Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Configuration / ConfigUtil.cs / 4 / ConfigUtil.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Threading; using System.Configuration; using System.Xml; using System.Web.Compilation; using System.Web.Util; internal class ConfigUtil { private ConfigUtil() { } internal static void CheckBaseType(Type expectedBaseType, Type userBaseType, string propertyName, ConfigurationElement configElement) { // Make sure the base type is valid if (!expectedBaseType.IsAssignableFrom(userBaseType)) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_type_to_inherit_from, userBaseType.FullName, expectedBaseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, XmlNode node, bool checkAptcaBit, bool ignoreCase) { // We should get either a propertyName/configElement or node, but not both. // They are used only for error reporting. Debug.Assert((propertyName != null) != (node != null)); Type val; try { val = BuildManager.GetType(typeName, true /*throwOnError*/, ignoreCase); } catch (Exception e) { if (e is ThreadAbortException || e is StackOverflowException || e is OutOfMemoryException) { throw; } if (node != null) { throw new ConfigurationErrorsException(e.Message, e, node); } else { if (configElement != null) { throw new ConfigurationErrorsException(e.Message, e, configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } else { throw new ConfigurationErrorsException(e.Message, e); } } } // If we're not in full trust, only allow types that have the APTCA bit (ASURT 139687), // unless the checkAptcaBit flag is false if (checkAptcaBit) { if (node != null) { HttpRuntime.FailIfNoAPTCABit(val, node); } else { HttpRuntime.FailIfNoAPTCABit(val, configElement != null ? configElement.ElementInformation : null, propertyName); } } return val; } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement) { return GetType(typeName, propertyName, configElement, true /*checkAptcaBit*/); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit) { return GetType(typeName, propertyName, configElement, checkAptcaBit, false); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit, bool ignoreCase) { return GetType(typeName, propertyName, configElement, null /*node*/, checkAptcaBit, ignoreCase); } internal static Type GetType(string typeName, XmlNode node) { return GetType(typeName, node, false /*ignoreCase*/); } internal static Type GetType(string typeName, XmlNode node, bool ignoreCase) { return GetType(typeName, null, null, node, true /*checkAptcaBit*/, ignoreCase); } internal static void CheckAssignableType(Type baseType, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static void CheckAssignableType(Type baseType, Type baseType2, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type) && !baseType2.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static bool IsTypeHandlerOrFactory(Type t) { return typeof(IHttpHandler).IsAssignableFrom(t) || typeof(IHttpHandlerFactory).IsAssignableFrom(t); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Threading; using System.Configuration; using System.Xml; using System.Web.Compilation; using System.Web.Util; internal class ConfigUtil { private ConfigUtil() { } internal static void CheckBaseType(Type expectedBaseType, Type userBaseType, string propertyName, ConfigurationElement configElement) { // Make sure the base type is valid if (!expectedBaseType.IsAssignableFrom(userBaseType)) { throw new ConfigurationErrorsException( SR.GetString(SR.Invalid_type_to_inherit_from, userBaseType.FullName, expectedBaseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, XmlNode node, bool checkAptcaBit, bool ignoreCase) { // We should get either a propertyName/configElement or node, but not both. // They are used only for error reporting. Debug.Assert((propertyName != null) != (node != null)); Type val; try { val = BuildManager.GetType(typeName, true /*throwOnError*/, ignoreCase); } catch (Exception e) { if (e is ThreadAbortException || e is StackOverflowException || e is OutOfMemoryException) { throw; } if (node != null) { throw new ConfigurationErrorsException(e.Message, e, node); } else { if (configElement != null) { throw new ConfigurationErrorsException(e.Message, e, configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } else { throw new ConfigurationErrorsException(e.Message, e); } } } // If we're not in full trust, only allow types that have the APTCA bit (ASURT 139687), // unless the checkAptcaBit flag is false if (checkAptcaBit) { if (node != null) { HttpRuntime.FailIfNoAPTCABit(val, node); } else { HttpRuntime.FailIfNoAPTCABit(val, configElement != null ? configElement.ElementInformation : null, propertyName); } } return val; } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement) { return GetType(typeName, propertyName, configElement, true /*checkAptcaBit*/); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit) { return GetType(typeName, propertyName, configElement, checkAptcaBit, false); } internal static Type GetType(string typeName, string propertyName, ConfigurationElement configElement, bool checkAptcaBit, bool ignoreCase) { return GetType(typeName, propertyName, configElement, null /*node*/, checkAptcaBit, ignoreCase); } internal static Type GetType(string typeName, XmlNode node) { return GetType(typeName, node, false /*ignoreCase*/); } internal static Type GetType(string typeName, XmlNode node, bool ignoreCase) { return GetType(typeName, null, null, node, true /*checkAptcaBit*/, ignoreCase); } internal static void CheckAssignableType(Type baseType, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static void CheckAssignableType(Type baseType, Type baseType2, Type type, ConfigurationElement configElement, string propertyName) { if (!baseType.IsAssignableFrom(type) && !baseType2.IsAssignableFrom(type)) { throw new ConfigurationErrorsException( SR.GetString(SR.Type_doesnt_inherit_from_type, type.FullName, baseType.FullName), configElement.ElementInformation.Properties[propertyName].Source, configElement.ElementInformation.Properties[propertyName].LineNumber); } } internal static bool IsTypeHandlerOrFactory(Type t) { return typeof(IHttpHandler).IsAssignableFrom(t) || typeof(IHttpHandlerFactory).IsAssignableFrom(t); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
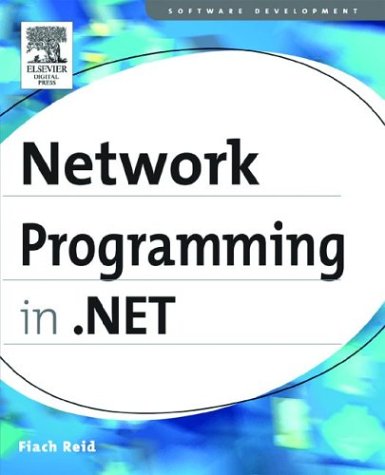
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageThemeParser.cs
- OdbcStatementHandle.cs
- SqlConnectionPoolGroupProviderInfo.cs
- AppDomainUnloadedException.cs
- CodeSubDirectory.cs
- ConstrainedDataObject.cs
- PerspectiveCamera.cs
- PropertyTabAttribute.cs
- WebMessageEncodingBindingElement.cs
- SchemaImporter.cs
- RegexCapture.cs
- RootProfilePropertySettingsCollection.cs
- TypeForwardedToAttribute.cs
- Translator.cs
- FieldMetadata.cs
- ConfigXmlCDataSection.cs
- AvtEvent.cs
- brushes.cs
- OpenFileDialog.cs
- WindowsEditBoxRange.cs
- DocumentSignatureManager.cs
- ValidationHelpers.cs
- ResizeGrip.cs
- SystemUdpStatistics.cs
- HealthMonitoringSection.cs
- CharAnimationBase.cs
- DataGridViewRowHeaderCell.cs
- FilterQuery.cs
- DataGridViewButtonColumn.cs
- MetaColumn.cs
- CrossSiteScriptingValidation.cs
- XmlSchemaNotation.cs
- TransportListener.cs
- DeploymentSectionCache.cs
- COM2ComponentEditor.cs
- LinqDataView.cs
- Html32TextWriter.cs
- SecureEnvironment.cs
- HttpProxyCredentialType.cs
- parserscommon.cs
- MapPathBasedVirtualPathProvider.cs
- ImageListDesigner.cs
- ObjectDataSourceStatusEventArgs.cs
- EnvironmentPermission.cs
- MouseGestureValueSerializer.cs
- FirstMatchCodeGroup.cs
- AdornerDecorator.cs
- DelayLoadType.cs
- PackageDigitalSignature.cs
- StickyNoteHelper.cs
- TextBox.cs
- MultipleCopiesCollection.cs
- DeflateStream.cs
- OperatingSystem.cs
- precedingquery.cs
- DropDownList.cs
- filewebrequest.cs
- RadioButton.cs
- XmlArrayAttribute.cs
- SmiTypedGetterSetter.cs
- InfoCardRSAPKCS1KeyExchangeDeformatter.cs
- WrappedReader.cs
- Message.cs
- ResourceReferenceExpressionConverter.cs
- ProvidePropertyAttribute.cs
- SessionPageStatePersister.cs
- ControlLocalizer.cs
- SrgsItemList.cs
- MultiView.cs
- AutomationPeer.cs
- HttpPostedFile.cs
- DayRenderEvent.cs
- StorageMappingItemLoader.cs
- VisualStyleElement.cs
- StatusBarItem.cs
- IriParsingElement.cs
- ExtensionDataObject.cs
- RadioButton.cs
- X500Name.cs
- Opcode.cs
- SqlDelegatedTransaction.cs
- XmlReflectionImporter.cs
- BaseValidator.cs
- XmlSerializerNamespaces.cs
- GraphicsPathIterator.cs
- TextParentUndoUnit.cs
- ProcessThreadCollection.cs
- CalendarButtonAutomationPeer.cs
- RequestDescription.cs
- XmlName.cs
- URLAttribute.cs
- StylusPointPropertyId.cs
- AutomationElementIdentifiers.cs
- PublisherMembershipCondition.cs
- Setter.cs
- ContentIterators.cs
- HttpCapabilitiesSectionHandler.cs
- ScriptComponentDescriptor.cs
- MarkerProperties.cs
- TransportReplyChannelAcceptor.cs