Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / DEVDIV / depot / DevDiv / releases / whidbey / QFE / ndp / fx / src / xsp / System / Web / UI / ControlAdapter.cs / 2 / ControlAdapter.cs
//How to set the _control //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Adapters { using System; using System.ComponentModel; using System.Security.Permissions; /* Defines the properties, methods, and events shared by all server control * adapters in the Web Forms page framework. */ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class ControlAdapter { private HttpBrowserCapabilities _browser = null; internal Control _control; //control associated with this adapter [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] protected Control Control { get { return _control; } } /* Indicates the page on which the associated control resides. */ [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] protected Page Page { get { if(Control != null) return Control.Page; return null; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] protected PageAdapter PageAdapter { get { if(Control != null && Control.Page != null) return Control.Page.PageAdapter; return null; } } protected HttpBrowserCapabilities Browser { get { if (_browser == null) { if (Page.RequestInternal != null) { _browser = Page.RequestInternal.Browser; } else { /* */ HttpContext context = HttpContext.Current; if (context != null && context.Request != null) { _browser = context.Request.Browser; } } } return _browser; } } protected internal virtual void OnInit(EventArgs e) { Control.OnInit(e); } protected internal virtual void OnLoad(EventArgs e) { Control.OnLoad(e); } protected internal virtual void OnPreRender(EventArgs e) { Control.OnPreRender(e); } protected internal virtual void Render(HtmlTextWriter writer) { // if(_control != null) { _control.Render(writer); } } protected virtual void RenderChildren(HtmlTextWriter writer) { if(_control != null) { _control.RenderChildren(writer); } } protected internal virtual void OnUnload(EventArgs e) { Control.OnUnload(e); } protected internal virtual void BeginRender(HtmlTextWriter writer) { writer.BeginRender(); } protected internal virtual void CreateChildControls() { Control.CreateChildControls(); } protected internal virtual void EndRender(HtmlTextWriter writer) { writer.EndRender(); } protected internal virtual void LoadAdapterControlState(object state) { } protected internal virtual void LoadAdapterViewState(object state) { } protected internal virtual object SaveAdapterControlState() { return null; } protected internal virtual object SaveAdapterViewState() { return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //How to set the _control //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Adapters { using System; using System.ComponentModel; using System.Security.Permissions; /* Defines the properties, methods, and events shared by all server control * adapters in the Web Forms page framework. */ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class ControlAdapter { private HttpBrowserCapabilities _browser = null; internal Control _control; //control associated with this adapter [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] protected Control Control { get { return _control; } } /* Indicates the page on which the associated control resides. */ [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] protected Page Page { get { if(Control != null) return Control.Page; return null; } } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), ] protected PageAdapter PageAdapter { get { if(Control != null && Control.Page != null) return Control.Page.PageAdapter; return null; } } protected HttpBrowserCapabilities Browser { get { if (_browser == null) { if (Page.RequestInternal != null) { _browser = Page.RequestInternal.Browser; } else { /* */ HttpContext context = HttpContext.Current; if (context != null && context.Request != null) { _browser = context.Request.Browser; } } } return _browser; } } protected internal virtual void OnInit(EventArgs e) { Control.OnInit(e); } protected internal virtual void OnLoad(EventArgs e) { Control.OnLoad(e); } protected internal virtual void OnPreRender(EventArgs e) { Control.OnPreRender(e); } protected internal virtual void Render(HtmlTextWriter writer) { // if(_control != null) { _control.Render(writer); } } protected virtual void RenderChildren(HtmlTextWriter writer) { if(_control != null) { _control.RenderChildren(writer); } } protected internal virtual void OnUnload(EventArgs e) { Control.OnUnload(e); } protected internal virtual void BeginRender(HtmlTextWriter writer) { writer.BeginRender(); } protected internal virtual void CreateChildControls() { Control.CreateChildControls(); } protected internal virtual void EndRender(HtmlTextWriter writer) { writer.EndRender(); } protected internal virtual void LoadAdapterControlState(object state) { } protected internal virtual void LoadAdapterViewState(object state) { } protected internal virtual object SaveAdapterControlState() { return null; } protected internal virtual object SaveAdapterViewState() { return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
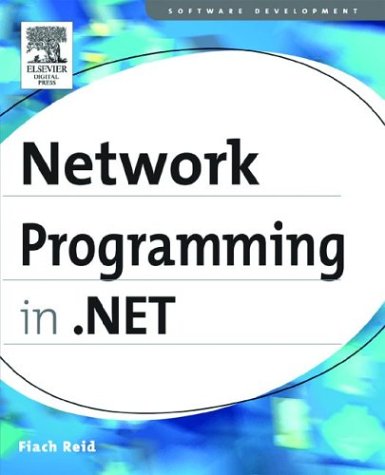
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartConnectionsConnectVerb.cs
- ProfileSettings.cs
- WebConfigurationHostFileChange.cs
- MulticastOption.cs
- figurelength.cs
- ProcessModule.cs
- FunctionDescription.cs
- WebResourceAttribute.cs
- PasswordTextNavigator.cs
- GridViewSortEventArgs.cs
- TypeElement.cs
- precedingsibling.cs
- ReliableSession.cs
- DesignSurfaceEvent.cs
- AuthenticationService.cs
- CodeSnippetExpression.cs
- PathBox.cs
- ManagementInstaller.cs
- DateTimeFormatInfo.cs
- UserPreferenceChangedEventArgs.cs
- DecoderReplacementFallback.cs
- BuildManagerHost.cs
- Application.cs
- RefreshEventArgs.cs
- Point3DKeyFrameCollection.cs
- PageAsyncTaskManager.cs
- InterleavedZipPartStream.cs
- WebPartsPersonalizationAuthorization.cs
- SrgsDocument.cs
- ClientUrlResolverWrapper.cs
- Size3DConverter.cs
- SecondaryViewProvider.cs
- Timeline.cs
- TailPinnedEventArgs.cs
- WebDescriptionAttribute.cs
- ObjectConverter.cs
- ConfigurationManagerHelperFactory.cs
- SqlProvider.cs
- MulticastNotSupportedException.cs
- SizeValueSerializer.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- DecimalSumAggregationOperator.cs
- RSAOAEPKeyExchangeDeformatter.cs
- EncryptedData.cs
- XmlWriter.cs
- OutputCacheSettings.cs
- sqlser.cs
- ObjectListFieldCollection.cs
- EventLog.cs
- TemplateModeChangedEventArgs.cs
- CompositeFontParser.cs
- FixedSOMSemanticBox.cs
- VariantWrapper.cs
- BCLDebug.cs
- LayoutDump.cs
- COM2PropertyDescriptor.cs
- ArgumentOutOfRangeException.cs
- HandlerWithFactory.cs
- CodeTypeMemberCollection.cs
- MaterialGroup.cs
- DataTable.cs
- HuffModule.cs
- InstanceCreationEditor.cs
- WSSecurityTokenSerializer.cs
- WebPartConnectionsCancelEventArgs.cs
- PropertyConverter.cs
- WindowsFormsHelpers.cs
- ClientViaElement.cs
- BindableAttribute.cs
- PresentationUIStyleResources.cs
- PtsPage.cs
- Transform.cs
- ResourceDescriptionAttribute.cs
- TreeChangeInfo.cs
- UnsafeNativeMethods.cs
- TagMapCollection.cs
- XmlSchemaSimpleContentRestriction.cs
- InstanceContextManager.cs
- DataRelation.cs
- HitTestWithGeometryDrawingContextWalker.cs
- TimelineCollection.cs
- EventQueueState.cs
- SqlParameterCollection.cs
- FontDialog.cs
- BoundColumn.cs
- HttpPostedFile.cs
- BuilderPropertyEntry.cs
- TextLine.cs
- WebPartCatalogAddVerb.cs
- FlowDocumentPaginator.cs
- Fonts.cs
- SafeMemoryMappedViewHandle.cs
- TransactionContextManager.cs
- Quaternion.cs
- SwitchCase.cs
- WindowsStreamSecurityElement.cs
- XmlAttributeProperties.cs
- PerspectiveCamera.cs
- Oid.cs
- RegexWorker.cs