Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / TrackBarRenderer.cs / 1 / TrackBarRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TrackBarRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; const int lineWidth = 2; //cannot instantiate private TrackBarRenderer() { } ////// This is a rendering class for the TrackBar control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalTrack(Graphics g, Rectangle bounds) { InitializeRenderer(VisualStyleElement.TrackBar.Track.Normal, 1); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal track. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalTrack(Graphics g, Rectangle bounds) { InitializeRenderer(VisualStyleElement.TrackBar.TrackVertical.Normal, 1); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical track. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.Thumb.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawLeftPointingThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbLeft.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a constant size left pointing thumb centered in the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawRightPointingThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbRight.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a constant size right pointing thumb centered in the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawTopPointingThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbTop.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a constant size top pointing thumb centered in the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawBottomPointingThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbBottom.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a constant size bottom pointing thumb centered in the given bounds. /// ////// /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] // PM team has reviewed and decided on naming changes already [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalTicks(Graphics g, Rectangle bounds, int numTicks, EdgeStyle edgeStyle) { if (numTicks <= 0 || bounds.Height <= 0 || bounds.Width <= 0 || g == null) { return; } InitializeRenderer(VisualStyleElement.TrackBar.Ticks.Normal, 1); //trivial case -- avoid calcs if (numTicks == 1) { visualStyleRenderer.DrawEdge(g, new Rectangle(bounds.X, bounds.Y, lineWidth, bounds.Height), Edges.Left, edgeStyle, EdgeEffects.None); return; } float inc = ((float)bounds.Width - lineWidth) / ((float)numTicks - 1); while (numTicks > 0) { //draw the nth tick float x = bounds.X + ((float)(numTicks - 1)) * inc; visualStyleRenderer.DrawEdge(g, new Rectangle((int)Math.Round(x), bounds.Y, lineWidth, bounds.Height), Edges.Left, edgeStyle, EdgeEffects.None); numTicks--; } } ////// Renders a horizontal tick. /// ////// /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] // PM team has reviewed and decided on naming changes already [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalTicks(Graphics g, Rectangle bounds, int numTicks, EdgeStyle edgeStyle) { if (numTicks<=0 || bounds.Height <= 0 || bounds.Width<=0 || g == null ) { return; } InitializeRenderer(VisualStyleElement.TrackBar.TicksVertical.Normal, 1); //trivial case if (numTicks == 1) { visualStyleRenderer.DrawEdge(g, new Rectangle(bounds.X, bounds.Y, bounds.Width, lineWidth), Edges.Top, edgeStyle, EdgeEffects.None); return; } float inc = ((float)bounds.Height - lineWidth) / ((float)numTicks - 1); while (numTicks > 0) { //draw the nth tick float y = bounds.Y + ((float)(numTicks - 1)) * inc; visualStyleRenderer.DrawEdge(g, new Rectangle(bounds.X, (int)Math.Round(y), bounds.Width, lineWidth), Edges.Top, edgeStyle, EdgeEffects.None); numTicks--; } } ////// Renders a vertical tick. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetLeftPointingThumbSize(Graphics g, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbLeft.Normal, (int)state); return (visualStyleRenderer.GetPartSize(g, ThemeSizeType.True)); } ////// Returns the size of a left pointing thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetRightPointingThumbSize(Graphics g, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbRight.Normal, (int)state); return (visualStyleRenderer.GetPartSize(g, ThemeSizeType.True)); } ////// Returns the size of a right pointing thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetTopPointingThumbSize(Graphics g, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbTop.Normal, (int)state); return (visualStyleRenderer.GetPartSize(g, ThemeSizeType.True)); } ////// Returns the size of a top pointing thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetBottomPointingThumbSize(Graphics g, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbBottom.Normal, (int)state); return (visualStyleRenderer.GetPartSize(g, ThemeSizeType.True)); } private static void InitializeRenderer(VisualStyleElement element, int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(element.ClassName, element.Part, state); } else { visualStyleRenderer.SetParameters(element.ClassName, element.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Returns the size of a bottom pointing thumb. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TrackBarRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; const int lineWidth = 2; //cannot instantiate private TrackBarRenderer() { } ////// This is a rendering class for the TrackBar control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalTrack(Graphics g, Rectangle bounds) { InitializeRenderer(VisualStyleElement.TrackBar.Track.Normal, 1); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal track. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalTrack(Graphics g, Rectangle bounds) { InitializeRenderer(VisualStyleElement.TrackBar.TrackVertical.Normal, 1); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical track. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.Thumb.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a horizontal thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbVertical.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a vertical thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawLeftPointingThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbLeft.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a constant size left pointing thumb centered in the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawRightPointingThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbRight.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a constant size right pointing thumb centered in the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawTopPointingThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbTop.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a constant size top pointing thumb centered in the given bounds. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawBottomPointingThumb(Graphics g, Rectangle bounds, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbBottom.Normal, (int)state); visualStyleRenderer.DrawBackground(g, bounds); } ////// Renders a constant size bottom pointing thumb centered in the given bounds. /// ////// /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] // PM team has reviewed and decided on naming changes already [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawHorizontalTicks(Graphics g, Rectangle bounds, int numTicks, EdgeStyle edgeStyle) { if (numTicks <= 0 || bounds.Height <= 0 || bounds.Width <= 0 || g == null) { return; } InitializeRenderer(VisualStyleElement.TrackBar.Ticks.Normal, 1); //trivial case -- avoid calcs if (numTicks == 1) { visualStyleRenderer.DrawEdge(g, new Rectangle(bounds.X, bounds.Y, lineWidth, bounds.Height), Edges.Left, edgeStyle, EdgeEffects.None); return; } float inc = ((float)bounds.Width - lineWidth) / ((float)numTicks - 1); while (numTicks > 0) { //draw the nth tick float x = bounds.X + ((float)(numTicks - 1)) * inc; visualStyleRenderer.DrawEdge(g, new Rectangle((int)Math.Round(x), bounds.Y, lineWidth, bounds.Height), Edges.Left, edgeStyle, EdgeEffects.None); numTicks--; } } ////// Renders a horizontal tick. /// ////// /// [SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] // PM team has reviewed and decided on naming changes already [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static void DrawVerticalTicks(Graphics g, Rectangle bounds, int numTicks, EdgeStyle edgeStyle) { if (numTicks<=0 || bounds.Height <= 0 || bounds.Width<=0 || g == null ) { return; } InitializeRenderer(VisualStyleElement.TrackBar.TicksVertical.Normal, 1); //trivial case if (numTicks == 1) { visualStyleRenderer.DrawEdge(g, new Rectangle(bounds.X, bounds.Y, bounds.Width, lineWidth), Edges.Top, edgeStyle, EdgeEffects.None); return; } float inc = ((float)bounds.Height - lineWidth) / ((float)numTicks - 1); while (numTicks > 0) { //draw the nth tick float y = bounds.Y + ((float)(numTicks - 1)) * inc; visualStyleRenderer.DrawEdge(g, new Rectangle(bounds.X, (int)Math.Round(y), bounds.Width, lineWidth), Edges.Top, edgeStyle, EdgeEffects.None); numTicks--; } } ////// Renders a vertical tick. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetLeftPointingThumbSize(Graphics g, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbLeft.Normal, (int)state); return (visualStyleRenderer.GetPartSize(g, ThemeSizeType.True)); } ////// Returns the size of a left pointing thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetRightPointingThumbSize(Graphics g, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbRight.Normal, (int)state); return (visualStyleRenderer.GetPartSize(g, ThemeSizeType.True)); } ////// Returns the size of a right pointing thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetTopPointingThumbSize(Graphics g, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbTop.Normal, (int)state); return (visualStyleRenderer.GetPartSize(g, ThemeSizeType.True)); } ////// Returns the size of a top pointing thumb. /// ////// /// [SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // Using Graphics instead of IDeviceContext intentionally public static Size GetBottomPointingThumbSize(Graphics g, TrackBarThumbState state) { InitializeRenderer(VisualStyleElement.TrackBar.ThumbBottom.Normal, (int)state); return (visualStyleRenderer.GetPartSize(g, ThemeSizeType.True)); } private static void InitializeRenderer(VisualStyleElement element, int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(element.ClassName, element.Part, state); } else { visualStyleRenderer.SetParameters(element.ClassName, element.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns the size of a bottom pointing thumb. /// ///
Link Menu
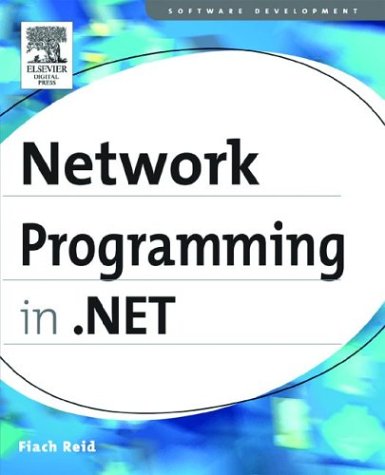
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebConfigurationFileMap.cs
- FixedNode.cs
- LocationFactory.cs
- WebPartConnectVerb.cs
- Monitor.cs
- SpellerInterop.cs
- DivideByZeroException.cs
- RefreshResponseInfo.cs
- Win32SafeHandles.cs
- SqlGenericUtil.cs
- BamlVersionHeader.cs
- DefaultValueAttribute.cs
- SqlDataSourceStatusEventArgs.cs
- BamlTreeNode.cs
- IdentityModelStringsVersion1.cs
- FamilyTypeface.cs
- TransformGroup.cs
- storepermissionattribute.cs
- XmlSchemaComplexContent.cs
- StringDictionary.cs
- FieldBuilder.cs
- LongAverageAggregationOperator.cs
- SyndicationCategory.cs
- OutputCacheSection.cs
- CustomErrorsSectionWrapper.cs
- IgnoreFileBuildProvider.cs
- PipeSecurity.cs
- WorkflowServiceOperationListItem.cs
- ConditionalDesigner.cs
- DataGridViewAccessibleObject.cs
- BitmapEffectInput.cs
- ContextMenuService.cs
- ProfileSettings.cs
- ParserStreamGeometryContext.cs
- ColorMatrix.cs
- CodeBinaryOperatorExpression.cs
- StringValidator.cs
- OleDbMetaDataFactory.cs
- Quad.cs
- ProfileProvider.cs
- FixUpCollection.cs
- X500Name.cs
- SqlDelegatedTransaction.cs
- __FastResourceComparer.cs
- Popup.cs
- Int16Storage.cs
- ConstNode.cs
- DataTableTypeConverter.cs
- SqlStatistics.cs
- GlyphInfoList.cs
- CodeGotoStatement.cs
- PackWebRequest.cs
- GeneralTransform3D.cs
- SQLGuid.cs
- ListViewItemEventArgs.cs
- SqlDataSourceView.cs
- UIElementCollection.cs
- StorageScalarPropertyMapping.cs
- ObjectDataSource.cs
- Repeater.cs
- webproxy.cs
- XmlElement.cs
- XmlNamespaceDeclarationsAttribute.cs
- VideoDrawing.cs
- ArrayList.cs
- HttpRequest.cs
- BaseDataListComponentEditor.cs
- ToolBarTray.cs
- MessagePropertyVariants.cs
- Binding.cs
- ProviderException.cs
- ExpressionVisitor.cs
- InputMethod.cs
- C14NUtil.cs
- QueryOutputWriter.cs
- SamlAuthenticationClaimResource.cs
- CheckPair.cs
- SurrogateEncoder.cs
- OleDbCommandBuilder.cs
- SafeFreeMibTable.cs
- PersistenceProviderBehavior.cs
- TextBoxBase.cs
- XmlFormatExtensionAttribute.cs
- RIPEMD160Managed.cs
- BmpBitmapDecoder.cs
- WebDisplayNameAttribute.cs
- dataprotectionpermissionattribute.cs
- CodeTypeDeclarationCollection.cs
- ContextDataSourceContextData.cs
- AudioDeviceOut.cs
- EdmComplexPropertyAttribute.cs
- ProgressChangedEventArgs.cs
- dbenumerator.cs
- CompilationSection.cs
- RelationalExpressions.cs
- DataGridTextColumn.cs
- HandlerWithFactory.cs
- DateTimeSerializationSection.cs
- AppliesToBehaviorDecisionTable.cs
- AnnotationResourceCollection.cs