Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / ProviderBase / TimeoutTimer.cs / 1305376 / TimeoutTimer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Class used to manage timeouts in complex system operations. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.ProviderBase { using System; using System.Data.Common; using System.Diagnostics; // Purpose: // Manages determining and tracking timeouts // // Intended use: // Call StartXXXXTimeout() to get a timer with the given expiration point // Get remaining time in appropriate format to pass to subsystem timeouts // Check for timeout via IsExpired for checks in managed code. // Simply abandon to GC when done. internal class TimeoutTimer { //------------------- // Fields //------------------- private long _timerExpire; private bool _isInfiniteTimeout; //------------------- // Timeout-setting methods //------------------- // Get a new timer that will expire in the given number of seconds // For input, a value of zero seconds indicates infinite timeout internal static TimeoutTimer StartSecondsTimeout(int seconds) { //-------------------- // Preconditions: None (seconds must conform to SetTimeoutSeconds requirements) //-------------------- // Method body var timeout = new TimeoutTimer(); timeout.SetTimeoutSeconds(seconds); //--------------------- // Postconditions Debug.Assert(timeout != null); // Need a valid timeouttimer if no error return timeout; } #if !ORACLE // Get a new timer that will expire in the given number of milliseconds // No current need to support infinite milliseconds timeout internal static TimeoutTimer StartMillisecondsTimeout(long milliseconds) { //-------------------- // Preconditions Debug.Assert(0 <= milliseconds); //-------------------- // Method body var timeout = new TimeoutTimer(); timeout._timerExpire = checked(ADP.TimerCurrent() + (milliseconds * TimeSpan.TicksPerMillisecond)); timeout._isInfiniteTimeout = false; //--------------------- // Postconditions Debug.Assert(timeout != null); // Need a valid timeouttimer if no error return timeout; } #endif //------------------- // Methods for changing timeout //------------------- internal void SetTimeoutSeconds(int seconds) { //-------------------- // Preconditions Debug.Assert(0 <= seconds || InfiniteTimeout == seconds); // no need to support negative seconds at present //-------------------- // Method body if (InfiniteTimeout == seconds) { _isInfiniteTimeout = true; } else { // Stash current time + timeout _timerExpire = checked(ADP.TimerCurrent() + ADP.TimerFromSeconds(seconds)); _isInfiniteTimeout = false; } //--------------------- // Postconditions:None } //------------------- // Timeout info properties //------------------- // Indicator for infinite timeout when starting a timer internal static readonly long InfiniteTimeout = 0; // Is this timer in an expired state? internal bool IsExpired { get { return !IsInfinite && ADP.TimerHasExpired(_timerExpire); } } // is this an infinite-timeout timer? internal bool IsInfinite { get { return _isInfiniteTimeout; } } // Special accessor for TimerExpire for use when thunking to legacy timeout methods. internal long LegacyTimerExpire { get { return (_isInfiniteTimeout) ? Int64.MaxValue : _timerExpire; } } // Returns milliseconds remaining trimmed to zero for none remaining // and long.MaxValue for infinite // This method should be prefered for internal calculations that are not // yet common enough to code into the TimeoutTimer class itself. internal long MillisecondsRemaining { get { //------------------- // Preconditions: None //------------------- // Method Body long milliseconds; if (_isInfiniteTimeout) { milliseconds = long.MaxValue; } else { milliseconds = ADP.TimerRemainingMilliseconds(_timerExpire); if (0 > milliseconds) { milliseconds = 0; } } //-------------------- // Postconditions Debug.Assert(0<=milliseconds); // This property guarantees no negative return values return milliseconds; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // Class used to manage timeouts in complex system operations. // //[....] //[....] //----------------------------------------------------------------------------- namespace System.Data.ProviderBase { using System; using System.Data.Common; using System.Diagnostics; // Purpose: // Manages determining and tracking timeouts // // Intended use: // Call StartXXXXTimeout() to get a timer with the given expiration point // Get remaining time in appropriate format to pass to subsystem timeouts // Check for timeout via IsExpired for checks in managed code. // Simply abandon to GC when done. internal class TimeoutTimer { //------------------- // Fields //------------------- private long _timerExpire; private bool _isInfiniteTimeout; //------------------- // Timeout-setting methods //------------------- // Get a new timer that will expire in the given number of seconds // For input, a value of zero seconds indicates infinite timeout internal static TimeoutTimer StartSecondsTimeout(int seconds) { //-------------------- // Preconditions: None (seconds must conform to SetTimeoutSeconds requirements) //-------------------- // Method body var timeout = new TimeoutTimer(); timeout.SetTimeoutSeconds(seconds); //--------------------- // Postconditions Debug.Assert(timeout != null); // Need a valid timeouttimer if no error return timeout; } #if !ORACLE // Get a new timer that will expire in the given number of milliseconds // No current need to support infinite milliseconds timeout internal static TimeoutTimer StartMillisecondsTimeout(long milliseconds) { //-------------------- // Preconditions Debug.Assert(0 <= milliseconds); //-------------------- // Method body var timeout = new TimeoutTimer(); timeout._timerExpire = checked(ADP.TimerCurrent() + (milliseconds * TimeSpan.TicksPerMillisecond)); timeout._isInfiniteTimeout = false; //--------------------- // Postconditions Debug.Assert(timeout != null); // Need a valid timeouttimer if no error return timeout; } #endif //------------------- // Methods for changing timeout //------------------- internal void SetTimeoutSeconds(int seconds) { //-------------------- // Preconditions Debug.Assert(0 <= seconds || InfiniteTimeout == seconds); // no need to support negative seconds at present //-------------------- // Method body if (InfiniteTimeout == seconds) { _isInfiniteTimeout = true; } else { // Stash current time + timeout _timerExpire = checked(ADP.TimerCurrent() + ADP.TimerFromSeconds(seconds)); _isInfiniteTimeout = false; } //--------------------- // Postconditions:None } //------------------- // Timeout info properties //------------------- // Indicator for infinite timeout when starting a timer internal static readonly long InfiniteTimeout = 0; // Is this timer in an expired state? internal bool IsExpired { get { return !IsInfinite && ADP.TimerHasExpired(_timerExpire); } } // is this an infinite-timeout timer? internal bool IsInfinite { get { return _isInfiniteTimeout; } } // Special accessor for TimerExpire for use when thunking to legacy timeout methods. internal long LegacyTimerExpire { get { return (_isInfiniteTimeout) ? Int64.MaxValue : _timerExpire; } } // Returns milliseconds remaining trimmed to zero for none remaining // and long.MaxValue for infinite // This method should be prefered for internal calculations that are not // yet common enough to code into the TimeoutTimer class itself. internal long MillisecondsRemaining { get { //------------------- // Preconditions: None //------------------- // Method Body long milliseconds; if (_isInfiniteTimeout) { milliseconds = long.MaxValue; } else { milliseconds = ADP.TimerRemainingMilliseconds(_timerExpire); if (0 > milliseconds) { milliseconds = 0; } } //-------------------- // Postconditions Debug.Assert(0<=milliseconds); // This property guarantees no negative return values return milliseconds; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
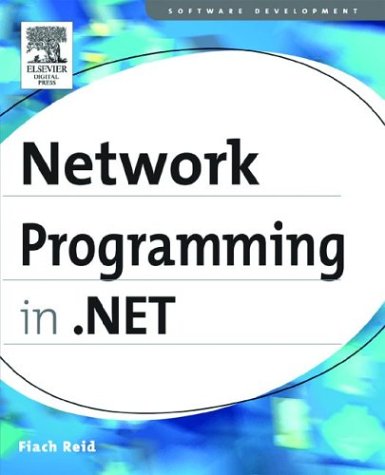
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Property.cs
- ActivationServices.cs
- ImmComposition.cs
- ScriptIgnoreAttribute.cs
- VirtualPathUtility.cs
- SingleAnimationBase.cs
- TextWriterEngine.cs
- ChannelManager.cs
- CustomTypeDescriptor.cs
- IPipelineRuntime.cs
- InputBinding.cs
- ProtocolInformationWriter.cs
- ExpressionNode.cs
- ControlTemplate.cs
- AdornerDecorator.cs
- DbExpressionVisitor_TResultType.cs
- XmlSortKeyAccumulator.cs
- DiffuseMaterial.cs
- SqlUserDefinedTypeAttribute.cs
- Scheduler.cs
- TraceUtility.cs
- GCHandleCookieTable.cs
- URL.cs
- MethodBody.cs
- XPathExpr.cs
- DeleteHelper.cs
- CqlQuery.cs
- MergablePropertyAttribute.cs
- TreeViewImageIndexConverter.cs
- EraserBehavior.cs
- DataGridRelationshipRow.cs
- X509CertificateClaimSet.cs
- RemoteWebConfigurationHostServer.cs
- ScriptRegistrationManager.cs
- PointAnimationUsingKeyFrames.cs
- ZipIOCentralDirectoryBlock.cs
- SpecularMaterial.cs
- BoundPropertyEntry.cs
- FormsAuthenticationTicket.cs
- AsyncOperation.cs
- ListDictionary.cs
- List.cs
- ListBoxItemWrapperAutomationPeer.cs
- SiteMapDataSourceView.cs
- DataObjectMethodAttribute.cs
- DefaultSection.cs
- WorkflowTransactionOptions.cs
- WebConvert.cs
- EncryptedPackage.cs
- MostlySingletonList.cs
- EnumerableCollectionView.cs
- GridPatternIdentifiers.cs
- RangeValuePattern.cs
- Trigger.cs
- MbpInfo.cs
- StateBag.cs
- NonSerializedAttribute.cs
- SafeNativeMethods.cs
- AspProxy.cs
- WebRequestModulesSection.cs
- Camera.cs
- ObjectStateEntryDbDataRecord.cs
- ScrollItemPatternIdentifiers.cs
- VisualBasicSettingsHandler.cs
- DataSourceHelper.cs
- LineUtil.cs
- FactoryId.cs
- Interlocked.cs
- UIElement.cs
- MimeXmlReflector.cs
- CroppedBitmap.cs
- AddingNewEventArgs.cs
- TrustVersion.cs
- XamlTemplateSerializer.cs
- CharacterMetricsDictionary.cs
- PageThemeBuildProvider.cs
- ConnectorMovedEventArgs.cs
- Exceptions.cs
- MobileListItemCollection.cs
- ActivationServices.cs
- HandlerMappingMemo.cs
- TraceSwitch.cs
- GACIdentityPermission.cs
- NumericUpDownAcceleration.cs
- SettingsPropertyIsReadOnlyException.cs
- FixedSchema.cs
- DetailsViewRow.cs
- ControlAdapter.cs
- OdbcEnvironmentHandle.cs
- ToolStripItemClickedEventArgs.cs
- DbCommandDefinition.cs
- UriSection.cs
- Partitioner.cs
- RotateTransform.cs
- ClientSettingsSection.cs
- XmlSchemaDatatype.cs
- DataGridViewCellConverter.cs
- ClientRuntimeConfig.cs
- DateTimeFormatInfoScanner.cs
- EventLogTraceListener.cs