Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / XamlBuildTask / Microsoft / Build / Tasks / Xaml / ClassData.cs / 1305376 / ClassData.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace Microsoft.Build.Tasks.Xaml { using System; using System.Collections.Generic; using System.Xml.Linq; using System.Xaml.Schema; using System.Xaml; using System.Windows.Markup; using System.Runtime; using System.Reflection; class ClassData { [Fx.Tag.Queue(typeof(NamedObject), Scope = Fx.Tag.Strings.DeclaringInstance)] private IListnamedObjects; [Fx.Tag.Queue(typeof(string), Scope = Fx.Tag.Strings.DeclaringInstance)] private IList codeSnippets; [Fx.Tag.Queue(typeof(XamlType), Scope = Fx.Tag.Strings.DeclaringInstance)] private IList typeArguments; public IList CodeSnippets { get { if (codeSnippets == null) { codeSnippets = new List (); } return codeSnippets; } } public string MarkupFileName { get; set; } public IDictionary Members { get; set; } public IList Attributes { get; set; } public string Name { get; set; } public IList NamedObjects { get { if (namedObjects == null) { namedObjects = new List (); } return namedObjects; } } public String Namespace { get; set; } public String RootNamespace { get; set; } public XamlType RootTypeName { get; set; } public IList TypeArguments { get { if (this.typeArguments == null) { this.typeArguments = new List (); } return this.typeArguments; } set { this.typeArguments = value; } } public XamlNodeList StrippedXaml { get; set; } public string Visibility { get; set; } public bool SourceFileExists { get; set; } public string FileName { get; set; } public bool RequiresCompilationPass2 { get; set; } public string HelperClassFullName { get; set; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace Microsoft.Build.Tasks.Xaml { using System; using System.Collections.Generic; using System.Xml.Linq; using System.Xaml.Schema; using System.Xaml; using System.Windows.Markup; using System.Runtime; using System.Reflection; class ClassData { [Fx.Tag.Queue(typeof(NamedObject), Scope = Fx.Tag.Strings.DeclaringInstance)] private IList namedObjects; [Fx.Tag.Queue(typeof(string), Scope = Fx.Tag.Strings.DeclaringInstance)] private IList codeSnippets; [Fx.Tag.Queue(typeof(XamlType), Scope = Fx.Tag.Strings.DeclaringInstance)] private IList typeArguments; public IList CodeSnippets { get { if (codeSnippets == null) { codeSnippets = new List (); } return codeSnippets; } } public string MarkupFileName { get; set; } public IDictionary Members { get; set; } public IList Attributes { get; set; } public string Name { get; set; } public IList NamedObjects { get { if (namedObjects == null) { namedObjects = new List (); } return namedObjects; } } public String Namespace { get; set; } public String RootNamespace { get; set; } public XamlType RootTypeName { get; set; } public IList TypeArguments { get { if (this.typeArguments == null) { this.typeArguments = new List (); } return this.typeArguments; } set { this.typeArguments = value; } } public XamlNodeList StrippedXaml { get; set; } public string Visibility { get; set; } public bool SourceFileExists { get; set; } public string FileName { get; set; } public bool RequiresCompilationPass2 { get; set; } public string HelperClassFullName { get; set; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
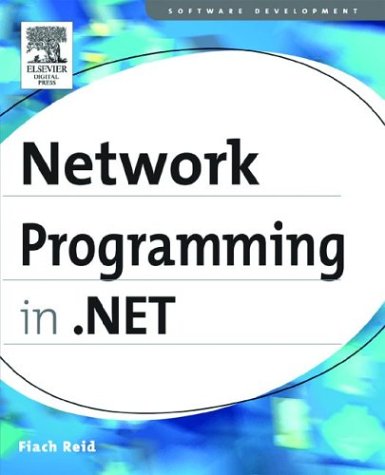
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UIElementCollection.cs
- CaretElement.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- PkcsUtils.cs
- XMLSyntaxException.cs
- SqlPersistenceWorkflowInstanceDescription.cs
- DesignerDataRelationship.cs
- IntMinMaxAggregationOperator.cs
- RefreshInfo.cs
- ConstructorBuilder.cs
- LinqDataSourceSelectEventArgs.cs
- XmlSerializationReader.cs
- TreeNodeStyleCollection.cs
- SoapParser.cs
- TraceSwitch.cs
- RequestUriProcessor.cs
- DataRecordInternal.cs
- FixedSOMFixedBlock.cs
- OleDbError.cs
- TextElementEnumerator.cs
- TextPattern.cs
- VectorCollection.cs
- SelectQueryOperator.cs
- ResourcesGenerator.cs
- AutoGeneratedField.cs
- BamlRecordReader.cs
- SecUtil.cs
- DefaultProxySection.cs
- MultiDataTrigger.cs
- MultiTargetingUtil.cs
- QueryRewriter.cs
- DataKey.cs
- RenderDataDrawingContext.cs
- RadioButtonRenderer.cs
- TextSpan.cs
- ContentElement.cs
- XmlTextReader.cs
- TemplateField.cs
- NavigationFailedEventArgs.cs
- MatrixCamera.cs
- DataRowChangeEvent.cs
- IssuedTokenClientElement.cs
- DirectoryObjectSecurity.cs
- InputScopeAttribute.cs
- SegmentInfo.cs
- ButtonChrome.cs
- ZipIOLocalFileDataDescriptor.cs
- RowToFieldTransformer.cs
- DebuggerAttributes.cs
- SecurityException.cs
- NetworkInterface.cs
- UpdateProgress.cs
- SpecialFolderEnumConverter.cs
- RewritingPass.cs
- DictionaryContent.cs
- VariableBinder.cs
- SqlParameterizer.cs
- connectionpool.cs
- ReferenceConverter.cs
- DbTypeMap.cs
- ValueCollectionParameterReader.cs
- HtmlTable.cs
- DataServiceQueryProvider.cs
- CodeDelegateCreateExpression.cs
- OleAutBinder.cs
- translator.cs
- DataGridLinkButton.cs
- CapabilitiesRule.cs
- Quad.cs
- TextClipboardData.cs
- CommandArguments.cs
- PassportIdentity.cs
- ImageField.cs
- LayoutManager.cs
- MediaTimeline.cs
- RayMeshGeometry3DHitTestResult.cs
- PlacementWorkspace.cs
- XLinq.cs
- RelationshipEntry.cs
- PageCache.cs
- RuntimeResourceSet.cs
- StartFileNameEditor.cs
- OpacityConverter.cs
- QilTargetType.cs
- UdpDiscoveryMessageFilter.cs
- RuleInfoComparer.cs
- WindowsClientElement.cs
- Camera.cs
- TrackingProfileManager.cs
- ImportedNamespaceContextItem.cs
- DeploymentExceptionMapper.cs
- BaseHashHelper.cs
- BindingContext.cs
- RoutedEventValueSerializer.cs
- MethodBuilder.cs
- OdbcException.cs
- XmlSchemaExporter.cs
- RijndaelManaged.cs
- XPathMultyIterator.cs
- SimpleApplicationHost.cs