Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / TextBox.cs / 1305376 / TextBox.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing; using System.Web; using System.Web.UI; using System.Web.UI.Design.WebControls; using System.Web.UI.HtmlControls; using System.Security.Permissions; namespace System.Web.UI.MobileControls { /* * Mobile TextBox class. * * Copyright (c) 2000 Microsoft Corporation */ ///[ ControlBuilderAttribute(typeof(TextBoxControlBuilder)), DataBindingHandler("System.Web.UI.Design.TextDataBindingHandler, " + AssemblyRef.SystemDesign), DefaultEvent("TextChanged"), DefaultProperty("Text"), Designer(typeof(System.Web.UI.Design.MobileControls.TextBoxDesigner)), DesignerAdapter(typeof(System.Web.UI.Design.MobileControls.Adapters.DesignerTextBoxAdapter)), ToolboxData("<{0}:TextBox runat=\"server\">{0}:TextBox>"), ToolboxItem("System.Web.UI.Design.WebControlToolboxItem, " + AssemblyRef.SystemDesign), ValidationProperty("Text") ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class TextBox : TextControl, IPostBackDataHandler { private static readonly Object EventTextChanged = new Object(); /// /// protected bool LoadPostData(String key, NameValueCollection data) { bool dataChanged = false; bool handledByAdapter = Adapter.LoadPostData(key, data, null, out dataChanged); if (!handledByAdapter) { String value = data[key]; if (Text != value) { Text = value; dataChanged = true; // this will cause a RaisePostDataChangedEvent() } } return dataChanged; } /// /// protected void RaisePostDataChangedEvent() { OnTextChanged(EventArgs.Empty); } /// protected virtual void OnTextChanged(EventArgs e) { EventHandler onTextChangedHandler = (EventHandler)Events[EventTextChanged]; if (onTextChangedHandler != null) { onTextChangedHandler(this,e); } } /// [ Bindable(true), Browsable(true), DefaultValue(false), MobileCategory(SR.Category_Behavior), MobileSysDescription(SR.TextBox_Password) ] public bool Password { get { Object b = ViewState["Password"]; return (b != null) ? (bool)b : false; } set { ViewState["Password"] = value; } } /// [ Bindable(true), Browsable(true), DefaultValue(false), MobileCategory(SR.Category_Behavior), MobileSysDescription(SR.TextBox_Numeric) ] public bool Numeric { get { Object b = ViewState["Numeric"]; return (b != null) ? (bool)b : false; } set { ViewState["Numeric"] = value; } } /// [ Bindable(true), DefaultValue(0), MobileCategory(SR.Category_Behavior), MobileSysDescription(SR.TextBox_Size) ] public int Size { get { Object i = ViewState["Size"]; return((i != null) ? (int)i : 0); } set { if (value < 0) { throw new ArgumentOutOfRangeException("Size", value, SR.GetString(SR.TextBox_NotNegativeNumber)); } ViewState["Size"] = value; } } /// [ Bindable(true), DefaultValue(0), MobileCategory(SR.Category_Behavior), MobileSysDescription(SR.TextBox_MaxLength) ] public int MaxLength { get { Object i = ViewState["MaxLength"]; return((i != null) ? (int)i : 0); } set { if (value < 0) { throw new ArgumentOutOfRangeException("MaxLength", value, SR.GetString(SR.TextBox_NotNegativeNumber)); } ViewState["MaxLength"] = value; } } /// [ MobileSysDescription(SR.TextBox_OnTextChanged) ] public event EventHandler TextChanged { add { Events.AddHandler(EventTextChanged, value); } remove { Events.RemoveHandler(EventTextChanged, value); } } /// [ Bindable(true), DefaultValue(""), MobileCategory(SR.Category_Appearance), MobileSysDescription(SR.Input_Title) ] public String Title { get { return ToString(ViewState["Title"]); } set { ViewState["Title"] = value; } } internal override bool TrimInnerText { get { return false; } } internal override bool TrimNewlines { get { return true; } } #region IPostBackDataHandler implementation bool IPostBackDataHandler.LoadPostData(String key, NameValueCollection data) { return LoadPostData(key, data); } void IPostBackDataHandler.RaisePostDataChangedEvent() { RaisePostDataChangedEvent(); } #endregion } /* * TextBox Control Builder * * Copyright (c) 2000 Microsoft Corporation */ /// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class TextBoxControlBuilder : MobileControlBuilder { // Textbox allows whitespace inside text. /// public override bool AllowWhitespaceLiterals() { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
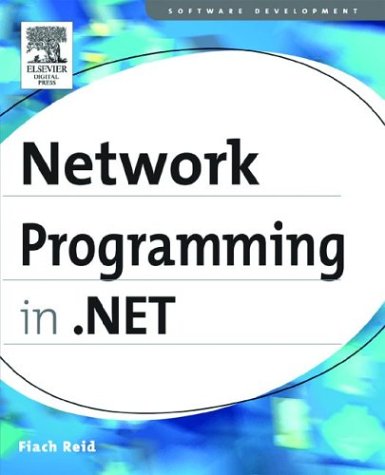
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ToolbarAUtomationPeer.cs
- FontSourceCollection.cs
- FixedPosition.cs
- ApplicationFileParser.cs
- SvcFileManager.cs
- RightsManagementInformation.cs
- DurableEnlistmentState.cs
- DBCommandBuilder.cs
- FeatureSupport.cs
- contentDescriptor.cs
- MSAAWinEventWrap.cs
- XhtmlBasicPageAdapter.cs
- ValidationErrorCollection.cs
- Vector3DCollection.cs
- SQLSingleStorage.cs
- DirectoryRootQuery.cs
- CrossAppDomainChannel.cs
- ApplicationSettingsBase.cs
- DBNull.cs
- DataGrid.cs
- InvalidCommandTreeException.cs
- ObjectCacheSettings.cs
- ContentIterators.cs
- ThreadNeutralSemaphore.cs
- PlainXmlWriter.cs
- AnnotationAuthorChangedEventArgs.cs
- RegexMatchCollection.cs
- EventLogLink.cs
- DataGridViewSelectedCellCollection.cs
- WorkflowWebService.cs
- HandleCollector.cs
- OrderByLifter.cs
- DBAsyncResult.cs
- BindingEntityInfo.cs
- PipeStream.cs
- SQLRoleProvider.cs
- DocumentSchemaValidator.cs
- GetImportFileNameRequest.cs
- XhtmlBasicCalendarAdapter.cs
- DuplexClientBase.cs
- LoginAutoFormat.cs
- XmlTextReaderImpl.cs
- PreviewPageInfo.cs
- TemplateBindingExpressionConverter.cs
- CatalogPartCollection.cs
- DbFunctionCommandTree.cs
- ClickablePoint.cs
- ToolStripItemEventArgs.cs
- WebControlParameterProxy.cs
- DurableEnlistmentState.cs
- DictionaryContent.cs
- DeadCharTextComposition.cs
- COM2PictureConverter.cs
- ZipIOExtraFieldZip64Element.cs
- AnnotationAdorner.cs
- MappingMetadataHelper.cs
- DefaultValidator.cs
- Drawing.cs
- HtmlTextBoxAdapter.cs
- unsafenativemethodstextservices.cs
- ReachDocumentReferenceCollectionSerializerAsync.cs
- InfoCardBaseException.cs
- NameNode.cs
- DynamicExpression.cs
- ApplicationInfo.cs
- JournalNavigationScope.cs
- activationcontext.cs
- AsymmetricAlgorithm.cs
- NotImplementedException.cs
- UnhandledExceptionEventArgs.cs
- HttpRuntimeSection.cs
- Collection.cs
- QueryStringHandler.cs
- RealizationDrawingContextWalker.cs
- GridView.cs
- ClientUtils.cs
- TypeRefElement.cs
- RemotingAttributes.cs
- DataKey.cs
- StylusButtonEventArgs.cs
- TagPrefixInfo.cs
- TabletDevice.cs
- ErrorEventArgs.cs
- SamlConditions.cs
- NativeStructs.cs
- CodeDirectiveCollection.cs
- StringSource.cs
- StatusBarDrawItemEvent.cs
- ExpressionEditorAttribute.cs
- AnnotationAuthorChangedEventArgs.cs
- JulianCalendar.cs
- ExecutionContext.cs
- AutomationProperties.cs
- SoapIgnoreAttribute.cs
- WpfWebRequestHelper.cs
- TextPointer.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- ContentPlaceHolder.cs
- EncoderExceptionFallback.cs
- TripleDESCryptoServiceProvider.cs