Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Tools / xws_reg / System / ServiceModel / Install / Configuration / TransportationConfigurationTypeInstallComponent.cs / 1 / TransportationConfigurationTypeInstallComponent.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Install.Configuration { using System; using System.ServiceModel; using System.Configuration; using System.Globalization; using System.Text; using System.Web.Configuration; using System.Reflection; using System.Collections.Generic; using System.ServiceModel.Configuration; internal class TransportConfigurationTypeInstallComponent : ServiceModelInstallComponent { ConfigurationLoader configLoader; string displayString; string name; string transportConfigurationType; TransportConfigurationTypeInstallComponent(string name, ConfigurationLoader configLoader) { this.name = name; string mapped = null; switch (name) { case ServiceModelInstallStrings.NetTcp: mapped = ServiceModelInstallStrings.Tcp; break; case ServiceModelInstallStrings.NetPipe: mapped = ServiceModelInstallStrings.NamedPipe; break; case ServiceModelInstallStrings.NetMsmq: mapped = ServiceModelInstallStrings.Msmq; break; case ServiceModelInstallStrings.MsmqFormatName: mapped = ServiceModelInstallStrings.MsmqIntegration; break; default: throw new InvalidOperationException(SR.GetString(SR.InvalidTransportConfigurationType, name)); } this.transportConfigurationType = ServiceModelInstallStrings.TransportConfigurationType.Replace(ServiceModelInstallStrings.ProtocolToken, mapped); this.configLoader = configLoader; } internal override string DisplayName { get {return this.displayString; } } protected override string InstallActionMessage { get {return SR.GetString(SR.TransportConfigurationInstall, this.name, this.configLoader.RootWebConfigurationFilePath); } } internal override string[] InstalledVersions { get { string[] installedVersions = new string[0]; if (null != configLoader.ServiceHostingEnvironmentSection) { if (configLoader.ServiceHostingEnvironmentSection.TransportConfigurationTypes.ContainsKey(name)) { TransportConfigurationTypeElement element = configLoader.ServiceHostingEnvironmentSection.TransportConfigurationTypes[name]; installedVersions = new string[] {SR.GetString(SR.TransportConfigurationInstalledVersion, element.TransportConfigurationType)}; } } return installedVersions; } } internal override bool IsInstalled { get { return configLoader.ServiceHostingEnvironmentSection != null && configLoader.ServiceHostingEnvironmentSection.TransportConfigurationTypes.ContainsKey(name); } } protected override string ReinstallActionMessage { get {return SR.GetString(SR.TransportConfigurationReinstall, this.name, this.configLoader.RootWebConfigurationFilePath); } } protected override string UninstallActionMessage { get {return SR.GetString(SR.TransportConfigurationUninstall, this.name, this.configLoader.RootWebConfigurationFilePath); } } internal static TransportConfigurationTypeInstallComponent CreateNativeTransportConfigurationTypeInstallComponent(string name) { TransportConfigurationTypeInstallComponent transportConfigurationTypes = new TransportConfigurationTypeInstallComponent(name, new NativeConfigurationLoader()); transportConfigurationTypes.displayString = SR.GetString(SR.TransportConfigurationName, name); return transportConfigurationTypes; } internal static TransportConfigurationTypeInstallComponent CreateWow64TransportConfigurationTypeInstallComponent(string name) { if (!InstallHelper.Is64BitMachine() || String.IsNullOrEmpty(InstallHelper.Wow64WebConfigFileName)) { throw new ConfigurationLoaderException(SR.GetString(SR.Wow64NotInstalled)); } TransportConfigurationTypeInstallComponent transportConfigurationTypes = new TransportConfigurationTypeInstallComponent(name, new Wow64ConfigurationLoader()); transportConfigurationTypes.displayString = SR.GetString(SR.TransportConfigurationNameWow64, name); return transportConfigurationTypes; } internal override void Install(OutputLevel outputLevel) { if (!IisHelper.ShouldInstallWas) { throw new WasNotInstalledException(SR.GetString(SR.WasNotInstalled, SR.GetString(SR.TransportConfigurationName, name))); } if (!this.IsInstalled) { if (null != configLoader.ServiceHostingEnvironmentSection) { TransportConfigurationTypeElement element = new TransportConfigurationTypeElement(name); element.TransportConfigurationType = transportConfigurationType; configLoader.ServiceHostingEnvironmentSection.TransportConfigurationTypes.Add(element); configLoader.Save(); } else { throw new InvalidOperationException(SR.GetString(SR.ConfigurationSectionNotInstalled, configLoader.ServiceHostingEnvironmentSectionPath, configLoader.RootWebConfigurationFilePath)); } } else { EventLogger.LogWarning(SR.GetString(SR.TransportConfigurationAlreadyExists, name, configLoader.RootWebConfigurationFilePath), OutputLevel.Verbose == outputLevel); } } internal override void Uninstall(OutputLevel outputLevel) { if (this.IsInstalled) { configLoader.ServiceHostingEnvironmentSection.TransportConfigurationTypes.RemoveAt(name); configLoader.Save(); } else { EventLogger.LogWarning(SR.GetString(SR.TransportConfigurationNotInstalled, name, configLoader.RootWebConfigurationFilePath), OutputLevel.Verbose == outputLevel); } } internal override InstallationState VerifyInstall() { InstallationState installState = InstallationState.Unknown; if (this.IsInstalled) { if (null != configLoader.ServiceHostingEnvironmentSection) { if (configLoader.ServiceHostingEnvironmentSection.TransportConfigurationTypes.ContainsKey(name)) { TransportConfigurationTypeElement element = configLoader.ServiceHostingEnvironmentSection.TransportConfigurationTypes[name]; if (element.TransportConfigurationType.Equals(transportConfigurationType, StringComparison.OrdinalIgnoreCase)) { installState = InstallationState.InstalledDefaults; } else { installState = InstallationState.InstalledCustom; } } } } else { installState = InstallationState.NotInstalled; } return installState; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
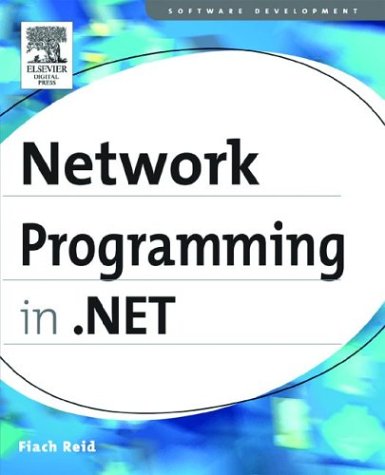
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProtocolsConfigurationEntry.cs
- SafeFindHandle.cs
- DateTimeValueSerializerContext.cs
- WorkflowDesigner.cs
- HttpCachePolicyElement.cs
- PhysicalOps.cs
- Point4D.cs
- BoolExpr.cs
- EnlistmentState.cs
- IntegerValidatorAttribute.cs
- CodeGeneratorOptions.cs
- Int16AnimationBase.cs
- SiteMapNodeItemEventArgs.cs
- _ScatterGatherBuffers.cs
- Point4DConverter.cs
- ParseElement.cs
- EntityTemplateUserControl.cs
- AQNBuilder.cs
- ControlTemplate.cs
- SEHException.cs
- ChannelProtectionRequirements.cs
- FamilyCollection.cs
- WebPartPersonalization.cs
- AuthenticationService.cs
- BindingCompleteEventArgs.cs
- EventSinkHelperWriter.cs
- BasePattern.cs
- DataGridTable.cs
- FamilyCollection.cs
- TriggerAction.cs
- Compiler.cs
- RangeValuePatternIdentifiers.cs
- Task.cs
- PrinterSettings.cs
- KeyValueConfigurationCollection.cs
- DiscoveryReference.cs
- WorkflowViewManager.cs
- DeferredElementTreeState.cs
- ClockGroup.cs
- Model3D.cs
- ToolStripSplitButton.cs
- UnauthorizedWebPart.cs
- TrackingAnnotationCollection.cs
- WebRequest.cs
- RestClientProxyHandler.cs
- TextPenaltyModule.cs
- XmlJsonWriter.cs
- CompositionAdorner.cs
- SerializationStore.cs
- CompiledQueryCacheEntry.cs
- BitStack.cs
- GeneralTransform.cs
- SQLDecimalStorage.cs
- DiagnosticsElement.cs
- XmlSerializationWriter.cs
- ChangeProcessor.cs
- EventSinkActivity.cs
- QueryUtil.cs
- ConfigPathUtility.cs
- InputChannelBinder.cs
- KeyValueInternalCollection.cs
- PasswordTextContainer.cs
- PenThread.cs
- XmlLoader.cs
- SvcMapFileLoader.cs
- WsdlInspector.cs
- ObjectKeyFrameCollection.cs
- DoubleAnimationUsingKeyFrames.cs
- VerificationException.cs
- PointLight.cs
- OleDbRowUpdatingEvent.cs
- SystemUnicastIPAddressInformation.cs
- DataTableCollection.cs
- ListenerConstants.cs
- UnlockInstanceCommand.cs
- ThrowHelper.cs
- NetworkStream.cs
- FirewallWrapper.cs
- HtmlContainerControl.cs
- SynchronousChannelMergeEnumerator.cs
- UnsignedPublishLicense.cs
- SoapInteropTypes.cs
- RunInstallerAttribute.cs
- ScrollBar.cs
- TypeDependencyAttribute.cs
- ObjectDataSourceStatusEventArgs.cs
- dataobject.cs
- EventSource.cs
- _KerberosClient.cs
- XmlWellformedWriter.cs
- CodeNamespaceImport.cs
- DynamicResourceExtensionConverter.cs
- EventListener.cs
- ObjectComplexPropertyMapping.cs
- CompilerHelpers.cs
- GeneralTransform3DGroup.cs
- SqlRemoveConstantOrderBy.cs
- SpinLock.cs
- PackWebRequestFactory.cs
- Journaling.cs