Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ALinq / DataServiceQueryProvider.cs / 3 / DataServiceQueryProvider.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Query Provider for Linq to URI translatation // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; ////// QueryProvider implementation /// internal sealed class DataServiceQueryProvider : IQueryProvider { ///DataServiceContext for query provider internal readonly DataServiceContext Context; ///Constructs a query provider based on the context passed in /// The context for the query provider internal DataServiceQueryProvider(DataServiceContext context) { this.Context = context; } #region IQueryProvider implementation ///Factory method for creating DataServiceOrderedQuery based on expression /// The expression for the new query ///new DataServiceQuery public IQueryable CreateQuery(Expression expression) { Util.CheckArgumentNull(expression, "expression"); Type et = TypeSystem.GetElementType(expression.Type); Type qt = typeof(DataServiceQuery<>.DataServiceOrderedQuery).MakeGenericType(et); object[] args = new object[] { expression, this }; ConstructorInfo ci = qt.GetConstructor( BindingFlags.NonPublic | BindingFlags.Instance, null, new Type[] { typeof(Expression), typeof(DataServiceQueryProvider) }, null); return (IQueryable)ci.Invoke(args); } ///Factory method for creating DataServiceOrderedQuery based on expression ///generic type /// The expression for the new query ///new DataServiceQuery public IQueryableCreateQuery (Expression expression) { Util.CheckArgumentNull(expression, "expression"); return new DataServiceQuery .DataServiceOrderedQuery(expression, this); } /// Creates and executes a DataServiceQuery based on the passed in expression /// The expression for the new query ///the results public object Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); MethodInfo mi = typeof(DataServiceQueryProvider).GetMethod("ReturnSingleton", BindingFlags.NonPublic | BindingFlags.Instance); return mi.MakeGenericMethod(expression.Type).Invoke(this, new object[] { expression }); } ///Creates and executes a DataServiceQuery based on the passed in expression ///generic type /// The expression for the new query ///the results public TResult Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); return ReturnSingleton (expression); } #endregion /// Creates and executes a DataServiceQuery based on the passed in expression which results a single value ///generic type /// The expression for the new query ///single valued results internal TElement ReturnSingleton(Expression expression) { IQueryable query = new DataServiceQuery .DataServiceOrderedQuery(expression, this); MethodCallExpression mce = expression as MethodCallExpression; Debug.Assert(mce != null, "mce != null"); SequenceMethod sequenceMethod; if (ReflectionUtil.TryIdentifySequenceMethod(mce.Method, out sequenceMethod)) { switch (sequenceMethod) { case SequenceMethod.Single: return query.AsEnumerable().Single(); case SequenceMethod.SingleOrDefault: return query.AsEnumerable().SingleOrDefault(); case SequenceMethod.First: return query.AsEnumerable().First(); case SequenceMethod.FirstOrDefault: return query.AsEnumerable().FirstOrDefault(); default: throw Error.MethodNotSupported(mce); } } // Should never get here - should be caught by expression compiler. Debug.Assert(false, "Not supported singleton operator not caught by Resource Binder"); throw Error.MethodNotSupported(mce); } /// Builds the Uri for the expression passed in. /// The expression to translate into a Uri ///the resulting Uri internal Uri Translate(Expression e) { // short cut analysis if just a resource set if (!(e is ResourceSetExpression)) { e = Evaluator.PartialEval(e); e = new ExpressionNormalizer().Visit(e); e = ResourceBinder.Bind(e); } string translation = UriWriter.Translate(this.Context, e); return Util.CreateUri(this.Context.BaseUriWithSlash, Util.CreateUri(translation, UriKind.Relative)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Query Provider for Linq to URI translatation // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; using System.Reflection; ////// QueryProvider implementation /// internal sealed class DataServiceQueryProvider : IQueryProvider { ///DataServiceContext for query provider internal readonly DataServiceContext Context; ///Constructs a query provider based on the context passed in /// The context for the query provider internal DataServiceQueryProvider(DataServiceContext context) { this.Context = context; } #region IQueryProvider implementation ///Factory method for creating DataServiceOrderedQuery based on expression /// The expression for the new query ///new DataServiceQuery public IQueryable CreateQuery(Expression expression) { Util.CheckArgumentNull(expression, "expression"); Type et = TypeSystem.GetElementType(expression.Type); Type qt = typeof(DataServiceQuery<>.DataServiceOrderedQuery).MakeGenericType(et); object[] args = new object[] { expression, this }; ConstructorInfo ci = qt.GetConstructor( BindingFlags.NonPublic | BindingFlags.Instance, null, new Type[] { typeof(Expression), typeof(DataServiceQueryProvider) }, null); return (IQueryable)ci.Invoke(args); } ///Factory method for creating DataServiceOrderedQuery based on expression ///generic type /// The expression for the new query ///new DataServiceQuery public IQueryableCreateQuery (Expression expression) { Util.CheckArgumentNull(expression, "expression"); return new DataServiceQuery .DataServiceOrderedQuery(expression, this); } /// Creates and executes a DataServiceQuery based on the passed in expression /// The expression for the new query ///the results public object Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); MethodInfo mi = typeof(DataServiceQueryProvider).GetMethod("ReturnSingleton", BindingFlags.NonPublic | BindingFlags.Instance); return mi.MakeGenericMethod(expression.Type).Invoke(this, new object[] { expression }); } ///Creates and executes a DataServiceQuery based on the passed in expression ///generic type /// The expression for the new query ///the results public TResult Execute(Expression expression) { Util.CheckArgumentNull(expression, "expression"); return ReturnSingleton (expression); } #endregion /// Creates and executes a DataServiceQuery based on the passed in expression which results a single value ///generic type /// The expression for the new query ///single valued results internal TElement ReturnSingleton(Expression expression) { IQueryable query = new DataServiceQuery .DataServiceOrderedQuery(expression, this); MethodCallExpression mce = expression as MethodCallExpression; Debug.Assert(mce != null, "mce != null"); SequenceMethod sequenceMethod; if (ReflectionUtil.TryIdentifySequenceMethod(mce.Method, out sequenceMethod)) { switch (sequenceMethod) { case SequenceMethod.Single: return query.AsEnumerable().Single(); case SequenceMethod.SingleOrDefault: return query.AsEnumerable().SingleOrDefault(); case SequenceMethod.First: return query.AsEnumerable().First(); case SequenceMethod.FirstOrDefault: return query.AsEnumerable().FirstOrDefault(); default: throw Error.MethodNotSupported(mce); } } // Should never get here - should be caught by expression compiler. Debug.Assert(false, "Not supported singleton operator not caught by Resource Binder"); throw Error.MethodNotSupported(mce); } /// Builds the Uri for the expression passed in. /// The expression to translate into a Uri ///the resulting Uri internal Uri Translate(Expression e) { // short cut analysis if just a resource set if (!(e is ResourceSetExpression)) { e = Evaluator.PartialEval(e); e = new ExpressionNormalizer().Visit(e); e = ResourceBinder.Bind(e); } string translation = UriWriter.Translate(this.Context, e); return Util.CreateUri(this.Context.BaseUriWithSlash, Util.CreateUri(translation, UriKind.Relative)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
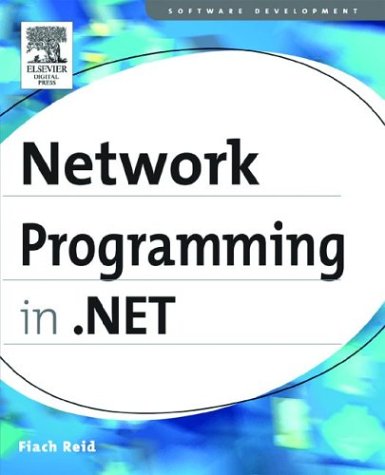
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferedGraphicsContext.cs
- BitmapDecoder.cs
- Stack.cs
- Int16Converter.cs
- Schema.cs
- ClientConfigurationHost.cs
- Pair.cs
- Annotation.cs
- Vector3DKeyFrameCollection.cs
- WebPartCatalogCloseVerb.cs
- NavigationProgressEventArgs.cs
- BStrWrapper.cs
- OuterGlowBitmapEffect.cs
- PropertyMappingExceptionEventArgs.cs
- PageHandlerFactory.cs
- SoapIgnoreAttribute.cs
- CorrelationTokenInvalidatedHandler.cs
- EntityDataSourceWrapperPropertyDescriptor.cs
- DbConnectionStringBuilder.cs
- DWriteFactory.cs
- EnvelopedSignatureTransform.cs
- ColumnResizeAdorner.cs
- BindingsCollection.cs
- ReservationNotFoundException.cs
- ImageKeyConverter.cs
- FrameSecurityDescriptor.cs
- SignatureTargetIdManager.cs
- Point.cs
- HtmlControlPersistable.cs
- DashStyles.cs
- OdbcConnectionHandle.cs
- EmptyEnumerator.cs
- InputReport.cs
- XmlSubtreeReader.cs
- XmlNodeChangedEventManager.cs
- CompositeTypefaceMetrics.cs
- CompoundFileReference.cs
- CancelAsyncOperationRequest.cs
- SoapAttributeAttribute.cs
- MouseBinding.cs
- ItemsControlAutomationPeer.cs
- SectionRecord.cs
- KnownTypeHelper.cs
- EntityContainerEmitter.cs
- DocumentPageView.cs
- WebPartChrome.cs
- ConstructorArgumentAttribute.cs
- MethodCallTranslator.cs
- DecimalConverter.cs
- HttpRawResponse.cs
- SQLGuidStorage.cs
- RawUIStateInputReport.cs
- MethodMessage.cs
- PopupRoot.cs
- Material.cs
- TextElement.cs
- FunctionDetailsReader.cs
- ColorContext.cs
- XmlAttributeOverrides.cs
- Tile.cs
- DirectoryRootQuery.cs
- WebPartAddingEventArgs.cs
- ValueOfAction.cs
- altserialization.cs
- Context.cs
- DataPager.cs
- ConnectionManager.cs
- SerializationTrace.cs
- TemplatePagerField.cs
- SafeLibraryHandle.cs
- Validator.cs
- LogReserveAndAppendState.cs
- ToolBar.cs
- GradientStop.cs
- XmlAttributeProperties.cs
- ConnectionManagementElementCollection.cs
- HtmlTableRowCollection.cs
- NoPersistProperty.cs
- DotNetATv1WindowsLogEntryDeserializer.cs
- StrokeNodeEnumerator.cs
- SemanticKeyElement.cs
- ProxyWebPartManager.cs
- TemplateColumn.cs
- ColorConvertedBitmap.cs
- WebPartCollection.cs
- Invariant.cs
- TextEditorSelection.cs
- ConnectionInterfaceCollection.cs
- EventLogEntryCollection.cs
- RequestCacheEntry.cs
- VirtualDirectoryMapping.cs
- ResourceIDHelper.cs
- SystemColors.cs
- BinHexDecoder.cs
- Application.cs
- DataGridTablesFactory.cs
- VariableBinder.cs
- PropertyCondition.cs
- Sentence.cs
- HtmlMeta.cs