScalarConst.cs
- ListViewItemMouseHoverEvent.cs
- MsmqChannelListenerBase.cs
- ListBoxDesigner.cs
- OleDbRowUpdatedEvent.cs
- SystemColors.cs
- SchemaConstraints.cs
- tooltip.cs
- PLINQETWProvider.cs
- SqlCacheDependencySection.cs
- HtmlContainerControl.cs
- URLMembershipCondition.cs
- MemberInfoSerializationHolder.cs
- OdbcConnectionStringbuilder.cs
- StatusBarPanelClickEvent.cs
- SecurityTokenProvider.cs
- DynamicEndpointElement.cs
- UInt16Storage.cs
- SqlVersion.cs
- AuthenticationSection.cs
- XmlSchemaElement.cs
- CommandConverter.cs
- SoapSchemaExporter.cs
- Encoding.cs
- InternalTransaction.cs
- HttpProfileGroupBase.cs
- Matrix3D.cs
- TrackingDataItemValue.cs
- XmlDataCollection.cs
- SourceChangedEventArgs.cs
- OracleFactory.cs
- Select.cs
- SqlProviderServices.cs
- OrderingInfo.cs
- OleDbConnection.cs
- ServiceDescriptionData.cs
- AsyncPostBackErrorEventArgs.cs
- DataViewSetting.cs
- LogSwitch.cs
- ReachDocumentPageSerializer.cs
- CanExecuteRoutedEventArgs.cs
- ExpressionLink.cs
- ScriptReferenceEventArgs.cs
- PageRouteHandler.cs
- UserControlCodeDomTreeGenerator.cs
- InputLangChangeEvent.cs
- DirectoryRedirect.cs
- ToolStripDropDownItem.cs
- PlainXmlDeserializer.cs
- IgnoreSectionHandler.cs
- SystemException.cs
- StreamReader.cs
- PathGeometry.cs
- Model3DCollection.cs
- BitmapEffect.cs
- EFTableProvider.cs
- ToolZone.cs
- DefaultValueAttribute.cs
- DocumentationServerProtocol.cs
- Imaging.cs
- StringUtil.cs
- IdentityValidationException.cs
- NavigationProperty.cs
- InputLanguageProfileNotifySink.cs
- HttpRuntime.cs
- FormViewPagerRow.cs
- StylusCaptureWithinProperty.cs
- DataRowCollection.cs
- ExternalFile.cs
- SHA1Managed.cs
- NotificationContext.cs
- recordstatefactory.cs
- SecurityMode.cs
- DesignerAutoFormat.cs
- PointLight.cs
- EntityDataSourceEntitySetNameItem.cs
- Constants.cs
- MobileCategoryAttribute.cs
- ServiceContractViewControl.cs
- ContentPropertyAttribute.cs
- ReflectionPermission.cs
- EntityDataSourceStatementEditor.cs
- MatrixTransform3D.cs
- AttributeExtensions.cs
- RenamedEventArgs.cs
- DoubleAnimationBase.cs
- ImageSource.cs
- ButtonField.cs
- BitmapScalingModeValidation.cs
- SafeFileMappingHandle.cs
- TrackingProfile.cs
- PermissionToken.cs
- SqlConnectionStringBuilder.cs